The Modern Angular Bootcamp
Get job ready with Angular! Understand how to build and deploy production-ready apps.
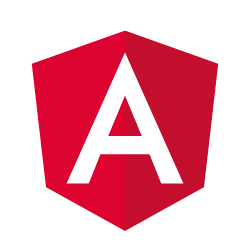
What you will learn?
- Build amazing single page applications with Angular and Typescript
- Master fundamental concepts behind structuring Angular applications
- Realize the power of building composable components
- Be the engineer who explains how Angular works to everyone else, because you know the fundamentals so well
- Build a portfolio of projects to show any potential employer
- Architect large apps effectively using Typescript + Angular's Module system
- Handle and process data declaratively using RxJs
Your trainer

Stephen Grider
Stephen Grider has been building complex Javascript front ends for top corporations in the San Francisco Bay Area. With an innate ability to simplify complex topics, Stephen has been mentoring engineers beginning their careers in software development for years, and has now expanded that experience onto Udemy, authoring the highest rated React course. He teaches on Udemy to share the knowledge he has gained with other software engineers. Invest in yourself by learning from Stephen's published courses.
511 lessons
Easy to follow lectures and videos covering subject details.
45.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- How to Get Help01:05
- Course Resources00:38
- Join Our Community!00:07
- Intro - Our First App08:49
- A Few Questions Answered07:23
- Four Major File Types3 questions
- Environment Setup - Node JS00:26
- Environment Setup - Angular CLI00:37
- Environment Setup - Visual Studio Code01:00
- Project Overview03:24
- Starting and Stopping an Angular Project02:32
- Updating a Component Template06:07
- Event Binding Syntax07:02
- Property Binding Syntax06:54
- Interpolation Syntax04:15
- Angular vs JavaScript Design Patterns04:13
- Tracking Input State04:38
- Tracking Additional Properties04:47
- Handling Text Input08:22
- Generating a Random Password06:35
- Review on Property Binding04:30
- Adding Third Party CSS03:18
- CSS Import Statements03:12
- Adding Some Styling02:24
- Structural Directives05:41
- Deploying Angular Apps03:53
- Terminology Review02:24
- Terminology Review4 questions
- App Overview02:16
- Project Setup03:37
- A Quick HTML Dump05:28
- Adding Static Files to Angular03:50
- Scoped CSS Files06:17
- Components in Angular06:59
- Creating Components05:22
- How Angular Creates Components07:01
- More on CSS Scoping04:13
- Tying Data to a Component05:01
- Accepting Data in a Child Component09:08
- Communicating from Parent to Child05:13
- Fixing Template References04:27
- Building Lists with NgFor04:30
- Two Notes on NgFor03:47
- Host Element Selectors06:41
- Deployment and Review04:31
- What We've Learned So Far4 questions
- App Overview03:54
- Initial Steps01:35
- Adding CSS04:36
- IMPORTANT Info About Faker Installation00:39
- Displaying Content08:50
- Randomly Generating Text03:32
- Handling User Input02:47
- Conditional Display08:54
- Character by Character Comparison06:20
- Styling by Comparison08:30
- Pipes Overview03:12
- Adding an Input03:43
- Pipes in Action02:26
- A Touch of Styling04:30
- Adding a Date Picker03:16
- Formatting Dates07:15
- Displaying Currency03:25
- Formatting the Currency03:03
- Formatting Numbers05:04
- Displaying JSON02:52
- Creating Custom Pipes06:57
- Custom Arguments05:03
- Two Neat Things with Pipes05:10
- App Overview02:02
- App Setup02:45
- Getting Some Data02:40
- Review on NgFor04:46
- The NgClass Directive03:39
- More on NgClass04:36
- Conditionally Disabling Buttons04:03
- Changes Pages02:56
- Displaying Images01:42
- Reminder on NgIf03:47
- Multiple Directives with Ng-Container08:19
- NgSwitch04:33
- Generating Custom Directives03:09
- Accessing Elements from a Custom Directive03:26
- Communicating Properties to Directives06:41
- Intercepting a Property Assignment06:12
- Input Aliasing02:58
- Replacing NgClass04:06
- Custom Structural Directives08:54
- Context in Structural Directives04:40
- App Overview04:46
- Tackling Some Challenges04:41
- Modules Overview05:03
- Generating Modules05:17
- Importing and Exporting Modules06:40
- Modules Exercise02:09
- Quick Note00:12
- Modules Exercise Solution03:28
- Module Property Definitions02:24
- Adding Basic Routing05:26
- Routing Exercise01:36
- Exercise Solution02:10
- The RouterOutlet Element04:03
- Navigating with RouterLink04:17
- A Touch of Styling04:24
- Styling an Active Link01:40
- Adding Home and NotFound Routes05:58
- Reordering Routing Rules04:37
- Landing and NotFound Components01:42
- Lazy vs Eager Loading04:14
- Implementing Lazy Loading09:13
- Lazy Loading Exercise02:53
- Exercise Solution03:43
- Creating a Placeholder Component05:14
- Customizing Components04:56
- Reminder on Structural Directives05:05
- Widget Modules04:38
- Implementing a Titled Divider04:59
- Grabbing Content with NgContent05:02
- Creating a Segment Component03:42
- NgContent with Selects09:36
- Hiding Empty Elements03:34
- Building a Reusable Table04:10
- Generating and Displaying the Table02:47
- Communicating Down Table Data04:30
- Assembling the Table07:27
- Passing Down Class Names04:07
- Tab Based Navigation03:04
- Adding Child Navigation Routes04:20
- Understanding Child Component Routing05:24
- RouterLink Configuration05:45
- Relative RouterLink References09:01
- Alternate RouterLink Syntax03:17
- Matching Exact Paths02:21
- A Reusable Tabs Component03:47
- Views Module Exercise01:54
- Exercise Solution04:36
- Displaying a List of Statistics07:00
- Displaying a List of Items09:02
- The Mods Module02:19
- Modal Window Setup05:15
- More Basic Modal Setup04:13
- Natural Issues with Modal Windows06:29
- Solving the Modal Issue05:50
- Lifecycle Hooks07:54
- Hiding the Modal with NgOnDestroy04:35
- Opening the Modal04:44
- Closing the Modal04:05
- Stopping Event Bubbling02:47
- Making the Modal Reusable11:15
- Building an Accordion02:12
- Listing Accordion Elements07:29
- Expanding the Active Element05:19
- Intro to TypeScript04:21
- Basic Types06:54
- Type Inference05:06
- Why TypeScript at All?04:41
- TS with Functions07:00
- Difficulties with Objects05:02
- Introducing Interfaces08:26
- Classes and Properties07:20
- Public and Private06:49
- Property Assignment Shortcut02:25
- Enabling Decorator Support05:38
- Decorators08:25
- The Module System02:14
- Strict Mode06:03
- Combining Interfaces and Classes06:19
- Class Generics07:22
- Function Generics08:24
- That's a Lot of Typescript!4 questions
- App Overview03:33
- App Architecture06:26
- Generating Services02:49
- Component Design Methodology07:17
- Handling Form Submission04:20
- Child to Parent Communication05:36
- The Wikipedia API06:19
- Notes on Services02:57
- Accessing Services04:59
- Really Weird Behavior13:52
- Where'd That Come From08:30
- Ok, But Why?06:48
- Why Dependency Injection is Useful12:18
- Making HTTP Requests05:45
- Seeing the Request's Response05:05
- More Parent to Child Communication06:51
- Building the Table05:15
- Escaping HTML Characters07:19
- XSS Attacks09:27
- More on XSS Attacks08:19
- Adding Title Links05:22
- Another CSS Gotcha04:09
- Last Bit of Styling04:42
- Notes on RxJs06:17
- A Quick JS Example07:34
- Adding RxJs Terminology06:05
- Creating an Observable07:49
- Implementing the Processing Pipeline09:06
- More Processing!09:29
- Adding an Observer07:09
- Operator Groups04:45
- Specific Operators05:24
- Low Level Observables11:38
- Alternative Observer Syntax03:20
- Unicast Observables04:31
- More on Unicast Observables07:03
- Multicast Observables03:05
- Multicast in Action05:24
- Hot vs Cold Observables04:47
- RxJs Terminology3 questions
- RxJs in an Angular World03:33
- Applying TypeScript to RxJs07:59
- Generics with RxJs06:53
- Using TypeScript to Catch Errors05:46
- TypeScript is Smart07:39
- Summary02:30
- App Overview02:02
- App Architecture Design02:55
- API Signup05:32
- HTTP Module Hookup03:34
- HTTP Dependency Injection06:21
- Making the Request04:11
- Displaying the Component02:33
- Making the Request04:01
- Using a Generic Type03:13
- Displaying the Image02:50
- Refetching Data04:22
- App Overview04:26
- Reactive Forms vs Template Forms05:50
- Creating a Form Instance07:12
- Binding a FormGroup to a Form05:37
- Validating Fields03:52
- Finding Validation Errors03:54
- Nasty Error Handling07:01
- Showing and Hiding Validation Messages07:41
- Making a Reusable Input06:20
- Adding Message Cases06:21
- Changing Styling on Validation Errors05:38
- Adding Additional Inputs03:39
- Handling Form Submission04:09
- Additional Validations06:58
- Input Masking04:08
- Hijacking Form Control Values08:53
- Inserting Extra Characters05:34
- Reformatting the Input06:57
- Using a Library for Masking09:48
- Resetting a Form06:10
- A Touch of Styling03:58
- Adding a Credit Card06:45
- App Overview01:13
- Basic Template Form Setup05:36
- Two Way Binding Syntax03:23
- Differences Between Template and Reactive Forms09:11
- Validation Around Template Forms03:51
- Conditional Validation04:24
- Handling Form Submission02:16
- Adding Styling and Wrapup04:07
- App Overview03:05
- App Setup02:04
- Possible Design Approaches05:29
- Displaying Form Values06:32
- Adding Custom Validation08:33
- Extracting Custom Validation Logic06:18
- Making Validators Reusable05:21
- RxJs with Reactive Forms03:05
- Handling Correct Answers05:18
- The Delay Operator03:45
- Adding a Statistic04:38
- RxJs Solution06:28
- A Touch of Styling03:08
- Helping the User Along03:04
- Accessing FormGroups from Custom Directives11:01
- Detecting Changes07:16
- Applying a Class Name03:14
- App Overview04:30
- The API Server01:55
- Contacting the Backend API08:46
- Cookie Based Authentication05:26
- File Generation03:58
- Navigation Reminder06:17
- Adding a Signup Form05:25
- Adding Basic Styling03:44
- Username and Password Validation09:05
- Writing Custom Validators09:39
- Connecting Custom Validators06:05
- Implementing Async Validators09:52
- Nasty Async Validators07:41
- Understanding Async Validators11:43
- Handling Errors from Async Validation10:37
- Building an Auth Service07:36
- Another Reusable Input06:09
- Robust Error Handling08:33
- Customizing Input Type04:59
- Fixing a Few Odds and Ends07:11
- Signup Process Overview04:09
- Making the Signup Request09:34
- Cleaning up the Auth Service06:30
- Handling Signup Errors07:59
- Generic Error Handling02:50
- Adding a Navigation Header04:53
- Maintaining Authentication State06:59
- Oh No, More RxJs12:16
- Using BehaviorSubjects08:22
- The Async Pipe04:36
- Exact Active Links01:52
- Checking Auth Status06:46
- A Gotcha Around the HttpClient05:36
- HTTP Interceptors08:59
- Wiring up an Interceptor06:35
- Modifying Outgoing Requests04:08
- Other Uses of Interceptors08:07
- A Little Record Keeping04:51
- Adding Sign Out06:31
- Automated Signouts06:39
- Programmatic Navigation03:19
- Building the Sign In Flow05:50
- Sign In Authentication08:13
- Showing Authentication Errors07:32
- Inbox Module Design08:14
- Navigation on Authentication03:51
- Restricting Routing with Guards09:15
- Issues With Guards10:29
- A Solution to the Guard with RxJs09:51
- Implementing Our RxJs Solution04:58
- Navigation on Failing a Guard03:21
- Generating Inbox Components04:41
- Retrieving Emails06:32
- Adding Email Fetching to the Service05:37
- Connecting the Service04:33
- Rendering a List of Emails04:55
- Child Route Navigation07:48
- Relative Links with Router Link04:05
- Styling the Selected Email04:34
- Placeholder Markup01:01
- Extracting URL Params06:26
- Accessing Route Information07:26
- Snapshot vs Observable Route Params04:21
- Issues with Nested Subscribes06:59
- Canceling Previous Email Requests04:49
- Handling Undefined Data07:26
- Reusable Data Fetching with Resolvers04:26
- Using a Resolver07:12
- Communicating Data Out of a Resolver08:33
- Error Handling with Resolvers08:14
- Showing Email HTML06:35
- Component Reuse in Email Creation03:08
- A Reusable Modal04:52
- Some More Modal Implementation08:52
- Where to Place the Modal?06:08
- Toggling Modal Visibility05:12
- Providing a Form Default Values08:12
- Displaying Email Inputs05:29
- Adding Form Validation05:28
- Displaying Textarea instead of Text Input03:41
- Capturing the Username06:46
- Submitting a New Email07:16
- Sending Original Email05:18
- Replying to Emails05:04
- Formatting Reply Emails09:56
- Sending Replies04:05
- Quick Bug Fix! (Final Lecture)01:14
- Type Annotations and Inference02:03
- Annotations and Variables04:53
- Object Literal Annotations06:53
- Annotations Around Functions05:55
- Understanding Inference03:51
- The Any Type07:47
- Fixing the "Any" Type01:49
- Delayed Initialization03:05
- When Inference Doesn't Work04:37
- Annotations Around Functions04:56
- Inference Around Functions06:08
- Annotations for Anonymous Functions01:42
- Void and Never02:49
- Destructuring with Annotations03:35
- Annotations and Objects07:05
- Arrays in TypeScript05:05
- Why Typed Arrays?04:30
- Multiple Types in Arrays02:57
- When to Use Typed Arrays00:54
- Tuples in TypeScript04:04
- Tuples in Action05:28
- Why Tuples?03:20
- Interfaces01:26
- Long Type Annotations04:42
- Fixing Annotations With Interfaces04:36
- Syntax Around Interfaces03:31
- Functions in Interfaces04:46
- Code Reuse with Interfaces04:15
- General Plan with Interfaces03:12
- Classes03:47
- Basic Inheritance03:03
- Class Method Modifiers06:41
- Fields in Classes06:18
- Fields with Inheritance04:18
- Where to Use Classes01:09
- Updated Parcel Instructions00:13
- App Overview02:45
- Bundling with Parcel04:54
- Project Structure03:19
- IMPORTANT Info About Faker Installation00:23
- Generating Random Data05:29
- Type Definition Files05:17
- Using Type Definition Files06:20
- Export Statements in TypeScript05:06
- Defining a Company04:43
- A Note on API Keys00:24
- Adding Google Maps Support07:38
- Required Update for New @types Library00:18
- Google Maps Integration with TypeScript04:06
- Exploring Type Definition Files12:46
- Hiding Functionality06:28
- Why Use Private Modifiers? Here's Why08:25
- Adding Markers09:18
- Duplicate Code02:45
- One Possible Solution06:38
- Restricting Access with Interfaces05:35
- Implicit Type Checks03:26
- Showing Popup Windows06:47
- Updating Interface Definitions07:11
- Optional Implements Clauses06:06
- App Wrapup08:08
- App Overview01:34
- Configuring the TS Compiler07:40
- Concurrently Compilation and Execution05:05
- A Simple Sorting Algorithm04:47
- Sorter Scaffolding03:10
- Sorting Implementation05:17
- Two Huge Issues07:37
- TypeScript is Really Smart09:34
- Type Guards09:13
- Why Is This Bad?02:22
- Extracting Key Logic07:29
- Seperating Swapping and Comparison13:58
- The Big Reveal04:38
- Interface Definition04:48
- Sorting Abritrary Collections11:08
- Linked List Implementation24:15
- Completed Linked List Code00:01
- Just... One... More... Fix...04:03
- Integrating the Sort Method02:44
- Issues with Inheritance06:54
- Abstract Classes06:25
- Abstract Classes in Action04:30
- Solving All Our Issues with Abstract Classes04:00
- Interfaces vs Abstract Classes03:23
- IMPORTANT - Unfinished Application00:17
- App Overview05:10
- Modules Overview06:37
- Generating Modules04:22
- Module Properties06:45
- Connecting Modules06:59
- Examining the API05:31
- Reading the Users Location04:59
- The Angular Way03:43
- Geolocation in an Observable05:26
- Connecting the Service to a Component03:33
- Transforming Coordinates to Query Params09:02
- SwitchMap vs MergeMap11:26
- But Why SwitchMap?05:33
- Making a Request08:03
- Further Processing04:24
- Generics on HTTP Requests08:56
- Filter, MergeMap, Pluck Operators14:18
- Map and toArray Operators02:48
- Accessing Data in the Template03:57
- Pipes Overview07:08
- Data Pipes04:06
- The Async Pipe06:15
- Adding Bootstrap02:29
- Styling the Forecast Component04:20
- Reminder on the Share Operator04:36
- How to Structure Services10:47
- Generating the Notification Module05:43
- Notifications Service Design04:45
- Introducing Subjects11:03
- Subject Variations08:13
- More Design on Notifications09:07
- Building a Command Structure09:18
- The Scan Operator12:57
- Scan in the Service03:02
- Fixing a Few Errors03:28
- Replay Subject in Action07:18
- A Preferred Solution05:16
- Displaying Messages05:49
- Automatic Timeout03:18
- Notification Styling03:32
- Clearing Messages03:51
- When to Add Notifications04:53
- Showing Success and Errors05:37
- CatchError and ThrowError09:37
- The Retry Operator04:47
- Service Design07:43
- More on API Pagination05:33
- Service Generation04:17
- Subject Declarations04:46
- Building the HTTP Params03:57
- Applying a Type to the Response06:24
- Calculating Pages Available04:43
- Wrapping the Input Subject04:23
- A Better Interface02:59
- The Article List Component03:44
- Accessing the Data04:06
- Rendering Articles03:34
- Fetching a Page01:17
- Fixing Some Styling02:16
- Creating Custom Pipes04:08
- Custom Pipe Arguments04:46
- The Shared Module Pattern02:36
- Connecting the Paginator03:41
- Paginator Strategy03:12
- Paginator Internals07:24
- Styling the Current Page03:09
- Parent to Child Communication03:45
- REMINDER - Unfinished Application00:12
- Bonus!00:29
Online Courses
Learning Angular doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Angular related books and take your
YouTube videos
The number of high-quality and free Angular video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more