REST APIs with Flask and Python in 2022
Build professional REST APIs with Python, Flask, Docker, Flask-Smorest, and Flask-SQLAlchemy
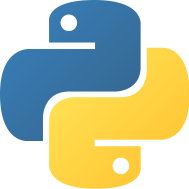
What you will learn?
- Connect web or mobile applications to databases and servers via REST APIs
- Create secure and reliable REST APIs which include authentication, deployments, and database migrations
- Understand the different layers of a web server and how web applications interact with each other
- Handle seamless user authentication with advanced features like token refresh
- Handle log-outs and prevent abuse in your REST APIs with JWT blacklisting
- Develop professional-grade REST APIs with expert instruction
- Optimize performance of your REST APIs using task queues and background workers
Your trainer

Jose Salvatierra
Hi, I'm Jose! I love helping students learn to code and master software development. I've been teaching online for over 7 years, and I founded Teclado to bring software development to everyone—my objective is for you to truly understand everything that goes on behind the scenes.
Coding is extremely rewarding. As you learn, things start to click and make sense. You can join the dots of all the things that weren't quite clear before. I'm here to make that journey quick and painless!
I can help you with Python and JavaScript issues, particularly in web and backend development. I'm experienced with programming libraries and frameworks like Flask, React, React Native, and AngularJS. I've worked extensively with UNIX systems, MongoDB, PostgreSQL, and advanced system architecture design.
122 lessons
Easy to follow lectures and videos covering subject details.
12 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- How to take this course01:20
- Python on Windows00:56
- Python on Mac00:20
- Introduction to this section01:01
- Access the code for this section here00:24
- Variables in Python08:26
- Variables (Python 3.10)1 question
- Solution to coding exercise: Variables02:00
- String formatting in Python06:26
- Getting user input05:16
- Writing our first Python app03:19
- Lists, tuples, and sets06:31
- Advanced set operations04:39
- Lists, tuples, and set (Python 3.10)1 question
- Solution to coding exercise: Lists, tuples, sets04:40
- Booleans in Python04:59
- If statements08:17
- The 'in' keyword in Python02:02
- If statements with the 'in' keyword08:18
- Loops in Python11:07
- Flow control—loops and ifs (Python 3.10)1 question
- Solution to coding exercise: Flow control03:08
- List comprehensions in Python07:24
- Dictionaries08:31
- Destructuring variables08:28
- Functions in Python10:41
- Function arguments and parameters07:40
- Default parameter values03:54
- Functions returning values07:19
- Functions (Python 3.10)1 question
- Solution to coding exercise: Functions02:30
- Lambda functions in Python07:52
- Dictionary comprehensions04:01
- Dictionaries and students (Python 3.10)1 question
- Solution to coding exercise: Dictionaries06:16
- Unpacking arguments10:24
- Unpacking keyword arguments08:44
- Object-Oriented Programming in Python15:52
- Magic methods: __str__ and __repr__06:25
- Classes and objects (Python 3.10)1 question
- Solution to coding exercise: Classes and objects05:04
- @classmethod and @staticmethod14:03
- @classmethod and @staticmethod (Python 3.10)1 question
- Solution to coding exercise: @classmethod and @staticmethod05:54
- Class inheritance08:32
- Class composition06:08
- Type hinting in Python 3.5+05:08
- Imports in Python09:33
- Relative imports in Python08:52
- Errors in Python12:47
- Custom error classes05:04
- First-class functions07:52
- Simple decorators in Python07:12
- The 'at' syntax for decorators03:33
- Decorating functions with parameters02:24
- Decorators with parameters04:50
- Mutability in Python06:03
- Mutable default parameters (and why they're a bad idea)04:27
- Access the course e-book here00:17
- Overview of the project we'll build04:00
- Initial set-up for a Flask app04:36
- Your first REST API endpoint04:05
- What is JSON?03:43
- How to interact with and test your REST API04:28
- How to create stores in our REST API05:37
- How to create items in each store07:55
- How to get a specific store and its items04:39
- What are Docker containers and images?13:28
- How to run a Flask app in a Docker container11:15
- In-depth Docker tutorial notes00:11
- Data model improvements for our API15:40
- General improvements to our first REST API07:07
- New endpoints for our first REST API09:05
- How to run the API in Docker with automatic reloading and debug mode06:26
- How to use Blueprints and MethodViews in Flask10:25
- How to write marshmallow schemas for our API04:01
- How to perform data validation with marshmallow04:31
- Decorating responses with Flask-Smorest04:39
- Overview and why use SQLAlchemy02:07
- How to code a simple SQLAlchemy model04:45
- How to write one-to-many relationships using SQLAlchemy09:41
- How to configure Flask-SQLAlchemy with your Flask app07:54
- How to insert data into a table using SQLAlchemy07:21
- How to find models in the database by ID or return a 40403:58
- How to update models with SQLAlchemy05:10
- How to retrieve list of all models00:49
- How to delete models with SQLAlchemy01:11
- Conclusion of this section03:53
- Changes in this section02:47
- One-to-many relationship between stores and tags10:53
- Many-to-many relationship between items and tags11:23
- Changes in this section00:06
- What is a JWT?00:11
- Who uses the JWT?10:15
- How to set up Flask-JWT-Extended with our app04:16
- Coding the User model and schema02:07
- How to add a register endpoint to the REST API09:35
- How to add a login endpoint to the REST API08:38
- Protect endpoints by requiring a JWT06:00
- JWT claims and authorization05:55
- How to add logout to the REST API06:18
- Request chaining with Insomnia04:32
- Token refreshing with Flask-JWT-Extended09:30
- Why use database migrations at all?00:05
- How to add Flask-Migrate to our Flask app01:38
- Initialize your database with Flask-Migrate05:42
- Change SQLAlchemy models and generate a migration05:01
- Manually review and modify database migrations03:19
- What are Git repositories and commits?09:43
- Initialize a Git repository for our project08:27
- Writing Markdown for documents and commits02:36
- Remote repositories and how to use them05:27
- Git branches and merging06:17
- Merge conflicts and how to resolve them05:30
- Overview of the final e-book chapters01:38
- Overview of this section00:06
- Creating a Render.com web service05:54
- How to run Flask with gunicorn in Docker06:13
- Get a deployed PostgreSQL database01:51
- Use PostgreSQL locally and in production17:45
- Test the finished production app01:38
- How to send emails with Python and Mailgun04:46
- How to send emails when users register09:37
- What is a task queue and setting up a Redis database05:05
- How to Populate and consume the task queue with rq06:26
- How to process background tasks with the rq worker05:24
- How to send HTML emails using Mailgun and Python10:17
- How to deploy a background worker to render.com05:39
- Bonus lecture: other courses and next steps01:12
Online Courses
Learning Python doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Python related books and take your
YouTube videos
The number of high-quality and free Python video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more