The Rust Programming Language
Learn a modern, powerful yet safe systems programming language!
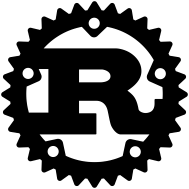
What you will learn?
- Solve problems in Rust
- Understand Rust's strengths and weaknesses
- Effectively leverage Rust's memory safety guarantees
- Write applications and libraries
- Test and document your code
Your trainer

Dmitri Nesteruk
Dmitri is a quant, developer, book author and course author. His interests lie in software development and integration practices in the areas of computation, quantitative finance and algorithmic trading. His technological interests include C# and C++ programming as well high-performance computing using technologies such as CUDA and FPGAs. He has been a C# MVP since 2009.
61 lessons
Easy to follow lectures and videos covering subject details.
8.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Course Introduction03:32
- Installing and Configuring Rust02:40
- Hello, Rust!03:31
- Introducing the Cargo Package Manager07:59
- Rust in IntelliJ IDEA06:53
- Numbers on the Computer25:59
- Application Entrypoint03:01
- Core Data Types21:24
- Operators10:25
- Scope and Shadowing06:13
- Declaring and Using Constants04:32
- Stack and Heap12:24
- Debugging Rust Applications with CLion07:40
- If Statement07:20
- While and Loop04:08
- For Loops04:47
- Match Statement05:25
- Combination Lock09:13
- Structs03:40
- Enumerations08:59
- Unions08:42
- Option<T> and if let/while let05:25
- Arrays13:03
- Slices05:26
- Tuples09:31
- Pattern Matching11:50
- Generics05:54
- Overview03:47
- Vec(tor)13:09
- HashMap07:26
- HashSet11:46
- Iterators09:53
- Strings11:00
- String Formatting (format!)07:43
- Number Guessing Game08:07
- Functions and Function Arguments06:38
- Methods02:58
- Closures10:48
- Higher-Order Functions09:55
- Traits12:41
- Trait Parameters06:50
- Into05:59
- Drop05:59
- Operator Overloading16:49
- Static Dispatch06:33
- Dynamic Dispatch03:07
- Why Dynamic Dispatch?05:03
- Vectors of Different Objects09:29
- Ownership08:31
- Borrowing07:12
- Lifetime07:50
- Lifetime in Structure Implementation04:41
- Reference-Counted Variables (Rc)06:27
- Atomic Reference-Counted Variables (Arc)03:57
- Using a Mutex for Thread-Safe Mutability07:05
- Circular References25:12
- Spawning and Joining Threads04:47
- Consuming Crates05:04
- Building Modules and Crates11:29
- Testing04:42
- Comments and Documentation06:03
Online Courses
Learning Rust doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Rust related books and take your
YouTube videos
The number of high-quality and free Rust video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more