Learn PHP Symfony Hands-On Creating Real World Application
Learn PHP Symfony Framework, write a full real world application and deploy it on DigitalOcean using CI/CD.
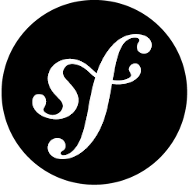
What you will learn?
- You will create a fully working, real world website in Symfony
- You will have strong understanding of the framework
- Learn and see how Continuous Deployment and Continuous Integration works
- You will automate deployment using single commit to deploy the website to outside world
- Deep understanding of how databases and Doctrine ORM works
- Learn how to create forms, save, edit and validate data
- Learn how to create and deal with complicated Database relations
- Send e-mails and understand Symfony Event system
- Secure the application and restrict access to certain parts of application to specific users
- Create SQL queries in Doctrine, Doctrine Table Inheritance and Lifycycle callbacks
- Learn how to translate your application into many different languages
- Understand how sessions work
- Test your application by writing Unit tests with PHPUnit
- Provision a remote server by installing Apache, PHP, MySQL using SSH
115 lessons
Easy to follow lectures and videos covering subject details.
13.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction05:49
- About Symfony02:34
- Tools overview02:10
- Full source code00:03
- Setting up Vagrant on Windows06:09
- Setting up Vagrant on Ubuntu03:21
- Setting up Vagrant on MacOS04:08
- IMPORTANT! Vagrant problems and FAQ00:59
- Running Vagrant and creating a new Symfony 4 project05:04
- IDE/Editor and tools overview and recommendations00:39
- Symfony project structure overview06:22
- About Service Container06:10
- Autowiring, Autoconfiguring services04:19
- Public, private services, service aliases05:14
- Service tags04:54
- Manual service wiring, parameter binding06:01
- About controllers12:33
- About routing08:53
- Introduction to views (Twig)10:39
- Twig filters & custom Twig extensions07:52
- Custom error pages using Twig05:59
- Global variables in Twig05:15
- Handling assets (JavaScript, CSS) using Symfony Encore (and Webpack)08:14
- Installing Bootstrap 4 and compiling assets using Encore (Webpack)08:35
- Doctrine introduction11:51
- Creating first Entity07:33
- Creating a database migration07:57
- Basics of doctrine repository10:31
- Creating fixtures (database seeding)06:24
- Creating a form and handling form submission10:45
- Repository find methods and Twig include10:42
- Repository findBy, form validation, handling Entity changes09:09
- Deleting an Entity and flash messages05:46
- Doctrine internals (Entity, EntityManager, Unit of Work, Identity Map)06:02
- Security concepts04:42
- Security component config and HTTP Basic login09:46
- Creating User entity08:50
- User Entity fixtures09:43
- Login form part 109:41
- Login form part 210:53
- Adding validation to User entity06:27
- User registration form08:19
- User registration controller11:48
- Assigning user to MicroPost (ManyToOne & OneToMany)13:24
- Fixtures for relations (using references in fixtures)04:38
- Verifying user permissions in Twig (is_granted)11:23
- Adding randomness to fixtures05:19
- Security Voters introduction03:18
- Restricting access to edit or delete a micro post using SecurityVoter11:10
- Securing controller actions and templates using Security Voter11:47
- Adding an Admin User with all permissions12:06
- Security role hierarchy02:27
- Restricting adding new posts to registered users (many ways of doing that)06:06
- Lazy loading, proxy classes, repository find* methods criteria09:44
- Doctrine Lifecycle Callbacks04:24
- ManyToMany self-referencing relation (following/followers)09:02
- Fetching collections lazily in templates07:30
- Follow/Unfollow functionality (controller, adding Entities to Collections)16:58
- Follow/Unfollow functionality (security, verifying uniqueness)05:40
- Doctrine QueryBuilder - creating queries in OOP manner11:53
- ArrayCollection vs PersistentCollection vs Collection09:43
- Creating custom query to fetch users with more than 5 posts12:43
- More on Doctrine Collections (from the database perspective)02:47
- Section introduction00:53
- ManyToMany relationship for liked posts07:29
- Likes controller09:32
- Implementing like functionality through XHR requests (including JavaScript)18:54
- Notifications introduction00:51
- Doctrine Table Inheritance - base Notification Entity10:10
- NotificationRepository - fetching notifications unseen by user06:40
- Unseen notification badge (Twig + JavaScript)09:40
- Doctrine EventSubscriber - saving a notification as a reaction to other events17:44
- Unseen notification list11:04
- Marking notifications as being read (Doctrine Query Builder UPDATE queries)10:11
- Introduction to EventDispatcher03:08
- Dispatching an event when user registers05:30
- EventSubscriber - listening to user register event04:13
- UPDATE: Missing code on next lecture00:22
- Sending e-mail after user registers using Swift Mailer09:44
- E-mail spooling05:07
- Creating a Mailer class to handle e-mail sending (and generation using Twig)05:15
- Account confirmation (using secure token, confirmation link on e-mail)15:02
- Installing and configuring translation component04:35
- Using trans Twig filter to translate strings and validation messages translation07:14
- Translation strings with variables (translating confirmation e-mail with links)10:16
- Translation pluralization (different translation depending on variable)05:14
- Introduction to sessions03:18
- Storing user sessions in database10:00
- Change: Event Changes in Symfony Version >= 4.300:09
- LocaleSubscriber - keeping user locale (language) in session09:54
- UserPreferences Entity & OneToOne relation with User Entity09:12
- Creating new UserPreferences after user registration (responding to event)06:32
- UserLocaleSubscriber - loading user language from DB and keeping in session06:17
- Introduction to unit testing (using PHPUnit)01:53
- Writing first unit test08:07
- Creating PHPUnit Mocks06:15
- A more complicated case with testing09:55
- Creating a GitHub account and adding an SSH key05:42
- DigitalOcean Coupon Code00:13
- Creating a DigitalOcean droplet01:42
- First login to virtual server and changing the root password01:33
- Creating and adding an SSH key to DigitalOcean (and managing SSH keys)04:43
- Installing Apache2 on virtual server04:42
- Installing PHP 7.2 on virtual server02:18
- Installing MySQL on virtual server and creating a database02:56
- Configuring Apache Virtual Host and using fake SMTP testing server08:15
- Moving application code to production server using Git & dependencies06:07
- Running migrations on server and folder permissions07:33
- Introduction to Continuous Integration & Continuous Deployment03:01
- Setting up CircleCI (automation server)10:17
- Running our first automation job (running tests)03:57
- Adding deployment SSH key to CricleCI01:12
- Deployment job and deployment scripts12:27
- Running the deployment job05:18
- Bonus!00:04
Online Courses
Learning Symfony doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Symfony related books and take your
YouTube videos
The number of high-quality and free Symfony video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more