Rust: Building Reusable Code with Rust from Scratch
Use generics, traits, and macros to write clean and reusable Rust libraries that are easy to understand and maintain
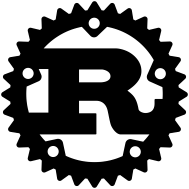
What you will learn?
- This course is aimed at developers, C/C++, Rust & System developers who are familiar with programming and want to learn how to code in Rust and re-use Rust code and libraries from scratch.
Your trainer

Packt Publishing
Packt has been committed to developer learning since 2004. A lot has changed in software since then - but Packt has remained responsive to these changes, continuing to look forward at the trends and tools defining the way we work and live. And how to put them to work.
With an extensive library of content - more than 4000 books and video courses -Packt's mission is to help developers stay relevant in a rapidly changing world. From new web frameworks and programming languages, to cutting edge data analytics, and DevOps, Packt takes software professionals in every field to what's important to them now.
From skills that will help you to develop and future proof your career to immediate solutions to every day tech challenges, Packt is a go-to resource to make you a better, smarter developer.
68 lessons
Easy to follow lectures and videos covering subject details.
6.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- The Course Overview01:34
- Bindings and Mutability02:15
- Built-In Types12:00
- Imports and Namespaces07:24
- The Standard Library04:24
- Recursive Fibonacci05:14
- Dynamic Fibonacci07:23
- Installing Rust with Rustup02:17
- Managing Toolchains with Rustup02:29
- Creating Projects with Cargo08:15
- Exploring the Crate Ecosystem05:16
- Rustdoc and the Documentation Ecosystem04:36
- Adding Dependencies with Cargo14:01
- Motivation for the Borrow Checker03:53
- Ownership, Borrowing, and RAII06:27
- Shared and Exclusive Access12:26
- Fighting with the Borrow Checker02:10
- Strings, Strs, Vecs, and Slices03:26
- Understanding Borrow Checker Errors01:14
- Structured Data08:20
- Enumerations04:35
- Match Expressions10:56
- Designing a Markup Language04:37
- Implementing the Markup Language13:19
- Introduction to Traits02:05
- Built-In Traits03:00
- Writing Your Own Traits07:58
- Generic Functions05:40
- Generic Types02:02
- Trait Objects and Dynamic Dispatch04:13
- Closures06:08
- Iterators05:17
- Map, Filter, and Fold05:05
- Building a Barycenter Finder10:58
- Parallelizing the Barycenter Finder11:10
- Breaking Up Code with Modules03:47
- Error Handling03:00
- API Design02:34
- Unit Testing18:00
- Integration Testing06:48
- Documentation07:27
- Test Your Knowledge5 questions
- The Course Overview03:15
- Setting Up the Rust Development Environment03:54
- Exploring Code Reuse in Rust03:15
- Loops and Iterators05:40
- Using Functional Programming Loops13:49
- Functions in Rust03:06
- Exploring Generics02:26
- Use Generic Functions to Reuse Algorithms03:49
- Reuse Structures in Enums and Structs02:30
- Working with Generic in Struct Methods03:43
- Generics in the Rust Standard Library – Part I03:45
- Generics in the Rust Standard Library – Part II05:48
- Exploring Traits03:12
- Using Trait Bounds and Trait Objects to Communicate Interfaces03:41
- Associated Types versus Generics and Trait Inheritance02:56
- Exploring Traits, Generics, and Performance03:30
- Traits in the Rust Standard Library – Part I07:42
- Traits in the Rust Standard Library – Part II06:24
- Write Code with Code – Metaprogramming in Rust02:28
- Use Declarative Macros to Write Less Code04:05
- Using Procedural Macros for Custom Derive04:43
- Macros in the Rust Standard Library – Part I04:55
- Macros in the Rust Standard Library – Part II05:39
- Introducing Crates05:05
- Using Modules to Define the Structure of Crates04:27
- Using a Crate with Cargo.toml06:06
- Publishing to crates.io02:47
- Test Your Knowledge5 questions
Online Courses
Learning Rust doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Rust related books and take your
YouTube videos
The number of high-quality and free Rust video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more