Mastering Multithreading Programming with Go (Golang)
Learn about Multithreading, Concurrency & Parallel programming with practical and fun examples in Google's Go Lang
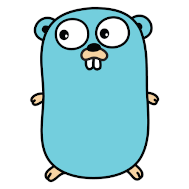
What you will learn?
- Discover how to create responsive and high performance software.
- See how to use multithreading for modeling certain types of problems and simulations.
- Develop programs with Golang that are highly Concurrent and Parallel.
- Understand the advantages, limits and properties of Parallel computing.
- Improve your programming skills in Go with more advanced, mulithreading topics.
- Learn about goroutines, mutexes, reader writers locks, waitgroups, channels, condition variables and more.
Your trainer

James Cutajar
James Cutajar is a software developer with an interest in scalable, high-performance computing, and distributed algorithms. He has worked in the technology field for over 15 years in various industries. He is also an author of various video courses on Scala and a book on data structures and algorithms. Through his career, he has been an instructor, open source contributor, blogger, and a tech evangelist. When he is not writing software, he is riding his motorbike, surfing, scuba diving, or flying light aircraft. He was born in Malta, lived in London for almost a decade, and is now working in Portugal.
47 lessons
Easy to follow lectures and videos covering subject details.
5.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Understanding Parallel Computing04:23
- More on Parallel Computing05:50
- Links and Resources for this course00:22
- Processes, Threads and Green threads12:27
- Using Goroutines for Boids04:18
- Groundwork for our simulation05:13
- Starting our Goroutines08:53
- Memory sharing between Threads06:46
- Memory Sharing example Part 105:37
- Memory Sharing example Part 206:40
- Using Threads Quiz4 questions
- Why do we need Locking?08:59
- Using Mutexes in Boid simulation05:46
- Adding Synchronization to simulation05:26
- Readers-Writer Locks07:12
- Boid Synchronization with Readers-Writers Locks07:13
- Mutexes Quiz4 questions
- Understanding WaitGroups06:42
- Concurrent file searching with WaitGroups07:49
- WaitGroup Quiz3 questions
- Thread Communication using Channels10:07
- Pipelining Example12:31
- Understanding Thread Pools07:59
- Thread Pool Example Part 108:17
- Thread Pool Example Part 206:06
- Shoelace algorithm explained (optional)00:05
- Channels Quiz3 questions
- What's a Condition Variable?07:00
- Parallel Algorithm for Matrix Multiplication09:27
- Implementing Parallel Matrix Multiplication Part 107:05
- Implementing Parallel Matrix Multiplication Part 206:06
- Condition Variable Quiz3 questions
- Deadlocking Robots, Philosophers and Trains08:29
- Simple Deadlock Example04:17
- Train Deadlock Example Part 103:40
- Train Deadlock Example Part 208:29
- Solving Deadlocks using Resource Hierarchy07:08
- Implementing Resource Hierarchy Solution06:54
- Solving Deadlocks using an Arbitrator08:10
- Implementing Arbitrator Solution05:36
- Barriers Explained07:45
- Building a Barrier08:02
- Barriers with Matrix Multiplication07:45
- Understanding Atomic Variables08:57
- Atomic Variables Example02:59
- Building a Letter Frequency Program07:24
- Using Atomic Variables in our Example07:03
- Locking using Spinning locks06:15
- Building our own Spinning locks04:54
- Ledger Example09:50
- Implementing the Ledger Part 106:42
- Implementing the Ledger Part 209:47
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more