The Modern JavaScript Bootcamp
Learn JavaScript by building real-world apps. Includes 3 real-world projects, 80 programming challenges, and ES6/ES7!
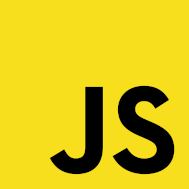
What you will learn?
- Learn JavaScript by building three real-world web applications
- Gain a deep understanding of how JavaScript works behind the scenes
- Explore the latest cutting-edge features from ES6 and ES7
- Test your skills and gain confidence by completing over 80 coding challenges
- Learn how to deploy your application to the web so you can share them with everyone
- Learn how to use Promises and Async/Await with asynchronous JavaScript
- Get more done by learning how to debug and fix your code when things go wrong
- Get access to a free 80 page PDF guide with lecture notes, code samples, and documentation links
Your trainer

Andrew Mead
I'm Andrew, a full-stack developer living in beautiful Philadelphia!
I launched my first Udemy course in 2014 and had a blast teaching and helping others. Since then, I've launched 3 courses with over 110,000 students and over 18,000 5-star reviews.
I currently teach JavaScript, React, and Node.
Before I ever heard about Udemy or thought about teaching, I created a web app development company. I've helped companies of all sizes launch production web applications to their customers. I've had the honor of working with awesome companies like Siemens, Mixergy, and Parkloco.
I have a Computer Science degree from Temple University, and I've been programming for just over a decade. I love creating, programming, launching, learning, teaching, and biking.
155 lessons
Easy to follow lectures and videos covering subject details.
29.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome!07:28
- Grab the PDF Guide01:16
- Section Intro: Setting up Your Computer01:03
- Installing Visual Studio Code05:25
- Installing Node.js02:43
- [Windows Only] Install cmder02:14
- Introduction to the Terminal07:52
- Hello JavaScript!05:31
- Section Intro: JavaScript Basics00:58
- Strings and Variables17:05
- Numbers13:40
- More on Variables06:09
- Build a Temperature Converter06:11
- Booleans and Comparison Operators13:28
- If Statements10:18
- Advanced If Statements12:01
- Logical "And" and "Or" Operators14:53
- Variable Scope: Part I10:38
- Variable Scope: Part II10:14
- Section Intro: JavaScript Functions01:31
- Function Basics16:15
- Undefined and Null09:54
- Multiple Arguments and Argument Defaults15:46
- Function Scope04:31
- Template Strings12:58
- Build a Grade Calculator13:12
- Section Intro: JavaScript Objects01:17
- Object Basics13:02
- Using Objects with Functions11:30
- Object References08:49
- Build an Expense Tracker09:52
- Methods13:05
- Exploring String Methods17:02
- Exploring Number Methods13:50
- Constant Variables08:10
- Bonus: Variables with var11:26
- Section Intro: JavaScript Arrays01:18
- Array Basics13:21
- Manipulating Arrays with Methods13:17
- Looping Over Arrays09:21
- The For Loop16:37
- Searching Arrays: Part I14:49
- Searching Arrays: Part II20:41
- Filtering Arrays17:45
- Sorting Arrays18:13
- Improve Our Expense Tracker18:58
- Section Intro: Javascript in the Browser01:24
- Setting up a Web Server15:12
- JavaScript in the Browser08:14
- DOM Manipulation12:13
- DOM Challenge11:13
- Adding Elements via the DOM14:25
- Handling User Interaction12:33
- Advanced Queries13:35
- Text Inputs and Live Data Filtering12:44
- Rendering Our Filtered Data12:35
- Todo Filter Challenge09:58
- Working With Forms17:20
- Checkboxes18:25
- Dropdowns08:00
- Section Intro: Data Storage, Libraries, and More00:42
- Saving Our Data in LocalStorage: Part I13:44
- Saving Our Data in LocalStorage: Part II11:23
- Splitting up Our Application Code13:36
- Refactor Challenge10:18
- Debugging Our Applications08:38
- Complex DOM Rendering14:16
- Setting up a Third-Party Library13:38
- Targeting by UUID10:41
- Checkbox Challenges08:48
- The Edit Note Page: Part I16:40
- The Edit Note Page: Part II18:03
- Syncing Data Across Pages13:09
- JavaScript Dates18:10
- Moment19:50
- Integrating Dates: Part I14:02
- Integrating Dates: Part II12:12
- Take a Break01:12
- Section Intro: Expanding Our JavaScript Knowledge01:33
- Arrow Functions: Part I12:21
- Arrow Functions: Part II16:57
- Conditional (Ternary) Operator14:27
- Truthy and Falsy Values17:03
- Type Coercion11:00
- Catching and Throwing Errors15:27
- Handling Application Errors05:20
- Working in Strict Mode08:29
- Section Intro: Advanced Objects and Functions01:09
- Object Oriented Programming06:40
- Constructor Functions15:32
- Setting up the Prototype Object13:45
- Hangman Challenge: Part I09:10
- Digging Into Prototypical Inheritance09:51
- Primitives and Objects: Part I16:20
- Primitives and Objects: Part II13:36
- Hangman Challenge: Part II13:30
- Hangman Challenge: Part III20:42
- Hangman Challenge: Part IV08:08
- The Class Syntax11:40
- Creating Subclasses19:56
- Getters and Setters18:29
- Update: Fixing an Edge Case03:06
- Section Intro: Asynchronous JavaScript01:21
- HTTP Requests from JavaScript17:27
- HTTP Headers and Errors14:53
- Exploring Another API11:31
- Callback Abstraction17:03
- Asynchronous vs. Synchronous Execution15:06
- Callback Abstraction Challenge07:15
- Closures17:16
- Exploring Promises16:01
- Converting to Promises11:58
- Promise Chaining15:13
- The Fetch API14:54
- A Fetch Challenge06:46
- A Promise Challenge12:16
- Async/Await17:36
- Async/Await Challenge10:29
- Integrating Data into the Application09:02
- Section Intro: App Themes01:00
- CSS at a Glance13:35
- Setting up the Hangman Theme18:13
- Setting up the Notes Theme: Part I18:27
- Setting up the Notes Theme: Part II16:00
- Setting up the To-Do Theme: Part I10:44
- Setting up the To-Do Theme: Part II17:15
- Hosting Your Applications14:01
- Section Intro: Cutting-Edge JavaScript with Babel and Webpack01:17
- The Problem: Cross-Browser Compatibility03:30
- Exploring Babel17:20
- Setting up Our Boilerplate14:17
- Avoiding Global Modules04:41
- Exploring Webpack05:41
- Setting up Webpack13:21
- JavaScript Modules: Part I14:54
- JavaScript Modules: Part II09:29
- Adding Babel into Webpack07:15
- Webpack Dev Server10:22
- Environments and Source Maps08:32
- Converting Hangman App18:00
- Using Third-Party Libraries12:23
- Converting Notes App: Part I07:53
- Converting Notes App: Part II13:21
- Converting Notes App: Part III14:13
- Converting Notes App: Part IV24:41
- To-Do App Conversion Setup11:56
- Converting To-Do App: Part I16:07
- Converting To-Do App: Part II13:44
- The Rest Parameter11:04
- The Spread Syntax11:47
- The Object Spread Syntax08:41
- Destructuring20:04
- Section Intro: Wrapping Up01:28
- New App Idea04:26
- Bonus: Where do I go from here?06:39
Online Courses
Learning JavaScript doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of JavaScript related books and take your
YouTube videos
The number of high-quality and free JavaScript video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more