Modern JavaScript From The Beginning
Learn and build projects with pure JavaScript (No frameworks or libraries)
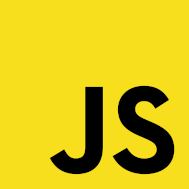
What you will learn?
- Modular learning sections & 10 real world projects with pure JavaScript
- Master the DOM (document object model) WITHOUT jQuery
- Asynchronous programming with Ajax, Fetch API, Promises & Async / Await
- OOP including ES5 prototypes & ES2015 classes
- Learn JavaScript Patterns
- Regular expressions, error handling, localStorage & more
Your trainer

Brad Traversy
Brad Traversy has been programming for around 12 years and teaching for almost 5 years. He is the owner of Traversy Media which is a successful web development YouTube channel and specializes in everything from HTML5 to front end frameworks like Angular as well as server side technologies like Node.js, PHP and Python. Brad has mastered explaining very complex topics in a simple manner that is very understandable. Invest in your knowledge by watching Brad's courses.
122 lessons
Easy to follow lectures and videos covering subject details.
21.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome To The Course05:41
- Project Files & Questions03:06
- Projects Github Repo00:09
- Visual Studio Code Setup10:04
- Section Intro & File Setup04:21
- Using The Console09:01
- Variables - var, let & const12:45
- Data Types in JavaScript10:19
- Type Conversion11:43
- Numbers & The Math Object07:18
- String Methods & Concatenation14:20
- Template Literals10:07
- Arrays & Array Methods15:59
- Object Literals07:49
- Dates & Times09:13
- If Statements & Comparison Operators19:02
- Switches04:49
- Function Declarations & Expressions11:44
- General Loops16:41
- A Look At The Window Object20:51
- Block Scope With let & const06:31
- What Is The DOM?03:16
- Examining The Document Object15:19
- DOM Selectors For Single Elements14:12
- DOM Selectors For Multiple Elements14:25
- Traversing The DOM15:48
- Creating Elements06:47
- Removing & Replacing Elements10:56
- Event Listeners & The Event Object10:12
- Mouse Events11:03
- Keyboard & Input Events12:42
- Event Bubbling & Delegation13:45
- Local & Session Storage15:14
- Task List [Part 1] - UI & Add Task Items15:52
- Task List [Part 2] - Delete & Filter Tasks14:04
- Task List [Part 3] - Persist To Local Storage11:03
- Loan Calculator [Part 1] - Build The UI15:07
- Loan Calculator [Part 2] - Calculate & Error16:47
- Loan Calculator [Part 3] - Loader & User Experience06:18
- Number Guesser [Part 1] - Build The UI07:00
- Number Guesser [Part 2] - Validation & Winning Case16:30
- Number Guesser [Part 3] - Lose Case & Game Over10:14
- Number Guesser [Part 4] - Play Again10:53
- Constructors & the 'this' Keyword11:56
- Built In Constructors12:16
- Prototypes Explained09:54
- Prototypal Inheritance08:23
- Using Object.create05:28
- ES6 Classes08:35
- Sub Classes05:46
- Build The Book List UI08:20
- Add Book To List12:32
- Validation & Alert08:28
- Delete Book From List06:29
- Convert to ES6 Classes06:06
- Bonus - Add Local Storage14:43
- What Is Asynchronous Programming?03:41
- Ajax & XHR Introduction06:00
- XHR Object Methods & Working With Text12:50
- Working With Ajax & JSON14:23
- Data From an External API - Chuck Norris Project14:56
- REST APIs & HTTP Requests06:33
- Callback Functions08:27
- Custom HTTP Library (Ajax With Callbacks) - Part 115:26
- Custom HTTP Library (Ajax With Callbacks) - Part 212:12
- ES6 Promises05:39
- The Fetch API12:40
- Error Handling With Fetch00:22
- Arrow Functions12:09
- Custom HTTP Library (Fetch With Promises) - Part 312:55
- Async & Await08:05
- Custom HTTP Library (Fetch With Async Await) - Part 405:33
- Github Finder [Part 1] - Intro & UI13:44
- Github Finder [Part 2] - Fetching Profile Data12:04
- Github Finder [Part 3] - Display The Profile10:43
- Github Finder [Part 4] - Show Alert Message10:06
- Github Finder [Part 5] - Fetch & Display Repos09:43
- WeatherJS [Part 1] - Intro & UI13:13
- WeatherJS [Part 2] - Fetch Weather From API08:02
- WeatherJS [Part 3] - Display The Weather08:47
- WeatherJS [Part 4] - Save Location To Local Storage11:12
- Error Handling with Try...Catch09:50
- Regular Expressions [Part 1] - Evaluation Functions13:33
- Regular Expressions [Part 2] - Metacharacter Symbols12:35
- Regular Expressions [Part 3] - Character Sets & Quantifiers12:41
- Regular Expressions [Part 4] - Shorthand Character Classes09:43
- Regular Expressions - Form Validation Project21:56
- Iterators & Generators09:46
- Profile Scroller - Iterator Mini Project15:06
- Symbols08:52
- Destructuring09:28
- ES6 Maps08:59
- ES6 Sets09:02
- What Are Patterns?02:29
- Module & Revealing Module Pattern12:53
- Singleton Pattern04:57
- Factory Pattern08:18
- Observer Pattern16:01
- Mediator Pattern10:14
- State Pattern - Small Project10:53
- Project Introduction03:14
- Creating The UI With Materialize CSS16:38
- Controllers & Data Structure11:02
- Get & Populate Items10:04
- Add Item To Data Structure16:34
- Add Item To The UI12:38
- Add Total Calories07:42
- Working With The Edit State20:26
- Updating Items & Total Calories18:57
- Delete & Clear Items15:46
- Add & Get From Local Storage12:34
- Delete & Clear From Local Storage10:10
- Babel & Webpack Environment Setup08:55
- Intro To ES2015 Modules09:21
- Create The UI07:07
- Create a fake REST API Using JSON Server07:14
- Get & Display Posts13:02
- Add Posts & Show Alert11:59
- Removing Posts06:09
- Post Edit State & Update [1]08:29
- Post Edit State & Update [2]18:06
- Wrap Up & Where To Go From Here02:11
Online Courses
Learning JavaScript doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of JavaScript related books and take your
YouTube videos
The number of high-quality and free JavaScript video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more