Go Bootcamp: Master Golang with 1000+ Exercises and Projects
Master and Deeply Understand Google's Go from Scratch with Illustrated In-Depth Tutorials & 1000+ Hands-On Exercises.
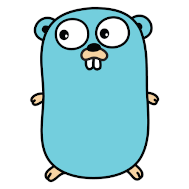
What you will learn?
- Learn from a Go Contributor
- Learn Go Tips & Tricks that you can't find easily anywhere else
- Go from a total Go beginner to a confident Go programmer
- Practice Go with 1000+ Exercises (with included solutions)
- Understand Go In-Depth with Animated Illustrations (Pass Interviews)
- Learn the Go internals and common Go idioms and best-practices
- Create a Log File Parser that parses log files
- Create a Spam Masker that masks spammy words within a block of text
- Create a command-line Retro Led Clock that shows time
- Create Console Animations, Dictionary Programs, and more
Your trainer

Jose Portilla
Jose Marcial Portilla has a BS and MS in Mechanical Engineering from Santa Clara University and years of experience as a professional instructor and trainer for Data Science and programming. He has publications and patents in various fields such as microfluidics, materials science, and data science technologies. Over the course of his career he has developed a skill set in analyzing data and he hopes to use his experience in teaching and data science to help other people learn the power of programming the ability to analyze data, as well as present the data in clear and beautiful visualizations. Currently he works as the Head of Data Science for Pierian Data Inc. and provides in-person data science and python programming training courses to employees working at top companies, including General Electric, Cigna, The New York Times, Credit Suisse, McKinsey and many more. Feel free to contact him on LinkedIn for more information on in-person training sessions or group training sessions in Las Vegas, NV.
196 lessons
Easy to follow lectures and videos covering subject details.
15.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Installation and Resources00:26
- Introduction to Variables08:05
- Example: Path Separator05:31
- When to use a short declaration?06:11
- Let's convert a value!07:09
- ⭐️ Get input from terminal ⭐️04:54
- Learn the basics of os.Args04:06
- Naming Things: Recommendations08:25
- ★ EXERCISES ★00:18
- What is a Raw String Literal?06:35
- How to get the length of a utf-8 string?04:36
- Example: Banger: Yell it back!04:41
- ★ STRINGS EXERCISES ★00:02
- Constants and iota09:53
- ★ IOTA EXERCISES ★00:01
- ⭐️ Print Formatted Output ⭐️00:05
- Println vs Printf07:44
- What is an Escape Sequence?04:08
- How to print using Printf?07:47
- The verbs can be type-safe too!05:09
- ★ PRINTF EXERCISES ★00:06
- ⭐️ If Statement ⭐️00:16
- If Statement05:12
- Else and Else If03:51
- ★ IF STATEMENT EXERCISES ★00:04
- Tiny Challenge: Validate a single user02:34
- Solution: Validate a single user07:50
- Tiny Challenge: Validate multiple users01:40
- Solution: Validate multiple users06:46
- ⭐️ Error Handling ⭐️00:08
- What is a nil value?04:27
- What is an error value?06:30
- Error handling example03:43
- Challenge: Feet to Meter00:53
- Solution: Feet to Meter03:12
- What is a Simple Statement?04:15
- Scopes of simple statements06:13
- Famous Shadowing Gotcha05:12
- ★ ERR HANDLING EXERCISES ★00:05
- ⭐️ Switch Statement ⭐️00:08
- Learn the Switch Statement Basics09:25
- What is a default clause?03:26
- Use multiple values in case conditions02:25
- Use bool expressions in case conditions03:51
- How does the fallthrough statement work?06:52
- What is a short switch?03:00
- Tiny Challenge: Parts of a Day04:03
- Solution: Parts of a Day03:36
- If vs Switch: Which one to use?05:58
- ★ SWITCH EXERCISES ★00:02
- ⭐️ Loops ⭐️00:07
- There is only one loop statement in Go06:02
- How to break from a loop?05:23
- How to continue a loop? (+BONUS: Debugging)05:25
- Create a multiplication table05:40
- How to loop over a slice?05:22
- For Range: Learn the easy way!07:25
- ★ LOOP EXERCISES #1 ★00:03
- Randomization in Go00:07
- Randomization and Go07:08
- Seed the randomizer with time04:11
- Write the Game Logic07:30
- Prove Yourself: Randomization5 questions
- ★ RANDOMIZATION EXERCISES ★00:02
- Mini Project: Word Finder00:05
- Build the Word Finder Program07:35
- Labeled Break and Continue09:08
- Break from a Switch using Labels04:09
- Yes there is a "goto" statement in Go05:18
- Prove Yourself: Labeled Statements5 questions
- ★ LABELED STATEMENT EXERCISES ★00:02
- Learn Go's Fixed Arrays00:24
- Introduction and Roadmap06:09
- What is an array in Go?09:19
- Let's create an array04:57
- Learn the gotcha when using a for range on arrays07:33
- Prove Yourself: Arrays #15 questions
- What is a composite literal?05:06
- Refactor the Hipster's Love Bookstore to array literals06:19
- Tiny Challenge #1: Moodly02:27
- Can you compare array values?07:48
- Can you assign an array value to another one?06:47
- ★ ARRAYS EXERCISES #1 ★00:04
- How to use multi-dimensional arrays?08:45
- Tiny Challenge #2: Moodly04:14
- Learn the rarely known feature of Go: The Keyed Elements08:46
- Learn the relation between composite and unnamed types10:43
- Prove Yourself: Arrays #25 questions
- Recap: Arrays04:09
- ★ ARRAYS EXERCISES #2 ★00:03
- Grab the Slides!00:02
- Challenge: Retro Led Clock06:41
- Challenge Explanation00:33
- Let's print the digits07:26
- Let's print the clock06:49
- It's time to animate the clock!08:16
- ★ RETRO CLOCK EXERCISES ★00:27
- Slices: Master Go's Dynamic Arrays00:24
- Introduction and Roadmap03:09
- Learn the differences between slices and arrays06:00
- Can you compare a slice to another one?10:15
- Create a unique number generator07:14
- Prove Yourself: Slices vs Arrays5 questions
- ★ SLICE EXERCISES ★00:07
- Append: Let's grow a slice!07:25
- Prove Yourself: Appending5 questions
- ★ APPEND EXERCISES ★00:08
- Slicing: Let's cut that slice!09:33
- How to create pagination using slices? (+ Sprintf)04:47
- Prove Yourself: Slicing5 questions
- ★ SLICING EXERCISES ★00:03
- ⭐️ Slice Internals ⭐️00:10
- What is a Backing Array?10:45
- Prove Yourself: Backing Array5 questions
- What's a slice header?05:28
- What does a slice header look like in the actual Go runtime code?07:28
- Prove Yourself: Slice Header5 questions
- What is the capacity of a slice?05:09
- Extend a slice using its capacity06:03
- Prove Yourself: Capacity5 questions
- When does the append function create a new backing array?07:16
- Animate: When the backing array of a slice grows?06:17
- Prove Yourself: Mechanics of Append4 questions
- ★ SLICE INTERNALS EXERCISES ★00:25
- ⭐️ Advanced Operations ⭐️00:09
- Full Slice Expressions: Limit the capacity of a slice06:03
- make(): Preallocate the backing array09:22
- copy(): Copy elements between slices07:39
- How to use multi-dimensional slices?09:18
- Prove Yourself: Advanced Slice Operations5 questions
- ★ ADVANCED SLICE OPS EXERCISES ★00:48
- Build an Empty File Finder Program00:16
- Fetch the Files05:27
- Write to a file04:28
- Optimize!04:15
- ★ FILE FINDER EXERCISES ★00:07
- Project: Animate a Bouncing Ball00:29
- Challenge04:10
- Challenge Document01:32
- Step #1: Create and Draw the Board06:26
- Step #2: Optimize by adding a Buffer07:02
- Step #3: Animate the Ball06:21
- ★ BOUNCING BALL EXERCISES ★00:35
- Learn how to encode and decode UTF-8 strings00:13
- Introduction and Roadmap01:22
- ★ Resources ★00:22
- Let's learn the basics of bytes, runes and strings03:36
- Let's write a character-set program06:44
- Let's convert, index, and slice bytes, runes and strings11:27
- How can you decode a string?07:48
- String Header: Why strings are immutable?08:15
- Recap: Strings Revisited01:40
- Prove Yourself: Strings, Bytes, and Runes5 questions
- ★ UTF-8 EXERCISES ★00:04
- Project: Let's Build a Spam Masker00:08
- Challenge03:04
- Challenge Document00:53
- Detect the link patterns05:03
- Mask the links06:17
- ★ Text Wrapper Challenge ★00:43
- Let's build a Unicode text wrapper06:01
- Learn Go's Maps and Internals00:09
- Create an English to Turkish dictionary08:28
- Populate the dictionary08:13
- Map Internals: How maps work behind the scenes?10:45
- Prove Yourself: Maps5 questions
- ★ MAP EXERCISES ★00:10
- Scan for Input using bufio.Scanner00:07
- Scan user input using bufio.Scanner07:07
- Use maps as sets09:22
- Create a Log Parser using maps and bufio.Scanner07:39
- Prove Yourself: Input Scanning5 questions
- ★ SCANNER EXERCISES ★00:30
- Structs: Organize Data00:09
- What is a struct?04:33
- Let's create a struct!07:40
- When can you compare struct values?08:11
- Go OOP: Struct Embedding06:46
- Rewrite: Log Parser to Structs05:44
- Encode values to JSON09:11
- Decode values from JSON06:42
- Prove Yourself: Structs5 questions
- ★ STRUCT EXERCISES ★00:18
- Functions: The Building Blocks00:15
- Learn the function basics09:35
- Confine variables to a function10:06
- Rewrite: Log Parser using functions08:11
- Learn the Pass By Value Semantics07:58
- Prove Yourself: Functions5 questions
- ★ FUNC EXERCISES ★00:27
- Pointers: Indirectly update data00:12
- What is a pointer?10:55
- Learn the pointer mechanics10:15
- Learn how to work with pointers to composite types08:01
- Rewrite the Log Parser using Pointers06:37
- Pointers or Values? Be Consistent07:24
- Prove Yourself: Pointers5 questions
- ★ POINTER EXERCISES ★00:20
- Methods: Grab the code00:10
- Methods: Enhance types with additional behavior11:02
- Pointer Receivers: Change the received value10:28
- Non-Structs: Attach methods to almost any type07:54
- Interfaces: Grab the code!00:15
- Interfaces: Be dynamic!11:37
- Type Assertion: Extract the dynamic value!11:48
- Empty Interface: Represent any type of value10:25
- Type Switch: Detect and extract multiple values06:27
- Promoted Methods: Let's make a little bit of refactoring09:32
- Famous Interfaces: Grab the code!00:33
- Don't interface everything!11:45
- Stringer: Grant a type the ability to represent itself as a string09:46
- Sorter: Let a type know how to sort itself09:50
- Marshalers: Customize JSON encoding and decoding of a type08:39
- Bonus Lecture00:13
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more