The Complete React Developer Course (w/ Hooks and Redux)
Learn how to build and launch React web applications using React, Redux, Webpack, React-Router, and more!
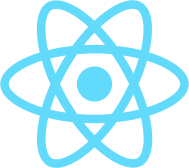
What you will learn?
- Build, test, and launch React apps
- Use cutting-edge ES6/ES7 JavaScript
- Setup authentication and user accounts
- Deploy your React apps live to the web
- Learn the latest React libraries and tools
- Master React, Redux, React-Router, and more
Your trainer

Andrew Mead
I'm Andrew, a full-stack developer living in beautiful Philadelphia!
I launched my first Udemy course in 2014 and had a blast teaching and helping others. Since then, I've launched 3 courses with over 110,000 students and over 18,000 5-star reviews.
I currently teach JavaScript, React, and Node.
Before I ever heard about Udemy or thought about teaching, I created a web app development company. I've helped companies of all sizes launch production web applications to their customers. I've had the honor of working with awesome companies like Siemens, Mixergy, and Parkloco.
I have a Computer Science degree from Temple University, and I've been programming for just over a decade. I love creating, programming, launching, learning, teaching, and biking.
200 lessons
Easy to follow lectures and videos covering subject details.
39 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Welcome & Asking Good Questions04:53
- "Why should I learn React?"05:10
- Section Intro: Setting up Your Environment00:50
- Installing Visual Studio Code01:35
- Installing Node.js & Yarn04:24
- Section Intro: Hello React02:07
- Setting up a Web Server12:00
- Hello React18:38
- Setting up Babel17:26
- Exploring JSX14:43
- JSX Expressions10:20
- Conditional Rendering in JSX22:34
- ES6 Aside: const and let16:29
- ES6 Aside: Arrow Functions12:36
- ES6 Aside: Arrow Functions Part II19:57
- Events and Attributes16:39
- Manual Data Binding11:52
- Forms and Inputs17:53
- Arrays in JSX14:15
- Picking an Option08:31
- Build It: Visibility Toggle09:10
- Section Intro: React Components01:18
- Thinking in React07:21
- ES6 Classes: Part I20:52
- ES6 Classes: Part II18:07
- Creating a React Component14:36
- Nesting Components05:43
- Component Props13:34
- Events & Methods10:34
- Method Binding11:24
- What Is Component State?06:50
- Adding State to Counter App: Part I08:18
- Adding State to Counter App: Part II11:00
- Alternative setState Syntax07:15
- Build It: Adding State to VisibilityToggle08:13
- Indecision State: Part I17:06
- Indecision State: Part II14:33
- Summary: Props vs. State03:41
- Section Intro: Stateless Functional Components00:54
- The Stateless Functional Component13:47
- Default Prop Values09:49
- React Dev Tools07:03
- Removing Individual Options16:22
- Lifecycle Methods10:07
- Saving and Loading Options Data14:36
- Saving and Loading the Count10:34
- Section Intro: Webpack01:15
- What Is Webpack?09:06
- Avoid Global Modules08:25
- Installing & Configuring Webpack16:31
- ES6 import/export18:32
- Default Exports11:18
- Importing npm Modules11:28
- Setting up Babel with Webpack09:27
- One Component per File18:12
- Source Maps with Webpack05:16
- Webpack Dev Server08:48
- ES6 class properties17:33
- Section Intro: Using a Third-Party Component01:13
- Passing Children to Component06:25
- Setting up React-Modal18:45
- Bonus: Refactoring Other Stateless Functional Components02:25
- Section Intro: Styling React00:49
- Setting up Webpack with SCSS13:16
- Architecture and Header Styles14:48
- Reset That $#!*04:58
- Theming with Variables14:18
- Big Button & Options List15:24
- Styling the Options List14:13
- Styling Option Item16:40
- Styling React-Modal18:00
- Mobile Considerations12:57
- Bonus: Favicon02:53
- Section Intro: React Router02:49
- Server vs. Client Routing04:39
- Setting Up Budget App06:54
- React-Router 10120:13
- Setting up a 40405:58
- Linking Between Routes14:08
- Organizing Our Routes12:30
- Query Strings and URL Parameters09:10
- Build It: Router for Portfolio Site18:27
- Section Intro: Redux01:35
- Why Do We Need Something Like Redux?13:33
- Setting up Redux07:53
- Dispatching Actions13:00
- Subscribing and Dynamic Actions09:35
- ES6 Object Destructuring15:49
- ES6 Array Destructuring10:38
- Refactoring and Organizing16:41
- Reducers12:05
- Working with Multiple Reducers13:40
- ES6 Spread Operator in Reducers17:36
- Spreading Objects14:36
- Wrapping up Our Reducers09:52
- Filtering Redux Data16:54
- Sorting Redux Data06:14
- Section Intro: Connecting React and Redux01:06
- Organizing Redux15:02
- The Higher Order Component15:30
- Connecting Store and Component with React-Redux15:40
- Rendering Individual Expenses09:09
- Controlled Inputs for Filters14:21
- Dropdown for Picking SortBy08:41
- Creating Expense Add/Edit Form20:09
- Setting up a Date Picker21:34
- Wiring up Add Expense16:44
- Wiring up Edit Expense19:06
- Redux Dev Tools05:33
- Filtering by Dates19:44
- Section Intro: Testing React Components03:03
- Setting up Jest19:34
- Testing Expenses Action Generators16:55
- Testing Filters Action Generators11:06
- Testing Expenses Selector17:03
- Testing Filters Reducer14:07
- Testing Expenses Reducer15:10
- Snapshot Testing12:13
- Enzyme21:53
- Snapshot Testing with Dynamic Components15:42
- Mocking Libraries with Jest11:51
- Testing User Interaction16:54
- Test Spies17:39
- Testing AddExpensePage14:39
- Testing EditExpensePage19:00
- Testing ExpenseListFilters14:50
- Testing ExpenseListFilters: Part II12:27
- Section Intro: Deploying Your Apps01:00
- Installing Git04:26
- What is Git?05:17
- Integrating Git into Our Project12:54
- Setting up SSH and Github18:08
- Production Webpack13:07
- Creating Separate CSS Files16:47
- A Production Web Server with Express13:14
- Deploying with Heroku16:44
- Regular vs Development Dependencies15:36
- New Feature Workflow12:32
- Build It: Adding Total Selector17:25
- Build It: Adding Summary Component18:39
- Section Intro: Firebase 10101:11
- Getting Firebase11:40
- Writing to the Database14:59
- ES6 Promises14:30
- Promises with Firebase10:54
- Removing Data from Firebase05:42
- Updating Data10:41
- Fetching Data From Firebase17:54
- Array Data in Firebase: Part I15:12
- Array Data in Firebase: Part II14:56
- Section Intro: Firebase with Redux01:18
- Asynchronous Redux Actions18:49
- Testing Async Redux Actions: Part I16:59
- Testing Async Redux Actions: Part II12:12
- Creating a Separate Test Database21:15
- Heroku Environment Variables06:38
- Fetching Expenses: Part I12:38
- Fetching Expenses: Part II13:52
- Remove Expense12:03
- Update Expense10:31
- Section Intro: Firebase Authentication00:59
- Login Page and Google Authentication19:26
- Logging Out11:05
- Redirecting Login or Logout12:48
- The Auth Reducer15:49
- Private Only Routes13:04
- Public Only Routes05:29
- Private Firebase Data18:33
- Data Validation and Deployment14:58
- Section Intro: Styling Budget App00:33
- Styling Login Page13:45
- Styling Buttons15:35
- Styling Summary Area12:20
- Styling List Filters10:05
- Styling Inputs11:30
- Styling Expense Form13:19
- Styling Expenses List: Part I13:03
- Styling Expenses List: Part II17:58
- Adding Loader09:26
- Babel Polyfill04:55
- Final Deployment01:57
- Section Into: What Now?01:35
- Creating the Final Boilerplate18:05
- Budget App Enhancements03:18
- Indecision App Enhancements01:09
- New App Idea: Blog02:48
- Until Next Time01:11
- Section Intro00:29
- Using Create React App16:46
- The useState Hook15:50
- useState vs. setState12:18
- Complex State with useState16:21
- The useEffect Hook11:24
- useEffect Dependencies11:57
- Cleaning up Effects08:11
- The useReducer Hook14:46
- The Context API & useContext Hook: Part I20:00
- The Context API & useContext Hook: Part II16:58
- Fragments02:43
- Creating Custom Hooks20:16
Online Courses
Learning React doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of React related books and take your
YouTube videos
The number of high-quality and free React video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more