JavaScript: Understanding the Weird Parts
An advanced JavaScript course for everyone! Scope, closures, prototypes, 'this', build your own framework, and more.
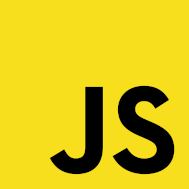
What you will learn?
- Grasp how Javascript works and it's fundamental concepts
- Write solid, good Javascript code
- Understand advanced concepts such as closures, prototypal inheritance, IIFEs, and much more.
- Drastically improve your ability to debug problems in Javascript.
- Avoid common pitfalls and mistakes other Javascript coders make
- Understand the source code of popular Javascript frameworks
- Build your own Javascript framework or library
Your trainer

Anthony Alicea
Tony has been programming since he was 12 years old, and got into web sites and web application development at 16. After graduating with a Computer Science degree from Case Western Reserve University, Tony continued with that interest as a Microsoft certified software application developer and architect, database designer, and user interface designer.
His experience has ranged across technologies such as HTML5, CSS3, ASP .NET MVC, JavaScript, jQuery, KnockoutJS, AngularJS, NodeJS, LESS, Bootstrap, SQL, Entity Framework and more.
He believes strongly that deeply understanding any topic allows you to properly learn it and, even more importantly in a real-world environment, quickly overcome problems.
89 lessons
Easy to follow lectures and videos covering subject details.
12 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction and The Goal of This Course04:56
- Setup03:27
- Setup (Visual Studio Code)00:25
- Big Words and Javascript01:25
- Watching this Course in High Definition00:50
- Understanding, Frameworks, and The Weird Parts04:17
- Conceptual Aside: Syntax Parsers, Execution Contexts, and Lexical Environments07:35
- Conceptual Aside: Name/Value Pairs and Objects04:08
- Downloading Source Code for This Course00:38
- The Global Environment and The Global Object10:58
- The Execution Context - Creation and Hoisting09:43
- Conceptual Aside: Javascript and 'undefined'08:04
- The Execution Context - Code Execution02:54
- Conceptual Aside: Single Threaded, Synchronous Execution02:16
- Function Invocation and the Execution Stack08:06
- Functions, Context, and Variable Environments07:56
- The Scope Chain17:25
- Scope, ES6, and let04:18
- What About Asynchronous Callbacks?10:26
- Conceptual Aside: Types and Javascript02:50
- Primitive Types05:17
- Conceptual Aside: Operators06:32
- Operator Precedence and Associativity14:14
- Operator Precedence and Associativity Table00:18
- Conceptual Aside: Coercion06:14
- Comparison Operators19:42
- Equality Comparisons Table00:18
- Existence and Booleans07:24
- Default Values07:51
- Framework Aside: Default Values07:11
- Objects and the Dot15:23
- Objects and Object Literals10:32
- Framework Aside: Faking Namespaces08:07
- JSON and Object Literals07:17
- Functions are Objects08:02
- Function Statements and Function Expressions20:32
- Conceptual Aside: By Value vs By Reference16:09
- Objects, Functions, and 'this'21:26
- Conceptual Aside: Arrays - Collections of Anything05:39
- 'arguments' and spread12:00
- Framework Aside: Function Overloading04:43
- Conceptual Aside: Syntax Parsers02:25
- Dangerous Aside: Automatic Semicolon Insertion05:46
- Framework Aside: Whitespace04:25
- Immediately Invoked Functions Expressions (IIFEs)17:07
- Framework Aside: IIFEs and Safe Code08:05
- Understanding Closures11:09
- Understanding Closures - Part 219:20
- Framework Aside: Function Factories12:24
- Closures and Callbacks08:26
- call(), apply(), and bind()20:54
- Functional Programming20:17
- Functional Programming - Part 208:05
- Conceptual Aside: Classical vs Prototypal Inheritance05:11
- Understanding the Prototype14:02
- Everything is an Object (or a primitive)05:46
- Reflection and Extend14:59
- Function Constructors, 'new', and the History of Javascript15:53
- Function Constructors and '.prototype'10:24
- Dangerous Aside: 'new' and functions04:16
- Conceptual Aside: Built-In Function Constructors10:32
- Dangerous Aside: Built-In Function Constructors03:57
- Dangerous Aside: Arrays and for..in03:16
- Object.create and Pure Prototypal Inheritance12:37
- ES6 and Classes06:27
- Initialization05:41
- 'typeof' , 'instanceof', and Figuring Out What Something Is06:41
- Strict Mode05:51
- Strict Mode Reference00:21
- Learning From Other's Good Code03:51
- Deep Dive into Source Code: jQuery - Part 121:31
- Deep Dive into Source Code: jQuery - Part 215:38
- Deep Dive into Source Code: jQuery - Part 311:22
- Requirements02:57
- Structuring Safe Code03:07
- Our Object and Its Prototype09:17
- Properties and Chainable Methods16:58
- Adding jQuery Support05:22
- Good Commenting02:21
- Let's Use Our Framework08:11
- A Side Note00:32
- TypeScript, ES6, and Transpiled Languages04:33
- Transpiled Languages References00:38
- Existing and Upcoming Features00:54
- ES6 Features Reference00:12
- ES6 In-Depth00:07
- Promises, Async, and Await42:43
- Learning to Love the Weird Parts01:14
- Bonus00:41
Online Courses
Learning JavaScript doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of JavaScript related books and take your
YouTube videos
The number of high-quality and free JavaScript video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more