Design Patterns in C# and .NET
Discover the modern implementation of design patterns with C# and .NET
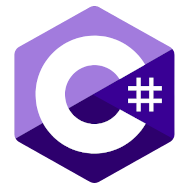
What you will learn?
- Recognize and apply design patterns
- Refactor existing designs to use design patterns
- Reason about applicability and usability of design patterns
Your trainer

Dmitri Nesteruk
Dmitri is a quant, developer, book author and course author. His interests lie in software development and integration practices in the areas of computation, quantitative finance and algorithmic trading. His technological interests include C# and C++ programming as well high-performance computing using technologies such as CUDA and FPGAs. He has been a C# MVP since 2009.
173 lessons
Easy to follow lectures and videos covering subject details.
20.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction07:12
- Overview01:16
- Single Responsibility Principle07:29
- Open-Closed Principle17:24
- Liskov Substitution Principle06:37
- Interface Segregation Principle06:33
- Dependency Inversion Principle11:11
- Summary05:25
- Gamma Categorization03:36
- Overview01:43
- Life Without Builder03:34
- Builder09:06
- Fluent Builder01:16
- Fluent Builder Inheritance with Recursive Generics12:37
- Stepwise Builder09:00
- Functional Builder10:19
- Faceted Builder11:11
- Builder Coding Exercise1 question
- Summary00:57
- Overview02:22
- Point Example04:38
- Factory Method05:03
- Asynchronous Factory Method05:01
- Factory02:59
- Object Tracking and Bulk Replacement12:01
- Inner Factory05:41
- Abstract Factory11:21
- Abstract Factory and OCP09:52
- Factory Coding Exercise1 question
- Summary01:05
- Overview01:59
- ICloneable is Bad07:37
- Copy Constructors03:55
- Explicit Deep Copy Interface02:34
- Prototype Inheritance20:24
- Copy Through Serialization09:05
- Prototype Coding Exercise1 question
- Summary01:08
- Overview02:43
- Singleton Implementation08:40
- Testability Issues07:22
- Singleton in Dependency Injection08:58
- Monostate03:46
- Per-Thread Singleton04:25
- Ambient Context12:26
- Singleton Coding Exercise1 question
- Summary02:15
- Overview02:36
- Vector/Raster Demo08:35
- Adapter Caching06:04
- Generic Value Adapter25:18
- Adapter in Dependency Injection09:07
- Adapter Coding Exercise1 question
- Summary01:10
- Overview02:50
- Bridge09:49
- Bridge Coding Exercise1 question
- Summary01:33
- Overview01:53
- Geometric Shapes07:33
- Neural Networks08:00
- Composite Specification05:58
- Composite Coding Exercise1 question
- Summary01:11
- Overview02:34
- Custom String Builder06:19
- Adapter-Decorator06:33
- Multiple Inheritance with Interfaces08:40
- Multiple Inheritance with Default Interface Members07:44
- Dynamic Decorator Composition07:39
- Detecting Decorator Cycles22:02
- Static Decorator Composition09:30
- Decorator in Dependency Injection06:10
- Decorator Coding Exercise1 question
- Summary02:02
- Overview03:08
- Façade07:59
- Facade Coding Exercise1 question
- Summary01:25
- Overview05:24
- Repeating User Names12:29
- Text Formatting08:53
- Flyweight Coding Exercise1 question
- Summary00:58
- Overview03:12
- Protection Proxy03:11
- Property Proxy09:24
- Value Proxy12:04
- Composite Proxy: SoA/AoS11:30
- Composite Proxy with Array-Backed Properties06:42
- Dynamic Proxy for Logging11:50
- Proxy vs. Decorator01:27
- ViewModel08:42
- Proxy Coding Exercise1 question
- Bit Fragging25:04
- Summary00:55
- Overview03:33
- Command Query Separation01:28
- Method Chain12:15
- Broker Chain13:50
- Chain of Responsibility Coding Exercise1 question
- Summary01:18
- Overview03:06
- Command07:47
- Undo Operations06:05
- Composite Command12:11
- Command Coding Exercise1 question
- Summary01:09
- Overview04:00
- Handmade Interpreter: Lexing07:52
- Handmade Interpreter: Parsing12:05
- ANTLR02:44
- Interpreter Coding Exercise1 question
- Summary01:03
- Overview01:42
- Iterator Object11:49
- Iterator Method06:57
- Iterators and Duck Typing04:06
- Array-Backed Properties05:51
- Iterator Coding Exercise1 question
- Summary01:28
- Overview01:13
- Chat Room10:42
- Event Broker15:30
- Introduction to MediatR13:20
- Mediator Coding Exercise1 question
- Summary01:16
- Overview01:41
- Memento06:04
- Undo and Redo07:15
- Memento for Interop06:38
- Memento Coding Exercise1 question
- Summary01:17
- Overview01:57
- Null Object08:53
- Null Object Singleton05:08
- Dynamic Null Object06:11
- Null Object Coding Exercise1 question
- Summary00:41
- Overview02:10
- Observer via the 'event' Keyword07:09
- Weak Event Pattern08:25
- Observer via Special Interfaces18:28
- Observable Collections09:45
- Bidirectional Observer14:48
- Property Dependencies13:30
- Declarative Event Subscriptions with Interfaces27:08
- Observer Coding Exercise1 question
- Summary00:56
- Overview03:08
- Classic Implementation12:32
- Handmade State Machine06:44
- Switch-Based State Machine06:38
- Switch Expressions08:49
- State Machine with Stateless05:37
- State Coding Exercise1 question
- Summary01:00
- Overview01:50
- Dynamic Strategy08:25
- Static Strategy04:07
- Equality and Comparison Strategies07:41
- Strategy Coding Exercise1 question
- Summary00:26
- Overview01:29
- Template Method07:22
- Functional Template Method03:38
- Template Method Coding Exercise1 question
- Summary00:45
- Overview04:46
- Intrusive Visitor04:41
- Reflective Visitor09:10
- Classic Visitor (Double Dispatch)10:21
- Reductions and Transforms14:24
- Dynamic Visitor via the DLR07:11
- Acyclic Visitor12:01
- Visitor Coding Exercise1 question
- Summary01:09
- Creational Paterns Summary04:41
- Structural Patterns Summary06:13
- Behavioral Patterns Summary08:48
- End of Course00:54
- Duck Typing Mixins09:53
- An ASCII C# String13:58
- Continuation Passing Style11:06
- Local Inversion of Control17:42
- DI Container and Event Broker Integration10:11
- Beyond the Elvis Operator14:46
- CQRS and Event Sourcing26:42
- Overview05:10
- Builder04:57
- Decorator04:39
- Factory08:15
- Interpreter09:58
- Strategy05:38
- Template Method10:06
- Summary02:55
Online Courses
Learning C# doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C# related books and take your
YouTube videos
The number of high-quality and free C# video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more