JavaScript - The Complete Guide 2023 (Beginner + Advanced)
Modern JavaScript from the beginning - all the way up to JS expert level! THE must-have JavaScript resource in 2023.
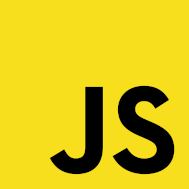
What you will learn?
- Learn JavaScript from scratch and in great detail - from beginner to advanced
- All core features and concepts you need to know in modern JavaScript development
- Everything you need to become a JavaScript expert and apply for JavaScript jobs
- Project-driven learning with plenty of examples
- All about variables, functions, objects and arrays
- Object-oriented programming
- Deep dives into prototypes, JavaScript engines & how it works behind the scenes
- Manipulating web pages (= the DOM) with JavaScript
- Event handling, asynchronous coding and Http requests
- Meta-programming, performance optimization, memory leak busting
- Testing, security and deployment
- And so much more!
Your trainer

Maximilian Schwarzmüller
Starting out at the age of 13 I never stopped learning new programming skills and languages. Early I started creating websites for friends and just for fun as well. Besides web development I also explored Python and other non-web-only languages. This passion has since lasted and lead to my decision of working as a freelance web developer and consultant. The success and fun I have in this job is immense and really keeps that passion burningly alive.
Starting web development on the backend (PHP with Laravel, NodeJS, Python) I also became more and more of a frontend developer using modern frameworks like React, Angular or VueJS 2 in a lot of projects. I love both worlds nowadays!
617 lessons
Easy to follow lectures and videos covering subject details.
52 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction01:50
- What is JavaScript?03:46
- JavaScript in Action!09:08
- Join our Online Learning Community00:24
- How JavaScript Is Executed03:14
- Dynamic vs Weakly Typed Languages03:28
- JavaScript Executes In A Hosted Environment04:40
- Course Outline - What's In This Course?06:00
- How To Get The Most Out Of This Course02:36
- Using Course Resources01:01
- JavaScript vs Java04:02
- A Brief History Of JavaScript06:03
- Setting Up a Development Environment11:12
- Course FAQs02:20
- Module Introduction01:17
- Setting Up the Project04:25
- More on Version Control & Git00:35
- Adding JavaScript to the Website06:42
- Introducing Variables & Constants05:17
- Declaring & Defining Variables07:10
- Working with Variables & Operators06:17
- Variables & Operators6 questions
- Understanding the Starting Code01:21
- Data Types: Numbers & Strings (Text)06:01
- Using Constants05:11
- More on Strings15:51
- Data Types & Constants5 questions
- Time to Practice: Variables, Constants, Operators & Core Data Types1 question
- Introducing Functions05:50
- Adding A Custom Function11:22
- Code Styles, Conventions & Syntax01:53
- Returning Values04:31
- The (Un)Importance of Code Order04:34
- An Introduction to Global & Local Scope05:31
- "Shadowed Variables"00:48
- More about the "return" Statement02:24
- Executing Functions "Indirectly"11:10
- "Indirect" vs "Direct" Function Execution - Summary01:09
- Functions & Scope6 questions
- Time to Practice: Functions1 question
- Converting Data Types06:14
- Mixing Numbers & Strings00:32
- Splitting Code into Functions05:49
- Connecting all Buttons to Functions07:43
- Working with Code Comments04:09
- More Operators!06:39
- More Core Data Types!04:31
- Using Arrays08:53
- Creating Objects06:02
- Objects - Common Syntax Gotchas00:33
- Accessing Object Data02:51
- Arrays & Objects5 questions
- Adding a Re-Usable Function That Uses Objects05:24
- undefined, null & NaN06:20
- The "typeof" Operator03:12
- "undefined", "null" & "NaN"4 questions
- Importing Scripts Correctly with "defer" & "async"14:37
- Importing JavaScript - Summary00:06
- Wrap Up02:13
- Useful Resources & Links00:23
- Module Introduction01:28
- Efficient Development & Debugging - An Overview03:18
- Configuring the IDE Look & Feel02:25
- Using Shortcuts04:12
- Working with Auto-Completion & IDE Hints04:34
- Installing IDE Extensions02:04
- Tweaking Editor Settings02:15
- Utilizing Different IDE Views01:41
- Finding Help & Working with MDN05:53
- The ECMAScript Standard00:16
- How to "google" Correctly01:44
- Debugging JavaScript - An Overview03:17
- An Error Message! No Reason To Panic!04:46
- Using console.log() to look "into the Code"03:49
- Next-Level Debugging with the Chrome Devtools & Breakpoints08:20
- Testing Code Changes Directly in the Devtools02:05
- Debugging Code directly Inside VS Code04:55
- Wrap Up01:22
- Useful Resources & Links00:12
- Module Introduction02:26
- Introducing "if" Statements & Boolean (Comparison) Operators09:26
- Using Booleans in Conditions & More on Text Comparisons01:05
- Using "if" Statements07:23
- Working with "if", "else" and "else-if"05:10
- Beware When Comparing Objects & Arrays for Equality!04:06
- The Logical AND and OR Operators09:10
- Understanding Operator Precedence07:20
- if & Boolean Operators - The Basics7 questions
- Beyond true/ false: "Truthy" and "Falsy" Values07:30
- Coercion vs Conversion01:08
- Falsy and Truthy Values4 questions
- Setting Up a Bigger Example Project (The "Monster Killer")02:59
- Adding an "Attack" Function07:57
- Using "if" Statements for Checking the Win-Condition09:17
- Adding More "if" Statements & A "Strong Attack" Functionality07:41
- Time for a "Heal Player" Functionality!10:15
- Controlling the Conditional Bonus Life (Without Boolean Operators!)05:59
- Adding a "Reset Game" Functionality06:00
- Validating User Input06:17
- Utilizing Global Constants as Identifiers in Conditional Code03:20
- Adding a Conditional Battle Log16:37
- Introducing the Ternary Operator07:31
- A Bit of Theory: Statements vs Expressions01:40
- Logical Operator "Tricks" & Shorthands12:58
- Logical Operators - A Quick Summary01:03
- Logical Operators & How They Work8 questions
- Working with the "switch-case" Statement07:10
- Introducing Loops06:40
- The "for" Loop07:38
- The "for-of" Loop05:16
- The "for-in" Loop06:49
- The "while" & "do-while" Loops08:00
- Loops - Basics7 questions
- Time to Practice: Control Structures1 question
- Controlling Loops with "break"08:11
- Controlling Iterations with "continue"02:21
- More Control with Labeled Statements06:26
- break & continue6 questions
- Error Handling with "try-catch" - An Introduction02:25
- Throwing Custom Errors05:16
- Working with "try-catch" to Catch & Handle Errors08:14
- Error Handling3 questions
- Wrap Up03:21
- Useful Resources & Links00:10
- Module Introduction01:43
- ES5 vs ES6+ ("Next Gen JS") - Evolution of JavaScript08:14
- var vs let & const - Introducing "Block Scope"14:32
- Understanding "Hoisting"04:07
- Strict Mode & Writing Good Code05:46
- "JavaScript Specialties"3 questions
- How Code is Parsed & Compiled08:16
- Inside the JavaScript Engine - How the Code Executes15:59
- [DEEP DIVE] JavaScript Language vs Browser APIs01:42
- Primitive vs Reference Values19:24
- Garbage Collection & Memory Management12:00
- Wrap Up01:55
- Useful Resources & Links00:10
- Module Introduction01:31
- Recapping Functions Knowledge - What We Know Thus Far01:52
- Parameters vs Arguments00:26
- Functions vs Methods05:46
- Functions are Objects!02:47
- Function Expressions: Storing Functions in Variables05:12
- Function Expressions vs Function Declarations02:47
- Anonymous Functions05:54
- Working on the Project: Adding User Choices to the Game07:44
- Implementing the Core Game Logic07:20
- Introducing Arrow Functions08:41
- Different Arrow Function Syntaxes01:08
- Creating Functions3 questions
- Outputting Messages to the User03:53
- Default Arguments in Functions10:45
- Introducing Rest Parameters ("Rest Operator")08:57
- Creating Functions Inside of Functions03:04
- Understanding Callback Functions06:09
- Time to Practice: Functions1 question
- Working with "bind()"08:39
- Functions - Advanced3 questions
- Adding bind() to the Calculator Project03:47
- call() and apply()01:18
- Wrap Up02:10
- Useful Resources & Links00:09
- Module Introduction01:48
- What's the "DOM"?06:00
- Document and Window Object06:20
- Understanding the DOM and how it's created07:07
- Nodes & Elements - Querying the DOM Overview05:55
- Selecting Elements in the DOM09:54
- Summary: Node Query Methods01:00
- Exploring and Changing DOM Properties07:37
- Attributes vs Properties08:58
- Selecting Multiple Elements & Summary05:13
- DOM Basics5 questions
- Time to Practice: DOM Querying1 question
- Traversing the DOM - Overview06:22
- Traversing Child Nodes09:15
- Using parentNode & parentElement05:01
- Selecting Sibling Elements04:05
- DOM Traversal vs Query Methods04:35
- Styling DOM Elements12:18
- Creating Elements with JS - Overview02:42
- Adding Elements via HTML in Code07:42
- Adding Elements via createElement()05:42
- Inserting DOM Elements08:15
- Cloning DOM Nodes01:45
- Live Node Lists vs Static Node Lists04:55
- Removing Elements01:56
- Insertion & Removal Method Summary02:38
- Summary: Insert, Replace, Remove01:52
- Setting Up the Practice Project02:16
- Selecting the Modal and "Add" Button08:58
- Opening a Modal by Changing CSS Classes05:01
- Controlling the Backdrop08:04
- Fetching and Validating User Input08:06
- Creating a Movie in JavaScript & Clearing the Input04:00
- Rendering Movie Items on the Screen08:24
- Deleting Movie Elements09:12
- Showing & Hiding the "Are you sure?" Dialog07:08
- Starting with the Confirmation Logic04:29
- Finishing the App11:45
- Wrap Up01:55
- Useful Resources & Links00:12
- Module Introduction01:08
- What are "Iterables" and "Array-like Objects"?02:11
- Creating Arrays08:55
- Which Data Can You Store In Arrays?03:47
- push(), pop(), unshift(), shift() - Adding & Removing Elements06:59
- The splice() Method05:37
- Selecting Ranges & Creating Copies with slice()06:06
- Adding Arrays to Arrays with concat()02:23
- Retrieving Indexes with indexOf() /& lastIndexOf()03:47
- Finding Stuff: find() and findIndex()05:20
- Is it Included?01:20
- Alternative to for Loops: The forEach() Method04:24
- Transforming Data with map()02:38
- sort()ing and reverse()ing04:15
- Filtering Arrays with filter()02:35
- Where Arrow Functions Shine!01:31
- The Important reduce() Method07:33
- Chaining Methods in JavaScript00:48
- Arrays & Strings - split() and join()04:21
- The Spread Operator (...)10:31
- Understanding Array Destructuring04:24
- Maps & Sets - Overview04:16
- Working with Sets07:21
- Working with Maps09:30
- Maps vs Objects03:41
- Understanding WeakSet04:50
- Understanding WeakMap02:51
- Time to Practice: Arrays & Iterables1 question
- Wrap Up01:25
- Useful Resources & Links00:12
- Module Introduction01:38
- What's an Object?05:54
- Objects & Primitive Values00:41
- Objects - Recap02:42
- Adding, Modifying & Deleting Properties06:46
- Special Key Names & Square Bracket Property Access08:36
- Property Types & Property Order03:55
- Dynamic Property Access & Setting Properties Dynamically04:11
- Object Properties4 questions
- Demo App & Shorthand Property Syntax09:22
- Rendering Elements based on Objects05:36
- for-in Loops & Outputting Dynamic Properties05:24
- Adding the Filter Functionality05:38
- Understanding "Chaining" (Property & Method Chaining)01:51
- The Object Spread Operator (...)05:54
- Understanding Object.assign()02:08
- Object Destructuring06:13
- Checking for Property Existance02:42
- Introducing "this"05:52
- The Method Shorthand Syntax01:07
- The "this" Keyword And Its Strange Behavior05:41
- call() and apply()03:22
- What the Browser (Sometimes) Does to "this"02:33
- "this" and Arrow Functions10:36
- "this" - Summary01:15
- "this"6 questions
- Getters & Setters07:05
- Wrap Up01:33
- Useful Resources & Links00:10
- Module Introduction01:55
- What is "Object-oriented Programming" (OOP)?03:17
- Getting Started with OOP Code12:10
- Defining & Using a First Class07:17
- Working with Constructor Methods04:51
- Fields vs Properties02:19
- Using & "Connecting" Multiple Classes09:06
- Binding Class Methods & Working with "this"04:57
- Adding a Cart and Shop Class04:37
- Communicating Can Be Challenging!03:54
- Static Methods & Properties07:51
- First Summary & Classes vs Object Literals04:06
- Getters & Setters05:43
- Introducing Inheritance02:34
- Implementing Inheritance11:50
- Using Inheritance Everywhere06:51
- Overriding Methods and the super() Constructor06:00
- super() Constructor Execution, Order & "this"06:46
- Different Ways of Adding Methods05:51
- Private Properties07:24
- "Pseudo-Private" Properties00:32
- Time to Practice: Classes & OOP1 question
- The "instanceof" Operator04:30
- Built-in Classes01:09
- Understanding Object Descriptors07:35
- Classes5 questions
- Wrap Up01:51
- Useful Resources & Links00:09
- Module Introduction01:34
- Introducing Constructor Functions04:02
- Constructor Functions vs Classes & Understanding "new"04:17
- Introducing Prototypes16:46
- Prototypes - Summary00:59
- Working with Prototypes05:19
- The Prototype Chain and the Global "Object"08:26
- Constructor Functions & Prototypes4 questions
- Classes & Prototypes05:24
- Methods in Classes & In Constructors10:16
- Built-in Prototypes in JavaScript02:12
- Setting & Getting Prototypes10:58
- Wrap Up02:49
- Useful Resources & Links00:09
- Module Introduction01:38
- First Project Steps & Planning04:46
- Creating Project Lists & Parsing Element Data04:08
- Starting with the "Switch Project" Logic09:59
- Passing Method References Around07:02
- Moving DOM Elements11:49
- Adding a Tooltip08:58
- Adding Inheritance06:13
- Wrap Up00:57
- Useful Resources & Links00:07
- Module Introduction02:31
- Using "dataset" (data-* Attributes)06:51
- Getting Element Box Dimensions05:54
- Working with Element Sizes & Positions04:56
- The DOM & Prototypes02:21
- Positioning the Tooltip10:57
- Handling Scrolling05:36
- Working with <template> Tags05:14
- Loading Scripts Dynamically07:35
- Setting Timers & Intervals07:37
- The "location" and "history" Objects04:20
- The "navigator" Object04:50
- Working with Dates03:17
- The "Error" Object & Constructor Function03:21
- Wrap Up00:43
- Useful Resources & Links00:12
- Module Introduction01:31
- Introduction to Events in JavaScript06:18
- Different Ways of Listening to Events06:59
- Removing Event Listeners05:14
- The "event" Object05:43
- Supported Event Types08:00
- Example: Basic Infinite Scrolling01:02
- Working with "preventDefault()"05:15
- Understanding "Capturing" & "Bubbling" Phases02:03
- Event Propagation & "stopPropagation()"07:39
- Using Event Delegation08:33
- Triggering DOM Elements Programmatically03:40
- Event Handler Functions & "this"02:28
- Events4 questions
- Drag & Drop - Theory04:59
- Configuring Draggable Elements06:23
- Marking the "Drop Area"08:52
- Dropping & Moving Data + Elements06:58
- Firefox Adjustments01:40
- Wrap Up01:15
- Useful Resources & Links00:11
- Module Introduction00:57
- Pure Functions & Side-Effects06:13
- Impure vs Pure Functions02:00
- Factory Functions05:41
- Closures07:45
- Closures in Practice07:01
- Closures & Memory Management01:23
- Optional: IIFEs00:57
- Introducing "Recursion"07:32
- Advanced Recursion09:01
- Advanced Functions4 questions
- Wrap Up01:14
- Useful Resources & Links00:10
- Module Introduction00:53
- How Numbers Work & Behave in JavaScript07:46
- Floating Point (Im)Precision11:04
- The BigInt Type03:36
- The Global "Number" and "Math" Objects02:47
- Example: Generate Random Number Between Min/ Max05:33
- Exploring String Methods01:43
- Tagged Templates10:28
- Introducing Regular Expressions ("RegEx")04:30
- More on Regular Expressions07:25
- Wrap Up01:20
- Useful Resources & Links00:10
- Module Introduction01:12
- Understanding Synchronous Code Execution ("Sync Code")02:51
- Understanding Asynchronous Code Execution ("Async Code")05:44
- Blocking Code & The "Event Loop"10:30
- Sync + Async Code - The Execution Order04:03
- Multiple Callbacks & setTimeout(0)03:20
- Asynchronous Code3 questions
- Getting Started with Promises08:25
- Chaining Multiple Promises05:53
- Promise Error Handling07:46
- Promise States & "finally"00:41
- Async/ await09:11
- Async/ await & Error Handling03:07
- Async/ await vs "Raw Promises"04:56
- Promise.all(), Promise.race() etc.07:59
- Promises & async/ await5 questions
- Wrap Up01:27
- Useful Resources & Links00:10
- Module Introduction01:07
- What & Why05:03
- How The Web Works00:13
- More Background about Http05:24
- Getting Started with Http03:35
- Sending a GET Request03:46
- JSON Data & Parsing Data09:14
- JSON Data Deep Dive01:16
- Promisifying Http Requests (with XMLHttpRequest)03:49
- Sending Data with a POST Request04:55
- Triggering Requests via the UI03:13
- The "Fetch" Button Always Appends00:11
- Sending a DELETE Request04:56
- Handling Errors05:03
- Using the fetch() API07:10
- POSTing Data with the fetch() API02:38
- Adding Request Headers03:01
- fetch() & Error Handling07:11
- XMLHttpRequest vs fetch()01:42
- Working with FormData06:58
- Wrap Up01:17
- Useful Resources & Links00:11
- Module Introduction00:59
- What & Why02:55
- Adding Libraries (Example: lodash)09:10
- Example: jQuery02:30
- Discovering Libraries03:25
- Axios Library & Http Requests10:46
- Third-Party Library Considerations04:54
- Wrap Up00:59
- Useful Resources & Links00:07
- Module Introduction00:56
- Splitting Code in a Sub-optimal Way07:12
- A First Step Towards JavaScript Modules03:25
- We Need a Development Server!05:58
- First import / export Work03:41
- Switching All Files To Use Modules04:26
- More Named Export Syntax Variations06:12
- Working With Default Exports03:35
- Dynamic Imports & Code Splitting05:24
- When Does Module Code Execute?02:06
- Module Scope & globalThis06:18
- Modules4 questions
- Wrap Up01:37
- Useful Resources & Links00:09
- Module Introduction03:23
- Project Limitations & Why We Need Tools08:11
- Workflow Overview02:42
- A Note About The NodeJS Version00:19
- Setting Up a npm Project03:45
- Working with npm Packages03:39
- Linting with ESLint08:38
- Configuring ESLint00:27
- Important: Webpack Version00:06
- Bundling with Webpack15:13
- Bonus: Multiple Entry Points00:47
- Development Mode & Fixing "Lazy Loading"04:00
- Using webpack-dev-server03:14
- Generating Sourcemaps03:05
- Building For Production03:45
- Final Optimizations06:35
- Using Third Party Packages with npm & Webpack04:06
- Wrap Up01:39
- Useful Resources & Links00:10
- Module Introduction01:05
- Browser Storage Options07:21
- localStorage & sessionStorage10:43
- Getting Started with Cookies06:06
- Working with Cookies08:10
- Getting Started with IndexedDB08:55
- Working with IndexedDB04:06
- Wrap Up00:48
- Useful Resources & Links00:11
- Module Introduction01:29
- What Is "Browser Support" About?07:36
- Determining Browser Support For A JavaScript Feature08:20
- Determining Required Support03:29
- Solution: Feature Detection + Fallback Code09:55
- Solution: Using Polyfills03:18
- Solution: Transpiling Code12:02
- Improvement: Automatically Detect + Add Polyfills11:02
- What about Support Outside of Browsers?00:58
- Browser Support Outside of JavaScript Files02:36
- Wrap Up01:18
- Useful Resources & Links00:10
- Module Introduction00:53
- Note: Use the LTS Version of NodeJS00:07
- Setting Up the Project03:05
- Getting DOM Access04:33
- Getting the User Location06:27
- Adding Feedback (Showing a Modal)11:16
- Hiding the Modal02:38
- Rendering a Map with Google Maps14:16
- Continuing without a Credit Card00:50
- Finding an Address & Getting the Coordinates08:22
- Converting User Input to Coordinates03:35
- Creating a "Share Place" Link08:30
- Copying the Link to the Clipboard03:36
- Rendering the "Shared Place" Screen06:22
- Useful Resources & Links00:07
- Module Introduction01:06
- What and Why?07:35
- The Idea Behind React.js02:46
- Analysing a React Project17:38
- Wrap Up01:35
- Useful Resources & Links00:10
- Module Introduction01:51
- Understanding Symbols08:27
- Well-known Symbols05:15
- Understanding Iterators05:32
- Generators & Iterable Objects11:02
- Generators Summary & Built-in Iterables Examples03:22
- The Reflect API07:10
- The Proxy API and a First "Trap"09:04
- Working with Proxy Traps02:57
- Wrap Up01:37
- Useful Resources & Links00:16
- Module Introduction01:42
- JavaScript is a Hosted Language02:21
- Installation & Basics06:16
- Understanding Modules & File Access04:39
- Working with Incoming Http Requests05:46
- Sending Responses (HTML Data)04:01
- Parsing Incoming Data10:53
- Introducing & Installing Express.js02:48
- Express.js: The Basics06:34
- Extracting Data04:23
- Rendering Server-side HTML with Templates & EJS06:52
- Enhancing Our Project03:44
- Adding Basic REST Routes12:09
- Understanding CORS (Cross Origin Resource Sharing)05:13
- Sending the Location ID to the Frontend02:16
- Adding the GET Location Route07:16
- Introducing MongoDB (Database)14:44
- NodeJS Error Handling00:52
- Wrap Up01:50
- Useful Resources & Links00:10
- Module Introduction01:35
- Security Hole Overview & Exposing Data in your Code06:45
- Cross-Site Scripting Attacks (XSS)14:39
- Third-Party Libraries & XSS05:17
- XSS & Exposing Confidential Details3 questions
- CSRF Attacks (Cross Site Request Forgery)04:16
- CORS (Cross Origin Resource Sharing)02:53
- Wrap Up01:30
- Useful Resources & Links00:09
- Module Introduction01:11
- Deployment Steps07:29
- Different Types of Websites00:32
- Example: Static Host Deployment (no Server-side Code)09:43
- Injecting Script Imports Into HTML Automatically00:18
- Example: Dynamic Page Deployment (with Server-side Code)12:45
- Useful Resources & Links00:10
- Module Introduction01:49
- What is "Performance Optimization" About?06:16
- Optimization Potentials06:48
- Measuring Performance03:42
- Diving Into The Browser Devtools (for Performance Measuring)15:30
- Further Resources02:13
- Preparing The Testing Setup04:03
- Optimizing Startup Time & Code Usage / Coverage11:51
- Updating The DOM Correctly09:53
- Updating Lists Correctly08:11
- Optimizing The Small Things03:58
- Micro-Optimizations (Think Twice!)08:49
- Finding & Fixing Memory Leaks09:43
- Server-side Performance Optimizations01:22
- Wrap Up03:26
- Useful Resources & Links00:11
- Module Introduction00:49
- What Is Testing? Why Does It Matter?07:09
- Testing Setup04:10
- Writing & Running Unit Tests11:15
- Writing & Running Integration Tests06:03
- Writing & Running e2e Tests10:16
- Dealing with Async Code10:20
- Working with Mocks07:51
- Useful Resources & Links00:11
- Module Introduction00:48
- What are Programming Paradigms?03:01
- Procedural Programming in Practice08:53
- Object Oriented Programming in Practice13:20
- Functional Programming in Practice13:52
- Wrap Up03:26
- Useful Resources & Links00:07
- Module Introduction02:02
- What are "Data Structures" & "Algorithms"?04:22
- A First Example10:22
- Solving the Same Problem Differently07:26
- Performance & The "Big O" Notation11:44
- More Time Complexities & Comparing Algorithms03:36
- More on Big O05:23
- More Examples10:15
- Diving into Data Structures & Time Complexities12:07
- Where to Learn More & Wrap Up03:22
- Useful Resources & Links00:13
- Module Introduction01:11
- What is TypeScript and Why would you use it?04:58
- Working with Types10:05
- Core Types & Diving Deeper17:26
- Object Types, Array Types & Function Types09:22
- Advanced Types (Literal Types, Union Types, Enums)09:20
- Classes & Interfaces12:02
- Generic Types05:02
- Configuring the TypeScript Compiler05:11
- Useful Resources & Links00:09
- Module Introduction02:01
- Web Components in Action06:30
- What are Web Components?03:22
- Why Web Components?02:44
- Getting Started!04:56
- Web Component Browser Support02:44
- Our Development Setup03:53
- A First Custom Element08:17
- Interacting with the Surrounding DOM05:04
- Understanding the Custom Element Lifecycle02:51
- Using "connectedCallback" for DOM Access02:05
- Listening to Events Inside the Component09:12
- Using Attributes on Custom Elements04:45
- Styling our Elements04:08
- Working with the "Shadow DOM"05:00
- Adding an HTML Template05:40
- Using Slots01:50
- Defining the Template in JavaScript03:21
- Using Style Tags in the Shadow DOM02:40
- Extending Built-in Elements07:33
- Time to Practice - The Basics1 question
- The Next Steps00:49
- Understanding Shadow DOM Projection02:26
- Styling "slot" Content Outside of the Shadow DOM03:44
- Styling "slot" Content Inside of the Shadow DOM03:10
- Styling the Host Component04:42
- Conditional Host Styling02:49
- Styling with the Host Content in Mind02:27
- Smart Dynamic Styling with CSS Variables06:20
- Cleaning Up the Overall Styling03:22
- Observing Attribute Changes06:17
- Adjusting the Component Behavior Upon Attribute Changes02:34
- Using "disconnectedCallback"06:10
- Adding a render() Method06:13
- Final Adjustment00:12
- The Next Steps00:52
- Creating the Basic Modal Component06:33
- Adding the Modal Container02:29
- Styling the Modal Elements05:35
- Adding Some General App Logic03:49
- Opening the Modal via CSS05:23
- Public Methods & Properties06:37
- Understanding Named Slots05:45
- Listening to Slot Content Changes05:10
- Closing the Modal06:57
- Dispatching Custom Events03:40
- Configuring Custom Events04:52
- Finishing it up!04:51
- Useful Resources & Links00:07
- Course Roundup00:55
- Bonus! (Next Steps Overview / Other Topics)00:45
Online Courses
Learning JavaScript doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of JavaScript related books and take your
YouTube videos
The number of high-quality and free JavaScript video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more