Learn Rust by Building Real Applications
Fundamentals of the Rust Programming Language
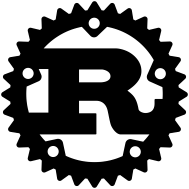
What you will learn?
- The fundamentals of the Rust Programming Language
- Low level memory management
- Rust’s unique approach to memory safety
- How to troubleshoot common compiler errors
Your trainer

Lyubomir Gavadinov
I'm a senior software engineer with over 7 years of experience working for a variety of companies.
For most of my career I was at Amazon and AWS where I got to work on various projects, from designing and building high scale distributed systems to optimising the performance of low level streaming protocols.
I have written plenty of C, Java and JavaScript in my days, but when I discovered Rust a couple of years ago I immediately fell in love with it.
61 lessons
Easy to follow lectures and videos covering subject details.
6.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Course Introduction02:05
- What is Rust03:38
- Installing Rust01:33
- Setting Up the Development Environment01:35
- Cargo06:37
- Code for this section00:02
- Introduction00:24
- The Stack03:42
- The Heap04:40
- Smart Pointers01:53
- Explore the Memory Layout in GDB04:53
- Memory Management4 questions
- Code for this section00:02
- Introduction00:48
- Basic Data Types02:29
- Functions06:30
- Macros05:43
- Mutability03:22
- The Standard Library05:14
- Ownership08:36
- References and Borrowing07:44
- Explore the Ownership and Borrowing in GDB04:43
- Finishing Touches07:08
- Rust Basics5 questions
- Code for this section00:02
- Introduction00:44
- The HTTP Protocol and the Architecture of Our Server04:15
- Structs10:50
- Strings17:11
- Enums11:15
- The Option Enum04:09
- Organising Our Code into Modules14:26
- Listening for TCP Connections02:39
- The Result Enum07:28
- Loops02:34
- Tuples03:20
- The Match Expression10:21
- Arrays10:07
- Logging the Incoming Requests to the Console06:32
- Traits and Type Conversions18:36
- Custom Errors13:18
- Advanced Error Handling08:42
- Iterating Over Strings09:51
- Converting an Option into a Result06:38
- Parsing Values From Strings07:13
- The "If Let" Expression07:14
- Lifetimes - Part 107:44
- Lifetimes - Part 219:29
- Lifetimes3 questions
- Silencing Compiler Warnings01:38
- Representing the Query String Using a Hash Map - Part 108:10
- Representing the Query String Using a Hash Map - Part 217:12
- The Derive Attribute04:48
- Modelling the HTTP Response03:41
- Copy and Clone Types11:00
- Writing Data to a TCP Stream13:24
- Dynamic vs Static Dispatch06:53
- Custom Traits06:15
- Implementing Getters02:59
- Routing Incoming Requests03:00
- Working with Environment Variables06:55
- Serving HTML Files03:49
- Serving Arbitrary Files Securely10:47
- Final Quiz6 questions
- Next Steps02:38
Online Courses
Learning Rust doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Rust related books and take your
YouTube videos
The number of high-quality and free Rust video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more