Modern React with Redux [2023 Update]
Master React and Redux Toolkit. Includes RTK Query, tons of custom hooks, and more! Course 100% Updated November 2022
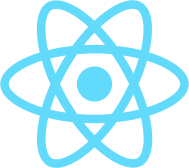
What you will learn?
- Create dynamic web apps using the latest in web technology
- Acquire the programming skills needed to obtain a software engineering job
- Practice your skills with many large projects, exercises, and quizzes
- Master the fundamentals concepts behind React and Redux
- Become fluent in the toolchain supporting React, including NPM, Webpack, Babel, and ES6/ES2015 Javascript syntax
- Be the engineer who explains how Redux works to everyone else, because you know the fundamentals so well
- Realize the power of building reusable components
Your trainer

Stephen Grider
Stephen Grider has been building complex Javascript front ends for top corporations in the San Francisco Bay Area. With an innate ability to simplify complex topics, Stephen has been mentoring engineers beginning their careers in software development for years, and has now expanded that experience onto Udemy, authoring the highest rated React course. He teaches on Udemy to share the knowledge he has gained with other software engineers. Invest in yourself by learning from Stephen's published courses.
721 lessons
Easy to follow lectures and videos covering subject details.
64 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- How to Get Help01:01
- Join Our Community!00:07
- Course Resources00:52
- Let's Build an App!07:56
- Critical Questions05:49
- Core Concepts4 questions
- A Few More Critical Questions08:51
- Node Setup01:58
- Creating a React Project02:38
- What is Create React App?05:50
- Showing Basic Content06:41
- What is JSX?05:48
- Printing JavaScript Variables in JSX04:29
- Shorthand JS Expressions01:51
- Showing Javascript Values in JSX3 questions
- Exercise Overview00:42
- Quick Practice with JSX1 question
- Exercise Solution00:35
- Typical Component Layouts01:58
- Customizing Elements with Props07:36
- Converting HTML to JSX05:07
- Applying Styling in JSX06:13
- More on JSX3 questions
- Practice JSX Conversion1 question
- Exercise Solution02:05
- Extracting Components04:26
- Module Systems Overview16:05
- Imports and Exports2 questions
- Cheatsheet for JSX00:05
- Project Overview05:37
- Creating Core Components05:00
- Introducing the Props System04:51
- Picturing the Movement of Data06:06
- Adding Props03:04
- Using Argument Destructuring04:57
- Practice with Props1 question
- Exercise Solution01:30
- The React Developer Tools02:37
- The Most Common Props Mistake04:42
- Communicating With Props3 questions
- Images for the App00:06
- Including Images08:09
- Handling Image Accessibility06:39
- Review on how CSS Works06:10
- Adding CSS Libraries with NPM05:57
- A Big Pile of HTML!09:38
- Last Bit of Styling05:45
- App Overview02:52
- Initial App Setup05:09
- Introducing the Event System03:14
- Events in Detail08:11
- Variations on Event Handlers06:42
- Quiz on Events2 questions
- Exercise with Events1 question
- Exercise Solution01:15
- Introducing the State System03:43
- More on State08:43
- Understanding the Re-Rendering Process08:10
- Got These Five Points?4 questions
- Why Array Destructuring?08:41
- Back to the App03:33
- Picking a Random Element07:11
- List Building in React09:46
- Images for the App00:06
- Loading and Showing SVGs05:50
- Increasing Image Size05:51
- Adding Custom CSS06:21
- Finalizing Styling05:58
- App Wrapup and Review08:52
- App Overview02:24
- Project Setup05:53
- The Path Forward04:06
- Overview of HTTP Requests11:15
- Understanding the API06:07
- Making an HTTP Request10:36
- [Optional] Using Async:Await02:38
- Data Fetching Cleanup03:20
- Thinking About Data Flow08:42
- Child to Parent Communication06:07
- Implementing Child to Parent Communication07:29
- Upward Communication1 question
- Handling Form Submission11:32
- Handling Input Elements09:21
- [Optional] OK But Why?14:21
- Creating a Controlled Input1 question
- Exercise Solution02:35
- Running the Search04:06
- Reminder on Async:Await03:00
- Communicating the List of Images Down12:31
- Building a List of Images05:03
- Handling List Updates09:10
- Notes on Keys09:22
- Displaying Images01:48
- A Touch of Styling04:26
- App Wrapup03:41
- App Overview03:20
- Initial Setup05:56
- State Location05:14
- Reminder on Event Handlers04:51
- Extra CSS00:06
- Receiving New Titles08:54
- Adding Styling02:12
- Updating State10:24
- Don't Mutate That State!04:26
- State Updates - Cheat Sheet00:17
- [Optional] Adding Elements to the Start or End01:24
- Adding Elements1 question
- [Optional] Exercise Solution01:12
- [Optional] Inserting Elements04:43
- Inserting Elements1 question
- [Optional] Exercise Solution02:00
- [Optional] Removing Elements07:04
- Removing Elements1 question
- [Optional] Exercise Solution02:09
- [Optional] Modifying Elements06:05
- Modifying Elements1 question
- [Super Optional] Why the Special Syntax?07:10
- [Optional] Exercise Solution02:26
- [Optional] Adding, Changing, or Removing Object Properties03:58
- Cheatsheet for State Changes00:05
- Adding a Book, For Real!01:49
- Generating Random ID's02:05
- Displaying the List05:35
- Deleting Records06:36
- Toggling Form Display06:31
- Default Form Values07:06
- Updating the Title08:44
- Closing the Form on Submit05:06
- A Better Solution!04:30
- Collapsing Two Handlers into One03:18
- Adding Images07:58
- Adding Data Persistence07:48
- Server Setup03:44
- What Just Happened?04:36
- How the API Works05:20
- Introducing the REST Client05:05
- Using the REST Client06:58
- Creating a New Record06:56
- Fetching a List of Records04:25
- Introducing useEffect02:51
- useEffect in Action06:24
- More on useEffect09:52
- When Does it Run?3 questions
- Updating a Record03:18
- Thinking About Updates05:27
- Deleting a Record01:18
- Introducing Context09:12
- Context in Action06:15
- Changing Context Values06:51
- More on Changing Context08:46
- Application vs Component State11:50
- Refactoring to Use Context04:38
- Refactoring the App05:32
- Quick Note00:11
- Reminder on Sharing with Context06:12
- Props and Context Together03:08
- Last Bit of Refactoring05:55
- A Small Taste of Reusable Hooks09:53
- Return to useEffect08:06
- Quick Note00:16
- Understanding the Issue07:17
- Applying the Fix06:23
- ESLint is Good, but be Careful!10:57
- Stable References with useCallback13:08
- Fixing Bugs with useCallback02:08
- Fixing UseEffect Bugs5 questions
- useEffect Cleanup Functions05:33
- The Purpose of Cleanup Functions05:37
- Project Overview04:25
- Project Setup01:54
- Some Button Theory08:19
- Underlying Elements03:48
- The Children Prop04:21
- Props Design06:21
- Validating Props with PropTypes08:29
- PropTypes in Action06:52
- Introducing TailwindCSS06:37
- Installing Tailwind04:50
- How to use Tailwind06:35
- Review on Styling10:22
- The ClassNames Library11:28
- Building Some Variations05:55
- Finalizing the Variations06:07
- Using Icons in React Projects09:49
- Issues with Event Handlers07:16
- Passing Props Through06:07
- Handling the Special ClassName Case05:16
- Project Organization10:43
- Refactoring with Organization05:56
- Component Overview04:48
- Component Setup03:42
- Reminder on Building Lists04:04
- Quick Note00:08
- State Design Process Overview29:30
- Finding the Expanded Item04:09
- Conditional Rendering06:25
- Inline Event Handlers10:00
- Variation on Event Handlers08:21
- Conditional Icon Rendering02:14
- Displaying Icons01:45
- Adding Styling03:48
- Toggling Panel Collapse02:06
- Quick Note00:13
- [Optional] Delayed State Updates08:34
- [Optional] Functional State Updates11:42
- Conditional Rendering2 questions
- Event Handlers with Lists1 question
- Exercise Solution01:26
- Component Overview01:08
- Designing the Props05:49
- Component Creation04:14
- [Optional] More State Design20:11
- Finally... Implementation!06:45
- Reminder on Event Handlers in Maps04:47
- Dropdown as a Controlled Component04:44
- Controlled Component Implementation05:36
- Existence Check Helper04:30
- Community Convention with Props Names06:34
- Form Controls - Prop Naming Convention1 question
- Exercise Solution01:37
- Adding Styling05:33
- The Panel Component07:06
- Creating the Reusable Panel05:59
- A Challenging Extra Feature05:19
- Document-Wide Click Handlers02:46
- Event Capture and Bubbling06:14
- Putting it All Together02:20
- Why a Capture Phase Handler?14:34
- Reminder on the useEffect Function03:52
- Reminder on useEffect Cleanup05:46
- Issues with Element References02:56
- useRef in Action05:04
- Checking Click Location03:14
- Traditional Browser Navigation09:33
- Theory of Navigation in React07:56
- Extracting the DropdownPage02:43
- Answering Critical Questions05:33
- The PushState Function02:56
- Handling Link Clicks02:28
- Handling Back:Forward Buttons06:23
- Navigation Context04:05
- Listening to Forward and Back Clicks06:19
- Programmatic Navigation05:36
- A Link Component05:25
- A Route Component05:49
- Handling Control and Command Keys02:52
- Link Styling01:18
- Custom Navigation Hook02:55
- Adding a Sidebar Component08:22
- Highlighting the Active Link07:25
- Navigation Wrapup01:54
- Modal Component Overview04:20
- Toggling Visibility05:49
- At First Glance, Easy!03:05
- We're Lucky it Works At All!09:26
- Fixing the Modal with Portals07:09
- Closing the Modal02:04
- Customizing the Modal05:03
- Additional Styling01:44
- One Small Bug01:53
- Modal Wrapup05:47
- Creating a Reusable table03:34
- Communicating Data to the Table03:03
- Reminder on Table HTML Structure04:25
- Building the Rows02:52
- Better Styling03:38
- Done! But It's Not Reusable05:10
- Here's the Idea02:29
- Dynamic Table Headers04:46
- Rendering Individual Cells05:18
- Fixed Cell Values05:00
- Nested Maps04:35
- Fixing the Color00:57
- Adding a Key Function04:12
- Adding Sorting to the Table06:38
- Reminder on Sorting in JavaScript06:24
- Sorting Strings02:45
- Sorting Objects03:56
- Object Sort Implementation07:38
- Reversing Sort Order04:26
- Optional Sorting04:10
- A Small Extra Feature03:14
- Customizing Header Cells03:49
- React Fragments05:57
- The Big Reveal10:39
- Adding SortableTable11:32
- Watching for Header Cell Clicks01:43
- Quick State Design06:57
- Adding Sort State05:24
- Yessssss, It Worked!11:00
- Determining Icon Set05:08
- Styling Header Cells03:37
- Resetting Sort Order02:55
- Table Wrapup03:53
- Exploring Code Reuse03:39
- Revisiting Custom Hooks03:10
- Creating the Demo Component04:46
- Custom Hook Creation03:16
- Quick Note00:16
- Hook Creation Process in Depth09:42
- Making a Reusable Sorting Hook11:09
- App Overview03:03
- Adding the Form04:26
- More on the Form06:58
- useReducer in Action09:47
- Rules of Reducer Functions09:22
- Understanding Action Objects10:45
- Constant Action Types06:35
- Refactoring to a Switch05:34
- Adding New State Updates06:20
- A Few Design Considerations Around Reducers09:51
- A Few Questions on Reducers5 questions
- Introducing Immer04:59
- Immer in Action03:21
- Into the World of Redux05:23
- Redux vs Redux Toolkit09:17
- App Overview03:03
- The Path Forward03:31
- Implementation Time!04:41
- Understanding the Store09:21
- The Store's Initial State03:32
- Understanding Slices07:50
- Understanding Action Creators06:33
- Connecting React to Redux04:24
- Updating State from a Component08:25
- Accessing State in a Component06:34
- Removing Content07:12
- Practice Updating State!06:55
- Practice Accessing State!02:02
- Even More State Updating!04:29
- Resetting State06:25
- Multiple State Updates04:47
- Understanding Action Flow06:45
- Watching for Other Actions05:11
- Getting an Action Creator's Type03:06
- Manual Action Creation09:31
- File and Folder Structure08:41
- Refactoring the Project Structure11:07
- Link to Completed Project00:03
- Project Overview04:10
- Adding Component Boilerplate06:08
- Thinking About Derived State07:54
- Thinking About Redux Design05:17
- Adding the Form Slice05:34
- Maintaining a Collection with a Slice09:40
- Creating the Store04:33
- Form Values to Update State08:44
- Receiving the Cost05:17
- Dispatching During the Form Submission04:31
- Awkward Double Keys07:18
- Listing the Records03:25
- Deleting Records02:15
- CSS File for Download00:06
- Adding Styling01:27
- Form Reset on Submission03:21
- Reminder on ExtraReducers02:23
- Adding a Searching Input05:36
- Derived State in useSelector05:07
- Total Car Cost06:22
- Highlighting Existing Cars06:30
- App Overview08:36
- Adding a Few Dependencies02:45
- Initial App Boilerplate02:09
- API Server Setup02:51
- Component Files00:06
- Adding a Few Components03:13
- Creating the Redux Store05:34
- Thinking About Data Structuring09:19
- Reminder on Request Conventions01:55
- Data Fetching Techniques02:37
- Optional Async Thunks Section00:35
- Adding State for Data Loading06:52
- Understanding Async Thunks04:14
- Steps for Adding a Thunk07:46
- More on Adding Thunks13:17
- Wrapping up the Thunk05:23
- Using Loading State04:30
- Adding a Pause for Testing03:27
- Adding a Skeleton Loader05:39
- Animations with TailwindCSS12:02
- Rendering the List of Users03:16
- Creating New Users12:07
- Unexpected Loading State06:03
- Strategies for Fine-Grained Loading State07:35
- Local Fine-Grained Loading State10:07
- More on Loading State02:55
- Handling Errors with User Creation05:03
- Creating a Reusable Thunk Hook11:34
- Creating a Fetch-Aware Button Component05:41
- Better Skeleton Display03:41
- A Thunk to Delete a User04:56
- Updating the Slice04:27
- Refactoring the Component03:31
- Deleting the User04:46
- Fixing a Delete Error05:13
- Album Feature Overview04:48
- Additional Components07:21
- Adding the ExpandablePanel04:51
- Wrapping Up the ExpandablePanel04:00
- Adding the Albums Listing02:43
- Skipping to this Section?00:25
- [Optional] Getting Caught Up02:47
- Introducing Redux Toolkit Query08:00
- Creating a RTK Query API06:20
- Creating an Endpoint14:01
- Using the Generated Hook11:01
- A Few Immediate Notes05:56
- Rendering the List04:25
- Changing Data with Mutations08:42
- Differences Between Queries and Mutations03:13
- Options for Refetching Data06:18
- Request De-Duplication03:50
- Some Internals of Redux Toolkit Query06:14
- Refetching with Tags07:33
- Fine-Grained Tag Validation09:13
- Styling Fixups03:20
- Adding a Pause for Testing03:21
- Implementing Delete Endpoints05:34
- Refactoring the List04:46
- Remove Implementation07:42
- Easy Tag Invalidation05:58
- Getting Clever with Cache Tags06:24
- More Clever Tag Implementation04:47
- Photos Feature Overview04:11
- Lots of Photos Setup!06:01
- Adding the Endpoints12:38
- Creating the Photo04:37
- Showing the List of Photos05:14
- Adding Mouse-Over Deletes06:19
- Adding Automatic Data Refetching08:42
- Note on the Following Sections00:14
- Note on the Following Lectures - Old Version of Course00:13
- Let's Build an App!07:56
- Critical Questions05:49
- A Few More Critical Questions08:51
- Node Setup01:58
- Creating a React Project02:38
- What is Create React App?05:50
- Showing Basic Content06:41
- So... What is JSX?05:48
- Printing JavaScript Variables in JSX04:29
- Shorthand JS Expressions01:51
- Typical Component Layouts01:58
- Customizing Elements with Props07:36
- Converting HTML to JSX05:07
- Applying Styling in JSX06:13
- Important Note About Viewing Errors00:42
- Three Tenets of Components02:54
- Application Overview02:50
- Semantic UI CDN Link00:10
- Getting Some Free Styling07:15
- Naive Component Approach04:32
- IMPORTANT Info About Faker Installation00:37
- Specifying Images in JSX06:06
- Duplicating a Single Component01:55
- Extracting JSX to New Components05:01
- Component Nesting06:42
- React's Props System03:42
- Passing and Receiving Props07:05
- Passing Multiple Props03:10
- Passing Props - Solutions06:07
- Component Reuse03:25
- Implementing an Approval Card05:51
- Showing Custom Children05:46
- Component Reuse02:45
- Optional Styling00:14
- Class-Based Components06:04
- Application Overview04:00
- Scaffolding the App05:57
- Getting a Users Physical Location05:56
- Resetting Geolocation Preferences02:33
- Handling Async Operations with Functional Components03:53
- Refactoring from Functional to Class Components05:12
- The Rules of State06:07
- Initializing State Through Constructors06:16
- Updating State Properties07:43
- App Lifecycle Walkthrough07:05
- Handling Errors Gracefully06:21
- Conditionally Rendering Content05:38
- Introducing Lifecycle Methods07:14
- Why Lifecycle Methods?06:35
- Refactoring Data Loading to Lifecycle Methods02:45
- Alternate State Initialization05:20
- Passing State as Props04:19
- Determining Season05:34
- Ternary Expressions in JSX03:39
- Icons Not Loading and CORS errors00:32
- Showing Icons04:34
- Extracting Options to Config Objects04:13
- Adding Some Styling09:32
- Showing a Loading Spinner06:19
- Specifying Default Props04:52
- Avoiding Conditionals in Render05:22
- Optional Styling00:14
- Breather and Review08:16
- App Overview05:02
- Component Design03:39
- Adding Some Project Structure04:30
- Showing Forms to the User03:53
- Adding a Touch of Style03:41
- Creating Event Handlers07:42
- Alternate Event Handler Syntax02:29
- Uncontrolled vs Controlled Elements03:38
- More on Controlled Elements10:27
- Handling Form Submittal03:43
- Understanding 'this' In Javascript11:27
- Solving Context Issues07:19
- Communicating Child to Parent03:36
- Invoking Callbacks in Children04:42
- Fetching Data04:30
- Axios vs Fetch04:41
- Viewing Request Results08:16
- Handling Requests with Async Await07:38
- Setting State After Async Requests06:19
- Binding Callbacks02:17
- Creating Custom Clients05:28
- Rendering Lists02:41
- Review of Map Statements05:40
- Rendering Lists of Components02:54
- The Purpose of Keys in Lists05:32
- Implementing Keys in Lists04:27
- Grid CSS06:20
- Issues with Grid CSS04:20
- Creating an Image Card Component05:16
- Accessing the DOM with Refs03:24
- Accessing Image Height07:43
- Callbacks on Image Load02:53
- Dynamic Spans07:18
- App Review03:55
- App Overview02:37
- Component Design03:06
- Scaffolding the App06:16
- Reminder on Event Handlers06:35
- Handling Form Submittal03:41
- Accessing the Youtube API09:11
- Searching for Videos06:54
- Adding a Video Type00:21
- Putting it All Together06:51
- Updating State with Fetched Data04:44
- Passing State as Props03:48
- Rendering a List of Videos04:41
- Rendering Video Thumbnails04:40
- Styling a List08:43
- Communicating from Child to Parent05:05
- Deeply Nested Callbacks05:59
- Conditional Rendering05:52
- Styling the VideoDetail03:10
- Displaying a Video Player08:15
- Fixing a Few Warnings06:38
- Defaulting Video Selection04:52
- React Hooks03:36
- Important Note00:29
- App Architecture06:02
- Communicating the Items Prop04:50
- Building and Styling the Accordion06:11
- Helper Functions in Function Components05:16
- Introducing useState03:34
- Understanding useState08:45
- Setter Functions01:37
- Expanding the Accordion03:11
- Creating Additional Widgets02:46
- The Search Widget Architecture04:30
- Scaffolding the Widget01:40
- Text Inputs with Hooks04:28
- When do we Search?06:45
- The useEffect Hook06:41
- Testing Execution04:02
- When Does It Run?3 questions
- Async Code in useEffect07:21
- Executing the Request from useEffect03:41
- Default Search Terms04:54
- List Building!03:55
- XSS Attacks in React07:04
- XSS Server Code00:21
- Linking to a Wikipedia Page03:39
- Only Search with a Term01:00
- Throttling API Requests04:05
- Reminder on setTimeout03:32
- useEffect's Cleanup Function06:08
- Implementing a Delayed Request02:59
- Searching on Initial Render02:24
- Edge Case When Clearing Out Input Form00:16
- Optional Video - Fixing a Warning20:21
- Dropdown Architecture03:25
- Scaffolding the Dropdown03:07
- A Lot of JSX05:16
- Selection State06:16
- Filtering the Option List01:45
- Hiding and Showing the Option List07:40
- Err... Why is this Hard?03:58
- Reminder on Event Bubbling04:34
- Applying What We've Learned03:32
- React v17 Update - capture: true00:12
- Binding an Event Handler02:47
- Why Stay Open!?05:11
- Which Element Was Clicked?04:21
- Making use of useRef04:12
- Important Update for Event Listeners00:18
- Body Event Listener Cleanup06:42
- The Translate Widget04:04
- Scaffolding the Translate Component05:04
- Adding the Language Input03:42
- Understanding the Convert Component06:24
- Google Translate API Key00:15
- Building the Convert Component03:42
- Using the Google Translate API06:31
- Displaying Translated Text05:31
- Debouncing Translation Updates06:45
- Reviewing UseState and UseEffect00:56
- Navigation in React02:23
- Basic Component Routing07:09
- Building a Reusable Route Component06:49
- Implementing a Header for Navigation02:41
- Handling Navigation03:57
- Building a Link05:21
- Changing the URL03:10
- Detecting Navigation04:01
- Updating the Route04:25
- Handling Command Clicks02:03
- Project Overview02:24
- Refactoring the SearchBar08:06
- Refactoring the App08:07
- Removing a Callback02:53
- Overview on Custom Hooks05:41
- Process for Building Custom Hooks06:39
- Extracting Video Logic06:52
- Using the Custom Hook05:28
- Deployment Overview03:29
- Deployment with Vercel06:06
- Deployment with Netlify07:09
- Introduction to Redux03:23
- Redux by Analogy07:05
- A Bit More Analogy05:20
- Finishing the Analogy09:23
- Mapping the Analogy to Redux05:44
- Modeling with Redux08:44
- Creating Reducers11:45
- Rules of Reducers04:56
- Testing Our Example08:26
- Important Redux Notes07:32
- Finished Insurance Policy Code00:02
- React Cooperating with Redux01:40
- React, Redux, and...React-Redux!?05:34
- Design of the Redux App04:36
- How React-Redux Works06:44
- Redux Project Structure04:06
- Named vs Default Exports03:50
- Building Reducers05:12
- createStore Strikethrough Warning in Editor00:26
- Wiring Up the Provider06:06
- The Connect Function05:54
- Configuring Connect with MapStateToProps06:43
- Building a List with Redux Data07:05
- Calling Action Creators from Components07:21
- Redux is Not Magic!06:04
- Functional Components with Connect06:32
- Conditional Rendering05:14
- App Overview and Goals07:37
- Initial App Setup06:16
- Tricking Redux with Dummy Reducers03:16
- A Touch More Setup02:25
- How to Fetch Data in a Redux App05:59
- Wiring Up an Action Creator03:01
- Making a Request From an Action Creator05:13
- Understanding Async Action Creators08:56
- More on Async Action Creators05:47
- Middlewares in Redux03:52
- Behind the Scenes of Redux Thunk10:07
- Shortened Syntax with Redux Thunk06:44
- Rules of Reducers02:54
- Return Values from Reducers03:07
- Argument Values05:04
- Pure Reducers02:35
- Mutations in Javascript06:50
- Equality of Arrays and Objects02:39
- A Misleading Rule12:24
- Safe State Updates in Reducers09:59
- Switch Statements in Reducers04:07
- Adding a Reducer Case1 question
- Reducer Case Solution00:26
- Dispatching Correct Values06:44
- List Building!04:00
- Displaying Users07:39
- Fetching Singular Records04:10
- Displaying the User Header05:07
- Finding Relevant Users07:49
- Extracting Logic to MapStateToProps07:47
- That's the Issue!02:38
- Memoizing Functions06:54
- Memoization Issues06:49
- One Time Memoization04:47
- Alternate Overfetching Solution04:24
- Action Creators in Action Creators!07:42
- Finding Unique User Ids08:36
- Quick Refactor with Chain04:11
- Optional Styling00:14
- App Wrapup03:34
- App Outline06:16
- Mockups in Detail05:13
- App Challenges05:09
- Initial Setup02:46
- IMPORTANT - React Router Installation Update00:11
- Introducing React Router08:05
- How React Router Works03:57
- How Paths Get Matched08:34
- How to *Not* Navigate with React Router04:56
- Navigating with React Router05:09
- [Optional] - Different Router Types18:17
- Component Scaffolding05:50
- Wiring Up Routes04:09
- Always Visible Components02:20
- Connecting the Header05:50
- Links Inside Routers02:44
- OAuth-Based Authentication09:02
- OAuth for Servers vs Browser Apps05:34
- Creating OAuth Credentials05:12
- Wiring Up the Google API Library08:54
- Required plugin_name Parameter - Do Not Skip00:17
- Sending a User Into the OAuth Flow05:47
- Rendering Authentication Status09:48
- Updating Auth State07:14
- Displaying Sign In and Sign Out Buttons03:22
- On-Demand Sign In and Sign Out03:02
- Redux Architecture Design09:29
- Redux Setup04:13
- Connecting Auth with Action Creators04:34
- Building the Auth Reducer05:16
- Handling Auth Status Through Redux05:24
- Fixed Action Types05:34
- Recording the User's ID06:07
- Using Redux Dev Tools to Inspect the Store09:41
- Debug Sessions with Redux Dev Tools04:13
- Important Note about Redux Form Installation00:42
- Forms with Redux Form06:10
- Useful Redux Form Examples02:41
- Connecting Redux Form04:04
- Creating Forms08:02
- Automatically Handling Events09:23
- Customizing Form Fields03:50
- Handling Form Submission05:34
- Validation of Form Inputs06:44
- Displaying Validation Messages05:25
- Showing Errors on Touch07:22
- Highlighting Errored Fields03:28
- Missing Lectures From Old Version of Course00:32
- Bonus!00:27
Online Courses
Learning React doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of React related books and take your
YouTube videos
The number of high-quality and free React video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more