React - The Complete Guide (incl Hooks, React Router, Redux)
Dive in and learn React.js from scratch! Learn Reactjs, Hooks, Redux, React Routing, Animations, Next.js and way more!
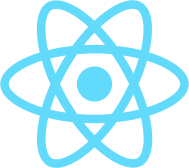
What you will learn?
- Build powerful, fast, user-friendly and reactive web apps
- Provide amazing user experiences by leveraging the power of JavaScript with ease
- Apply for high-paid jobs or work as a freelancer in one the most-demanded sectors you can find in web dev right now
- Learn all about React Hooks and React Components
Your trainer

Maximilian Schwarzmüller
Starting out at the age of 13 I never stopped learning new programming skills and languages. Early I started creating websites for friends and just for fun as well. Besides web development I also explored Python and other non-web-only languages. This passion has since lasted and lead to my decision of working as a freelance web developer and consultant. The success and fun I have in this job is immense and really keeps that passion burningly alive.
Starting web development on the backend (PHP with Laravel, NodeJS, Python) I also became more and more of a frontend developer using modern frameworks like React, Angular or VueJS 2 in a lot of projects. I love both worlds nowadays!
495 lessons
Easy to follow lectures and videos covering subject details.
49 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome To The Course!01:04
- What is React.js?04:37
- Why React Instead Of "Just JavaScript"?07:25
- Building Single-Page Applications (SPAs) with React01:33
- Exploring React.js Alternatives (Angular / Vue)03:33
- Join our Online Learning Community00:25
- About This Course & Course Outline08:27
- The Two Ways (Paths) Of Taking This Course04:22
- Getting The Most Out Of This Course04:16
- Setting Up The Course Dev Environment (Code Editor)04:55
- Module Introduction01:35
- Understanding "let" and "const"03:05
- Arrow Functions05:27
- Exports and Imports04:43
- Understanding Classes04:37
- Classes, Properties and Methods03:03
- The Spread & Rest Operator06:30
- Destructuring03:13
- Reference and Primitive Types Refresher04:26
- Refreshing Array Functions02:45
- Wrap Up00:52
- Next-Gen JavaScript - Summary03:51
- JS Array Functions00:20
- Module Introduction03:44
- What Are Components? And Why Is React All About Them?07:10
- React Code Is Written In A "Declarative Way"!03:42
- Creating a new React Project12:23
- The Starting Project00:07
- Analyzing a Standard React Project13:03
- Introducing JSX03:58
- How React Works04:22
- Building a First Custom Component09:00
- Writing More Complex JSX Code05:05
- Adding Basic CSS Styling04:47
- Outputting Dynamic Data & Working with Expressions in JSX08:20
- Passing Data via "props"12:38
- Adding "normal" JavaScript Logic to Components05:57
- Splitting Components Into Multiple Components10:46
- Time to Practice: React & Component Basics1 question
- The Concept of "Composition" ("children props")13:07
- A First Summary04:06
- A Closer Look At JSX09:09
- Organizing Component Files03:05
- An Alternative Function Syntax02:39
- Learning Check: React Basics, Components, Props & JSX10 questions
- Module Resources00:19
- Module Introduction02:27
- Listening to Events & Working with Event Handlers09:39
- How Component Functions Are Executed05:44
- Working with "State"10:53
- A Closer Look at the "useState" Hook07:36
- State can be updated in many ways!00:18
- Adding Form Inputs09:58
- Listening to User Input05:17
- Working with Multiple States06:44
- Using One State Instead (And What's Better)04:51
- Updating State That Depends On The Previous State04:37
- Handling Form Submission04:54
- Adding Two-Way Binding02:57
- Child-to-Parent Component Communication (Bottom-up)13:56
- Lifting The State Up07:00
- Time to Practice: Working with Events & State1 question
- Controlled vs Uncontrolled Components & Stateless vs Stateful Components05:22
- Learning Check: Working with Events & State9 questions
- Module Resources00:19
- Module Introduction00:45
- Rendering Lists of Data07:07
- Using Stateful Lists04:30
- Understanding "Keys"06:59
- Time to Practice: Working with Lists1 question
- Outputting Conditional Content06:47
- Adding Conditional Return Statements05:21
- Time to Practice: Conditional Content1 question
- Demo App: Adding a Chart07:25
- Adding Dynamic Styles07:28
- Wrap Up & Next Steps11:04
- Fixing a Small Bug00:14
- Learning Check: Outputting Lists & Conditional Content3 questions
- Module Resources00:19
- Module Introduction03:43
- Setting Dynamic Inline Styles08:59
- Setting CSS Classes Dynamically05:00
- Introducing Styled Components09:46
- Styled Components & Dynamic Props08:31
- Styled Components & Media Queries02:27
- Using CSS Modules06:58
- Dynamic Styles with CSS Modules05:31
- Module Resources00:19
- Module Introduction01:34
- Understanding React Error Messages06:19
- Analyzing Code Flow & Warnings05:53
- Working with Breakpoints06:03
- Using the React DevTools05:59
- Module Resources00:19
- Module Introduction02:56
- Adding a "User" Component07:06
- Adding a re-usable "Card" Component08:37
- Adding a re-usable "Button" Component03:50
- Managing the User Input State04:57
- Adding Validation & Resetting Logic04:34
- Adding a Users List Component10:45
- Managing a List Of Users via State09:27
- Adding The "ErrorModal" Component08:04
- Managing the Error State08:57
- Module Resources00:19
- Module Introduction00:49
- JSX Limitations & Workarounds08:46
- Creating a Wrapper Component04:14
- React Fragments02:42
- Introducing React Portals04:20
- Working with Portals12:24
- Working with "ref"s11:47
- Controlled vs Uncontrolled Components03:04
- Module Resources00:19
- Module Introduction01:35
- What are "Side Effects" & Introducing useEffect06:52
- Using the useEffect() Hook10:58
- useEffect & Dependencies09:01
- What to add & Not to add as Dependencies01:46
- Using the useEffect Cleanup Function08:55
- useEffect Summary03:38
- Introducing useReducer & Reducers In General09:17
- Using the useReducer() Hook14:12
- useReducer & useEffect09:52
- Adding Nested Properties As Dependencies To useEffect00:36
- useReducer vs useState for State Management03:35
- Introducing React Context (Context API)07:22
- Using the React Context API10:44
- Tapping Into Context with the useContext Hook01:42
- Making Context Dynamic04:35
- Building & Using a Custom Context Provider Component09:07
- React Context Limitations02:45
- Learning the "Rules of Hooks"07:10
- Refactoring an Input Component06:06
- Diving into "Forward Refs"13:39
- Module Resources00:19
- Module Introduction02:49
- Starting Setup04:10
- Adding a "Header" Component09:07
- Adding the "Cart" Button Component05:26
- Adding a "Meals" Component09:08
- Adding Individual Meal Items & Displaying Them09:30
- Adding a Form10:09
- Fixing Form Input IDs01:11
- Working on the "Shopping Cart" Component05:10
- Adding a Modal via a React Portal07:42
- Managing Cart & Modal State10:48
- Adding a Cart Context07:43
- Using the Context04:52
- Adding a Cart Reducer10:45
- Working with Refs & Forward Refs10:49
- Outputting Cart Items07:23
- Working on a More Complex Reducer Logic05:29
- Making Items Removable08:18
- Using the useEffect Hook07:44
- Module Resources00:19
- Module Introduction02:16
- How React Really Works07:22
- Component Updates In Action06:51
- A Closer Look At Child Component Re-Evaluation10:41
- Preventing Unnecessary Re-Evaluations with React.memo()11:36
- Preventing Function Re-Creation with useCallback()04:13
- useCallback() and its Dependencies07:10
- A First Summary04:01
- A Closer Look At State & Components03:30
- Understanding State Scheduling & Batching09:05
- Optimizing with useMemo()09:01
- Module Resources00:19
- Module Introduction02:10
- What & Why04:53
- Adding a First Class-based Component06:54
- Working with State & Events11:38
- The Component Lifecycle (Class-based Components Only!)05:20
- Lifecycle Methods In Action11:46
- Class-based Components & Context04:53
- Class-based vs Functional Components: A Summary02:42
- Introducing Error Boundaries09:52
- Module Resources00:19
- Module Introduction01:46
- How To (Not) Connect To A Database03:32
- Using the Star Wars API00:14
- Our Starting App & Backend03:52
- Sending a GET Request10:35
- Using async / await02:01
- Handling Loading & Data States04:45
- Handling Http Errors11:13
- Using useEffect() For Requests06:27
- Preparing The Project For The Next Steps06:16
- Sending a POST Request09:16
- Wrap Up01:16
- Module Resources00:19
- Module Introduction01:25
- What are "Custom Hooks"?01:44
- Creating a Custom React Hook Function06:39
- Using Custom Hooks04:45
- Configuring Custom Hooks05:51
- Onwards To A More Realistic Example06:45
- Building a Custom Http Hook09:16
- Using the Custom Http Hook07:45
- Adjusting the Custom Hook Logic08:45
- Using The Custom Hook In More Components08:53
- Module Resources00:19
- Module Introduction01:36
- Our Starting Setup01:41
- What's So Complex About Forms?05:08
- Dealing With Form Submission & Getting User Input Values10:04
- Adding Basic Validation03:57
- Providing Validation Feedback03:54
- Handling the "was touched" State05:40
- React To Lost Focus04:15
- Refactoring & Deriving States09:07
- Managing The Overall Form Validity07:30
- Time to Practice: Forms1 question
- Adding A Custom Input Hook12:44
- Re-Using The Custom Hook02:54
- A Challenge For You!01:41
- Applying Our Hook & Knowledge To A New Form11:05
- Summary03:17
- Bonus: Using useReducer()08:22
- Module Resources00:19
- Module Introduction03:21
- Moving "Meals" Data To The Backend04:05
- Fetching Meals via Http09:27
- Handling the Loading State04:00
- Handling Errors07:44
- Adding A Checkout Form10:47
- Reading Form Values04:51
- Adding Form Validation12:04
- Submitting & Sending Cart Data06:48
- Adding Better User Feedback09:28
- Summary01:31
- Module Resources00:19
- Module Introduction01:05
- Another Look At State In React Apps05:14
- Redux vs React Context06:19
- How Redux Works05:48
- Exploring The Core Redux Concepts15:14
- More Redux Basics03:04
- Preparing a new Project01:59
- Creating a Redux Store for React04:54
- Providing the Store03:13
- Using Redux Data in React Components05:08
- Dispatching Actions From Inside Components03:33
- Redux with Class-based Components10:20
- Attaching Payloads to Actions04:15
- Working with Multiple State Properties06:19
- How To Work With Redux State Correctly05:07
- Redux Challenges & Introducing Redux Toolkit05:27
- Adding State Slices08:11
- Connecting Redux Toolkit State04:47
- Migrating Everything To Redux Toolkit06:19
- Working with Multiple Slices11:50
- Reading & Dispatching From A New Slice06:56
- Splitting Our Code05:03
- Summary03:53
- Module Resources00:19
- Module Introduction00:39
- Redux & Side Effects (and Asynchronous Code)03:27
- Refresher / Practice: Part 1/220:12
- Refresher / Practice: Part 2/218:00
- Redux & Async Code04:28
- Frontend Code vs Backend Code05:40
- Where To Put Our Logic08:59
- Using useEffect with Redux06:00
- A Problem with useEffect()00:14
- Handling Http States & Feedback with Redux12:49
- Using an Action Creator Thunk12:07
- Getting Started with Fetching Data08:39
- Finalizing the Fetching Logic05:16
- Exploring the Redux DevTools05:37
- Summary01:53
- Module Resources00:19
- Module Introduction02:53
- What is Routing & Why?05:06
- Installing React Router02:42
- Defining & Using Routes10:32
- Working with Links06:53
- Using NavLinks03:25
- Adding Dynamic Routes with Params06:20
- Extracting Route Params02:26
- Using "Switch" and "exact" For Configuring Routes06:54
- Working with Nested Routes03:52
- Redirecting The User02:25
- Time to Practice: Onwards to a New Project11:00
- Practice Redirecting & Extracting Params03:57
- Practicing Nested Routes02:56
- Adding a Layout Wrapper Component06:45
- Adding Dummy Data & More Content05:16
- Outputting Data on the "Details" Page06:08
- Adding a "Not Found" Page03:37
- Implementing Programmatic (Imperative) Navigation04:15
- Preventing Possibly Unwanted Route Transitions with the "Prompt" Component07:27
- Working with Query Parameters13:41
- Getting Creative With Nested Routes04:17
- Writing More Flexible Routing Code08:43
- Sending & Getting Quote Data via Http17:33
- Adding the "Comments" Features18:40
- Upgrading To React Router v629:57
- Better Data Fetching with React Router 6.444:30
- Module Resources00:19
- Module Introduction01:39
- Deployment Steps03:35
- Adding Lazy Loading13:27
- Building The Code For Production03:41
- Getting Started With Deployment (Uploading Files)07:49
- Exploring Routing Issues & Finishing Deployment07:49
- Module Resources00:19
- Module Introduction01:39
- What, How & Why?12:11
- More on "Authentication Tokens"00:15
- Starting Setup & First Steps04:45
- Adding User Signup15:32
- Showing Feedback To The User05:36
- Adding User Login06:36
- Managing The Auth State With Context11:49
- Using The Token For Requests To Protected Resources11:08
- Redirecting The User02:59
- Adding Logout02:42
- Protecting Frontend Pages07:01
- Persisting The User Authentication Status07:06
- Adding Auto-Logout07:57
- Finishing Steps11:10
- Module Resources00:19
- Module Introduction02:08
- What is NextJS?04:45
- Key Feature 1: Built-in Server-side Rendering (Improved SEO!)06:37
- Key Feature 2: Simplified Routing with File-based Routing03:13
- Key Feature 3: Build Fullstack Apps01:50
- Creating a New Next.js Project & App05:39
- Analyzing the Created Project02:52
- Adding First Pages06:05
- Adding Nested Paths & Pages (Nested Routes)03:47
- Creating Dynamic Pages (with Parameters)03:36
- Extracting Dynamic Parameter Values04:07
- Linking Between Pages07:13
- Onwards to a bigger Project!03:32
- Preparing the Project Pages03:42
- Outputting a List of Meetups05:03
- Adding the New Meetup Form03:54
- The "_app.js" File & Layout Wrapper06:17
- Using Programmatic (Imperative) Navigation03:47
- Adding Custom Components & CSS Modules10:00
- How Pre-rendering Works & Which Problem We Face05:52
- Data Fetching for Static Pages08:56
- More on Static Site Generation (SSG)05:44
- Exploring Server-side Rendering (SSR) with "getServerSideProps"06:27
- Working with Params for SSG Data Fetching05:14
- Preparing Paths with "getStaticPaths" & Working With Fallback Pages07:16
- Introducing API Routes06:20
- Working with MongoDB09:32
- Sending Http Requests To Our API Routes06:49
- Getting Data From The Database07:09
- Getting Meetup Details Data & Preparing Pages09:41
- Adding "head" Metadata09:19
- Deploying Next.js Projects12:26
- Using Fallback Pages & Re-deploying04:13
- Summary02:15
- Module Resources00:19
- Module Introduction02:58
- Preparing the Demo Project04:56
- Using CSS Transitions04:34
- Using CSS Animations05:32
- CSS Transition & Animations Limitations04:04
- Using ReactTransitionGroup12:19
- Using the Transition Component03:24
- Wrapping the Transition Component03:16
- Animation Timings03:14
- Transition Events02:33
- The CSSTransition Component05:23
- Customizing CSS Classnames02:30
- Animating Lists06:53
- Alternative Animation Packages04:29
- Wrap Up01:57
- Module Resources00:19
- Module Introduction01:01
- React 18 & This Section00:20
- Starting Project & Why You Would Replace Redux04:19
- Alternative: Using the Context API07:13
- Toggling Favorites with the Context API05:43
- Context API Summary (and why NOT to use it instead of Redux)02:30
- Getting Started with a Custom Hook as a Store08:11
- Finishing the Store Hook05:53
- Creating a Concrete Store04:11
- Using the Custom Store05:40
- Custom Hook Store Summary03:13
- Optimizing the Custom Hook Store04:04
- Bonus: Managing Multiple State Slices with the Custom Store Hook00:11
- Wrap Up02:00
- Module Resources00:19
- Module Introduction01:23
- What & Why?03:23
- Understanding Different Kinds Of Tests04:04
- What To Test & How To Test01:29
- Understanding the Technical Setup & Involved Tools02:39
- Running a First Test07:16
- Writing Our First Test10:14
- Grouping Tests Together With Test Suites02:14
- Testing User Interaction & State14:00
- Testing Connected Components03:19
- Testing Asynchronous Code09:11
- Working With Mocks08:30
- Summary & Further Resources03:47
- Module Resources00:19
- Module Introduction01:26
- What & Why?06:34
- Installing & Using TypeScript06:38
- Exploring the Base Types03:55
- Working with Array & Object Types05:33
- Understanding Type Inference02:47
- Using Union Types02:48
- Understanding Type Aliases02:42
- Functions & Function Types05:19
- Diving Into Generics08:01
- A Closer Look At Generics01:20
- Creating a React + TypeScript Project08:34
- Working with Components & TypeScript05:41
- Working with Props & TypeScript14:20
- Adding a Data Model09:09
- Time to Practice: Exercise Time!07:02
- Form Submissions In TypeScript Projects05:21
- Working with refs & useRef10:56
- Working with "Function Props"07:26
- Managing State & TypeScript05:13
- Adding Styling02:19
- Time to Practice: Removing a Todo09:27
- The Context API & TypeScript13:55
- Summary02:18
- Bonus: Exploring tsconfig.json05:46
- Module Resources00:19
- Module Introduction02:10
- What Are React Hooks?04:56
- The Starting Project04:51
- React 18 & This Section00:20
- Getting Started with useState()09:20
- More on useState() & State Updating11:54
- Array Destructuring02:34
- Multiple States03:47
- Rules of Hooks02:20
- Passing State Data Across Components07:56
- Time to Practice: Hooks Basics1 question
- Sending Http Requests07:16
- useEffect() & Loading Data08:06
- Understanding useEffect() Dependencies02:21
- More on useEffect()09:37
- What's useCallback()?05:28
- Working with Refs & useRef()05:21
- Cleaning Up with useEffect()03:21
- Deleting Ingredients02:28
- Loading Errors & State Batching08:48
- More on State Batching & State Updates00:35
- Understanding useReducer()09:43
- Using useReducer() for the Http State10:40
- Working with useContext()08:27
- Performance Optimizations with useMemo()10:30
- Getting Started with Custom Hooks13:45
- Sharing Data Between Custom Hooks & Components14:58
- Using the Custom Hook08:11
- Wrap Up03:05
- Module Resources00:19
- Module Introduction02:16
- What Is React?03:48
- Why React?07:25
- Building SPAs (Single Page Applications)02:00
- React Alternatives03:06
- Creating a React Project08:15
- Setting Up A Code Editor03:04
- React 1800:53
- Diving Into The Created Project06:31
- How React Works & Understanding Components07:36
- More Component Work & Styling with CSS Classes05:34
- Building & Re-Using Another Component07:19
- Working with "props" & Dynamic Content05:17
- Handling Events06:40
- Adding More Components05:40
- Introducing State08:18
- Working with "Event Props" (Passing Function As Props)07:07
- Use The Right React Router Version00:12
- Adding Routing16:52
- Adding Links & Navigation05:20
- Styling with CSS Modules05:45
- Outputting Lists of Data05:49
- Adding More React Components09:47
- Building Wrapper Components with props.children08:39
- Adding a Form08:58
- Getting User Input & Handling Form Submission09:45
- Preparing the App for Http06:51
- Sending a POST Request05:18
- Navigating Programmatically03:42
- Getting Started with Fetching Data09:25
- Using the useEffect() Hook10:07
- Introducing React Context11:02
- Context Logic & Different Ways Of Updating State05:54
- Using Context In Components12:10
- More Context Usage06:54
- Summary01:04
- Module Resources00:19
- What Now? Next Steps You Could Take!03:19
- Explore The React Ecosystem!04:27
- Finishing Thoughts01:10
- Course Roundup00:55
- Bonus!00:19
- Course Update Overview, Explanation & Migration Guide14:06
- Course Update & Migration Guide05:12
- Old Course Content Download00:17
Online Courses
Learning React doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of React related books and take your
YouTube videos
The number of high-quality and free React video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more