Complete Modern C++ (C++11/14/17)
Learn about move semantics, lambda expressions, smart pointers, concurrency, template, STL & more
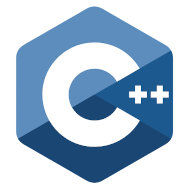
What you will learn?
- Use C++ as an object-oriented language
- Demystify function & class templates
- Use STL components in your applications
- Write real-world applications in C++
- Apply Modern C++ (C++11/14/17) in programs
Your trainer

Umar Lone
Civil engineer who found his calling in software development. Never worked as a Civil engineer, jumped at the first chance and started teaching C++ & Visual C++ 15 years ago. Currently, trains software professionals in various software companies in different technologies, such as Modern C++, Advanced C++, STL, Design Patterns, Android, Unity, Linux, etc. Very passionate about teaching and has trained more than 20,000 software professionals in a teaching career spanning more than 17 years. An avid gamer, currently trying his hand with game development in Unity & Unreal. Has a few Android applications to his credit, including one on Design Patterns.
233 lessons
Easy to follow lectures and videos covering subject details.
20 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction03:01
- Source Code00:02
- Required software00:31
- Visual Studio 2015 Installation (DEPRECATED)04:32
- Visual Studio 2017 Installation (DEPRECATED)02:44
- Visual Studio 2019 Installation05:24
- Code::Blocks Installation05:06
- Cevelop Installation05:31
- XCode Installation on MacOS03:32
- Course Slides00:11
- What is C++?03:31
- First C++ Program09:12
- The C++ Compilation Process03:09
- Primitive Types & Variables07:57
- Basic Input/Output05:56
- Functions Basics - Part I09:13
- Functions Basics - Part II09:05
- Overview of Debugging in Visual Studio05:40
- Uniform Initialization (C++11)09:21
- Pointers08:25
- Assignment00:17
- Reference05:32
- Assignment00:12
- Reference Vs Pointer06:58
- The const Qualifier04:19
- const Qualifer & Compound Types07:30
- Assignment00:23
- Automatic Type Inference (C++11)07:00
- Basics Quiz - I5 questions
- Range-Based For Loop - I (C++11)04:32
- Range-Based For Loop - II (C++11)07:02
- Function Overloading13:27
- Default Function Arguments03:43
- Inline Functions07:36
- Function Pointers04:53
- Namespace09:24
- Basics Quiz - II7 questions
- Dynamic Memory Allocation - Part I (malloc function)09:36
- Dynamic Memory Allocation - Part II (new operator)06:19
- Dynamic Memory Allocation - Part III (new[] operator)06:40
- Dynamic Memory Allocation - Part IV (2D arrays)05:07
- Dynamic Memory Allocation - Source Code Only00:02
- Object Oriented Programming Basics05:50
- Class05:31
- Constructor & Destructor04:28
- Structures02:38
- Non-static Data Member Initializers (C++11)04:39
- this Pointer04:46
- Static Class Members05:12
- Constant Member Functions03:14
- Copy Constructor - Part I04:52
- Copy Constructor - Part II04:40
- Delegating Constructors (C++11)04:01
- Default & Deleted Functions (C++11)05:18
- Quiz I8 questions
- L-values, R-values & R-value References (C++11)07:10
- Move Semantics - Basics (C++11)03:23
- Move Semantics - Implementation (C++11)04:12
- Rule of 5 & 014:30
- Copy Elision09:01
- std::move Function (C++11)07:52
- Quiz7 questions
- Operator Overloading Demo Code00:05
- Operator Overloading - Part I (Basics)08:28
- Operator Overloading - Part II (Assignment Operator)04:18
- Operator Overloading - Part III (Global Overloads)05:48
- Operator Overloading - Part IV (Friend Keyword)02:29
- Operator Overloading - Part V (Smart Pointer Basics)05:04
- Operator Overloading - Part VI (Smart Pointers in C++11)04:34
- Operator Overloading - Part VII (Rules)01:55
- Type Conversions - Part I (Basics)05:56
- Type Conversions - Part II (Primitive to User Type)05:39
- Type Conversions - Part III (User to Primitive Type)02:50
- Type Conversions - Part IV (User Defined to User Defined)04:38
- Initialization Vs. Assignment & Member Initialization List05:18
- Quiz8 questions
- Raw Pointers05:11
- std::unique_ptr10:11
- Sharing Pointers06:09
- Sharing std::unique_ptr04:34
- std::shared_ptr05:18
- Weak Ownership08:49
- std::weak_ptr Internals06:26
- Circular References10:27
- Deleter07:05
- Dynamic Arrays03:26
- Make Functions05:41
- Enums - Part I (Basics)03:42
- Enums - Part II (Scoped Enums C++11)05:32
- Strings - Part I (Raw Strings)06:49
- Strings - Part II (std::string)11:41
- Assignment I00:14
- Strings - Part III (String Streams)09:45
- Assignment II00:24
- User-Defined Literals05:59
- Constant Expressions - constexpr (C++11)09:24
- std::initializer_list (C++11)08:27
- Dynamic Array (std::vector)08:43
- Assignment III00:19
- Union - I04:51
- Union - II04:56
- Quiz5 questions
- Account Hierarchy Source Code00:06
- Inheritance & Composition06:01
- Inheritance & Access Modifiers03:55
- Project - I (Introduction)02:06
- Project - II (Account & Savings Class)06:59
- Assignment00:14
- Project - III (C++11 Inheriting Constructors)06:31
- Project - IV (Virtual Keyword)08:53
- Quiz I6 questions
- Project -V (Virtual Mechanism Internals - I)07:11
- Project -VI (Virtual Mechanism Internals - II)06:42
- Project - Part VIII (override & final specifier in C++11)06:13
- Project - VIII (Object Slicing)05:45
- Project - IX (typeid Operator)07:11
- Project - Part X (dynamic_cast Operator)06:08
- Abstract Class06:03
- Multiple (Diamond) Inheritance09:22
- Quiz II5 questions
- Exception Handling - Part I (Basics)07:59
- Exception Handling - Part II (Multiple Catch Blocks)03:58
- Exception Handling - Part III (Stack Unwinding)06:31
- Exception Handling - Part IV (Nested Exceptions)05:11
- Exception Handling - Part V (Constructor & Destructor)07:06
- Exception Handling - Part VI - (noexcept keyword in C++11)08:20
- Quiz5 questions
- Raw String Literals (C++11)04:03
- Introduction to Filesystem Library (C++17) (DEPRECATED)03:49
- File IO - Part I (Basics)06:44
- File IO - Part II (Error Handling)05:43
- File IO - Part III (Copy Utility)03:29
- File IO - Part IV (Character IO & Seeking)07:22
- File IO - Part V (Binary IO)08:30
- Assignment I00:22
- Assignment II00:15
- File Copy Utility Source00:05
- File IO4 questions
- Source Code00:03
- Introduction to Templates07:48
- Assignment I00:15
- Template Argument Deduction & Instantiation06:28
- Assignment II00:05
- Explicit Specialization05:54
- Non-type Template Arguments07:14
- Assignment III00:13
- Perfect Forwarding - Part I (C++11)07:23
- Perfect Forwarding - Part II (C++11)05:32
- Variadic Templates - Part I (C++11)09:47
- Variadic Templates - Part II (C++11)04:50
- Assignment IV00:14
- Class Templates08:39
- Class Template Explicit Specialization - Part I05:41
- Class Template Explicit Specialization - Part II04:02
- Assignment V00:09
- Class Template Partial Specialization06:52
- Typedef, Type Alias & Alias Templates (C++11)08:07
- Type Traits (C++11)07:21
- static_assert (C++11)04:19
- Templates8 questions
- Lambda Expressions Source Code00:03
- Callbacks Revisited - Function Pointers06:39
- Callbacks - Function Objects06:57
- Lambda Expressions04:36
- Lambda Expressions - Internals05:01
- Lambda Expressions Capture List - Part I08:25
- Lambda Expressions Capture List - Part II04:08
- Lambda Expressions Capture List - Part III05:57
- Generalized Lambda Capture04:33
- Assignment00:14
- Quiz5 questions
- Introduction02:50
- std::array (C++11)04:02
- std::vector04:38
- std::deque02:21
- std::list & std::forward_list (C++11)04:59
- Sequence Containers Demo Code00:02
- std::set & std::multiset05:42
- std::map & std::multimap07:35
- Associative Containers Demo Code00:03
- Unordered Containers (C++11) - I04:38
- Unordered Containers (C++11) - II05:48
- std::hash (C++11)03:48
- Unordered Containers Demo Code00:02
- Big O Notation & Performance of Containers05:04
- Algorithms - Part I08:15
- Algorithms - Part II07:04
- Container Changes in C++11 - I06:33
- Container Changes in C++11 - II04:06
- Container Changes in C++11 - III06:20
- Container Changes in C++11 - IV02:28
- Container Changes in C++11 - V07:00
- STL Project00:20
- Quiz8 questions
- Concurrency Source Code00:03
- Concurrency Basics05:43
- Thread Creation (std::thread)05:47
- Passing Arguments To Threads04:18
- Thread Synchronization (std::mutex)06:17
- std::lock_guard02:45
- std::thread Functions & std::this_thread Namespace07:48
- Task Based Concurrency - Part I05:07
- Task Based Concurrency - Part II03:44
- Launch Policies04:38
- std::future Wait Functions05:07
- Using std::promise05:36
- Propagating Exceptions Across Threads03:15
- Source Code Information00:14
- Deprecated & Removed Features03:53
- Changes03:12
- Attributes05:58
- Feature Test Macros05:51
- If & switch With Initialization07:56
- inline Variables05:45
- Nested Namespaces01:51
- noexcept03:35
- constexpr Lambda07:47
- Structured Bindings08:07
- Expression Evaluation Order06:36
- Mandatory Copy Elision - I04:28
- Mandatory Copy Elision - II04:33
- Class Template Argument Deduction (CTAD)05:41
- Folding Basics04:05
- Fold Expressions - Unary Folds04:27
- Fold Expressions - Binary Folds05:51
- Fold Expressions - Recap02:34
- Type Traits Suffixes03:22
- if constexpr - I07:42
- if constexpr - II04:42
- std::optional - I05:18
- std::optional - II03:50
- std::optional - III03:38
- std::variant - I05:57
- std::variant - II04:34
- std::variant - III05:58
- std::any06:33
- std::string_view - I08:39
- std::string_view - II08:13
- Filesystem - path05:49
- Filesystem - directory_entry07:00
- Filesystem - Directory Functions05:09
- Filesystem - Permissions05:44
- Parallel Algorithms - I03:49
- Parallel Algorithms - II07:32
- Parallel Algorithms - III04:24
- BONUS LECTURE00:01
Online Courses
Learning C++ doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C++ related books and take your
YouTube videos
The number of high-quality and free C++ video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more