Beginning C++ Programming - From Beginner to Beyond
Obtain Modern C++ Object-Oriented Programming (OOP) and STL skills. C++14 and C++17 covered. C++20 info see below.
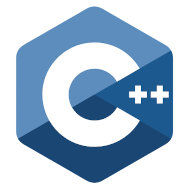
What you will learn?
- Learn to program with one of the most powerful programming languages that exists today, C++.
- Obtain the key concepts of programming that will also apply to other programming languages
- Learn Modern C++ rather than an obsolete version of C++ that most other courses teach
- Learn C++ features from basic to more advanced such as inheritance and polymorphic functions
- Learn C++ using a proven curriculum that covers more material than most C++ university courses
- Learn C++ from an experienced university full professor who has been using and teaching C++ for more than 25 years
- Includes Quizzes, Live Coding Exercises, Challenge Coding Exercises and Assignments
- New Section: Learn to use Visual Studio Code with C++
- New Section: Learn all about using C++ Lambda Expressions
Your trainer

Tim Buchalka's Learn Programming Academy
The Learn Programming Academy was created by Tim Buchalka, a software developer with 35 years experience, who is also an instructor on Udemy, with over 990K + students in his courses on Java, Python, Android, C# and the Spring framework.
The Academy’s goal in the next three years, is to teach one million people to learn how to program.
Apart from Tim’s own courses, which are all available here, we are working with the very best teachers, creating courses to teach the essential skills required by developers, at all levels.
One other important philosophy is that our courses are taught by real professionals; software developers with real and substantial experience in the industry, who are also great teachers. All our instructors are experienced, software developers!
305 lessons
Easy to follow lectures and videos covering subject details.
46 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- About the Course03:39
- Why Learn C++?05:00
- Modern C++ and the C++ Standard02:58
- How does all this work?08:13
- FAQ -- Please Read!00:10
- Installation and Setup Overview02:53
- Installing the C++ Compiler on Windows09:25
- Installing CodeLite on Windows03:41
- Configuring CodeLite on Windows20:39
- Installing the C++ Compiler on Mac OSX03:44
- Installing CodeLite on Mac OSX04:27
- Configuring CodeLite on Mac OSX22:45
- Using the Command-Line interface01:32
- Installing CodeLite on Ubuntu Linux08:48
- Configuring CodeLite on Ubuntu Linux19:00
- Creating a Default CodeLite Project Template (All Versions)08:06
- Using the Command-Line Interface on Windows09:43
- Using the Command-Line Interface on Mac OSX07:49
- Using the Command-Line Interface on Linux (Ubuntu)08:55
- Using a Web-based C++ Compiler03:22
- Using the Included Source Code Course Resources05:08
- Curriculum Overview06:58
- Overview of the Section Challenge Exercises01:29
- Overview of the Section Quizzes01:06
- Section Overview00:58
- An Overview of the CodeLite Interface14:52
- Writing our first program08:23
- Building our first program12:33
- What are Compiler Errors?09:07
- What are Compiler Warnings?03:51
- What are Linker Errors?04:07
- What are Runtime Errors?00:41
- What are Logic Errors?01:49
- Section Challenge01:21
- Section Challenge - Solution05:09
- Section 4 Quiz10 questions
- Section Overview00:56
- Overview of the Structure of a C++ Program04:20
- #include Preprocessor Directive02:11
- Comments07:29
- The main() function03:05
- Namespaces03:56
- Basic Input and Output (I/O) using cin and cout20:39
- Using cout and the insertion operator to say Hi to Frank1 question
- Using cout and the insertion operator1 question
- Using cin and the extraction operator1 question
- Section 5 Quiz10 questions
- Section Overview01:10
- What is a variable?03:08
- Declaring and Initializing Variables14:04
- Declaring and Initializing Variables1 question
- Global Variables03:23
- C++ Built-in Primitive Types15:59
- What is the Size of a Variable (sizeof)06:52
- What is a Constant?05:03
- Declaring and Using Constants18:26
- Section Challenge01:50
- Section Challenge - Solution03:08
- Section 06 Quiz10 questions
- Section Overview01:07
- What is an Array?04:40
- Declaring and Initializing Arrays02:56
- Accessing and Modifying Array Elements14:13
- Declaring, Initializing and Accessing an Array1 question
- Multidimensional Arrays03:11
- Declaring and Initializing Vectors06:26
- Accessing and Modifying Vector Elements15:29
- Declaring, Initializing and Accessing Vectors1 question
- Section Challenge02:07
- Section Challenge - Solution06:03
- Section 07 Quiz10 questions
- Section Overview02:02
- Expressions and Statements03:20
- Using Operators01:58
- The Assignment Operator12:31
- Arithmetic Operators14:55
- Using the Assignment Operator1 question
- Using the Arithmetic Operators1 question
- Increment and Decrement Operators13:11
- Mixed Expressions and Conversions12:56
- Testing for Equality09:04
- Relational Operators04:17
- Logical Operators15:48
- Compound Assignment Operators02:15
- Operator Precedence03:48
- Logical Operators and Operator Precedence - Can you work?1 question
- Section Challenge02:30
- Section Challenge - Solution09:51
- Section 08 Quiz10 questions
- Section Overview03:06
- if Statement18:08
- If Statement - Can you Drive?1 question
- if else Statement05:48
- If-Else Statement - Can you Drive?1 question
- Nested if Statements22:04
- Nested If Statements - Can you Drive?1 question
- switch-case Statement20:14
- Switch Statement - Day of the Week1 question
- Conditional Operator10:37
- Looping03:12
- for Loop22:08
- For loop - Sum of Odd Integers1 question
- range-based for Loop14:59
- Using the range-based for loop1 question
- while Loop16:39
- While loop exercise1 question
- do while Loop13:46
- continue and break02:33
- Infinite Loops03:17
- Nested Loops19:47
- Nested Loops - Sum of the Product of all Pairs of Vector Elements1 question
- Section Challenge06:09
- Section Challenge - Solution Part 114:47
- Section Challenge - Solution Part 210:40
- Section 09 Quiz10 questions
- Section Overview01:54
- Character Functions02:30
- C-Style Strings07:45
- Working with C-style Strings13:45
- Using C-style Strings1 question
- C++ Strings15:28
- Working with C++ Strings16:45
- Using C++ Strings - Exercise 11 question
- Using C++ Strings - Exercise 21 question
- Section Challenge06:48
- Section Challenge - Solution13:15
- Section 10 Quiz10 questions
- Challenge Assignment - Letter Pyramid1 question
- Section Overview02:31
- What is a Function?18:22
- Using Functions from the cmath Library1 question
- Function Definition17:00
- Function Prototypes10:55
- Function Parameters and the return Statement14:52
- Functions and Prototypes - Converting Temperatures1 question
- Default Argument Values13:08
- Using Default Argument Values - Grocery List1 question
- Overloading Functions10:50
- Overloading Functions - Calculating Area1 question
- Passing Arrays to Functions13:33
- Passing Arrays to Functions - Print a Guest List1 question
- Pass by Reference13:23
- Using Pass by Reference - Print a Guest List1 question
- Scope Rules12:09
- How do Function Calls Work?11:23
- inline Functions01:31
- Recursive Functions13:18
- Implementing a Recursive Function - Save a Penny1 question
- Section Challenge02:25
- Section Challenge-Solution16:01
- Section 11 Quiz10 questions
- Section Overview03:14
- What is a Pointer?02:20
- Declaring Pointers03:02
- Accessing the Pointer Address and Storing Address in a Pointer16:33
- Dereferencing a Pointer13:13
- Dynamic Memory Allocation14:03
- The Relationship Between Arrays and Pointers11:37
- Pointer Arithmetic15:06
- Const and Pointers02:34
- Passing Pointers to Functions18:30
- Returning a Pointer from a Function12:11
- Potential Pointer Pitfalls04:10
- What is a Reference?08:50
- L-values and R-values04:03
- Using the CodeLite IDE Debugger10:26
- Section Recap03:31
- Section Challenge04:54
- Section Challenge - Solution09:54
- Section 12 Quiz10 questions
- Section Overview03:13
- What is Object-Oriented Programming?09:54
- What are Classes and Objects?03:23
- Declaring a Class and Creating Objects18:21
- Accessing Class Members17:41
- Creating and Accessing Objects1 question
- public and private07:57
- Implementing Member Methods20:26
- Adding public methods that access private class attributes1 question
- Add more public methods to an existing class1 question
- Constructors and Destructors10:28
- The Default Constructor11:40
- Add a Default Constructor to an Existing Class1 question
- Overloading Constructors02:50
- Add an Overloaded Constructor to an Existing Class1 question
- Constructor Initialization lists11:35
- Delegating Constructors09:01
- Constructor Parameters and Default Values08:28
- Copy Constructor17:49
- Add a Copy Constructor to an Existing Class1 question
- Shallow Copying with the Copy Constructor14:30
- Deep Copying with the Copy Constructor08:53
- Move Constructors17:06
- The 'this' Pointer02:32
- Using const with Classes11:00
- Static Class Members14:23
- Structs vs Classes02:18
- Friends of a class03:32
- Section Challenge12:52
- Section Challenge - Solution11:00
- Section 13 Quiz10 questions
- Section Overview01:34
- What is Operator Overloading?17:06
- Overloading the Assignment Operator (copy)19:03
- Overloading the Assignment Operator (move)15:07
- Overloading Operators as Member Functions17:45
- Operator Overloading as Member Functions1 question
- Overloading Operators as Global Functions14:33
- Operator Overloading as Non-member Functions1 question
- Overloading the Stream Insertion and Extraction Operators10:46
- Operator Overloading the Stream Insertion Operator1 question
- Section Challenge13:22
- Section Challenge - Solution 113:25
- Section Challenge - Solution 207:29
- Section 14 Quiz10 questions
- Section Overview01:53
- What is Inheritance?05:54
- Terminology and Notation05:22
- Inheritance vs. Composition04:01
- Deriving Classes from Existing Classes14:14
- Protected Members and Class Access11:59
- Constructors and Destructors17:31
- Passing Arguments to Base Class Constructors09:27
- Copy/Move Constructors and Operator = with Derived Classes16:35
- Redefining Base Class Methods15:50
- Multiple Inheritance01:41
- The Updated Accounts Example19:48
- Section Challenge05:02
- Section Challenge - Solution14:31
- Section 15 Quiz10 questions
- Section Overview01:22
- What is Polymorphism?17:38
- Using a Base Class Pointer12:38
- Virtual Functions05:21
- Virtual Destructors05:13
- Using the Override Specifier07:06
- Using the Final Specifier01:39
- Using Base Class References07:17
- Pure Virtual Functions and Abstract Classes16:27
- Abstract Classes as Interfaces18:05
- Section Challenge04:21
- Section Challenge - Solution Part 111:33
- Section Challenge - Solution Part 210:46
- Section Challenge - Final Solution06:48
- Section 16 Quiz10 questions
- Section Overview01:54
- Some Issues with Raw Pointers01:44
- What is a Smart Pointer? Ownership and RAII03:48
- Unique Pointers16:36
- Shared Pointers19:14
- Weak Pointers06:11
- Custom Deleters08:12
- Section Challenge 109:30
- Section Challenge 1 - Solution06:27
- Section 17 Quiz10 questions
- Section Overview02:30
- Basic Concepts and a Simple Example: Dividing by Zero16:27
- Throwing an Exception from a Function08:21
- Handling Multiple Exceptions05:28
- Stack Unwinding and How it Works10:26
- Creating User-Defined Exception Classes06:53
- Class Level Exceptions07:01
- The C++ std::exception Class Hierarchy04:11
- Section Challenge04:16
- Section Challenge - Solution05:34
- Section 18 Quiz10 questions
- Section Overview02:21
- Files, Streams and I/O05:41
- Stream Manipulators03:21
- Stream Manipulators - boolean08:28
- Stream Manipulators - integers12:08
- Stream Manipulators - floating point15:21
- Stream Manipulators - align and fill14:09
- Section Challenge 111:00
- Section Challenge 1 - Solution14:11
- Reading from a Text File11:35
- Reading from a Text File - Live Demo - Part 114:05
- Reading from a Text File - Live Demo - Part 205:40
- Reading a text file1 question
- Section Challenge 203:08
- Section Challenge 2 - Solution07:17
- Section Challenge 303:08
- Section Challenge 3 - Solution05:24
- Writing to a Text File09:33
- Writing to a Text File - Live Demo07:32
- Section Challenge 402:29
- Section Challenge 4 - Solution04:03
- Using String Streams14:50
- File locations with some Popular IDEs06:13
- Section 19 Quiz10 questions
- Section Overview01:42
- What is the STL?09:11
- Generic Programming with Macros07:19
- Generic Programming with Function Templates20:38
- Generic Programming with Class Templates14:07
- Creating a Generic Array Template Class13:56
- Introduction to STL Containers04:41
- Introduction to STL Iterators10:47
- Introduction to Iterators - Demo16:01
- Introduction to STL Algorithms10:46
- Introduction to Algorithms - Demo17:08
- Sequence Container - Array22:56
- Sequence Containers - Vector24:56
- Sequence Containers - Deque14:11
- Section Challenge 104:46
- Section Challenge 1 - Solution03:35
- Sequence Containers - List and Forward List22:06
- Section Challenge 211:21
- Section Challenge 2 - Solution07:29
- Associative Containers - Sets15:50
- Associative Containers - Maps16:55
- Section Challenge 308:15
- Section Challenge 3 - Solution06:04
- Container Adaptors - Stack09:27
- Container Adaptors - Queue07:39
- Section Challenge 404:08
- Section Challenge 4 - Solution03:57
- Container Adaptors - Priority Queue08:04
- Section 20 Quiz10 questions
- Section Overview02:14
- Motivation20:34
- Structure of a Lambda Expression04:41
- Stateless Lambda Expressions13:22
- Stateless Lambda Expressions Demo - Part 114:16
- Stateless Lambda Expressions Demo - Part 213:05
- Stateful Lambda Expressions06:21
- Stateful Lambda Expressions Demo - Part112:38
- Stateful Lambda Expressions Demo - Part215:26
- Lambdas and the STL19:50
- Section Overview02:03
- Installing VSCode on Windows04:40
- Building and Running C++ Programs with VSCode on Windows14:04
- Debugging C++ Programs with VSCode on Windows06:08
- Using the Course Source Code with VSCode on Windows08:00
- Installing VSCode on Mac OSX05:45
- Building and Running C++ Programs with VSCode on Mac OSX11:33
- Debugging C++ Programs with VSCode on Mac05:48
- Using the Course Source Code with VSCode on Mac08:47
- Installing VSCode on Linux04:17
- Building and Running C++ Programs with VSCode on Linux11:24
- Debugging C++ Programs with VSCode on Linux05:23
- Using the Course Source Code with VSCode on Linux08:30
- Section Overview02:30
- Motivation06:10
- The Structure of an Enumeration07:37
- Unscoped Enumerations23:21
- Scoped Enumerations13:00
- Installation and Setup Overview02:16
- Installing the C++ Compiler on Windows04:53
- Installing CodeLite on Windows03:06
- Configuring CodeLite on Windows12:02
- Installing the C++ Compiler on Mac OSX02:01
- Installing CodeLite on Mac OSX01:56
- Configuring CodeLite on Mac OSX10:29
- Installing CodeLite on Ubuntu Linux04:04
- Configuring CodeLite on Ubuntu Linux10:26
- Creating a Default CodeLite Project Template (All Versions)06:21
- Using the Included Source Code Course Resources03:44
- Source Code for all Sections00:33
- Bonus - Course Slides and Free Programming EBook02:52
Online Courses
Learning C++ doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C++ related books and take your
YouTube videos
The number of high-quality and free C++ video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more