Concurrency in Go (Golang)
Learn the Why's and How's of concurrency in Go.
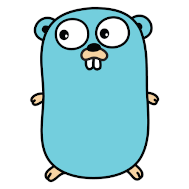
What you will learn?
- How to spin Goroutines and communicate data between them.
- How to avoid Race conditions and Deadlocks.
- How to synchronize the execution of Goroutines.
- How Go Scheduler works.
- How to build streaming pipelines with Goroutines and Channels.
- How to propagate data across API boundary.
Your trainer

Deepak kumar Gunjetti
At Andcloud, we have cloud practice focused on Google Cloud Platform, to help customers in DevOps, Data Analytics and Security.
As a Trainer I try to teach to students to create positive impact in their careers.
I have more than 15 years of experience in software development. Previously I have worked at Cisco Systems as Software Engineer. I have worked on Cisco's Routing and Switching Platforms for Enterprise and Data Center networks.
Previous to that I have worked at LG Soft as Senior Systems Analyst. I have developed Embedded Software for consumer devices like car audio systems, media devices and camera systems.
77 lessons
Easy to follow lectures and videos covering subject details.
5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction06:05
- Course Overview05:06
- Processes and Threads07:51
- Why Concurrency is hard06:27
- Goroutines04:37
- Clone Coding Exercise Github Repository00:01
- Exercise-Hello04:25
- Exercise-ClientServer04:44
- WaitGroups05:28
- Exercise-WaitGroup01:48
- Goroutines & Closures00:48
- Exercise-Closure01:37
- Exercise-Closure0202:02
- Deep Dive - Go Scheduler06:53
- Deep Dive - Go Scheduler - Context Switching due to synchronous system call03:16
- Deep Dive - Go Scheduler - Context Switching due to Asynchronous system call05:40
- Deep Dive - Go Scheduler - Work Stealing03:14
- Channels03:39
- Exercise - Channels01:22
- Range, Buffered Channels02:06
- Exercise - Range01:41
- Exercise - Buffered Channel01:24
- Channel Direction01:05
- Exercise - Channel Direction03:35
- Channel Ownership01:43
- Exercise - Channel Ownership02:18
- Deep Dive - Channels03:01
- Deep Dive - Channels - Send and Recieve02:30
- Deep Dive - Channels - Buffer full03:00
- Deep Dive - Channels - Buffer empty03:01
- Deep Dive - Channels - Unbuffer channel02:01
- Deep Dive - Channels - Summary01:11
- Select04:14
- Exercise - Select02:28
- Exercise - Timeout01:43
- Exercise - Non-blocking communication01:58
- Mutex02:54
- Exercise - Mutex03:13
- Atomic00:46
- Exercise - Atomic02:22
- Conditional Variable05:51
- Exercise - Conditional Variable - Signal02:06
- Exercise - Conditional Variable - Broadcast02:55
- Sync Once00:46
- Exercise - Sync Once03:17
- Sync Pool01:14
- Exercise - Sync Pool03:54
- Race Detector01:30
- Exercise - Race05:12
- Web Crawler - Sequential02:30
- Web Crawler - Concurrent07:30
- Pipelines04:29
- Exercise - Pipelines05:41
- Fan-out & Fan-in03:09
- Exercise - Fan-out & Fan-in05:05
- Cancelling Goroutines03:10
- Exercise - Cancelling Goroutines04:51
- Image Processing Sequential05:31
- Image Processing Pipeline16:10
- Context Package05:14
- Context Package for Cancellation08:15
- Context Package as Data bag01:57
- Exercise - WithCancel04:21
- Exercise - WithDeadline04:52
- Exercise - WithTimeout02:36
- Exercise - WithValue04:28
- Context Package - Go Idioms04:44
- HTTP Server Timeouts with Context Package12:41
- Interface12:20
- Interface-implicit02:31
- Interfaces from standard library05:24
- Exercise io.Writer interface02:20
- Exercise Stringer interface02:10
- Interface Satisfaction04:15
- Type Assertion04:57
- Exercise Type Assertion05:47
- Empty Interface03:21
Online Courses
Learning Go doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Go related books and take your
YouTube videos
The number of high-quality and free Go video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more