Angular & NodeJS - The MEAN Stack Guide [2023 Edition]
Learn how to connect your Angular Frontend to a NodeJS & Express & MongoDB Backend by building a real Application
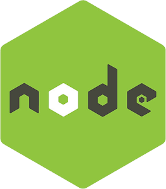
What you will learn?
- Build real Angular + NodeJS applications
- Understand how Angular works and how it interacts with Backends
- Connect any Angular Frontend with a NodeJS Backend
- Use MongoDB with Mongoose to interact with Data on the Backend
- Use ExpressJS as a NodeJS Framework
- Provide a great user experience by using Optimistic Updating on the Frontend
- Improve any Angular (+ NodeJS) application by adding Error Handling
Your trainer

Maximilian Schwarzmüller
Starting out at the age of 13 I never stopped learning new programming skills and languages. Early I started creating websites for friends and just for fun as well. Besides web development I also explored Python and other non-web-only languages. This passion has since lasted and lead to my decision of working as a freelance web developer and consultant. The success and fun I have in this job is immense and really keeps that passion burningly alive.
Starting web development on the backend (PHP with Laravel, NodeJS, Python) I also became more and more of a frontend developer using modern frameworks like React, Angular or VueJS 2 in a lot of projects. I love both worlds nowadays!
152 lessons
Easy to follow lectures and videos covering subject details.
12.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction01:57
- What is MEAN?10:35
- Join our Online Learning Community00:25
- What is a Single Page Application (SPA)?02:08
- How Does the MEAN Stack Work?02:58
- MUST READ: Angular CLI - Latest Version00:16
- Installing Node & the Angular CLI07:50
- Installing our IDE03:13
- Exploring the Project Structure04:02
- Course Outline03:27
- How To Get The Most Out Of This Course02:53
- Section Resources00:20
- Module Introduction01:40
- Understanding the Folder Structure06:40
- Understanding Angular Components02:39
- Adding our First Component08:01
- Listening to Events03:43
- Outputting Content05:34
- Getting User Input06:03
- Installing Angular Material15:34
- Adding a Toolbar03:57
- Outputting Posts06:37
- Diving Into Structural Directives05:40
- Creating Posts with Property & Event Binding07:38
- Creating a Post Model04:35
- Adding Forms08:18
- Getting Posts from Post-Create to Post-List09:26
- Calling GET Post11:16
- More About Observables05:51
- Working on our Form03:51
- Section Resources00:09
- Module Introduction02:03
- Connecting Node & Angular - Theory04:37
- What is a RESTful API?04:45
- Adding the Node Backend08:20
- Adding the Express Framework07:37
- Improving the server.js Code04:40
- Fetching Initial Posts05:57
- Using the Angular HTTP Client09:20
- Understanding CORS05:31
- Adding the POST Backend Point05:27
- Adding Angular05:07
- Section Resources00:04
- Module Introduction01:04
- What is MongoDB?01:48
- Comparing SQL & NoSQL04:26
- Connecting Angular to a Database02:07
- Setting Up MongoDB05:14
- Using MongoDB Atlas & IP Whitelist00:19
- Adding Mongoose03:01
- Understanding Mongoose Schemas & Models05:45
- Creating a POST Instance04:08
- Connecting our Node Express App to MongoDB03:10
- Storing Data in a Database05:51
- Fetching Data From a Database05:34
- Transforming Response Data05:07
- Deleting Documents07:37
- Updating the Frontend after Deleting Posts03:02
- Adding Posts with an ID07:07
- Section Resources00:02
- Module Introduction01:11
- Adding Routing10:12
- Styling Links04:00
- Client Side vs Server Side Routing02:44
- Possible Error00:08
- Creating the "edit" Form10:38
- Finishing the Edit Feature11:09
- Updating Posts on the Server09:21
- Re-Organizing Backend Routes05:54
- Adding Loading Spinners09:05
- Section Resources00:01
- Module Introduction00:44
- Adding the File Input Button04:51
- Converting the Form from a Template Driven to a Reactive Approach13:41
- Adding Image Controls to Store the Image06:59
- Adding an Image Preview04:58
- Starting with the Mime-Type Validator08:20
- Finishing the Image Validator07:17
- Adding Server Side Upload08:23
- Uploading Files06:05
- Working with the File URL06:18
- Beware of the Spread (...) Operator00:37
- Fetching Images on the Frontend06:37
- Updating Posts with Images13:20
- Wrap Up00:54
- Section Resources00:02
- Module Introduction01:04
- Adding the Pagination Component07:18
- Working on the Pagination Backend08:08
- Connecting the Angular Paginator to the Backend05:24
- Fetching Posts Correctly06:09
- Finishing Touches04:48
- Section Resources00:02
- Module Introduction00:59
- Adding the Login Input Fields08:15
- Handling User Input03:42
- Adding the Signup Screen03:32
- Creating the User Model06:23
- Creating a New User Upon Request05:30
- Connecting Angular to the Backend06:38
- Understanding SPA Authentication03:39
- Implementing SPA Authentication09:32
- Sending the Token to the Frontend05:43
- Adding Middleware to Protect Routes11:47
- Adding the Token to Authenticate Requests13:45
- Improving the UI Header to Reflect the Authentication Status07:23
- Improving the UI Messages to Reflect the Authentication Status06:19
- Connecting the Logout Button to the Authentication Status02:32
- Redirecting Users04:25
- Adding Route Guards06:48
- Reflecting the Token Expiration in the UI06:12
- Saving the Token in the Local Storage15:54
- Section Resources00:02
- Module Introduction01:09
- Adding a Reference to the Model03:28
- Adding the User ID to Posts07:02
- Changed Mongoose Syntax00:14
- Protecting Resources with Authorization08:19
- Passing the User ID to the Frontend07:24
- Using the User ID on the Frontend05:11
- Section Resources00:03
- Module Introduction01:14
- Testing Different Places to Handle Errors09:49
- The Error Interceptor08:01
- Displaying the Basic Error Dialog05:13
- Adding an Error Dialog05:26
- Returning Error Messages on the Server07:10
- Finishing Touches03:03
- Section Resources00:01
- Module Introduction00:34
- Using Controllers08:16
- Separating the Middleware03:30
- Creating an Angular Material Module04:20
- Splitting the App Into Feature Modules05:14
- Fixing an Update Bug02:31
- Creating the Auth Module04:08
- Adding Lazy Loading09:35
- Fixing the AuthGuard00:04
- Using a Global Angular Config06:18
- Using Node Environment Variables05:26
- Section Resources00:02
- Module Introduction00:40
- Deployment Options04:00
- Deploying the REST API00:26
- Deploying the REST Api13:34
- Deploying Angular00:27
- Angular Deployment - Finishing the Two App Setup09:51
- Using the Integrated Approach09:37
- Section Resources00:01
- Thanks for being part of the course!00:55
- Possible Addition: Add Web Sockets for Realtime Refresh00:18
- Bonus: More Content!00:20
Online Courses
Learning NodeJS doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of NodeJS related books and take your
YouTube videos
The number of high-quality and free NodeJS video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more