Unreal Engine 5 C++ Developer: Learn C++ & Make Video Games
Created in collaboration with Epic Games. Learn C++ from basics while making your first 5 video games in Unreal
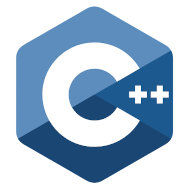
What you will learn?
- How to make games in Unreal Engine 5.
- C++, the games industry standard language.
- Object Oriented Programming and how to put it into practice.
- Game design principles.
- Programming patterns and best practices.
- Artificial Intelligence behaviour programming for enemies.
- Write code that is clean and to understand.
- When to use Blueprint or C++.
- How to achieve anything you want in Unreal using C++.
- Unreal Gameplay Framework to make games easily.
- Strong and transferable problem solving skills.
- Modern game development technologies and techniques.
Your trainer
207 lessons
Easy to follow lectures and videos covering subject details.
29.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- For users of Unreal 4.2200:36
- Welcome To The Course02:39
- Installing Unreal Engine07:13
- Community & Support01:40
- Navigating The Viewport06:36
- Moving & Placing Actors05:27
- C++ versus Blueprint04:29
- Helping Us To Help You07:24
- Section Intro - Warehouse Wreckage01:40
- Project Setup04:16
- Blueprint Event Graph05:30
- Physics Simulation04:36
- Objects and References09:43
- Adding an Impulse08:18
- Blueprint Classes and Instances07:45
- Spawning Actors06:20
- Data Types06:26
- Pawns and Actor Location08:36
- Control Rotation04:50
- Vector Addition & Multiplication06:54
- Get Forward Vector06:13
- Importing Assets10:37
- Geometry Brushes (BSP)08:52
- Materials and Lighting05:50
- Actor Components06:08
- Collision Meshes09:04
- Variables07:47
- Booleans and Branches05:45
- Functions09:11
- Return Types06:48
- Pure Functions06:08
- Member Functions08:33
- Loading Levels & Delay Nodes04:42
- Wrap-up and Recap01:49
- Section Intro - Obstacle Assault03:08
- Project Setup06:33
- Customizing The Character06:25
- Compilers and Editors05:35
- PC - Installing Visual Studio05:50
- Mac - Installing XCode04:27
- Installing VSCode04:16
- Compiling a C++ Project08:56
- UPROPERTY Variables10:37
- Live Coding Issues03:20
- C++ Files & BeginPlay08:00
- Using Structs In C++08:36
- Calling Functions in C++06:14
- Tick06:06
- Local Variables06:58
- Pseudo Code04:07
- Function Return Values08:45
- Velocity & DeltaTime07:46
- Scope Resolution Operator08:34
- If Statements07:33
- Using Member Functions06:06
- Blueprint Child Classes09:07
- Forcing Character Collisions11:35
- GameMode06:25
- Writing To The Output Log09:57
- FString07:57
- Member Functions13:25
- Return Statements09:05
- Const Member Functions05:35
- FRotator10:08
- Level Design & Polish09:47
- Obstacle Assault: Wrap-Up02:20
- Section Intro - Crypt Raider04:33
- Project Setup06:23
- Modular Level Design09:56
- Modular Level Layout12:30
- Solution: Modular Level Layout14:24
- Light Types08:38
- Lumen & Light Bleed11:56
- Level Lighting09:13
- Character Blueprint06:24
- Inheritance vs Composition04:50
- C++ Actor Component04:36
- Pointer Types & GetOwner()08:44
- Dereferencing & Arrow (->) Operator07:30
- Linkers, Headers and Includes07:51
- FMath::VInterpConstantTo13:05
- Scene Components09:19
- Line Tracing & Sweeping06:30
- GetWorld()08:12
- DrawDebugLine()08:15
- References vs Pointers08:37
- Const References & Out Parameters09:32
- Geometry Sweeping10:10
- Input Action Mappings06:00
- Blueprint Callable06:45
- FindComponentByClass() & nullptr09:11
- DrawDebugSphere()07:29
- Grabbing With Physics Handle11:53
- Waking Physics Objects08:27
- Returning Out Parameters06:08
- Overlap Events14:37
- Constructors08:50
- TArray08:13
- While & For Loops08:55
- Range Based For Loops02:37
- Actor Tags05:23
- Early Returns05:44
- Dependency Injection09:16
- Casting & Actor Attachment05:52
- Adding and Removing Tags06:20
- Boolean Logical Operators08:00
- Level Polish13:05
- Crypt Raider: Wrap-Up02:07
- Project Intro06:19
- Pawn Class Creation07:52
- Creating Components10:50
- Forward Declaration13:23
- Constructing The Capsule06:31
- Static Mesh Components06:15
- Deriving Blueprint Classes05:19
- Instance vs Default08:37
- Editing Exposed Variables14:07
- Exposing The Components11:37
- Creating Child C++ Classes12:06
- Possessing The Pawn04:07
- Handling Input14:04
- Local Offset12:31
- Movement Speed13:46
- Local Rotation17:57
- Casting11:16
- Using the Mouse Cursor16:46
- Rotating the Turret20:33
- The Tower Class12:45
- Fire08:34
- Timers13:39
- The Projectile Class05:58
- Spawning The Projectile11:44
- Projectile Movement Component08:31
- Hit Events14:34
- Health Component15:15
- Applying Damage14:40
- The Game Mode Class07:35
- Handling Pawn Death20:48
- Custom Player Controller11:20
- Starting The Game10:19
- The Start Game Widget12:15
- Countdown Timer13:58
- Displaying Countdown Time07:45
- Winning And Losing14:12
- Game Over HUD08:39
- Hit Particles10:05
- Smoke Trail06:36
- Death Particles06:39
- Sounds10:46
- Camera Shake14:57
- Polish And Wrap-Up12:40
- Section Intro: Simple Shooter02:02
- Project Setup09:55
- Pawns vs Characters in C++12:33
- Character Movement Functions18:11
- Controller Aiming13:30
- Third Person Camera Spring Arm08:57
- Skeletal Animations 10108:20
- Editing Collision Meshes05:09
- Animation Blueprints 10113:16
- 2D Blend Spaces10:39
- Connecting Animation To Gameplay08:59
- Inverse Transforming Vectors09:38
- Calculating Animation Speeds12:05
- Gun Actors11:34
- Spawning Actors At Runtime06:37
- Attaching To Meshes Via Sockets10:20
- Shooting Architecture06:14
- Spawning Particle Effects08:14
- Player View Point10:52
- Line Tracing By Channel12:40
- Impact Effects04:37
- Dealing Damage To Actors08:04
- Virtual Methods In C++10:15
- Overriding TakeDamage09:06
- Blending Animations By Booleans04:42
- Blueprint Pure Nodes06:28
- Create and Setup an AI controller03:59
- AI Aiming06:13
- Nav Mesh And AI Movement10:48
- Checking AI Line Of Sight07:39
- BehaviorTrees And Blackboards08:06
- Setting Blackboard Keys In C++05:54
- Behavior Tree Tasks And Sequences08:24
- BT Decorators And Selectors12:12
- Custom BTTasks In C++07:53
- Executing BTTasks10:31
- BTTasks That Use The Pawn10:48
- BTServices In C++15:05
- Ignoring Actors In Line Traces06:04
- Ending The Game10:16
- Setting Timers In C++10:51
- Displaying A Lose Screen11:32
- Iterating Over Actors10:25
- Calculating The Win Condition12:20
- Refactoring PullTrigger07:53
- Weapon Sound Effects06:35
- Randomized Sound Cues08:35
- Sound Spatialization06:45
- Crosshairs and HUDs07:42
- Health Bars07:50
- AimOffsets12:05
- Animation State Machines10:03
- Complex State Machines13:00
- Wrap-up And Challenges08:49
- For users of Unreal 4.2200:27
- Bonus Lecture00:28
Online Courses
Learning C++ doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C++ related books and take your
YouTube videos
The number of high-quality and free C++ video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more