Symfony API Platform with React Full Stack Masterclass
Learn how to make a robust REST API in Symfony using API Platform and create a React SPA application
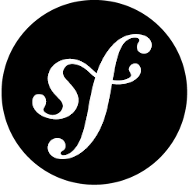
What you will learn?
- You will know how to create a robust APIs in Symfony 4 using API Platform
- You will understand the basics of Symfony Framework
- You will know how to create a pretty complicated ReactJS application that works with API Platform (or any API!)
223 lessons
Easy to follow lectures and videos covering subject details.
19.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction06:45
- Course outline and how to make most of this course! (PLEASE WATCH!)09:28
- Tools (required/optional) overview08:35
- Installing PHP and Composer on Windows05:49
- Install PHP & Composer on MacOS commands00:07
- Installing PHP and Composer on Mac01:51
- Installing Visual Studio Code01:18
- Namespaces04:42
- Class Fields and Methods05:46
- Method & Field Visibility05:13
- Inheritance10:40
- Abstract Classes03:39
- Interfaces11:15
- Typed Arguments & Function Return Types12:28
- Anonymous Functions (Closures)04:48
- Reflection API (Reverse-Engineer Classes, Methods, Functions)05:04
- Dependency Injection (In Practice)05:46
- Simple Service Container (Hands-On Coding!)12:29
- Service Autowiring Implementation Part 110:51
- Service Autowiring Implementation Part 211:24
- Annotations and Kernel Part 108:04
- Annotations and Kernel Part 212:40
- Creating new Symfony project01:24
- Routing annotations in controllers02:46
- Routing - route parameter wildcards05:32
- Routing - default parameter values02:23
- Routing - generating urls using route names01:57
- AbstractController, Request, Response04:38
- Installing ORM, maker, configuring database02:09
- Generating first Entity03:16
- Entity explained02:30
- Migration - modifying database structure02:58
- Persisting entities and serializing data05:14
- Fetching objects using repositories03:21
- ParamConverter - type hinting actions for automatic fetching of entities05:59
- Deleting entities03:39
- Doctrine Fixtures - seeding fake data04:51
- Admin panel introduction - EasyAdmin03:14
- Install API Platform and create the first resource06:19
- Generate User and Comment entity02:18
- ManyToOne relation and migration09:12
- Fixtures with references (for relations)05:33
- Password encoding in fixtures04:01
- Generate fake data in fixtures using Faker07:55
- BlogPost with Comment relation and fixtures04:44
- Built-in API Platform operations05:40
- Disabling operations03:12
- Introduction to serialization/deserialization04:33
- Serialization groups (controlling which properties are serialized)02:15
- EventSubscriber (hashing password)06:50
- Validator and validation constraints04:43
- Validation using regular expressions04:29
- Virtual property (not persisted to database)02:57
- Validating uniqueness of fields (username, email)02:02
- JWT Tokens introduction05:25
- Preparing JWT token library and keys03:24
- Configuring UserProvider04:24
- Firewall configuration08:38
- JSON login configuration and Guard Authentication explained06:10
- Authentication final configuration and first login using JWT token05:42
- Using is_granted() to control access to operations02:55
- BlogPost validation on POST06:16
- Setting author of BlogPost automatically (EventSubscriber)08:15
- Making sure only owner of BlogPost can modify it (PUT)06:07
- Controlling which properties can be changed (no username change)06:44
- Hasing password on User changes (PUT) - with Events07:07
- Comment resource operations (POST/PUT)05:08
- More randomness in fixtures07:22
- Setting author automatically (EventSubscriber) - using generic Interface05:58
- Setting published date automatically (EventSubscriber)07:10
- API subresources05:19
- Controlling how deep relations are serialized07:43
- Embedding Author resource inside BlogPost04:09
- Adding user role field with migration05:47
- User role fixtures09:26
- Defining role hierarchy01:40
- Verifying only users with specific role can POST resources09:43
- Different User view for admins (different serialization of all User entities)09:20
- Verifying only admin can see all User's email03:02
- User can view his full profile (including email and roles) - custom Normalizer11:16
- Verifying only the profile owner can see all properties01:25
- Disabling password hashing for PUT operation05:52
- Configuring custom operation for password reset in User02:20
- Creating custom Action class10:31
- Implementing custom PasswordReset action08:35
- Invalidating JWT tokens after password reset11:17
- User enabled property migration and fixtures05:16
- Implementing UserChecker to verify if account is enabled03:50
- Secure confirmation token generation05:42
- Generating confirmation token when user signs-up04:34
- UserConfirmation custom API Resource03:35
- UserConfirmation EventSubscriber06:38
- Verifying Confirmation Token endpoint02:48
- Install and configure Swift Mailer03:49
- Sending a test e-mail through Gmail02:17
- Refactoring UserConfirmation and Mailer into services08:25
- Traditional (non API) account confirmation route (for e-mail)04:56
- Verifying confirmation link received in e-mail02:05
- Install and configure uploading library04:03
- Image entity with migration and @Uploadable annotation05:12
- API Resource for Image entity02:48
- Implementing custom Action for upload05:50
- Creating Form for file upload05:37
- Assigning Image to BlogPost (ManyToMany relation)03:59
- Verifying assigning Image to BlogPost, embedding Image inside BlogPost04:21
- Configuring default collection sorting order03:33
- Search filter06:39
- Date filter04:31
- Range filter02:05
- Sorting filter04:25
- Filtering by nested properties02:03
- Property filter02:59
- Configuring collection pagination06:06
- Configuring collection pagination part 204:35
- Partial pagination (performance gain)01:45
- Empty request body for POST/PUT requests problem02:57
- Handling empty request body06:24
- Fixing validation groups01:56
- Handling business logic exceptions03:50
- Installing Monolog library for application logging07:55
- Defining custom logging channel04:31
- Logging to separate files per channel02:41
- Production configuration for logger explained03:38
- EasyAdmin basic built-in features07:01
- Fixing empty body subscriber for form submittal04:17
- Custom Resource controller, customizing saving and updating an Entity08:20
- Image upload in admin panel03:57
- Custom page for individual BlogPost05:26
- Securing Admin panel area06:06
- Installing PHPUnit and writing first basic unit test07:05
- Testing EventSubscriber static configuration04:26
- Mocks (stubs) Part 1 - Mocking dependencies, Entities05:31
- Mocks (stubs) Part 2 - Mocking dependencies, Entites05:09
- Event class Mock, extracting repeated mock factory code03:27
- Parameters for mocks04:49
- Full test for AuthoredEntitySubscriber06:37
- Data providers in tests (using many data sets in 1 test case)04:42
- Finding potential errors using unit tests (null Token example)05:45
- Installing Behat & all extensions, first suite run02:59
- Configuring Behat04:51
- FeatureContext - creating database schema for each feature08:25
- First blog post test scenario05:49
- Testing protected URLs07:04
- Validating returned JSON using patterns (not matching exact values)06:30
- Looking for errors and issues using functional tests03:10
- Finding a comment problem06:27
- Custom error listener13:05
- Image upload feature06:20
- Working image upload functional test02:40
- Testing assigning Images to BlogPost, full suite run04:49
- Installing node & npm on Windows01:18
- Installing node & npm on MacOS01:00
- Before you start coding a React app!00:11
- Installing dependencies and creating main index.js03:05
- Creating store, using Provider, Router and components05:44
- BlogPostList empty component, App as a container component02:40
- BlogPostContainer, using key for list of elements06:18
- Redux: actions, reducer, mapping state and dispatch to props 107:01
- Redux: actions, reducer, mapping state and dispatch to props 204:35
- Adding BlogPost (dummy action), Reducer explained04:28
- Making API requests with Superagent04:59
- Thunk Redux Middleware09:18
- Header component (navigation)03:30
- Loading indicator when making API requests03:41
- Formatting BlogPost list, formatting time (timeago.js)03:31
- Route with parameter - individual BlogPost04:06
- Fetching BlogPost from API06:27
- ComponentWillUnmount lifecycle method04:41
- BlogPost component - API changes02:43
- Spinner and Message components (loading state, simple message)05:35
- CommentListContainer component10:30
- CommentList reducer03:44
- Rendering comments02:58
- Introducing redux-form02:23
- Render redux form component06:26
- Adding redux-form reducer02:09
- Submitting Form to an API endpoint03:56
- Storing JWT token in LocalStorage, Token Middleware03:14
- Token Middleware and JWT authentication plugin 107:10
- Token Middleware and JWT authentication plugin 206:05
- Reading token in App component constructor06:29
- Redux Form submission, handling login errors03:13
- Checking whether user is authenticated (in Header component)03:21
- Fetching user profile through API06:19
- Rendering signed-in username in Header07:42
- Fetching user profile on page refresh05:35
- Create a redux-form for Comment posting04:40
- Redux-form submitting state simulation02:26
- Fully working CommentForm04:20
- Displaying errors in redux-form03:41
- Parsing API errors (validation constraint messages from API)04:11
- Animations - animated comment adding05:46
- Logout functionality (resetting stored JWT token)06:19
- Logging out user when token expires05:56
- Logging out user when he submits an expired token (401)03:02
- Paginator component03:46
- Paginator component - showing current page02:46
- Fetching blog post collection on page change07:09
- Query parameter based pagination (page in route parameter)04:33
- Pagination - previous/next buttons09:26
- Modify API - missing User embedded data on new Comment01:47
- LoadMore component - fetching next comments (different way of pagination)08:46
- Append new comments inside reducer02:48
- Registration form05:31
- Registration action creators and form validation10:21
- ConfirmationToken - modify e-mail to contain token, not only link03:14
- RegistrationContainer - 2 step registration process03:51
- Showing ConfirmationToken form on successful account creation05:20
- Keeping state of registration/confirmation process05:16
- Registration/Confirmation process, with redirect timer08:16
- BlogPost form for creating posts and user permissions05:19
- Functional BlogPost form05:13
- ImageUpload component (styling the file input)06:31
- Uploading images - upload request09:49
- ImageBrowser (preview uploaded images)05:04
- A new reducer for BlogPostForm04:05
- Clear the images when BlogPostForm unmounts07:16
- DELETE operation on Image (API Changes)04:10
- ImageBrowser animations03:37
- Remove button on ImageBrowser03:57
- Remove button on ImageBrowser sending DELETE request03:15
- Locking all buttons during Image upload/removal04:46
- Bonus!00:04
Online Courses
Learning Symfony doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Symfony related books and take your
YouTube videos
The number of high-quality and free Symfony video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more