Complete Kotlin development masterclass 2022
Master the fundamentals and advanced features of Kotlin development
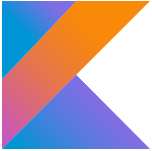
What you will learn?
- Kotlin development
- Kotlin coroutines
- Functional programming
- Object Oriented Programming
- Kotlin language fundamentals
- Kotlin extensions
- Generics
- Principles of software development
Your trainer

Catalin Stefan
I am an avid learner and teaching enthusiast. I love creating content and courses that enrich people's lives and help them have a better experience, both personally and professionally.
I am an expert mobile application developer with over 10 years development experience. I enjoy making courses related to software development and mobile apps, and want to share the knowledge I have acquired.
I create courses based on my professional experience. I hope to teach people skills that help them in their careers, allow them to acquire new skills and improve their personal relationships.
456 lessons
Easy to follow lectures and videos covering subject details.
30.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Course introduction04:43
- How this course is structured03:15
- Important message about Udemy reviews01:08
- How to take this course05:36
- How to ask questions06:57
- Section objectives02:41
- Installation03:21
- Hello world06:43
- Run the code04:28
- Change the code01:33
- Explanation08:11
- Exercise: Build your own project00:16
- Solution: Build your own project01:42
- Quiz: First code3 questions
- Comments07:49
- Quiz: Comments2 questions
- BONUS: REPL03:49
- Section recap03:22
- Section objectives03:15
- Strings05:22
- Chars01:17
- Variables09:11
- Variable names04:30
- Practice: Variables and Strings03:18
- Exercise: Variables and Strings00:03
- Solution: Variables and Strings02:21
- String functions07:48
- Bonus: How to find all available string functions01:31
- Exercise: Discover a new function and use it00:05
- String templates04:01
- Practice: Strings05:40
- Exercise: Strings00:07
- Solution: Strings01:48
- Constants02:21
- Section recap03:31
- Section objectives01:15
- Getting user input03:28
- Input a number06:39
- Generating a random number04:59
- Practice: Getting user input02:40
- Exercise: Getting user input00:04
- Solution: Getting user input02:22
- Section recap01:28
- Section objectives02:22
- What are numbers in Kotlin02:31
- Types of numbers10:07
- Practice: Numbers03:29
- Exercise: Numbers00:09
- Solution: Numbers03:26
- Bonus: How are numbers stored in memory07:38
- Implicit and explicit values09:14
- Type conversions07:28
- Let's talk about Strings again05:23
- Practice: Type conversions10:02
- Exercise: Type conversions00:05
- Solution: Type conversions02:17
- Section recap02:04
- Section objectives02:34
- Arithmetic operators09:36
- Result types06:00
- Assignment operators04:40
- Augmented assignment operators04:51
- Practice: Operators08:16
- Exercise: Operators00:08
- Solution: Operators03:26
- Booleans01:45
- Logical operators05:53
- Comparison operators07:22
- Practice: Logical operators08:48
- Exercise: Logical operators00:14
- Solution: Logical operators03:58
- Section recap03:11
- Section objectives03:17
- Null value09:13
- Null safe calls07:48
- Arithmetic operators on null values04:24
- Practice: Null05:42
- Exercise: Null00:07
- Solution: Null03:07
- The Elvis operator05:48
- Non-null Assertions04:27
- Practice: Elvis and assertions06:00
- Exercise: Elvis and assertions00:07
- Solution: Elvis and assertions02:58
- Section recap03:11
- Section objectives02:40
- What are exceptions06:09
- Try catch08:20
- Finally05:16
- Throw04:56
- Practice: Try catch finally05:32
- Exercise: Try catch finally00:09
- Solution: Try catch finally04:35
- Section recap02:05
- Section objectives02:38
- Why are collections useful04:21
- What are collections08:00
- List03:25
- Set02:07
- Map02:02
- Iterators02:10
- Section recap02:16
- Section objectives01:57
- What is a List10:25
- ArrayList09:12
- Practice: List and ArrayList04:44
- Exercise: List and ArrayList00:10
- Solution: List and ArrayList03:48
- List functions05:38
- ArrayList functions06:14
- Practice: List functions07:04
- Exercise: List functions00:11
- Solution: List functions03:18
- Section recap01:52
- Section objectives02:23
- What is a Set05:39
- HashSet07:23
- Practice: Set and HashSet05:00
- Exercise: Set and HashSet00:06
- Solution: Set and HashSet03:57
- Set functions05:31
- HashSet functions05:55
- Practice: Set functions05:14
- Exercise: Set functions00:13
- Solution: Set functions04:55
- Section recap02:06
- Section objectives02:16
- What is a Map07:54
- HashMap06:32
- Map functions06:07
- Practice: Map and HashMap06:20
- Exercise: Map and HashMap00:11
- Solution: Map and HashMap05:54
- Section recap02:19
- Section objectives03:36
- What is flow control03:52
- If conditional02:07
- When conditional03:55
- For loop03:04
- While loop02:34
- Section recap02:53
- Section objectives02:21
- If statement09:37
- More if statements05:41
- Practice: If statement08:29
- Exercise: If statement00:12
- Solution: If statement03:45
- Shorthand if statement06:15
- Multiple branches07:25
- Practice: If conditional07:08
- Exercise: If conditional00:16
- Solution: If conditional04:45
- Section recap02:22
- Section objectives02:43
- Arithmetic and logical expressions08:20
- in keyword08:53
- Boolean returning functions07:16
- Practice: Expressions07:40
- Exercise: Expressions00:11
- Solution: Expressions03:43
- Section recap01:50
- Section objectives01:43
- When conditional07:22
- When as a statement06:11
- Capturing the subject04:34
- Practice: When conditional06:47
- Exercise: When conditional00:09
- Solution: When conditional02:42
- Section recap01:48
- Section objectives01:58
- For loop07:34
- Practice: For loops06:36
- Exercise: For loops00:12
- Solution: For loops04:06
- For loop with ranges06:02
- Nested for loops07:18
- Practice: Extended for loops05:02
- Exercise: Extended for loops00:10
- Solution: Extended for loops03:08
- Section recap02:06
- Section objectives01:10
- While loop05:51
- Practice: While loop08:59
- Exercise: While loop00:06
- Solution: While loop02:33
- Do while loop02:24
- Nested while loops03:47
- Practice: While loop09:12
- Exercise: Do while loop00:08
- Solution: Do while loop02:45
- Section recap02:32
- Section objectives01:06
- The break keyword03:08
- The continue keyword02:49
- Labels03:48
- Practice: Break and continue04:18
- Exercise: Break and continue00:12
- Solution: Break and continue03:06
- Section recap01:32
- Section objectives02:00
- What are functions07:13
- Practice: Functions03:25
- Exercise: Functions00:08
- Solution: Functions03:07
- Function parameters10:17
- Function return11:10
- Practice: More functions06:58
- Exercise: More functions00:08
- Solution: More functions03:01
- Section recap02:44
- Section objectives01:48
- Overloading08:04
- Practice: Overloading06:32
- Exercise: Overloading00:12
- Solution: Overloading04:59
- Scope03:55
- Practice: Scope02:48
- Exercise: Scope00:07
- Solution: Scope03:28
- Vararg02:41
- Practice: Vararg02:28
- Exercise: Vararg00:08
- Solution: Vararg02:46
- Local functions05:44
- Practice: Local functions05:01
- Exercise: Local functions00:08
- Solution: Local functions02:45
- Section recap03:02
- Section objectives01:41
- Lambdas04:38
- Higher order functions08:51
- Practice: Lambdas and Higher Order Functions05:43
- Exercise: Lambdas and Higher Order Functions00:15
- Solution: Lambdas and Higher Order Functions06:27
- Common Higher Order functions11:14
- Practice: Common Higher Order Functions08:14
- Exercise: Common Higher Order Functions00:07
- Solution: Common Higher Order Functions03:17
- Section recap02:39
- Section objectives01:26
- Packages11:41
- Naming convention04:49
- Imports03:49
- Practice: Packages02:47
- Exercise: Packages00:10
- Solution: Packages06:22
- Section recap02:15
- Intro01:44
- Creating the project05:38
- Game art07:32
- Starting position03:43
- Game algorithm06:16
- Completing the game03:49
- Intro01:39
- Create the board05:30
- Player move05:03
- Check winner07:59
- Check tie04:07
- Completing the game06:45
- Conclusion01:12
- Section objectives03:18
- What is OOP10:59
- Classes06:51
- Objects07:26
- Practice: Object Oriented Programming03:59
- Practice: Object Oriented Programming 204:45
- Practice: Object Oriented Programming 305:36
- Exercise: Object Oriented Programming00:15
- Solution: Object Oriented Programming05:04
- The "Object" construct07:03
- Classes and Objects08:27
- Inheritance05:10
- Practice: Inheritance04:04
- Practice: Inheritance205:51
- Exercise: Inheritance00:14
- Solution: Inheritance05:00
- Section recap03:03
- Section objectives02:48
- Constructors07:38
- Practice: Constructors04:12
- Exercise: Constructors00:11
- Solution: Constructors06:58
- Getters and Setters07:52
- Practice: Getters and Setters04:00
- Exercise: Getters and Setters00:15
- Solution: Getters and Setters03:51
- The this keyword06:11
- Practice: The 'this' keyword02:17
- Exercise: The 'this' keyword00:09
- Solution: The 'this' keyword03:08
- The init block03:45
- Practice: The init block02:19
- Exercise: The init block00:05
- Solution: The init block01:31
- Companion object04:20
- Practice: Companion object02:56
- Practice: Companion object10:40
- Exercise: Companion object00:08
- Solution: Companion object02:46
- Section recap02:57
- Section objectives02:17
- The 4 principles of OOP06:05
- Inheritance08:44
- Practice: Inheritance04:09
- Exercise: Inheritance00:14
- Solution: Inheritance03:27
- Encapsulation and scope10:23
- Practice: Encapsulation and scope05:44
- Exercise: Encapsulation and scope00:13
- Solution: Encapsulation and scope03:37
- Abstraction10:17
- Practice: Abstraction08:01
- Exercise: Abstraction00:11
- Solution: Abstraction04:38
- Polymorphism07:02
- Practice: Polymorphism03:24
- Exercise: Polymorphism00:09
- Solution: Polymorphism04:32
- Section recap02:15
- Section objectives01:35
- What is an interface07:53
- Implementation05:47
- Practice: Interfaces04:06
- Practice: Interfaces205:06
- Exercise: Interfaces00:15
- Solution: Interfaces07:16
- More interfaces10:07
- Practice: More interfaces04:53
- Exercise: More interfaces00:12
- Solution: More interfaces05:24
- Section recap01:46
- Section objectives01:11
- Standard functions04:57
- Let08:44
- Practice: Let05:15
- Exercise: Let00:07
- Solution: Let05:37
- With05:29
- Practice: With02:39
- Exercise: With00:08
- Solution: With02:17
- Run05:56
- Practice: Run03:09
- Exercise: Run00:10
- Solution: Run02:02
- Apply05:25
- Practice: Apply04:33
- Exercise: Apply00:12
- Solution: Apply04:20
- Also03:27
- Practice: Also02:01
- Exercise: Also00:05
- Solution: Also02:09
- takeIf and takeUnless05:03
- Practice: takeIf and takeUnless05:29
- Exercise: takeIf and takeUnless00:05
- Solution: takeIf and takeUnless03:17
- Section recap01:45
- Section objectives01:31
- Data classes05:04
- Data class methods08:17
- Practice: Data classes05:51
- Exercise: Data classes00:08
- Solution: Data classes08:14
- Enum classes07:58
- Practice: Enum classes05:50
- Exercise: Enum classes00:15
- Solution: Enum classes04:42
- Sealed classes07:05
- Practice: Sealed classes02:47
- Exercise: Sealed classes00:11
- Solution: Sealed classes05:52
- Nested classes08:23
- Practice: Nested classes02:19
- Exercise: Nested classes00:09
- Solution: Nested classes02:57
- Section recap02:21
- Section objectives01:27
- Extensions04:17
- Extension functions06:13
- Extension properties04:26
- Practice: Extension functions02:20
- Exercise: Extension functions00:07
- Solution: Extension functions01:29
- Companion object extensions03:22
- Practice: Companion object extensions01:19
- Exercise: Companion object extensions00:05
- Solution: Companion object extensions01:11
- Section recap02:13
- Section objectives01:12
- Generic type parameters08:34
- Practice: Generics03:04
- Exercise: Generics00:09
- Solution: Generics03:14
- Type constraints05:44
- Practice: Type constraints03:19
- Exercise: Type constraints00:18
- Solution: Type constraints05:01
- Section recap01:25
- Section objectives01:11
- Type casting (as)08:33
- Practice: type casting03:59
- Exercise: type casting00:12
- Solution: type casting03:44
- lateinit05:58
- Practice: lateinit02:18
- Exercise: lateinit00:17
- Solution: lateinit02:11
- lazy03:28
- Practice: lazy02:33
- Exercise: lazy00:13
- Solution: lazy02:52
- Section recap01:08
- Project description04:10
- Set up project05:35
- Building the GUI05:39
- Adding the CSS01:41
- CSS00:13
- Building the application and running the project05:13
- Operators03:20
- Adding the functionality14:36
- Section objectives04:37
- Installing Android Studio (short version)04:39
- Install Android Studio on a Mac07:14
- Install Android Studio on a PC09:48
- How to test the app on your phone03:23
- Create the project07:36
- App functionality06:50
- Section objectives02:28
- Multithreading (briefly)07:47
- Coroutines07:01
- Hello World05:54
- Scope06:37
- Context04:54
- Suspending functions05:57
- Jobs07:55
- Dispatchers10:03
- async06:20
- withContext03:12
- Exception Handling08:48
- Section objectives01:57
- Setting up the project02:56
- Gradle dependencies00:03
- Downloading an image06:40
- Challenge00:40
- Applying the filter04:24
- Intro02:06
- Setting up the project07:20
- Gradle dependencies00:03
- Setting up Retrofit05:45
- Creating the coroutines06:09
- Debugging10:43
- Finding keyboard shortcuts03:51
- Common shortcuts07:24
- Cleaning and rebuilding02:28
- Conclusion01:19
- Further resources00:02
- Thank youProcessing..
Online Courses
Learning Kotlin doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Kotlin related books and take your
YouTube videos
The number of high-quality and free Kotlin video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more