Complete C# Masterclass
Learn C# Programming - WPF, Databases, Linq, Collections, Game Development with Unity. More than just the C# basics!
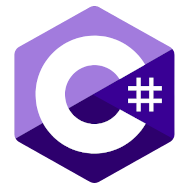
What you will learn?
- Learn the fundamentals of programming using C#
- Learn how to use variables, methods, loops, conditions
- Fully understand how OOP (object oriented Programming) works and how to use it.
- Build beautiful GUIs (Graphical User Interfaces) with WPF (Windows Presentation Foundation)
- Create video games using C# and Unity 3D
- Learn how to handle errors and avoid them
- Work with files and text
- Learn how to use Linq and Lambda Expressions
- Learn how to use Databases with MS SQL Server and Linq
- Learn advanced techniques like threading and asynchronus development
Your trainer

Denis Panjuta
Hi. I'm Denis. I have a degree in engineering from the University for Applied Science Konstanz in Germany and discovered my love for programming there.
Currently over 100,000 students learn from my courses. This gives me a lot of energy to create new courses with the highest quality possible. My goal is to make learning to code accessible for everyone, as I am convinced, that IT is THE FUTURE!
So join my courses and learn to create apps, games, websites or any other type of application. The possibilities are limitless.
283 lessons
Easy to follow lectures and videos covering subject details.
37 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction01:46
- What Do You Want To Achieve?02:09
- Installing Visual Studio Community06:24
- Udemy Reviews Update00:25
- Difference between the .NET5 and .NET6 Console Template07:06
- Hello World - First Program13:09
- Hello World on a Mac02:03
- Visual Studio Interface07:42
- What To Do If You Get Stuck00:20
- BONUS: additional materials00:34
- Intro Quiz3 questions
- Chapter 1 Summary01:09
- Get the most from Tutorials.EU00:08
- Quick Hint00:15
- High Level Overview of Variables and Datatypes04:25
- More Datatypes and Their Limits08:39
- Other Datatypes00:13
- Datatypes Int, Float and Double14:56
- Datatype String And Some Of Its Methods04:57
- Datatypes And Variables4 questions
- Coding Standards03:54
- Value vs Reference Types03:58
- Console Class and some of its Methods11:03
- Changing The Consoles Colors07:54
- Naming Conventions and Coding Standards05:43
- Implicit and Explicit Conversion08:31
- Parsing a String To An Integer05:19
- Exercise: Parsing1 question
- String Manipulation09:18
- Some String Methods05:25
- Challenge String and its methods00:46
- Solution For Exercise: Strings 105:30
- Challenge String and its methods 200:36
- Solution For Exercise: Strings 205:36
- Challenge - Datatypes And Variables00:29
- Solution For The Challenge "Datatypes And Variables"04:26
- Constants05:31
- Datatypes and Variables Part 23 questions
- C# Cheat sheets00:36
- DataTypes Summary00:53
- Methods Intro01:14
- Intro To Functions / Methods07:08
- Void Methods08:14
- Methods With Return Value And Parameters08:54
- Methods3 questions
- Challenge - Methods00:18
- Solution For The Challenge "Methods"04:04
- Test your methods1 question
- User Input09:00
- Try Catch and Finally14:39
- Operators17:41
- Operators3 questions
- Methods Summary00:58
- Making Decisions Intro00:54
- Introduction To Decision Making In C#11:31
- Intro to TryParse05:20
- IF And Else If + Try Parse04:34
- If & Else Coding Exercise1 question
- Nested If Statements09:53
- Nested If Statements1 question
- Challenge - If Statements00:19
- Solution For The Challenge "If Statements"07:14
- Switch Statement06:34
- Challenge - If Statements 200:23
- Solution For The Challenge "If Statements 2"05:47
- Enhanced If Statements - Ternary Operator09:02
- Enhanced If Statements - Ternary Operator - Challenge00:41
- Enhanced If Statements - Ternary Operator - Challenge - Solution05:24
- If-Statements5 questions
- Making Decisions Summary01:54
- Loops Intro00:55
- Basics of Loops05:38
- For Loops06:58
- Do While Loops07:59
- While Loops06:03
- Loops1 question
- break and continue05:34
- break and continue practice1 question
- Challenge - Loops 1 - Average00:29
- Solution For The Challenge "Loops"12:17
- Loops3 questions
- Loops Summary01:16
- Objects Intro02:07
- Introduction To Classes And Objects03:15
- Our First Own Class11:09
- Using Constructors11:50
- Using Multiple Constructors11:45
- Constructors1 question
- Access Modifiers00:05
- Constructors and Member-Variables3 questions
- Properties Part 120:03
- Properties Part 218:24
- Challenge - Properties02:10
- Members And Finalizers/Destructors14:43
- Objects Summary01:23
- Arrays Intro00:58
- Basics of Arrays04:08
- Declaring and Initializing Arrays and the Length Property07:07
- Foreach Loops09:28
- Arrays, for and foreach loops1 question
- Foreach Loops and Switch Statement challenge01:02
- Foreach Loops and Switch Statement challenge - solution09:08
- Multi Dimensional Arrays14:25
- Nested For Loops And 2D Arrays11:45
- Nested For Loops And 2D Arrays - Two Examples09:06
- Tic Tac Toe winner identifier1 question
- Arrays Quiz5 questions
- Challenge - Tic Tac Toe48:34
- Jagged Arrays09:08
- Challenge - Jagged Arrays06:36
- Using Arrays As Parameters09:01
- Why you can change an array by passing it as a variable00:19
- Params Keyword11:44
- Getting The Min Value Of Many Given Numbers Using Params07:30
- Overview Generic and Non-Generic Collections05:30
- ArrayLists11:24
- Lists04:04
- Lists1 question
- Hashtables15:35
- Hashtables - Challenge05:01
- Dictionaries18:13
- Editing And Removing Entries in a Dictionairy06:41
- Dictionary practice1 question
- Queues and Stacks Overview05:00
- Stacks in Csharp08:59
- Queues14:52
- Arrays Summary02:08
- Debugging Intro00:49
- Debugging Basics12:35
- Locals and Autos10:42
- Debugging, Creating Copies of Lists and solving some bugs10:34
- Debugging Call Stack, Throwing Errors and defensive programming14:33
- Welcome to Inheritance01:03
- Introduction To Inheritance04:41
- Simple Inheritance Example18:22
- Virtual and Override Keywords13:35
- Inheritance Demo23:04
- Inheritance Challenge - Videopost and Timer with Callback24:04
- Inheritance Challenge 2 - Employees, Bosses and Trainees00:30
- Inheritance Challenge 2 - Employees, Bosses and Trainees Solution16:32
- Interfaces Intro06:49
- Creating And Using Your Own Interfaces18:25
- Inheritances and Interfaces1 question
- IEnumerator and IEnumerable14:35
- IEnumerable Example 107:49
- IEnumerable Example 204:10
- IEnumerable1 question
- Inheritance Outro00:39
- Polymorphism Intro00:25
- Polymorphic Parameters16:41
- Sealed Key Word05:22
- Has A - Relationships07:41
- Abstract13:37
- Abstract and as & is Keyword / Polymorphism06:58
- Interfaces vs Abstract Classes07:10
- Read from a Textfile05:32
- Write into a Text File11:55
- Parsing game (part 1) / Read from and write into a file1 question
- Polymorphism Summary01:09
- Advanced Topics Intro00:43
- .net core vs .net framework00:10
- Access Modifiers06:21
- Structs07:47
- Enums05:35
- Math Class08:35
- Math Class1 question
- Random Class06:20
- VS 2019 and Regular expressions00:14
- Regular Expressions43:36
- Parsing game (part 2) / Regular Expressions1 question
- DateTime21:06
- Nullables12:01
- Garbage Collector07:58
- Main Args Explained part 107:20
- Main Args Explained Using User Input - Create A CMD App06:38
- Delegates intro01:28
- Delegates Introduction04:43
- Delegates Basics05:37
- Creating your own Delegates06:07
- Delegates1 question
- Anonymous Methods06:45
- Lambda Expressions06:31
- A hint for the next exercise00:22
- Lambda expressions1 question
- Events and Multicast Delegates20:13
- Delegates Outro01:35
- WPF Intro01:22
- Introduction To WPF - And When To Use It06:26
- XAML Basics and Code Behind20:01
- StackPanel - Listbox - Visual and Logical Tree10:16
- Routed Events - Direct, Bubbling and Tunneling08:48
- More details on Routed Events00:09
- Grid13:01
- Dependency Properties15:26
- Data Binding11:11
- INotifyPropertyChanged Interface15:33
- ListBox and a List of Current Matches21:45
- ComboBox05:10
- CheckBox12:04
- ToolTip02:48
- RadioButtons and Images11:29
- Property, Data and Event Triggers19:52
- PasswordBox05:19
- WPF Summary01:16
- Databases Intro01:04
- Setup MS SQL Server and VS For DB work09:53
- Intro And Setting Up Our DataSet And Table13:35
- Relationship or Associative Tables14:07
- Showing Data in a ListBox13:36
- Showing Associated Data10:24
- Displaying all Animals In The ListBox08:11
- Deleting From A Table With A Click16:07
- Added Note - Adding the base tables and entries back01:22
- Delete Animals, Remove Animals and Add Animals Functionality17:00
- Updating Entries in Our Tables15:53
- Databases Outro00:39
- Linq Intro00:52
- Linq gentle Introduction03:56
- Linq Demo05:35
- Linq with Lists - and our University Manager Part 113:28
- Sorting and Filtering with Linq15:10
- Creating collections based on other collections06:52
- Linq with XML17:38
- Setting up the project for LinqToSQL15:48
- Inserting Objects into our Database19:12
- Using assiociative tables with Linq16:25
- Joining tables next level09:22
- Deleting and Updating13:30
- Linq Outro00:33
- Part 1 - Building The GUI And Using Static Data00:09
- Part 2 - Building A Grid Table And Using A Database00:07
- Part 3 - Using Live Currency Values from the Internet Using An API And JSON30:56
- Threads Intro00:51
- Threads Basics11:51
- Thread Start and End &Completion09:53
- ThreadPools and Threads in The Background09:06
- Join And IsAlive13:35
- Tasks and WPF24:10
- Threads Readings00:08
- Threads Outro00:39
- Intro Unity Basics02:35
- Installing Unity00:02
- Overview of the Unity Interface07:43
- Creating your own Layout03:46
- Player Movement13:12
- Making Sure We Make Changes Correctly03:05
- Physis Basics04:35
- RigidBody - A Physical Body09:38
- Colliders And Their Different Types12:05
- Triggers10:21
- Prefabs And GameObjects05:11
- Components And More On Prefabs04:12
- Keeping The Hierarchy Tidy02:29
- Class Structure08:58
- Mathf And Random Class04:57
- Unity Basics Outro01:48
- Pong Introduction02:05
- Basics - UI Elements06:47
- Basics - Accessing Text Through Code05:44
- Basics - Buttons05:48
- Basics - Switching Scenes09:35
- Basics - Play Sound08:52
- Project Outline - Pong07:26
- Creating The Main Menu15:03
- Switching Scenes and Using Buttons09:01
- Building Our Game Scene13:58
- 2D vs 3D Colliders and Rigidbody For Our Ball06:56
- Moving Our Ball Left And Right11:14
- Racket Movement09:43
- Bouncing Off Correctly09:12
- Scoring System08:22
- Restarting A Round05:27
- The Game Over Screen04:23
- Adding Sound To The Game07:26
- Adding a Basic AI05:17
- Chapter Summary01:22
- Chapter Intro00:45
- Zig Zag Intro00:40
- Basics- Instatiating (Creating Via Code) An Object05:02
- Basics - Invoke And InvokeRepeating For Delayed Calls And Repeated Calls04:23
- Basics - Playerpreferences - Saving Data06:38
- Basics - Raycast07:48
- Setup For Zig Zag07:31
- Setting The Perspective07:23
- Moving The Character06:28
- Make Camera Follow Player03:32
- Animate The Character13:30
- Start The Game08:49
- Restart The Game04:01
- Collecting Crystals And Increasing The Score09:35
- Adding A Highscore05:08
- Adding The Particle Effect12:58
- Background Music Loop06:26
- Procedural Creation Of Our Map20:53
- Chapter Intro01:47
- Create Fruits And Explode Them16:22
- Fruit Spawner16:52
- Creating Our Blade13:38
- GUI and Bombs21:17
- Game Over and Restart12:12
- Adding The Highscore09:05
- Extend The Game06:46
- Prepare Code For Android06:35
- Test On An Android Device04:01
- Make Some Adjustments02:15
- Adding Unity Ads to Your Game06:28
- Setting Up Your Device as Developer Device01:20
- Adding Sound09:45
- Thanks for finishing the course04:29
- YOUR SPECIAL BONUS00:17
Online Courses
Learning C# doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of C# related books and take your
YouTube videos
The number of high-quality and free C# video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more