Master Laravel 8 for Beginners & Intermediate
Get from zero to proficiency in the Laravel Framework! Course for beginners and intermediate students!
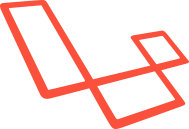
What you will learn?
- Eloquent - ORM for interacting with the database
- Advanced features like Queues, Polymorphic relationships, Service Container
- Learn all the theory while building a real application as you progress!
- Setting up PHP with Apache on Windows and Mac
- How to use Visual Studio Code effectively
- Creating APIs, serializing data, API resources and API testing
- Routes and Controllers
- Laravel Tinker - command line playground to Laravel
- Blade templates
- Blade componenets
- Creating Forms, CSRF tokens
- One to One, One to Many, Many to Many relationships
- Polymorphic relationships
- Testing
- Local and Global Eloquent Query Scopes
- Database migrations
- Database seeding and factories
- Authentication (Guard)
- Authorization (Policies and Gates)
- Authorization
- Caching
- How to use queues
- Files and file uploads
- How to send e-mails
- Observers, Events, Listeners and Subscribers
- Localization
- Services, Service Container, Contracts and Facades
- Using Traits in Laravel - SoftDeletes and creating your own!
289 lessons
Easy to follow lectures and videos covering subject details.
33 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome to the Course!02:09
- About Laravel Versions (OPTIONAL)01:48
- Setup Recommendations and Course Prerequisites03:51
- Code Editor: Visual Studio Code Setup and Recommendations05:59
- Course Source Code02:23
- Additional Learning Resource (Cheat Sheet, Diagrams)01:41
- Windows - Setting Up XAMPP (PHP/Apache/MySQL)03:15
- Windows - PHP in the Command Line02:36
- Windows - Composer01:53
- Mac - Setting Up XAMPP (PHP/Apache/MySQL)02:58
- Mac - PHP in the Command Line04:26
- Mac - Composer01:38
- Creating New Laravel Project Using Composer01:19
- Windows - Setting Up Virtual Host in Apache04:21
- Windows - Setting Up Host on the System01:41
- Mac - Setting Up Virtual Host in Apache03:19
- Mac - Setting Up Host on the System02:16
- Laravel Project Structure Overview03:24
- Artisan Command03:10
- Understanding Routing in Laravel01:56
- Defining Routes03:04
- Managing and Naming Routes02:53
- Route Parameters03:26
- Optional Route Parameters03:39
- Constraining Possible Route Parameters Values04:11
- Understanding Templating, Views and Blade05:26
- Template Inheritance and Layouts07:48
- Passing and Rendering Data in Templates04:57
- Simple View Rendering Routes02:05
- Conditional Rendering03:28
- Conditional Rendering Alternatives03:26
- Loops in Templates06:20
- More Control Inside Loops06:15
- Partial Templates (Including Templates)04:10
- Partial Templates in Loops02:51
- Responses, Codes, Headers, and Cookies04:57
- Redirect Responses04:18
- Returning JSON01:20
- Returning File Downloads02:28
- Grouping Routes03:22
- Request Input (Reading User Input)04:31
- Request Input - An Overview of Options01:44
- Middleware - Running Code Before & After Request04:19
- Controllers05:08
- Single Action Controllers01:56
- Resource Controllers01:31
- Implementing a Resource Controller05:39
- Configuration and Environments Overview03:46
- Configuring the Database Connection03:27
- Databases Bird's Eye Overview01:45
- Migrations Overview02:40
- Creating and Running Migrations05:05
- Understanding Eloquent ORM Models02:08
- Models - Creating and Updating, Introducing Tinker03:19
- Models - Retrieving Single Model02:11
- Models - Retrieving Multiple Models and Collections Overview02:16
- Models - Using the Query Builder04:14
- Practical - List of Blog Posts and Single Blog Post03:21
- Forms Markup04:14
- Cross Site Request Forgery Explained04:29
- Forms - Storing Submitted Data03:36
- Forms - Input Validation04:01
- Forms - Displaying Validation Errors02:50
- Forms - Form Request Classes03:21
- Session Flash Messages02:15
- Forms - Old Input Helper01:29
- Forms/Models - Model Mass Assignment05:16
- CRUD - Edit Form08:57
- CRUD - Update Action03:39
- CRUD - Deleting Using Forms04:51
- CRUD - Deleting Models03:01
- Introduction to Laravel Mix03:30
- Installing Bootstrap CSS Framework02:14
- Using NPM and Compiling Assets With Mix/Webpack03:29
- Including Assets in Views01:30
- Versioned Assets (Cache Improvements)02:57
- Introduction to Bootstrap CSS02:13
- Understanding CSS Flexbox in General and in Bootstrap03:23
- Layout Grid and Styling Header Bar08:03
- Styling Forms02:55
- Styling Post List Page02:56
- Styling Single Post Page03:13
- Styling Flash Messages and Error Messages01:54
- Testing07:03
- Testing configuration and environment04:49
- Writing first functional test07:17
- Testing database interactions08:13
- Testing store() action (model creation)05:34
- Testing for failure07:41
- Testing update() action (model updates)07:20
- Testing delete() action (model deletion)05:19
- One to One relation with migration08:47
- One to One assigning relationship08:44
- One to One querying relationship07:38
- One to Many relation with migration07:22
- One to Many assigning relationship05:58
- One to Many querying relationship05:24
- Lazy Loading vs Eager Loading07:20
- Querying relationship existence07:43
- Querying relationship absence03:56
- Counting related models05:11
- Using withCount() in practice (fetching count of comments) with test05:12
- Model Factory introduction12:05
- Model Factory states14:53
- Model Factory callbacks (afterCreating, afterMaking)08:40
- Application: Implementing comment list06:51
- IMPORTANT: Laravel 7 and Laravel 8 Changes00:14
- Authentication overview12:25
- How user registration works in Laravel15:38
- Guard component and how logging users in works16:57
- Custom registration form and Auth routes07:45
- Formatting validation errors07:49
- RedirectIfAuthenticated middleware03:15
- Log-in form with "Remember Me" feature07:12
- Logging out, @guest directive, debugging CSRF token errors11:42
- Retrieving the currently authenticated user04:31
- Protecting routes (requiring authentication)05:12
- Testing routes that require authentication10:20
- Refreshing database, database foreign keys and existing data14:45
- Problem: SQLite test database NOT NULL problem02:31
- Database seeding basics05:37
- Using Model Factory inside Seeder05:43
- Model relations inside seeder10:20
- Individual seeder classes08:51
- Making seeder interactive11:52
- Deleting related model using model events06:03
- Deleting related models using cascading05:22
- Soft deletes04:58
- Soft deletes querying06:43
- Restoring soft deleted model10:42
- Testing soft deleted models03:05
- Authorization introduction06:14
- Introduction to Gates08:47
- Using authorize() helper02:51
- Verifying permissions of the user04:12
- Admin users and overriding permissions13:10
- Policies introduction11:37
- Policy or Gate?14:01
- Verifying permissions in Blade templates05:45
- Using middleware to authorize routes10:37
- Application: updating tests06:24
- Application: setting user_id for the new BlogPost03:48
- Global Query Scopes introduction09:05
- Global Query Scopes and potential issues10:39
- Local Query Scopes introduction10:00
- Practical: Local Query Scope - most commented posts09:55
- Practical: Local Query Scope - most active users10:04
- Practical: Local Query Scope - most active users last month12:16
- Practical: Global Query Scope - admin can see deleted posts15:30
- Blade Components introduction09:33
- Component aliases03:33
- Conditional rendering in Component05:31
- Practical: creating reusable component for dates08:19
- Complicated example of conditional rendering10:10
- Application: Fixing an issue with HAVING clause02:07
- Caching introduction08:01
- Laravel Debugbar10:31
- Storing data in cache11:15
- Removing from cache07:17
- Cache facade04:03
- Practical: using cache as storage06:45
- Practical: using cache for storage implementation13:25
- Using and setting up Redis as cache storage10:26
- Cache tags introduction06:10
- Practical: using cache tags08:27
- ManyToMany introduction02:39
- ManyToMany migration05:37
- Defining ManyToMany on models03:36
- Associating models in ManyToMany10:35
- Querying the ManyToMany relation and Pivot tables07:30
- Practical: displaying the list of tags using Blade component06:16
- Practical: list of blog posts by tag10:21
- Blade View Composers08:39
- View Composer with @include07:59
- ManyToMany seeding14:04
- Practical: User to Comment OneToMany relation and migration07:01
- Practical: comments form and reusable errors component09:05
- Route Model Binding13:33
- Eager loading nested relationships08:31
- Converting repeating queries to query scopes03:37
- File Storage introduction09:48
- File upload form02:17
- Handling file uploads09:06
- Using Storage facade to store files10:41
- Getting the URL of stored file10:02
- Practical: Image model, OneToOne relation and migrations08:22
- Uploaded image URL06:34
- Practical: Displaying uploaded image and styling05:19
- Deleting files05:06
- Validating uploaded files (size, type, dimensions)06:19
- Section intrduction02:13
- Practical: Scaffolding UserController and UserPolicy, using authorizeResource12:13
- Practical: Views for showing/editing user profile13:47
- OneToOne Polymorphic explained04:05
- OneToOne Polymorphic migration06:27
- OneToOne Polymorphic defining relation02:42
- OneToOne Polymorphic associating04:02
- Practical: OneToOne Polymorphic with BlogPost and Image13:02
- OneToMany Polymorphic explained02:03
- OneToMany Polymorphic migration & relation06:15
- OneToMany Polymorphic associating09:55
- Practical: OneToMany Polymorphic views14:21
- Practical: Running tests on MySQL database07:28
- OneToMany Polymorphic seeder07:17
- ManyToMany Polymorphic explained05:48
- ManyToMany Polymorphic migration14:52
- ManyToMany Polymorphic relations05:12
- Understanding model Traits04:20
- Creating Taggable model trait19:15
- Development setup for sending emails06:21
- The Mailable class explained10:18
- Rendering e-mail content and e-mail sending10:27
- Attaching files & data to e-mails10:39
- Embedding an image inside the e-mail05:22
- Markdown Mailable classes explained04:55
- Creating the Markdown Mailable class09:22
- Custom Markdown e-mail component and styling07:08
- Rendering e-mail previews in browser02:11
- Queues and background processing introduction08:13
- Configuring queues, creating and running the first job10:07
- Optional e-mail queuing and execution delay05:18
- Dealing with failed jobs09:54
- Creating and dispatching custom jobs06:07
- Implementing custom job that dispatches other jobs17:04
- Rate Limiting queues16:04
- Named queues and prioritizing06:14
- Model Observers11:06
- Events and Listeners08:52
- Practical: Custom Event and Listener10:30
- Second example of Event and Listener09:51
- Logging basics in Laravel07:09
- Handling built-in Laravel events with Subscriber08:21
- Localization introduction and demo04:41
- Configuring locale and translation overview04:40
- Translating plural forms, passing data13:47
- Storing translations in JSON05:50
- Translating the application07:47
- Storing user preffered language in database11:37
- Creating custom Locale Middleware10:52
- Adding language to URL through Apache configuration09:33
- What is a Service and creating a custom one07:07
- Service Container in practice09:56
- Basic Dependency Injection08:15
- Dependency Injection and Contracts12:16
- Contracts explained10:43
- Facades explained07:47
- Postman - a quite long introduction16:48
- How Model serialization works05:58
- Hiding model attributes04:48
- Serializing model relations05:45
- API Resources introduction09:35
- Serializing model relations using a Resource class04:33
- Limiting serialization only to eager loaded relations03:01
- Conditional serialization of properties03:23
- API routes and controllers14:40
- Practice defining API routes16:48
- Returning a resource collection and response wrapping04:58
- Collection pagination03:50
- Collection pagination and custom parameters04:23
- Storing a new resource07:38
- API Tokens explained and implemented15:32
- Returning one, updating and deleting resources08:55
- Handling 404 (resource not found)09:38
- API Authorization16:18
- Testing API GET methods, verifying JSON structure11:24
- Testing API GET methods, verifying JSON structure II08:08
- Test API storing (POST) resources, authentication and validation10:17
- Laravel 8 Breaking Changes!00:21
- Routes, Views & Layouts13:48
- View Routes, Route Parameters, Rendering Data14:33
- Named routes and generating URLs06:29
- Controller basics10:06
- Database configuration and environment variables08:40
- Introduction to database migrations09:26
- Creating and managing migrations12:44
- Laravel Tinker and Eloquent models11:49
- Creating, updating and reading data and making simple database queries12:36
- Resource controllers11:56
- Blade directives @if, @foreach, dates18:12
- Forms and CSRF tokens12:52
- Redirects03:09
- Session flash messages04:33
- Validation basics05:57
- Displaying validation errors07:04
- Form requests06:01
- Mass assignment in Eloquent model04:28
- old() helper in forms03:03
- Creating data editing forms11:37
- Bonus!00:04
Online Courses
Learning Laravel doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Laravel related books and take your
YouTube videos
The number of high-quality and free Laravel video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more