Mastering Go Programming
Master programming with Golang by learning how to write idiomatic, effective code
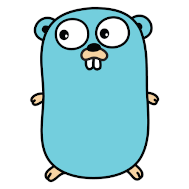
What you will learn?
- Apply modern software design patterns utilizing the Go language
- Take a deep dive into Go’s master topics like advanced concurrency patterns, and the flexible reflection capabilities
- Write unit tests and benchmarks for your Go code
- Create advanced backend microservices for the Hydra spaceship software and design an end to end chat system
- Handle JSON, XML, and CSV data formats in your code
- Interact with Mongodb, MySQL and BoltDB
- Build powerful communications layer for your microservices using protocol buffers, TCP, and UDP
- Develop powerful web applications and Restful APIs utilizing http requests, forms, and cookies
- Design beautiful dynamic UI for the hydra software utilizing Go templates and websockets
- Secure your applications with certificates, https, and secure web sockets
Your trainer

Packt Publishing
Packt has been committed to developer learning since 2004. A lot has changed in software since then - but Packt has remained responsive to these changes, continuing to look forward at the trends and tools defining the way we work and live. And how to put them to work.
With an extensive library of content - more than 4000 books and video courses -Packt's mission is to help developers stay relevant in a rapidly changing world. From new web frameworks and programming languages, to cutting edge data analytics, and DevOps, Packt takes software professionals in every field to what's important to them now.
From skills that will help you to develop and future proof your career to immediate solutions to every day tech challenges, Packt is a go-to resource to make you a better, smarter developer.
56 lessons
Easy to follow lectures and videos covering subject details.
20 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- The Course Overview12:33
- Welcome to the World of Go!11:52
- How to Write Go Code?18:14
- Let’s Build a Rest API Client in Go!18:32
- Packages, Variables, and Functions12:22
- Flow Control06:20
- Applying Your New Knowledge – Binary Search Algorithm10:20
- Structs, Slices, and Maps10:45
- Applying Your New Knowledge – Let’s Create a Set05:26
- Slicing Slices14:43
- Methods and Interfaces – Linked List Data Structure Implementation17:10
- Concurrency in Go – goroutines07:40
- Concurrency in Go – Channels13:49
- Concurrency in Go – Select07:45
- Errors and Troubleshooting13:54
- A Tour into Go’s Packages15:50
- Project Hydra –Write the First Piece of the Spaceship Web Server06:51
- A Closer Look at Interfaces and Methods in Go 123:03
- A Closer Look at Interfaces and Methods II10:44
- Factory Design Pattern in Go11:58
- The Singleton Pattern –Building the Hydra Custom Logger10:31
- Builder Pattern in Go – Construct the Ship’s Defense Shields06:12
- Syncs and Locks26:58
- Timers and Tickers20:09
- Channel Generators05:58
- Pipelines –Build the Hydra Chat System28:19
- Pipelines – Build the Hydra Chat System (Continued)29:19
- Go Laws of Reflection34:53
- Reflection on Structs and Interfaces32:50
- Hydra – Custom Configuration File Reader31:31
- Unit Testing in Go24:25
- Benchmarking in Go11:37
- Practices, Cross-Compilation, and the Go Tool12:16
- File Handling in Go25:24
- Go and JSON Format32:47
- Go and the XML Format34:16
- Go and CSV Format20:05
- Go with MySQL27:51
- Go with MongoDB I21:29
- Go with MongoDB II21:32
- Go with BoltDB22:41
- Building Hydra’s Database Interface21:57
- TCP communication – Clients20:36
- TCP Communication – Servers18:56
- UDP Communications36:18
- Protocol Buffers with Go37:17
- Hydra Protocol Buffer Layer38:35
- Web Server Applications in Go42:31
- Client and RESTful API Application in Go36:25
- Build Hydra’s RESTful API I22:52
- Build Hydra’s RESTful API II26:20
- Building the Hydra Website – Templates34:53
- Building the Hydra Website – Backend27:20
- Hydra Chat Portal I32:16
- Hydra Chat Portal II46:59
- Web Security38:08
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more