The Complete JavaScript Course 2023: From Zero to Expert!
The modern JavaScript course for everyone! Master JavaScript with projects, challenges and theory. Many courses in one!
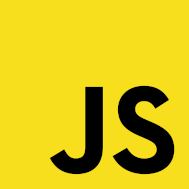
What you will learn?
- Become an advanced, confident, and modern JavaScript developer from scratch
- Build 6 beautiful real-world projects for your portfolio (not boring toy apps)
- Become job-ready by understanding how JavaScript really works behind the scenes
- How to think and work like a developer: problem-solving, researching, workflows
- JavaScript fundamentals: variables, if/else, operators, boolean logic, functions, arrays, objects, loops, strings, etc.
- Modern ES6+ from the beginning: arrow functions, destructuring, spread operator, optional chaining (ES2020), etc.
- Modern OOP: Classes, constructors, prototypal inheritance, encapsulation, etc.
- Complex concepts like the 'this' keyword, higher-order functions, closures, etc.
- Asynchronous JavaScript: Event loop, promises, async/await, AJAX calls and APIs
- How to architect your code using flowcharts and common patterns
- Modern tools for 2022 and beyond: NPM, Parcel, Babel and ES6 modules
- Practice your skills with 50+ challenges and assignments (solutions included)
- Get fast and friendly support in the Q&A area
- Course pathways: design your unique learning path according to your goals!
Your trainer

Jonas Schmedtmann
Hi, I'm Jonas! I have been identified as one of Udemy's Top Instructors and all my premium courses have recently earned the best-selling status for outstanding performance and student satisfaction.
I'm a full-stack web developer and designer with a passion for building beautiful things from scratch. I've been building websites and apps since 2007 and also have a Master's degree in Engineering.
It was in college where I first discovered my passion for teaching and helping others by sharing all I knew. And that passion brought me to Udemy in 2015, where my students love the fact that I take the time to explain important concepts in a way that everyone can easily understand.
320 lessons
Easy to follow lectures and videos covering subject details.
69 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Course Structure and Projects05:25
- Read Before You Start!00:34
- Watch Before You Start!06:09
- Migration Guide to v2 + Old Course01:51
- Setting Up Our Code Editor08:23
- Section Intro00:53
- Hello World!05:57
- A Brief Introduction to JavaScript11:18
- Linking a JavaScript File15:55
- Values and Variables16:05
- Practice Assignments00:23
- Data Types19:19
- let, const and var09:58
- Basic Operators19:31
- Operator Precedence11:19
- Coding Challenge #110:28
- Strings and Template Literals10:57
- Taking Decisions: if / else Statements12:50
- Coding Challenge #206:21
- Type Conversion and Coercion16:40
- Truthy and Falsy Values10:03
- Equality Operators: == vs. ===15:41
- Boolean Logic08:30
- Logical Operators10:37
- Coding Challenge #315:07
- The switch Statement13:10
- Statements and Expressions06:08
- The Conditional (Ternary) Operator10:02
- Coding Challenge #409:11
- JavaScript Releases: ES5, ES6+ and ESNext14:19
- Section Intro00:36
- Activating Strict Mode10:27
- Functions19:08
- Function Declarations vs. Expressions10:39
- Arrow Functions09:52
- Functions Calling Other Functions10:07
- Reviewing Functions15:37
- Coding Challenge #118:12
- Introduction to Arrays21:34
- Basic Array Operations (Methods)12:52
- Coding Challenge #209:36
- Introduction to Objects06:08
- Dot vs. Bracket Notation19:21
- Object Methods23:04
- Coding Challenge #312:58
- Iteration: The for Loop11:11
- Looping Arrays, Breaking and Continuing22:02
- Looping Backwards and Loops in Loops11:53
- The while Loop11:52
- Coding Challenge #415:35
- Pathways and Section Roadmaps04:08
- Course Pathways00:07
- Section Intro00:39
- Section Roadmap00:22
- Setting up Prettier and VS Code16:22
- Installing Node.js and Setting Up a Dev Environment11:32
- Learning How to Code17:42
- How to Think Like a Developer: Become a Problem Solver!10:53
- Using Google, StackOverflow and MDN26:36
- Debugging (Fixing Errors)05:09
- Debugging with the Console and Breakpoints19:25
- Coding Challenge #115:24
- Section Intro00:40
- Basic HTML Structure and Elements09:32
- Attributes, Classes and IDs13:09
- Basic Styling with CSS12:00
- Introduction to the CSS Box Model20:57
- Section Intro00:49
- Section Roadmap00:29
- PROJECT #1: Guess My Number!08:47
- What's the DOM and DOM Manipulation06:48
- Selecting and Manipulating Elements08:22
- Handling Click Events12:50
- Implementing the Game Logic19:33
- Manipulating CSS Styles08:17
- Coding Challenge #111:40
- Implementing Highscores07:47
- Refactoring Our Code: The DRY Principle15:05
- PROJECT #2: Modal Window13:21
- Working With Classes16:55
- Handling an "Esc" Keypress Event14:11
- PROJECT #3: Pig Game17:08
- Rolling the Dice15:58
- Switching the Active Player15:16
- Holding Current Score24:55
- Resetting the Game15:38
- Section Intro01:31
- Section Roadmap00:30
- An High-Level Overview of JavaScript12:11
- The JavaScript Engine and Runtime13:47
- Execution Contexts and The Call Stack17:45
- Scope and The Scope Chain25:37
- Scoping in Practice21:13
- Variable Environment: Hoisting and The TDZ11:00
- Hoisting and TDZ in Practice14:42
- The this Keyword06:30
- The this Keyword in Practice13:11
- Regular Functions vs. Arrow Functions18:04
- Primitives vs. Objects (Primitive vs. Reference Types)16:06
- Primitives vs. Objects in Practice14:57
- Section Intro00:53
- Section Roadmap00:22
- Destructuring Arrays19:33
- Destructuring Objects19:46
- The Spread Operator (...)21:26
- Rest Pattern and Parameters19:01
- Short Circuiting (&& and ||)15:55
- The Nullish Coalescing Operator (??)03:32
- Logical Assignment Operators11:39
- Coding Challenge #114:57
- Looping Arrays: The for-of Loop07:20
- Enhanced Object Literals07:02
- Optional Chaining (?.)16:10
- Looping Objects: Object Keys, Values, and Entries10:09
- Coding Challenge #214:30
- Sets13:18
- Maps: Fundamentals14:03
- Maps: Iteration12:41
- Summary: Which Data Structure to Use?09:40
- Coding Challenge #309:37
- Working With Strings - Part 116:53
- Working With Strings - Part 221:45
- Working With Strings - Part 321:41
- Coding Challenge #415:15
- String Methods Practice16:36
- Section Intro00:48
- Section Roadmap00:22
- Default Parameters09:17
- How Passing Arguments Works: Value vs. Reference13:36
- First-Class and Higher-Order Functions05:24
- Functions Accepting Callback Functions15:20
- Functions Returning Functions06:36
- The call and apply Methods16:51
- The bind Method21:33
- Coding Challenge #118:47
- Immediately Invoked Function Expressions (IIFE)07:52
- Closures19:48
- More Closure Examples15:30
- Coding Challenge #205:25
- Section Intro00:53
- Section Roadmap00:29
- Simple Array Methods16:37
- The new at Method06:04
- Looping Arrays: forEach13:46
- forEach With Maps and Sets05:32
- PROJECT: "Bankist" App09:48
- Creating DOM Elements18:44
- Coding Challenge #108:31
- Data Transformations: map, filter, reduce04:40
- The map Method15:40
- Computing Usernames12:14
- The filter Method06:19
- The reduce Method20:57
- Coding Challenge #209:56
- The Magic of Chaining Methods19:39
- Coding Challenge #303:57
- The find Method06:47
- Implementing Login24:18
- Implementing Transfers20:54
- The findIndex Method12:34
- some and every15:11
- flat and flatMap09:32
- Sorting Arrays21:55
- More Ways of Creating and Filling Arrays20:33
- Summary: Which Array Method to Use?06:24
- Array Methods Practice32:21
- Coding Challenge #423:47
- Section Intro00:50
- Section Roadmap00:22
- Converting and Checking Numbers16:46
- Math and Rounding18:14
- The Remainder Operator10:56
- Numeric Separators06:57
- Working with BigInt11:18
- Creating Dates12:55
- Adding Dates to "Bankist" App22:21
- Operations With Dates15:27
- Internationalizing Dates (Intl)17:18
- Internationalizing Numbers (Intl)19:06
- Timers: setTimeout and setInterval13:53
- Implementing a Countdown Timer28:31
- Section Intro01:04
- Section Roadmap00:22
- PROJECT: "Bankist" Website08:49
- How the DOM Really Works10:36
- Selecting, Creating, and Deleting Elements20:28
- Styles, Attributes and Classes21:54
- Implementing Smooth Scrolling15:56
- Types of Events and Event Handlers10:33
- Event Propagation: Bubbling and Capturing05:03
- Event Propagation in Practice17:46
- Event Delegation: Implementing Page Navigation18:48
- DOM Traversing14:35
- Building a Tabbed Component24:08
- Passing Arguments to Event Handlers18:43
- Implementing a Sticky Navigation: The Scroll Event07:55
- A Better Way: The Intersection Observer API24:00
- Revealing Elements on Scroll12:39
- Lazy Loading Images18:30
- Building a Slider Component: Part 123:16
- Building a Slider Component: Part 219:35
- Lifecycle DOM Events09:26
- Efficient Script Loading: defer and async13:30
- Section Intro01:07
- Section Roadmap00:22
- What is Object-Oriented Programming?20:55
- OOP in JavaScript10:08
- Constructor Functions and the new Operator14:19
- Prototypes14:36
- Prototypal Inheritance and The Prototype Chain10:58
- Prototypal Inheritance on Built-In Objects14:46
- Coding Challenge #107:27
- ES6 Classes12:57
- Setters and Getters13:15
- Static Methods06:24
- Object.create10:56
- Coding Challenge #206:00
- Inheritance Between "Classes": Constructor Functions21:03
- Coding Challenge #310:37
- Inheritance Between "Classes": ES6 Classes10:46
- Inheritance Between "Classes": Object.create08:53
- Another Class Example10:44
- Encapsulation: Protected Properties and Methods06:47
- Encapsulation: Private Class Fields and Methods16:11
- Chaining Methods04:43
- ES6 Classes Summary07:11
- Coding Challenge #408:54
- Section Intro00:58
- Section Roadmap00:27
- Project Overview05:02
- How to Plan a Web Project17:41
- Using the Geolocation API08:16
- Displaying a Map Using Leaflet Library13:48
- Displaying a Map Marker19:54
- Rendering Workout Input Form16:42
- Project Architecture09:28
- Refactoring for Project Architecture24:05
- Managing Workout Data: Creating Classes16:18
- Creating a New Workout34:06
- Rendering Workouts24:19
- Move to Marker On Click16:32
- Working with localStorage25:31
- Final Considerations05:41
- Section Intro00:55
- Section Roadmap00:22
- Asynchronous JavaScript, AJAX and APIs17:57
- IMPORTANT: API URL Change00:13
- Our First AJAX Call: XMLHttpRequest19:10
- [OPTIONAL] How the Web Works: Requests and Responses13:38
- Welcome to Callback Hell13:52
- Promises and the Fetch API09:25
- Consuming Promises09:24
- Chaining Promises09:13
- Handling Rejected Promises16:13
- Throwing Errors Manually15:24
- Coding Challenge #116:31
- Asynchronous Behind the Scenes: The Event Loop17:53
- The Event Loop in Practice09:15
- Building a Simple Promise20:17
- Promisifying the Geolocation API13:26
- Coding Challenge #215:53
- Consuming Promises with Async/Await15:06
- Error Handling With try...catch10:22
- Returning Values from Async Functions14:39
- Running Promises in Parallel10:56
- Other Promise Combinators: race, allSettled and any13:19
- Coding Challenge #317:30
- Section Intro00:47
- Section Roadmap00:22
- An Overview of Modern JavaScript Development06:38
- An Overview of Modules in JavaScript15:01
- Exporting and Importing in ES6 Modules22:38
- Top-Level await (ES2022)14:44
- The Module Pattern10:19
- CommonJS Modules04:24
- A Brief Introduction to the Command Line12:27
- Introduction to NPM18:40
- Bundling With Parcel and NPM Scripts21:39
- Configuring Babel and Polyfilling18:03
- Review: Writing Clean and Modern JavaScript10:26
- Let's Fix Some Bad Code: Part 123:20
- Declarative and Functional JavaScript Principles12:25
- Let's Fix Some Bad Code: Part 238:09
- Section Intro00:56
- Section Roadmap00:27
- Project Overview and Planning (I)14:21
- Latest Code Updates (Parcel v2 and more)00:47
- Loading a Recipe from API24:11
- Rendering the Recipe22:23
- Listening For load and hashchange Events11:02
- The MVC Architecture16:32
- Refactoring for MVC38:53
- Helpers and Configuration Files21:23
- Event Handlers in MVC: Publisher-Subscriber Pattern15:08
- Implementing Error and Success Messages11:34
- Implementing Search Results - Part 125:46
- Implementing Search Results - Part 228:15
- Implementing Pagination - Part 112:18
- Implementing Pagination - Part 237:05
- Project Planning II03:13
- Updating Recipe Servings26:40
- Developing a DOM Updating Algorithm34:22
- Implementing Bookmarks - Part 125:59
- Implementing Bookmarks - Part 218:31
- Storing Bookmarks With localStorage18:22
- Project Planning III02:08
- Uploading a New Recipe - Part 117:43
- Uploading a New Recipe - Part 240:37
- Uploading a New Recipe - Part 319:58
- Wrapping Up: Final Considerations14:45
- Section Intro00:44
- Section Roadmap00:22
- Simple Deployment With Netlify11:18
- Setting Up Git and GitHub07:39
- Git Fundamentals19:36
- Pushing to GitHub09:31
- Setting Up Continuous Integration With Netlify10:06
- Where to Go from Here03:00
- My Other Courses + Updates01:03
- Access the Old Course00:51
Online Courses
Learning JavaScript doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of JavaScript related books and take your
YouTube videos
The number of high-quality and free JavaScript video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more