Web Development w/ Google’s Go (golang) Programming Language
Learn Web Programming from a University Professor in Computer Science with over 20 years of teaching experience.
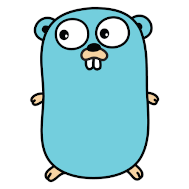
What you will learn?
- Construct server-side applications using today's best practices
- Acquire an outstanding foundation in the fundamentals of web programming
- Learn from a University Professor in Computer Science with over 20 years of experience teaching individuals of all ability levels
- Achieve mastery in the understanding and application of web development
- Understand servers, routing, restful applications, JSON, templates, and file servers
- Understand cookies, state, UUIDs, HTTP methods, HTTP response codes, and sessions
- Create web applications using only the Go programming language's standard library
- Create web applications without using third-party frameworks
- Build performant distributed applications that dynamically scale
- Apply cutting-edge web development practices
Your trainer

Todd McLeod
I am a tenured professor in California. I have taught at both the college and university level. I have also taught in multiple disciplines including business, information systems, computer science, and online education. In 1997, I was one of the first professors at the university to teach online. I did post-graduate work in online education at UC San Diego. I co-founded and taught in the “Online Teacher’s Training Program” which trained professors how to teach online. In 2008, I was selected as one of the best instructors in the entire California Community College system. Currently, when measured by the number of students served, I am the world’s leading trainer in Google’s new programming language which is one of the fastest growing, highest paying programming languages in America. In addition, I have started three businesses (two of them profitable, so far). My background in business, information systems, computer science, online education, and real-world entrepreneurship has prepared me to teach all of these courses. I look forward to helping you learn great skills to improve your life. Better skills, better life.
173 lessons
Easy to follow lectures and videos covering subject details.
19 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Why choose Go ( golang ) for web development?07:39
- Course outline01:22
- Language review17:28
- How to succeed08:12
- Miscellaneous resources00:04
- Understanding templates03:27
- Templating with concatenation06:25
- Understanding package text/template: parsing & executing templates16:52
- Passing data into templates03:57
- Variables in templates02:28
- Passing composite data structures into templates14:57
- Functions in templates15:11
- Pipelines in templates09:53
- Predefined global functions in templates06:10
- Nesting templates - modularizing your code07:51
- Passing data into templates & composition05:38
- Using methods in templates03:37
- Hands-on exercises01:58
- Using package html/template, character escaping, & cross-site scripting03:36
- Understanding servers10:08
- TCP server - write to connection06:55
- TCP server - read from connection using bufio.Scanner10:10
- TCP server - read from & write to connection04:25
- TCP server - code a client04:47
- TCP server - rot13 & in-memory database09:56
- TCP server - HTTP request / response foundation hands-on exercise06:56
- TCP server - HTTP method & URI retrieval hands-on exercise02:45
- TCP server - HTTP multiplexer05:03
- net/http package - an overview09:21
- Understanding & using ListenAndServe05:21
- Foundation of net/http: Handler, ListenAndServe, Request, ResponseWriter05:21
- Retrieving form values - exploring *http.Request15:07
- Retrieving other request values - exploring *http.Request08:10
- Exploring http.ResponseWriter - writing headers to the response07:35
- Review07:13
- Understanding ServeMux16:26
- Disambiguation: func(ResponseWriter, *Request) vs. HandlerFunc08:02
- Third-party servemux - julien schmidt’s router08:46
- Hands-on exercises01:14
- Hands-on exercises - solutions #104:20
- Hands-on exercises - solutions #219:56
- Serving a file with io.Copy05:02
- Serving a file with http.ServeContent & http.ServeFile03:53
- Serving a file with http.FileServer03:49
- Serving a file with http.FileServer & http.StripPrefix06:00
- Creating a static file server with http.FileServer03:10
- log.Fatal & http.Error04:00
- Hands-on exercises00:59
- Hands-on exercises - solutions05:21
- The http.NotFoundHandler05:47
- Buying a domain - google domains02:16
- Deploying to google cloud07:52
- State overview01:19
- Passing values through the URL06:22
- Passing values from forms05:29
- Uploading a file, reading the file, creating a file on the server10:40
- Enctype12:00
- Redirects - overview06:32
- Redirects - diagrams & documentation07:55
- Redirects - in practice07:43
- Cookies - overview03:31
- Cookies - writing and reading05:19
- Writing multiple cookies & hands-on exercise02:11
- Hands-on exercise solution: creating a counter with cookies03:19
- Deleting a cookie06:37
- Sessions07:26
- Universally unique identifier - UUID08:31
- Your first session11:59
- Sign-up06:00
- Encrypt password with bcrypt05:20
- Login07:07
- Logout04:04
- Permissions03:42
- Expire session08:25
- Overview07:20
- Creating a virtual server instance on AWS EC210:50
- Hello World on AWS12:01
- Persisting an application04:42
- Hands-on Exercise02:13
- Hands-on Solution15:59
- Terminating AWS services01:31
- Overview06:24
- Installing MySQL - Locally02:44
- Installing MySQL - AWS06:28
- Connect Workbench to MySQL on AWS04:39
- Go & SQL - Setup04:37
- Go & SQL - In Practice07:43
- Overview of Load Balancers03:20
- Create EC2 Security Groups06:31
- Create an ELB Load Balancer07:59
- Implementing The Load Balancer13:48
- Connecting To Your MySQL Server Using MySQL Workbench05:11
- Hands-on Exercise01:05
- Hands-on Solution18:51
- Autoscaling & CloudFront12:42
- Starting Files04:32
- User Data05:44
- Storing Multiple Values03:36
- Uploading Pictures08:51
- Displaying Pictures01:25
- Keyed-Hash Message Authentication Code (HMAC)04:28
- Base64 Encoding03:06
- Web Storage05:30
- Context13:18
- TLS & HTTPS09:45
- JSON - JavaScript Object Notation09:26
- Go & JSON - Marshal & Encode09:47
- Unmarshal JSON with Go10:27
- Unmarshal JSON with Go using Tags10:00
- Hands-On Exercise Solution02:08
- AJAX Introduction11:49
- AJAX Server Side12:12
- Organizing Code Into Packages10:12
- Create User & Delete User04:28
- MVC Design Pattern - Model View Controller06:36
- Install Mongodb04:57
- Connect to Mongodb05:28
- CRUD with Go & Mongodb08:51
- Hands on Exercise & Solution05:23
- Hands on Exercise & Solution06:19
- Hands on Exercise & Solution02:38
- Introduction to Docker02:39
- Virtual Machines & Containers10:03
- Installing Docker05:18
- Docker Whalesay Example08:04
- Using a Dockerfile to Build An Image12:47
- Launching a Container Running Curl04:49
- Running a Go Web App In a Docker Container05:22
- Pushing & Pulling To Docker Hub08:55
- Go, Docker & Amazon Web Services (AWS)07:34
- Installing Postgres07:32
- Create Database06:25
- Create Table05:16
- Insert Records05:34
- Auto Increment Primary Key03:22
- Hands-on Exercise00:48
- Hands-on Exercise - Solution02:31
- Relational Databases05:42
- Query - Cross Join07:06
- Query - Inner Join06:28
- Query - Three Table Inner Join05:37
- Query - Outer Joins09:56
- Clauses03:52
- Update a Record01:07
- Delete a Record00:42
- Users - Create, Grant, Alter, Remove04:45
- Go & Postgres07:37
- Select Query08:58
- Web App05:06
- Query Row04:55
- Insert Record07:28
- Update Record04:05
- Delete Record01:29
- Code Organization11:23
- NoSQL11:32
- MongoDB09:27
- Installing Mongo10:52
- Database06:57
- Collection03:52
- Document03:49
- Find (aka, query)06:24
- Update06:58
- Remove01:59
- Projection02:44
- Limit01:04
- Sort02:51
- Index01:23
- Aggregation04:32
- Users09:19
- JSON04:40
- Create Read Update Delete (CRUD)16:58
- Congratulations05:58
- Bonus lecture05:37
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more