Kotlin for Android & Java Developers: Clean Code on Android
Become a professional Kotlin developer and write cleaner code in your Android apps than in Java, avoid boilerplate code
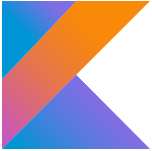
What you will learn?
- Write clean code with Kotlin
- Avoid ceremony and boilerplate code around Android APIs
- Use functional programming to write concise, expressive code
- Create Android apps with Kotlin
- Write readable SQLite transactions using extension functions in Kotlin
- Use the Kotlin Android Extensions to avoid findViewById()
- Create multiple activities, layouts and menus
Your trainer

Peter Sommerhoff
Hi, it's Peter, glad you made it here! If you're anything like me, you want to always keep learning, master new skills, and ultimately achieve your life goals -- whether that's a well-paying career, traveling the world, working from the comfort of your home, or sharing your expertise with and helping other people.
Does that sound like you? Then make sure to enroll in one my courses and learn about awesome programming languages, software design, productivity, or anything else you're interested in.
I feel honored for every student I have and will make sure you have a great learning experience. You can always ask me personally if you get stuck or have anything at all I can help you with.
So please check out my courses below and I'll see you there!
105 lessons
Easy to follow lectures and videos covering subject details.
9 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- What You'll Learn in this Course04:51
- Make the Most of this Course!02:43
- Who's Using Kotlin?02:51
- Introduction01:44
- Install the Java JDK 803:11
- Install Android Studio07:11
- Set Up a Project in Android Studio12:04
- Recap00:28
- Introduction01:26
- Variables and Data Types08:26
- Null Safety in Kotlin08:55
- Conditionals: "if" Expressions08:20
- Conditionals: "when" Expressions08:35
- Collections09:41
- "for" Loops05:15
- "while" Loops03:41
- Functions06:22
- Get Ready for Serious Kotlin Coding (Set up IntelliJ IDEA)04:59
- Create a main() Function02:32
- Challenge: Reading the User's Name from the Console00:25
- Challenge: Using Collections and Loops00:26
- Named Arguments & Default Parameter Values05:43
- Exceptions06:12
- Why No Checked Exceptions?05:31
- Recap01:52
- Introduction01:22
- What is Functional Programming?03:10
- Functional Programming II04:14
- Lambdas & Higher-Order Functions10:05
- Use map() and flatMap()07:46
- Use take() and drop()06:27
- Use zip()04:44
- Challenge: Functional Programming for Data Analysis00:40
- Chain Functions Together06:19
- Lazy Sequences08:17
- Case Study: Performance of Lazy Sequences08:10
- Code Along: Infinite Lazy Sequence of All Prime Numbers12:28
- Use "let" Operator for Scoping and Nullables06:26
- Use "with" for Many Calls on the Same Object03:55
- Use "use" for Closeable (like try-with-resources)04:29
- Inline Functions06:59
- Recap02:23
- Introduction01:58
- Classes02:26
- Properties with Getters & Setters06:01
- Primary and Secondary Constructors08:26
- Methods03:46
- Extension Functions06:23
- Data Classes09:50
- Enums06:12
- Inheritance05:21
- Code Along: Inheritance Example05:57
- Abstract Classes05:46
- Interfaces07:18
- Overriding Rules08:52
- Smart Casts07:55
- Visibilities08:47
- Companion Objects for "Static" Members06:31
- Object Declarations as Singletons05:09
- Packages and Imports04:15
- Generic Classes07:52
- Generic Functions04:59
- Covariance07:06
- Covariance: Java vs Kotlin10:34
- Covariance vs Contravariance: "out" and "in"09:44
- Recap01:46
- Introduction02:50
- Create the App Project04:51
- Understand the Android Versions03:08
- Create a CardView Layout and Add UI Elements09:43
- Use the "Kotlin Android Extensions"08:55
- Challenge: Set Texts and Image Source Programmatically00:19
- Challenge Solution03:30
- Introduction01:01
- What is a RecyclerView?05:17
- Create the Data Class "Habit"04:22
- Implement the "HabitsAdapter" - Part I05:00
- Implement the "HabitsAdapter" - Part II03:35
- Implement the "HabitsAdapter" - Part III09:43
- Introduction01:36
- Add a Menu01:57
- Add a Second Activity03:09
- Use Intents to Switch Activities07:14
- Challenge: Build the Activity Layout00:30
- Challenge Solution08:01
- Let Users Choose an Image06:44
- Get the Selected Image Back (onActivityResult)08:11
- Show Error Messages to the User07:39
- Code Along: An Extension Function for "EditText"03:42
- Introduction01:11
- Introducing SQLite01:28
- Define the Contracts04:14
- Implement the Database Helper06:32
- Store a Habit to SQLite08:03
- Implementing Secure Transactions (that support Rollback)04:08
- Clean Code: Improving Transactions in Kotlin06:51
- Clean Code: Further Improving Transactions and Performance06:33
- Store the Habit in "CreateHabitActivity"09:22
- Read All Habits from SQLite09:00
- Challenge: Improve SQLiteDatabase.query(...)00:12
- Challenge Solution04:27
- Challenge: Improve Cursor.getString(...)00:13
- Challenge Solution07:58
- Add Files to the Virtual Device (Excursus)01:42
- Bonus: Reach Your Full Potential As A Software Developer00:15
Online Courses
Learning Kotlin doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Kotlin related books and take your
YouTube videos
The number of high-quality and free Kotlin video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more