C++: From Beginner to Expert
Designed for people who don't have any knowledge about the programming and want to program in C++
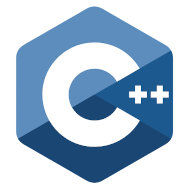
What you will learn?
- How to use C++ language in action
- What is compiler / IDE / Variables / types of variables etc.
- How to work with files - fstream library (i/o operation on files)
- operators - arithmetic, assigment, logical, bitwise
- conditions like if / else / switch
- arrays / multi-dimensional arrays
- loops - for / while / do-while
- functions, overloading functions, passing variables to functions etc.
- structures
- referencers
- pointers
- dynamic allocation of memory
- creating project in IDE
- classes
- object oriented programming
- class and function templates
- namespaces
- exceptions
- input / output streams and validation of data
- STL: vector
Your trainer

Arkadiusz Włodarczyk
I am the author of 27 very popular video courses about programming, web-development and math in Poland. I've also created 7 video courses in English. Over 245,000 people enrolled in my Udemy courses. I put all my heart into creation of courses. I always try to explain everything the easiest possible way. I'm sure that my courses will help you in the programming and web development adventure :)
I believe that everyone has the ability to develop software if they are taught properly. Including you. I'm going to give you the context of each new concept I teach you. After my course you will finally understand everything that you code.
I've been developing websites for over 15 years and I've been programming for over 10 years. I have enormous experience in that field and today I want to share with you my knowledge.
95 lessons
Easy to follow lectures and videos covering subject details.
17 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Intro & info about 100+ exercises00:33
- What is programming, compiler. Installing IDE15:40
- Problems when compiling C++ project? Solution INSIDE for mac users too01:56
- Basics of programming16:35
- Variables17:00
- Types of Variables18:54
- Downloading data from the input04:11
- Basics Quiz (Updated May 22')6 questions
- Arithmetic and Assigment Operators14:16
- Relational Operators06:21
- Logical Operators09:33
- Bitwise Operators (Advanced - Optional at start)20:32
- Operators Quiz (Updated Jan 22')9 questions
- Conditional Statements14:26
- Switch09:15
- Conditional Operator05:02
- Calculator - exercise15:11
- Conditional Statements Quiz (Updated Feb 22')5 questions
- Arrays16:35
- Multidimensional Arrays07:44
- Array Quiz (Updated March 22')4 questions
- for12:02
- while and do while10:15
- exercises, nested loops14:19
- break and continue13:11
- Loops Quiz (Updated Mar 22')6 questions
- pre-function Scope of Variables10:38
- Functions29:13
- Functions - return, and invoke explained in text01:00
- Overloading functions12:46
- Loops and functions - exercises20:13
- Data Validation - exercise21:25
- Why do we use cin.ignore and cin.clear()?07:19
- Functions Quiz (Updated June 22')5 questions
- Enum Type11:18
- Reference Variables09:49
- Reference Variables in functions08:33
- References and Enums Quiz (Updated Aug 22')4 questions
- What are pointers?19:38
- Name of array - Pointer13:22
- Dynamic allocation of memory22:15
- Strings and a pointer on a char type20:43
- How to make sure to correctly free memory of dynamically allocated array?01:50
- Functions and pointers17:26
- Lottery - pseudo-random numbers generator17:50
- Type Casting06:30
- Why do we need pointers?00:18
- Pointers FAQ - most confusing parts explained!03:28
- Pointers Quiz (Updated Jan 21')7 questions
- Creating project, external execution of program17:45
- Preprocessor directives, Multi-file project15:08
- Data Structures and Pointers11:23
- Classes31:12
- Static variables and functions10:40
- Constant classes and methods08:02
- Friend functions04:17
- Friend classes06:37
- Copy constructor11:51
- Convert constructor and overloading operators14:43
- Inheritance between classes28:02
- Polymorphism, virtual functions, abstract classes19:47
- Why do we need polymorphism?00:33
- Virtual destructor03:40
- Structures and Classes Quiz (Updated Nov 21')8 questions
- Function templates15:25
- Class templates20:37
- Templates Quiz (Updated Apr 21')3 questions
- Exceptions14:54
- Namespaces14:13
- Streams - what is input and output06:27
- How to open and close files?06:27
- File opening modes13:24
- Stream's error flags17:41
- Reading position pointer - seekg and tellg08:53
- Writing position pointer - seekp and tellp03:55
- Extracting characters from files15:26
- Comparing content of two files25:11
- Put - loading characters from cin stream directly to file04:17
- Peek - peeking characters without extracting03:42
- Putback - returning extracted character on stream02:38
- Write - writing bytes to file05:42
- Gcount - counting characters from last operation03:35
- Mini database42:03
- What is STL?02:30
- VECTOR - how to use it? Why is it useful?14:02
- C++11 - how to enable the flag? How to set starting values of vector?03:00
- What are iterators? How to use them?10:56
- What are iterators - NOTES01:32
- How to iterate vector? How to use loops to process elements in a container?04:16
- auto keyword - C++1104:07
- ranged based loop for - C++1103:41
- ranged-based loop for - & - how to prevent making a copy07:16
- Finding specific amount of odd numbers08:51
- The absolute value03:32
- Spelling the word backwards04:43
- How many digits are inside a number?07:31
- Multiples of number up to...04:25
- Finding largest number (max value)12:20
- Can 3 sides create a triangle?02:18
- Is it a rectangular triangle?03:30
- Factorial iteratively04:40
- Factorial recursively11:31
- Fibonacci iteratively09:12
- Fibonacci recursively07:56
- What's next?03:54
Online Courses
Learning C++ doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C++ related books and take your
YouTube videos
The number of high-quality and free C++ video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more