Learn How To Code: Google's Go (golang) Programming Language
The Ultimate Comprehensive Course - Perfect for Both Beginners and Experienced Developers
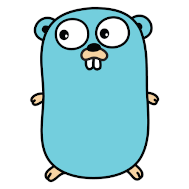
What you will learn?
- The ultimate comprehensive course
- For beginners and experienced devs
- Taught by a university professor
- From beginning to advanced concepts
- Concurrency, channels, benchmarking
- Testing, error handling, documentation
- Hands-on exercises with solutions
- Access to valuable code base
- This course is tried, tested, and proven
- Over 2.65 Million students taught
- Lifetime course access
- Learn at your own pace
- 100% satisfaction guaranteed
Your trainer

Todd McLeod
I am a tenured professor in California. I have taught at both the college and university level. I have also taught in multiple disciplines including business, information systems, computer science, and online education. In 1997, I was one of the first professors at the university to teach online. I did post-graduate work in online education at UC San Diego. I co-founded and taught in the “Online Teacher’s Training Program” which trained professors how to teach online. In 2008, I was selected as one of the best instructors in the entire California Community College system. Currently, when measured by the number of students served, I am the world’s leading trainer in Google’s new programming language which is one of the fastest growing, highest paying programming languages in America. In addition, I have started three businesses (two of them profitable, so far). My background in business, information systems, computer science, online education, and real-world entrepreneurship has prepared me to teach all of these courses. I look forward to helping you learn great skills to improve your life. Better skills, better life.
368 lessons
Easy to follow lectures and videos covering subject details.
45.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome!02:56
- Why go?11:14
- How to succeed11:17
- Course outline07:06
- Documentation06:49
- Miscellaneous resources00:20
- The terminal06:51
- Bash on windows08:17
- Shell / bash commands I11:13
- Shell / bash commands II07:35
- Installing Go10:19
- Go modules - introduction02:53
- Go workspace06:09
- Environment variables09:07
- Intro to IDE's01:47
- VS Code IDE03:13
- Using go get to get course code13:49
- IDE's11:21
- Go commands15:08
- Github repos11:01
- Github explored08:34
- Package management06:07
- Go modules overview06:15
- Creating a go module04:52
- Adding a dependency04:51
- Upgrading dependencies03:54
- Playground06:19
- Hello world10:31
- Introduction to packages16:05
- Short declaration operator12:53
- The var keyword11:40
- Exploring type13:01
- Zero value04:02
- The fmt package09:36
- Creating your own type05:47
- Conversion, not casting06:04
- Hands-on exercise #105:32
- Hands-on exercise #203:11
- Hands-on exercise #302:49
- Hands-on exercise #405:22
- Hands-on exercise #503:19
- Quiz for "Variables, Values & Type"40 questions
- Hands-on exercise #610:29
- Bool type08:07
- How computers work21:25
- Numeric types15:01
- String type15:30
- Numeral systems17:50
- Constants03:36
- Iota02:47
- Bit shifting11:12
- Hands-on exercise #101:54
- Hands-on exercise #203:08
- Hands-on exercise #301:13
- Hands-on exercise #402:35
- Hands-on exercise #500:52
- Hands-on exercise #601:12
- Quiz for Fundamentals of Programming26 questions
- Understanding control flow01:47
- Loop - init, condition, post04:14
- Loop - nesting loops03:54
- Loop - for statement10:22
- Loop - break & continue06:37
- Loop - printing ascii06:39
- Conditional - if statement07:19
- Conditional - if, else if, else03:10
- Loop, conditional, modulus03:24
- Conditional - switch statement08:35
- Conditional - switch statement documentation08:27
- Conditional logic operators03:59
- Hands-on exercise #102:18
- Hands-on exercise #202:35
- Hands-on exercise #301:55
- Hands-on exercise #401:11
- Hands-on exercise #502:34
- Hands-on exercise #601:04
- Hands-on exercise #701:28
- Hands-on exercise #801:25
- Hands-on exercise #902:06
- Hands-on exercise #1005:31
- Array04:47
- Slice - composite literal05:29
- Slice - for range03:23
- Slice - slicing a slice04:34
- Slice - append to a slice05:17
- Slice - deleting from a slice04:53
- Slice - make09:48
- Slice - multi-dimensional slice03:57
- Map - introduction11:49
- Map - add element & range04:16
- Map - delete03:15
- Hands-on exercise #102:38
- Hands-on exercise #201:15
- Hands-on exercise #303:59
- Hands-on exercise #403:13
- Hands-on exercise #502:24
- Hands-on exercise #605:42
- Hands-on exercise #709:13
- Hands-on exercise #804:01
- Hands-on exercise #901:25
- Hands-on exercise #1001:36
- Struct04:07
- Embedded structs05:36
- Reading documentation07:39
- Anonymous structs03:31
- Housekeeping18:47
- Hands-on exercise #104:11
- Hands-on exercise #204:08
- Hands-on exercise #303:54
- Hands-on exercise #403:55
- Syntax12:36
- Variadic parameter09:04
- Unfurling a slice13:24
- Defer06:13
- Methods07:16
- Interfaces & polymorphism20:14
- Anonymous func04:29
- func expression03:47
- Returning a func11:50
- Callback12:50
- Closure09:27
- Recursion09:34
- Hands-on exercise #110:09
- Hands-on exercise #204:05
- Hands-on exercise #200:15
- Hands-on exercise #306:06
- Hands-on exercise #405:19
- Hands-on exercise #510:03
- Hands-on exercise #602:06
- Hands-on exercise #704:14
- Hands-on exercise #802:24
- Hands-on exercise #908:24
- Hands-on exercise #1003:12
- What are pointers?11:54
- When to use pointers08:28
- Method sets06:31
- Hands-on exercise #101:02
- Hands-on exercise #205:36
- JSON documentation12:12
- JSON marshal06:28
- JSON unmarshal16:14
- Writer interface15:37
- Sort05:44
- Sort custom10:32
- bcrypt12:01
- Hands-on exercise #104:07
- Hands-on exercise #208:00
- Hands-on exercise #305:17
- Hands-on exercise #401:47
- Hands-on exercise #507:14
- Concurrency vs parallelism05:50
- WaitGroup12:28
- Method sets revisited12:01
- Documentation13:18
- Race condition12:29
- Mutex04:28
- Atomic05:44
- Hands-on exercise #116:14
- Hands-on exercise #211:56
- Hands-on exercise #311:05
- Hands-on exercise #404:42
- Hands-on exercise #504:48
- Hands-on exercise #605:39
- Understanding channels15:52
- Directional channels09:55
- Using channels06:35
- Range05:34
- Select10:28
- Comma ok idiom05:56
- Fan in07:57
- Fan out06:35
- Context14:39
- Hands-on exercise #103:19
- Hands-on exercise #202:36
- Hands-on exercise #303:26
- Hands-on exercise #406:16
- Hands-on exercise #502:44
- Hands-on exercise #603:07
- Hands-on exercise #704:54
- Understanding14:42
- Checking errors07:04
- Printing and logging09:11
- Recover10:07
- Errors with info18:26
- Hands-on exercise #103:45
- Hands-on exercise #211:53
- Hands-on exercise #310:37
- Hands-on exercise #406:00
- Hands-on exercise #501:39
- Introduction05:19
- go doc07:53
- godoc06:46
- godoc.org04:25
- Writing documentation12:04
- Hands-on exercise #104:39
- Introduction14:14
- Table tests07:44
- Example tests09:47
- Golint04:45
- Benchmark09:26
- Coverage08:36
- Benchmark examples12:13
- Review12:32
- Hands-on exercise #113:31
- Hands-on exercise #219:05
- Hands-on exercise #315:04
- Congratulations05:58
- The original course00:27
- Why choose the Go programming language?12:15
- Hello World!09:37
- Section Overview03:12
- The Terminal06:13
- Installation Insights08:48
- Go Workspace08:48
- Environment Variables06:51
- Windows - Configuring Path Variables08:01
- Mac - Configuring Path Variables10:23
- IMPORTANT - REGARDING LINUX VIDEOS THAT FOLLOW00:21
- Linux - Machine Setup33:39
- Linux - Machine Configuration12:43
- Linux - Configuring Path Variables21:41
- Testing Your Installation04:32
- Section Review04:04
- Section Overview01:51
- Go Editors09:07
- WebStorm & Atom.io06:34
- Creating Your First Project09:03
- Hello World with Webstorm08:14
- The Go Command & Documentation05:47
- Understanding Github07:37
- Using Github14:23
- Section Review03:40
- Section Overview02:03
- How Computers Work - Part I09:56
- How Computers Work - Part II12:29
- Github Update Command08:55
- Numeral Systems04:07
- Binary Numbering System07:40
- Hexadecimal Numbering System07:08
- Text Encoding09:25
- Coding Scheme Programs09:33
- Format Printing09:46
- Section Review09:16
- Section Overview05:50
- Packages07:54
- Go Commands05:46
- Variables08:45
- Scope07:50
- Scope II10:08
- Closure11:14
- Blank Identifier04:25
- Constants09:02
- Constants II07:21
- Words of Encouragement03:42
- Pointers06:19
- Using Pointers07:45
- Remainder05:46
- Section Review15:03
- Section Overview07:04
- For Loop06:53
- Nested Loops06:23
- Conditions, Break, & Continue07:23
- Documentation & Terminology13:01
- Rune06:23
- String Type10:40
- Switch Statements07:27
- If Statements07:08
- Exercise Solutions14:01
- Section Review08:41
- Section Overview01:51
- Intro To Functions07:48
- Func Returns05:27
- Variadic Functions06:49
- Variadic Arguments05:07
- Func Expressions05:41
- Closure06:04
- Callbacks06:49
- Callback Example05:34
- Recursion05:04
- Defer04:20
- Pass By Value06:41
- Reference Types05:06
- Anonymous Self-Executing Functions01:39
- Bool Expressions07:23
- Exercises - Part I07:46
- Exercises - Part II06:40
- Section Review10:27
- Data Structures Overview06:07
- Array08:28
- Array Examples10:45
- Slices10:04
- Slice Examples09:38
- More Slice Examples06:33
- Creating A Slice12:43
- Incrementing A Slice Item05:16
- Section Review12:29
- Maps Introduction06:18
- Map Examples - Part I08:30
- Map Examples - Part II08:46
- Map Examples - Part III05:08
- Map Documentation10:43
- Map Range Loop03:43
- GitHub Pull04:08
- Hash Tables13:52
- Hashing Words10:57
- Hashing Words II12:05
- Build A Hash Table09:13
- Finished Hash Algorithm11:41
- Structs Introduction06:14
- OOP in Go11:37
- User-Defined Types10:46
- Composition10:28
- JSON Marshal11:40
- JSON Unmarshal03:13
- JSON Encode06:41
- JSON Decode05:35
- Interfaces10:24
- Code Substitutability12:26
- Bill Kennedy08:31
- Donovan & Kernighan11:54
- Sort Package10:27
- Sort Solution09:40
- Sort Reverse15:55
- Sort Slice Int03:35
- Empty Interface08:56
- Method Sets11:39
- Conversion vs Assertion10:21
- Concurrency & WaitGroup05:10
- Parallelism04:41
- Race Conditions04:09
- Mutex03:46
- Atomicity03:58
- Review & Channels Preview08:35
- Channels - Introduction09:30
- Range Clause05:36
- N-to-105:22
- Semaphores - Part 107:29
- Semaphores - Part 201:37
- 1-to-N04:14
- Channels as Arguments & Returns07:02
- Channel Direction05:47
- Incrementor With Channels06:01
- Deadlock Challenge07:14
- Factorial Challenge04:43
- Pipeline Pattern06:35
- Factorial Challenge Redux05:37
- Factorial Challenge Redux Solution04:38
- Fan Out / Fan In Pattern - Overview03:32
- Fan In Pattern07:03
- Fan Out / Fan In - Example16:20
- Fan Out / Fan In - Challenge01:31
- Fan Out / Fan In - Solution09:11
- Fan Out / Fan In - Challenge: Factorial02:06
- Fan Out / Fan In - Solution: Factorial11:22
- Deadlock Challenge00:42
- Deadlock Solution04:22
- Incrementor Challenge Revisited01:11
- Incrementor Solution05:44
- Additional Resources04:53
- An Introduction to Error Handling in Go05:41
- Improving Your Code with Golint05:56
- Handling Errors & Logging Errors to a File08:45
- Four Common Ways to Handle Errors05:45
- Custom Errors - Creating Values of Type error07:25
- Idiomatic Error Handling04:28
- Providing Context with Errors05:11
- Providing Even More Context with Errors07:12
- Error Handling Review & Resources04:47
- Congratulations05:58
- Bonus lecture05:37
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more