Go: The Complete Developer's Guide (Golang)
Master the fundamentals and advanced features of the Go Programming Language (Golang)
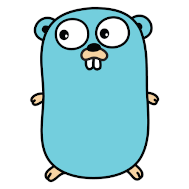
What you will learn?
- Build massively concurrent programs with Go Routines and Channels
- Learn the advanced features of Go
- Understand the differences between commonly used data structures
- Prove your knowledge with dozens of included quiz questions
- Apply Interfaces to dramatically simplify complex programs
- Use types to future-proof your code and reduce the difficulty of refactors
Your trainer

Stephen Grider
Stephen Grider has been building complex Javascript front ends for top corporations in the San Francisco Bay Area. With an innate ability to simplify complex topics, Stephen has been mentoring engineers beginning their careers in software development for years, and has now expanded that experience onto Udemy, authoring the highest rated React course. He teaches on Udemy to share the knowledge he has gained with other software engineers. Invest in yourself by learning from Stephen's published courses.
83 lessons
Easy to follow lectures and videos covering subject details.
9 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- How to Get Help00:19
- Course Resources00:38
- Join Our Community!00:07
- Environment Setup02:55
- VSCode Installation02:46
- Go Support in VSCode03:22
- Boring Ol' Hello World03:11
- Five Important Questions06:17
- Go Packages06:10
- Import Statements03:58
- File Organization03:34
- Test Your Knowledge: Packages4 questions
- How to Access Course Diagrams00:15
- Project Overview02:31
- New Project Folder01:55
- Variable Declarations10:40
- Test Your Knowledge: Variable Assignment9 questions
- Functions and Return Types07:42
- Test Your Knowledge: Functions5 questions
- Slices and For Loops10:49
- Test Your Knowledge: Slices and For Loops5 questions
- OO Approach vs Go Approach04:33
- Custom Type Declarations06:40
- Receiver Functions06:16
- Test Your Knowledge: Functions with Receivers4 questions
- Creating a New Deck10:57
- Slice Range Syntax05:56
- Multiple Return Values08:11
- Test Your Knowledge: Multiple Return Values5 questions
- Byte Slices06:59
- Deck to String07:26
- Joining a Slice of Strings06:36
- Saving Data to the Hard Drive07:02
- Reading From the Hard Drive11:18
- Error Handling07:52
- Shuffling a Deck09:42
- Random Number Generation10:30
- Testing With Go03:40
- Writing Useful Tests12:03
- Asserting Elements in a Slice04:23
- Testing File IO09:52
- Project Review06:17
- Even and Odd1 question
- Structs in Go04:43
- Defining Structs03:54
- Declaring Structs04:51
- Updating Struct Values05:36
- Embedding Structs06:30
- Structs with Receiver Functions07:26
- Pass By Value05:52
- Structs with Pointers03:12
- Pointer Operations10:13
- Pointer Shortcut06:26
- Gotchas With Pointers04:09
- Test Your Knowledge: Pointers9 questions
- Reference vs Value Types07:38
- Test Your Knowledge: Value vs Reference Types6 questions
- What's a Map?05:47
- Manipulating Maps04:55
- Iterating Over Maps05:16
- Differences Between Maps and Structs05:54
- Test Your Knowledge: Maps4 questions
- Purpose of Interfaces09:25
- Problems Without Interfaces10:14
- Interfaces in Practice09:16
- Rules of Interfaces07:48
- Extra Interface Notes06:58
- The HTTP Package07:59
- Reading the Docs05:35
- More Interface Syntax03:13
- Interface Review01:53
- The Reader Interface08:17
- More on the Reader Interface07:23
- Working with the Read Function05:38
- The Writer Interface04:15
- The io.Copy Function05:12
- The Implementation of io.Copy04:33
- A Custom Writer07:56
- Test Your Knowledge: Interfaces8 questions
- Interfaces1 question
- Hard Mode Interfaces1 question
- Website Status Checker05:28
- Printing Site Status04:32
- Serial Link Checking03:27
- Go Routines07:12
- Theory of Go Routines08:41
- Channels06:09
- Channel Implementation09:16
- Blocking Channels09:55
- Receiving Messages03:32
- Repeating Routines06:45
- Alternative Loop Syntax03:58
- Sleeping a Routine06:14
- Function Literals04:48
- Channels Gotcha!10:32
- Channels and Go Routines6 questions
- Bonus!00:29
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more