Master Laravel with Vue.js Fullstack Development
Learn how to build a Single Page Application with Laravel PHP Framework and Vue.js
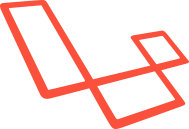
What you will learn?
- Using Vue to build a Single Page Application
- Using Laravel to build a robust API backend for any application
- Combining Laravel with Vue to create a powerful SPA
- Vue/API SPA Authentication using Laravel Sanctum
- Using Vue Router
- Using VueX for global state management
144 lessons
Easy to follow lectures and videos covering subject details.
17.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction02:26
- Required and optional software for the course05:04
- MacOS specific setup05:14
- Windows specific setup03:35
- Where to find the full source code?03:57
- Visual Studio Code - extensions, shortcuts and tricks07:27
- Creating a new Laravel project using Composer03:40
- Setting up Vue.js application13:17
- Fallback route in Laravel05:57
- Installing and setting up Vue Router13:02
- HTML5 history mode in Vue Router04:43
- Vue component registration (global/local) and Index component07:38
- Router Link component and routing inside a Vue application02:27
- Bootstrap, styling and Laravel Mix04:13
- v-bind, v-bind shorthand and named Vue routes05:24
- Styling the navigation bar03:23
- Single file Vue components04:09
- Child components (using components in other components)08:51
- Component props and prop types09:43
- One-way data flow04:59
- Lifecycle hooks06:41
- Component state and reactivity11:38
- Reactivity gotchas07:18
- Conditional rendering (v-if)03:58
- List rendering and v-else09:01
- Computed properties09:52
- Component methods13:24
- Setting up database06:38
- Specified key was too long problem02:52
- Model and migrations06:37
- Model factory, database seeder and faker12:01
- Routes and model all() method07:42
- API testing in Postman07:47
- Using Model::find() and mandatory/optional route parameters06:36
- Using Model::findOrFail() and Accept header03:33
- JavaScript Promises explained13:05
- Making HTTP requests with Axios06:45
- CSS Flexbox and Bootstrap utility classes02:53
- Vue router parameters07:05
- Passing all object properties as props (v-bind trick)05:57
- Vue router links02:27
- Understanding CSS grid system05:29
- Laravel controllers09:38
- Resource controllers04:23
- API Resources10:14
- Availability component (horizontal forms, scoped styles)10:37
- Form input binding (v-model)03:57
- Handling DOM events in Vue04:47
- Laravel Debugbar (seeing what happens behind the scenes)03:22
- Booking model and relations04:54
- Seeding bookings (generating random, non overlapping time series)13:57
- Single Action Controller04:43
- Request input validation (validating data clients send to our server)07:22
- Eloquent Local Query Scopes10:40
- Returning bookable availability (HTTP response statuses)05:17
- Getting object availability in Vue.js08:04
- Displaying form errors09:46
- Final touches - displaying availability03:40
- ReviewList component markup (using responsive grid options)11:57
- Review model & migration (using UUID as model primary key)07:20
- Review model relationships (one-to-one, one-to-many)03:52
- Review model factory & seeder08:43
- Reviews controller (using latest() query scope)06:52
- Reviews HTTP resource (serializing model)03:59
- Loading reviews in Vue component09:54
- Vue filters and moment.js07:19
- Star Rating component (using FontAwesome)09:47
- Star Rating component logic (calculating stars to display from average rating)14:03
- Review list cleanup and section wrap-up02:48
- Review Page component markup and route07:43
- Emitting and handling custom Vue.js events09:12
- Parent and child components communication03:48
- v-model explained, adding v-model support to custom components06:32
- Leaving a rating flow explained07:14
- Checking if review already exists on backend07:44
- Checking if review already exists on frontend06:44
- Booking review key and Eloquent model events09:10
- Fetching booking by review key (custom model methods)07:55
- Creating Resource classes for booking05:35
- Understanding promise chaining11:44
- Displaying the booking information on review page10:23
- A new API endpoint for storing a review (validation rules, complex flow)12:04
- Handling request errors in Vue13:13
- Storing a new review (using POST in axios)05:00
- Fixing a 500 error in BookingByReview controller03:06
- FatalError component (custom Vue component for unhandled errors)04:45
- Handling only specific errors (HTTP error codes, validation errors)11:07
- Validation on Review sending page06:16
- Reusable validation errors component10:36
- Vue Mixins - how to reuse the same code in different components08:55
- Async/Await explained03:34
- Using async/await in Review component07:53
- Vue Component Slots08:16
- Fixing application Vue issues02:47
- Vuex introduction - managing global state05:01
- Setting up Vuex11:29
- State mutations - storing last search dates02:51
- Reading Vuex state02:49
- Binding to Vuex state06:43
- Using Vuex actions to add side effects when committing to the store07:45
- Initializing Vuex state from the browser local storage06:40
- Vue Transitions07:14
- Using animated icons03:36
- Bookable price (controller, migration, factory), Carbon date library09:37
- Async/Await in Availability, emitting a custom event06:20
- Loading price in Bookable component and using Vuex bindings06:15
- Price breakdown component09:05
- Vuex - a basket (adding/removing)08:02
- Vuex getters - how many items in the basket?06:47
- Checking what's in the basket, removing from the basket11:31
- Vuex getters - property vs method access, higher order functions, composition07:22
- Keeping basket state in localStorage08:53
- Creating a Basket/Checkout page07:49
- Rendering contents of the basket and allowing removal08:01
- List transitions - animating adding/deleting to a list (v-for)04:59
- Checkout form markup09:06
- Address model and booking changes10:57
- Checkout logic explained04:55
- Validating nested fields06:08
- Validating arrays of input13:40
- Storing booking and address11:47
- Refactoring price calculations07:07
- Binding form07:01
- Making a booking10:05
- Empty basket state04:37
- Clearing the basket after purchase05:25
- Displaying booking errors08:05
- Rendering purchase confirmation07:48
- Laravel Sanctum00:23
- Laravel Sanctum introduction09:25
- Laravel Sanctum installation06:13
- Sanctum configuration - domains02:24
- Understanding authentication with Sanctum16:43
- Authentication using Vue practical example05:38
- Logic component (page) markup11:16
- Login component logic07:47
- Is user authenticated - local storage? (Part I)07:21
- Is user authenticated - VueX? (Part II)13:38
- Handling unauthenticated state (401) - using Axios interceptors08:11
- Header changes and logout12:20
- Fixing "is logged in" delay03:13
- Registration component markup06:42
- Registration component logic06:55
- Bonus!00:04
Online Courses
Learning Laravel doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Laravel related books and take your
YouTube videos
The number of high-quality and free Laravel video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more