Spring Boot Microservices and Spring Cloud
Learn to build Microservices with Spring Boot and Spring Cloud. Use Docker and publish to AWS.
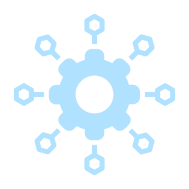
What you will learn?
- Build and run RESTful Microservices
- Implement User Authentication
- Eureka Discovery Service
- Implement User Authorization with Spring Security and JWT
- Spring Cloud API Gateway
- Learn to use JPA to persist data into a Database
- Cloud Cloud Config Server
- Learn to install MySQL Server and persist data into MySQL
- Spring Cloud Bus and Rabbit MQ
- H2 in-memory database and H2 Console
- Spring Boot Actuator
- Learn to use HTTP Postman
- Use Spring Security
- Learn to use Spring Initializer
- Distributed Tracing with Sleuth and Zipkin
- Learn to use Spring Tool Suite
- Centralized Logging with ELK Stack(Logstash, Elasticsearch, Kibana)
- Run Microservices in Docker Containers
Your trainer

Sergey Kargopolov
I have more than 20 years experience of building different types of software. I started my career as an Entrepreneur with an award winning web projects and currently I’m a Full Stack Mobile App Developer with passion for teaching beginner programmers to reach their goals and assisting them in development of their first mobile apps. If you Google my name you will find hundreds of video tutorials, technical articles and code examples that I’ve published over the years teaching how to build mobile apps for iOS and Android Platforms.
259 lessons
Easy to follow lectures and videos covering subject details.
19.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Source Code00:14
- Course Overview07:23
- A few suggestions03:29
- What is a Microservice?07:11
- Sample Microservices Architecture07:20
- Download and Install Postman HTTP Client04:48
- Postman Overview09:09
- Resource and Collection URIs11:12
- HTTP Methods: GET, POST, DELETE and PUT03:44
- HTTP Headers: Accept and Content Type04:12
- Install Java Platform(JDK)05:48
- Download and Install Spring Tool Suite(STS)03:26
- Introduction00:41
- Creating a New Project06:12
- Creating a new Spring project using Spring Boot Initializr04:42
- Create Users Rest Controller class02:45
- Adding Methods to Handle POST, GET, PUT, DELETE HTTP requests03:13
- Running Web Service Application04:36
- Reading Path Variables with @PathVariable annotaion03:31
- Reading Query String Request Parameters04:51
- Making Parameters Optional or Required04:35
- Returning Java Object as Return Value04:11
- Returning Object as JSON or XML Representation05:35
- Set Response Status Code04:58
- Reading HTTP POST Request Body. The @RequestBody annotation.08:13
- Validating HTTP POST Request Body08:56
- Store Users Temporary04:50
- Handle HTTP PUT Request09:06
- Handle HTTP Delete Request04:52
- Handle an Exception07:44
- Return Custom Error Message Object05:35
- Handle a Specific Exception03:06
- Throw and Handle You Own Custom Exception04:13
- Catch More Than One Exception with One Method02:54
- Dependency Injection: Create and Autowire a Service Layer Class10:54
- Constructor Based Dependency Injection06:24
- Run Web Service as a Standalone Application04:52
- Introduction to Eureka Discovery Service04:29
- Startup Eureka Service Discovery05:39
- Troubleshooting00:16
- Introduction to Building a Users Microservice02:10
- Users Microservice - Create new Spring Boot Project03:40
- Enable Eureka Client03:01
- Create Users Rest Controller02:04
- Access Users Web Service Endpoint via Eureka Discovery Service02:59
- Exercise - Create Account Management Microservice00:09
- Introduction to Building an Account Management Microservice01:34
- Create a new Spring Boot Project03:59
- Access Account Management Microservice via Eureka Discovery Service02:56
- Important note01:48
- Introduction to Zuul API Gateway03:24
- Create a ZUUL API Gateway Project03:37
- Access Microservices via API Gateway04:14
- Important note00:04
- Load Balancer - Introduction00:50
- Starting Up More Microservices08:58
- Trying How Load Balancer Works04:47
- Important Note01:43
- Introduction06:27
- Creating API Gateway Project05:04
- Automatic Mapping of Gateway Routes06:49
- Manually Configuring API Gateway Routes07:49
- Trying how it works04:08
- Rewriting URL Path05:06
- Automatic & Manual Routing01:57
- Build-In Predicate Factories03:30
- Gateway Filters00:10
- Starting Up More Microservices08:58
- Trying How Load Balancer Works03:53
- H2 In-memory Database. Introduction.03:19
- H2 Database Console Overview07:40
- Adding Support for the H2 Database04:40
- Introduction00:41
- Adding method to handle HTTP Post Request01:14
- Implementing the Create User Request Model class03:17
- Validating HTTP Request Body04:20
- Application Layers02:48
- Implementing Service Layer Class02:10
- Create a Shared DTO Class03:15
- Generate Unique Public User Id02:16
- Adding Support for Spring Data JPA02:01
- Implementing User Entity Class06:07
- Implementing Spring Data JPA CRUD Repository02:34
- Save User Details in Database11:15
- Return Http Status Code02:29
- Implementing Create User Response Model04:30
- Add Spring Security to Users Microservice01:15
- Add WebSecurity Configuration04:12
- Encrypt User Password04:50
- Allow only IP address of Zuul API Gateway02:15
- Adding Support to Return JSON or XML04:59
- Introduction01:10
- Implementing LoginRequestModel01:21
- AuthenticationFilter. Implementing attemptAuthentication()06:03
- Implementing loadUserByUserName()11:37
- AuthenticationFilter. Implementing successfulAuthentication().13:12
- Trying How User Login Works05:45
- Customize User Authentication URL03:30
- Important note00:03
- Introduction to Spring Security on API Gateway01:53
- Adding Support for Spring Security and JWT Tokens01:17
- Enable Web Security in Zuul04:29
- Allow Access to Registration and Login Urls05:06
- Implementing Authorization Filter08:43
- Accessing Protected Microservices with Access Token05:22
- Introduction03:10
- Using Header Predicate04:04
- Adding Support for JWT Token Validation01:51
- Creating AuthorizationFilter class03:49
- Assign Custom Filter to a Gateway Route01:12
- Signup and Login Routes03:05
- Reading Authorization HTTP Header07:42
- Validating JWT Access Token07:27
- Accessing Protected Microservices with Access Token06:39
- Introduction01:02
- Creating Global Pre Filter03:51
- Accessing Request Path and HTTP Headers03:32
- Trying how Pre Filter Works03:49
- Creating Global Post Filter03:05
- Trying how the Post Filter works02:38
- Defining Filters in a Single Class06:41
- Ordering Global Filters06:42
- Trying how ordered filters work02:22
- Introduction to Spring Cloud Config Server04:03
- Create Your Own Config Server02:53
- Create Private GitHub Repository02:24
- Naming Property Files Served by Config Server02:32
- Configure Config Server to Access Private GitHub Repository02:46
- Adding Properties File to Git Repository03:16
- Configure Users Microservice to be a Client of Config Server07:24
- Make Zuul Gateway a Client of Config Server02:18
- Introduction to Spring Cloud Bus03:52
- Add Spring Cloud Bus & Actuator Dependencies02:27
- Enable the /bus-refresh URL Endpoint01:47
- Download and Run Rabbit MQ03:20
- Rabbit MQ Default Connection Details02:15
- Trying how Spring Cloud Bus Works08:37
- Change default Rabbit MQ Password03:29
- Introduction to Spring Cloud Config File System as a Backend00:45
- Setting up File System Backend04:51
- Previewing Values Returned by Config Server01:41
- Trying how Microservices work02:38
- Introduction02:31
- Shared and a Microservice specific properties07:01
- Introduction to Spring Boot Actuator02:15
- Add Spring Boot Actuator to API Gateway04:59
- Trying How It Works05:02
- Enable Actuator for Users Microservice05:51
- Introduction01:00
- Download and Install MySQL03:16
- Start MySQL Server and Login06:14
- Create MySQL Database And a New User06:25
- Downloading and Installing MySQL Workbench03:02
- Connect to MySQL Database using MySQL WorkBench05:26
- MySQL WorkBench brief overview04:15
- Configure MySQL Database Access Details09:48
- Use H2 Console to Access MySQL Database02:48
- Copy MySQL properties to a Config Server01:40
- Introduction to Encryption and Decryption of Configuration Properties02:43
- A note about Java Cryptography Extension(JCE)00:23
- Add Java Cryptography Extension06:14
- Symmetric Encryption of Properties08:50
- Creating a Keystore for Asymmetric Encryption05:05
- Asymmetric Encryption of Properties05:25
- Introduction to Microservices Communication03:48
- Albums Microservices Source Code00:08
- Clone Source Code of Albums Microservice02:11
- A walk through an Albums Microservice05:37
- Implementing Get User Details05:43
- Make Users Microservice call Albums Microservice10:25
- Trying how it works03:59
- Feign Web Service Client - Introduction01:32
- Enable Feign in Spring Boot Project01:54
- Create Feign Client05:07
- Using Feign Client01:47
- Trying How Feign Client Works03:11
- Enable HTTP Requests Logging in Feign Client05:41
- Handle FeignException04:36
- Handle Response Errors with Feign Error Decoder12:57
- Introduction02:55
- Configure Project to use Hystrix Circuit Breaker04:28
- Trying How Hystrix Circuit Breaker & Feign work03:10
- Error Handling with Feign Hystrix FallbackFactory11:05
- Introduction07:54
- Removing Hystrix Circuit Breaker02:41
- Adding Resilience4j03:16
- Actuator /health Endpoint02:32
- Feign Client & Circuit Breaker Fallback method04:22
- Circuit Breaker configuration properties07:04
- Configure Access to Actuator endpoints04:50
- Monitoring Circuit Breaker events in Actuator07:26
- @Retry annotation01:36
- Aspect Order03:12
- Configuration properties03:02
- Trying how it works03:52
- Introduction to Distributed Tracing with Sleuth and Zipkin03:07
- Add Spring Cloud Sleuth to Users Microservice04:12
- Checking Trace ID and Span ID in a Console05:26
- Startup Zipkin Server02:18
- View Traces in Zipkin05:14
- Introduction to Aggregating Log Files with ELK Stack02:04
- Configure Microservices to Log into a File02:55
- Download Logstash01:39
- Configure Logstash to Read Log Files09:11
- [New]Download and Run Elasticsearch with Security Enabled05:56
- [New]Configure Elasticsearch Security in Logstash05:04
- Run Search Query06:50
- [Updated]Download, Install and Run Kibana04:58
- [Updated]View Aggregated Logs in Kibana05:31
- Configure Spring Security to Eureka Server02:15
- Enable Web Security02:40
- Configure Eureka Clients to use Username and Password03:10
- Configure Eureka Service URL in Config Server04:19
- Move Username and Password to Config Server04:00
- Encrypting Username and Password06:03
- Introduction to Running Microservices in Docker Containers07:59
- Start up a new Linux Server on AWS EC214:56
- Connect to EC2 Instance04:46
- Docker Commands Used in this Video Course00:57
- Install Docker on AWS EC201:51
- Docker Hub Introduction01:57
- Run RabbitMQ Docker Container09:12
- Basic Docker Commands: Run, Stop, Start, Remove Containers and Images06:49
- Create Config Server Docker Image09:48
- Publish Config Server Docker Image to Docker Hub04:27
- Run Config Server on EC2 from Docker Hub10:22
- Start New EC2 Instance for Eureka03:23
- Build Docker Image for Eureka Discovery Service04:01
- Run Eureka in Docker container11:20
- Elastic IP address for EC2 Instance04:41
- Create Zuul Api Gateway Docker Image04:41
- Run Zuul Api Gateway in Docker Container08:03
- Run Elastic Search in Docker container05:55
- Run Kibana in Docker Container06:34
- Run Kibana and Elastic Search on the same Network04:31
- Docker Image for Albums Microservice04:27
- Run Albums Microservice Docker Image on EC210:49
- Logstash Docker Image for Albums Microservice08:50
- Run Logstash in Docker container04:34
- Run MySQL in Docker Container08:37
- Make MySQL Docker Container Persist Data on EC203:19
- Build Users Microservice Docker Image04:22
- Run Users Microservice in Docker container09:59
- Run Logstash for Users Microservice07:51
- Introduction05:09
- Preparing Configuration for another environment08:31
- Creating Beans Based on Spring Boot @Profile used11:32
- Running Docker Container for Different Environments01:02
- Introduction06:30
- Pass Authorization Header to Downstream Microservice01:22
- Add AuthorizationFilter to Users Microservice06:01
- Configure HttpSecurity02:30
- Trying how it works03:11
- Introduction to Method-Level security04:55
- Enable Method-Level Security02:00
- @PreAuthorize annotation example03:30
- Trying how @PreAuthorize annotation works03:02
- @PostAuthorize annotation example07:11
- Bonus lecture01:44
Online Courses
Learning Microservices doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Microservices related books and take your
YouTube videos
The number of high-quality and free Microservices video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more