Learn Advanced C++ Programming
Discover intermediate to advanced C++, including C++ 11's fantastic additions to the C++ standard.
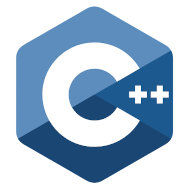
What you will learn?
- Develop complex C++ applications
- Understand C++ 11
- Be in a position to apply for jobs requiring good C++ knowledge
Your trainer

John Purcell
After working as a software developer and contractor for over 14 years for a whole bunch of companies including CSC, Proquest, SPSS and AT&T in the UK and Netherlands, I decided to work full-time as a private software trainer. After spending four years in the beautiful city of Budapest, Hungary and a year in Berlin, I now live in my home town of Derby, UK.
105 lessons
Easy to follow lectures and videos covering subject details.
15 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction03:15
- Source Code01:06
- Exceptions Basics13:56
- Standard Exceptions07:06
- Custom Exceptions07:41
- Exception Catching Order07:23
- Writing Text Files06:35
- Reading Text Files07:08
- Parsing Text Files12:12
- Structs and Padding07:57
- Reading and Writing Binary Files13:07
- Vectors10:57
- Vectors and Memory13:36
- Two-Dimensional Vectors07:26
- Lists12:53
- Maps13:48
- Custom Objects as Map Values08:44
- Custom Objects as Map Keys14:02
- Multimaps10:53
- Sets15:20
- Stacks and Queues16:48
- Sorting Vectors, Deque and Friend10:23
- STL Complex Data Types06:32
- Overloading the Assignment Operator16:04
- Printing - Overloading Left Bit Shift11:21
- A Complex Number Class17:44
- Overloading Plus11:52
- Overloading Equality Test06:48
- Overloading the Dereference Operator06:04
- Templates - An Important Note04:01
- Template Classes08:27
- Template Functions04:21
- Template Functions and Type Inference06:37
- Function Pointers09:55
- Using Function Pointers08:11
- Object Slicing and Polymorphism11:56
- Abstract Classes and Pure Virtual Functions12:17
- Functors14:19
- Decltype, Typeid and Name Mangling10:40
- The Auto Keyword08:30
- Range-Based Loops06:00
- Nested Template Classes15:10
- A Ring Buffer Class09:55
- Making Classes Iterable13:38
- Initialization in C++ 9806:38
- Initialization in C++ 1111:09
- Initializer Lists06:25
- Object Initialization, Default and Delete10:11
- Introducing Lambda Expressions08:28
- Lambda Parameters and Return Types09:10
- Lambda Capture Expressions08:23
- Capturing this With Lambdas04:48
- The Standard Function Type12:25
- Mutable Lambdas01:31
- Delegating Constructors06:11
- Elision and Optimization.avi10:06
- Constructors and Memory08:07
- Rvalues and LValues07:22
- LValue References05:31
- Rvalue References06:24
- Move Constructors05:07
- Move Assignment Operators05:53
- Static Casts10:32
- Dynamic Cast04:50
- Reinterpret Cast03:18
- Perfect Forwarding08:13
- Bind16:16
- Unique Pointers12:10
- Shared Pointers07:41
- GUI Programming Overview11:16
- Source Code00:08
- Fractal Creator - Hello World04:41
- Bitmap File Headers10:51
- Bitmap Info Headers05:23
- A Bitmap Class08:47
- Bitmap Memory04:26
- Bitmap Header Values06:58
- Writing a Bitmap11:08
- Setting Bitmap Pixels11:09
- Scaling09:48
- A Mandelbrot Class05:35
- The Fractal Algorithm16:45
- Improving the Image06:11
- The Colour Histogram07:04
- Validating the Histogram06:23
- Eliminating Max Iterations03:18
- Storing the Iterations02:52
- Using the Histogram09:17
- Colour Transforms04:04
- A Zoom Class05:30
- The Zoom List Class07:45
- Centering and Scaling11:01
- Applying the Zoom05:40
- Zooming In04:27
- Reorganising Main06:12
- Implementing FractalCreator14:13
- An RGB Class10:13
- Using the RGB Class06:25
- Specifying Color Ranges08:01
- Calculating Range Totals11:34
- Checking the Range Totals02:46
- Getting the Pixel Range11:23
- Range-Based Coloring07:54
- More Exercises06:14
- Multiple Inheritance06:13
Online Courses
Learning C++ doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C++ related books and take your
YouTube videos
The number of high-quality and free C++ video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more