Angular - The Complete Guide (2023 Edition)
Master Angular (formerly "Angular 2") and build awesome, reactive web apps with the successor of Angular.js
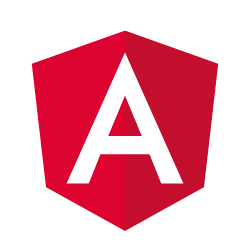
What you will learn?
- Develop modern, complex, responsive and scalable web applications with Angular 14
- Fully understand the architecture behind an Angular application and how to use it
- Use the gained, deep understanding of the Angular fundamentals to quickly establish yourself as a frontend developer
- Create single-page applications with one of the most modern JavaScript frameworks out there
Your trainer

Maximilian Schwarzmüller
Starting out at the age of 13 I never stopped learning new programming skills and languages. Early I started creating websites for friends and just for fun as well. Besides web development I also explored Python and other non-web-only languages. This passion has since lasted and lead to my decision of working as a freelance web developer and consultant. The success and fun I have in this job is immense and really keeps that passion burningly alive.
Starting web development on the backend (PHP with Laravel, NodeJS, Python) I also became more and more of a frontend developer using modern frameworks like React, Angular or VueJS 2 in a lot of projects. I love both worlds nowadays!
471 lessons
Easy to follow lectures and videos covering subject details.
34.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Course Introduction00:57
- What is Angular?01:59
- Join our Online Learning Community00:25
- Angular vs Angular 2 vs Latest Angular Version02:55
- CLI Deep Dive & Troubleshooting01:13
- Project Setup and First App08:49
- Editing the First App10:05
- The Course Structure04:00
- How to get the Most out of the Course02:25
- What is TypeScript?02:09
- Optional: TypeScript Quick Introduction00:18
- A Basic Project Setup using Bootstrap for Styling04:27
- About the Course Code / Code Snapshots01:06
- Module Introduction00:56
- How an Angular App gets Loaded and Started07:11
- Components are Important!03:08
- Creating a New Component06:51
- Understanding the Role of AppModule and Component Declaration05:15
- Using Custom Components01:12
- Creating Components with the CLI & Nesting Components03:51
- Working with Component Templates03:08
- Working with Component Styles04:02
- Fully Understanding the Component Selector03:50
- Practicing Components1 question
- [OPTIONAL] Assignment Solution06:42
- What is Databinding?02:49
- String Interpolation05:19
- Property Binding06:34
- Property Binding vs String Interpolation03:07
- Event Binding04:09
- Bindable Properties and Events00:21
- Passing and Using Data with Event Binding04:37
- Important: FormsModule is Required for Two-Way-Binding!00:12
- Two-Way-Databinding02:47
- Combining all Forms of Databinding01:46
- Practicing Databinding1 question
- [OPTIONAL] Assignment Solution05:12
- Understanding Directives02:17
- Using ngIf to Output Data Conditionally03:53
- Enhancing ngIf with an Else Condition02:46
- Styling Elements Dynamically with ngStyle05:01
- Applying CSS Classes Dynamically with ngClass02:53
- Outputting Lists with ngFor03:43
- Practicing Directives1 question
- [OPTIONAL] Assignment Solution07:06
- Getting the Index when using ngFor02:52
- Project Introduction01:04
- Planning the App05:33
- Creating a New App Correctly00:41
- Setting up the Application04:31
- Creating the Components09:32
- Using the Components03:15
- Adding a Navigation Bar05:09
- Alternative Non-Collapsable Navigation Bar00:27
- Creating a "Recipe" Model04:33
- Adding Content to the Recipes Components07:41
- Outputting a List of Recipes with ngFor04:37
- Displaying Recipe Details05:52
- Working on the ShoppingListComponent01:49
- Creating an "Ingredient" Model02:35
- Creating and Outputting the Shopping List02:27
- Adding a Shopping List Edit Section03:28
- Wrap Up & Next Steps00:54
- Understanding Angular Error Messages04:36
- Debugging Code in the Browser Using Sourcemaps05:32
- Module Introduction00:36
- Splitting Apps into Components05:56
- Property & Event Binding Overview01:44
- Binding to Custom Properties05:43
- Assigning an Alias to Custom Properties01:59
- Binding to Custom Events09:05
- Assigning an Alias to Custom Events00:57
- Custom Property and Event Binding Summary02:02
- Understanding View Encapsulation04:59
- More on View Encapsulation02:43
- Using Local References in Templates04:36
- @ViewChild() in Angular 8+00:24
- Getting Access to the Template & DOM with @ViewChild05:02
- Projecting Content into Components with ng-content03:22
- Understanding the Component Lifecycle04:54
- Seeing Lifecycle Hooks in Action11:55
- Lifecycle Hooks and Template Access02:33
- @ContentChild() in Angular 8+00:02
- Getting Access to ng-content with @ContentChild03:00
- Wrap Up00:49
- Practicing Property & Event Binding and View Encapsulation1 question
- [OPTIONAL] Assignment Solution12:21
- Introduction00:50
- Adding Navigation with Event Binding and ngIf07:31
- Passing Recipe Data with Property Binding04:43
- Passing Data with Event and Property Binding (Combined)10:31
- Make sure you have FormsModule added!00:09
- Allowing the User to Add Ingredients to the Shopping List07:22
- Module Introduction01:23
- ngFor and ngIf Recap03:36
- ngClass and ngStyle Recap02:57
- Creating a Basic Attribute Directive06:42
- Using the Renderer to build a Better Attribute Directive07:04
- More about the Renderer00:13
- Using HostListener to Listen to Host Events03:00
- Using HostBinding to Bind to Host Properties03:17
- Binding to Directive Properties06:43
- What Happens behind the Scenes on Structural Directives03:04
- Building a Structural Directive06:13
- Understanding ngSwitch02:49
- Building and Using a Dropdown Directive06:25
- Closing the Dropdown From Anywhere00:18
- Module Introduction01:39
- Why would you Need Services?02:04
- Creating a Logging Service03:32
- Injecting the Logging Service into Components06:21
- Creating a Data Service06:41
- Understanding the Hierarchical Injector02:40
- How many Instances of Service Should It Be?02:12
- Injecting Services into Services05:13
- Using Services for Cross-Component Communication04:06
- A Different Way Of Injecting Services00:31
- Practicing Services1 question
- [OPTIONAL] Assignment Solution09:37
- Introduction01:23
- Setting up the Services01:15
- Managing Recipes in a Recipe Service04:07
- Using a Service for Cross-Component Communication05:07
- Adding the Shopping List Service05:04
- Using Services for Pushing Data from A to B03:08
- Adding Ingredients to Recipes03:41
- Passing Ingredients from Recipes to the Shopping List (via a Service)07:03
- Module Introduction01:26
- Why do we need a Router?01:16
- Understanding the Example Project00:10
- Setting up and Loading Routes08:01
- Navigating with Router Links05:18
- Understanding Navigation Paths04:54
- Styling Active Router Links05:01
- Navigating Programmatically03:28
- Using Relative Paths in Programmatic Navigation05:09
- Passing Parameters to Routes03:10
- Fetching Route Parameters04:01
- Fetching Route Parameters Reactively07:25
- An Important Note about Route Observables02:42
- Passing Query Parameters and Fragments05:53
- Retrieving Query Parameters and Fragments03:15
- Practicing and some Common Gotchas06:07
- Setting up Child (Nested) Routes04:28
- Using Query Parameters - Practice05:36
- Configuring the Handling of Query Parameters01:50
- Redirecting and Wildcard Routes04:36
- Important: Redirection Path Matching00:37
- Outsourcing the Route Configuration04:40
- An Introduction to Guards01:45
- Protecting Routes with canActivate08:55
- Protecting Child (Nested) Routes with canActivateChild02:51
- Using a Fake Auth Service01:43
- Controlling Navigation with canDeactivate12:28
- Passing Static Data to a Route05:56
- Resolving Dynamic Data with the resolve Guard09:53
- Understanding Location Strategies04:43
- Wrap Up00:51
- Planning the General Structure01:36
- Setting Up Routes07:58
- Adding Navigation to the App01:41
- Marking Active Routes01:31
- Fixing Page Reload Issues02:51
- Child Routes: Challenge01:54
- Adding Child Routing Together04:21
- Configuring Route Parameters06:22
- Passing Dynamic Parameters to Links03:01
- Styling Active Recipe Items01:14
- Adding Editing Routes03:44
- Retrieving Route Parameters03:19
- Programmatic Navigation to the Edit Page04:46
- One Note about Route Observables00:52
- Project Cleanup00:20
- Module Introduction04:25
- Install RxJS00:07
- Analyzing Angular Observables02:15
- Getting Closer to the Core of Observables06:50
- Building a Custom Observable04:46
- Errors & Completion06:39
- Observables & You!01:55
- Understanding Operators08:39
- Subjects08:17
- Wrap Up01:15
- Useful Resources & Links00:02
- Improving the Reactive Service with Observables (Subjects)06:04
- Changed the Subscription Name00:07
- Module Introduction01:19
- Why do we Need Angular's Help?02:21
- Template-Driven (TD) vs Reactive Approach01:16
- An Example Form01:32
- TD: Creating the Form and Registering the Controls05:42
- TD: Submitting and Using the Form06:15
- TD: Understanding Form State02:29
- TD: Accessing the Form with @ViewChild03:03
- TD: Adding Validation to check User Input04:14
- Built-in Validators & Using HTML5 Validation00:25
- TD: Using the Form State04:27
- TD: Outputting Validation Error Messages02:23
- TD: Set Default Values with ngModel Property Binding02:15
- TD: Using ngModel with Two-Way-Binding02:48
- TD: Grouping Form Controls03:30
- TD: Handling Radio Buttons03:27
- TD: Setting and Patching Form Values04:42
- TD: Using Form Data04:44
- TD: Resetting Forms01:48
- Practicing Template-Driven Forms1 question
- Introduction to the Reactive Approach00:40
- Reactive: Setup02:26
- Reactive: Creating a Form in Code04:07
- Reactive: Syncing HTML and Form03:58
- Reactive: Submitting the Form02:15
- Reactive: Adding Validation02:50
- Reactive: Getting Access to Controls03:47
- Reactive: Grouping Controls03:46
- Fixing a Bug00:29
- Reactive: Arrays of Form Controls (FormArray)07:31
- Reactive: Creating Custom Validators06:11
- Reactive: Using Error Codes03:20
- Reactive: Creating a Custom Async Validator04:38
- Reactive: Reacting to Status or Value Changes02:11
- Reactive: Setting and Patching Values02:09
- Practicing Reactive Forms1 question
- [OPTIONAL] Assignment Solution13:48
- Introduction00:59
- TD: Adding the Shopping List Form03:42
- Adding Validation to the Form02:21
- Allowing the Selection of Items in the List04:24
- Loading the Shopping List Items into the Form03:02
- Updating existing Items03:15
- Resetting the Form02:34
- Allowing the the User to Clear (Cancel) the Form01:16
- Allowing the Deletion of Shopping List Items02:52
- Creating the Template for the (Reactive) Recipe Edit Form04:59
- Creating the Form For Editing Recipes05:06
- Syncing HTML with the Form03:52
- Fixing a Bug00:22
- Adding Ingredient Controls to a Form Array05:39
- Adding new Ingredient Controls03:38
- Validating User Input05:10
- Submitting the Recipe Edit Form07:30
- Adding a Delete and Clear (Cancel) Functionality04:47
- Redirecting the User (after Deleting a Recipe)01:47
- Adding an Image Preview01:48
- Providing the Recipe Service Correctly01:58
- Deleting Ingredients and Some Finishing Touches03:34
- Deleting all Items in a FormArray00:10
- Introduction & Why Pipes are Useful02:01
- Using Pipes03:28
- Parametrizing Pipes02:10
- Where to learn more about Pipes01:56
- Chaining Multiple Pipes02:20
- Creating a Custom Pipe06:42
- Parametrizing a Custom Pipe02:36
- Example: Creating a Filter Pipe07:01
- Pure and Impure Pipes (or: How to "fix" the Filter Pipe)04:46
- Understanding the "async" Pipe04:03
- Practicing Pipes1 question
- A New IDE00:43
- Module Introduction01:06
- How Does Angular Interact With Backends?03:09
- The Anatomy of a Http Request02:27
- Backend (Firebase) Setup02:35
- Sending a POST Request10:11
- GETting Data02:56
- Using RxJS Operators to Transform Response Data03:59
- Using Types with the HttpClient05:07
- Outputting Posts02:15
- Showing a Loading Indicator02:03
- Using a Service for Http Requests06:37
- Services & Components Working Together03:28
- Sending a DELETE Request03:27
- Handling Errors06:38
- Using Subjects for Error Handling02:39
- Using the catchError Operator02:13
- Error Handling & UX02:07
- Setting Headers03:18
- Adding Query Params04:01
- Observing Different Types of Responses07:32
- Changing the Response Body Type03:16
- Introducing Interceptors07:24
- Manipulating Request Objects02:32
- Response Interceptors02:46
- Multiple Interceptors04:52
- Wrap Up01:57
- Useful Resources & Links00:01
- Module Introduction00:55
- Backend (Firebase) Setup02:03
- Setting Up the DataStorage Service05:32
- Storing Recipes06:39
- Fetching Recipes05:38
- Transforming Response Data04:17
- Resolving Data Before Loading07:31
- Fixing a Bug with the Resolver01:43
- Module Introduction00:52
- How Authentication Works05:01
- Adding the Auth Page06:44
- Switching Between Auth Modes04:02
- Handling Form Input03:44
- Preparing the Backend03:15
- Make sure you got Recipes in your backend!00:13
- Preparing the Signup Request08:24
- Sending the Signup Request04:36
- Adding a Loading Spinner & Error Handling Logic06:34
- Improving Error Handling05:39
- Sending Login Requests06:00
- Login Error Handling04:28
- Creating & Storing the User Data10:17
- Reflecting the Auth State in the UI09:41
- Adding the Token to Outgoing Requests10:15
- Attaching the Token with an Interceptor08:48
- Adding Logout03:18
- Adding Auto-Login09:55
- Adding Auto-Logout07:39
- Adding an Auth Guard10:56
- Wrap Up01:55
- Useful Resources & Links00:02
- Module Introduction01:09
- Adding an Alert Modal Component07:33
- Understanding the Different Approaches02:28
- Using ngIf03:05
- Preparing Programmatic Creation08:27
- Creating a Component Programmatically04:46
- Understanding entryComponents05:06
- Data Binding & Event Binding05:05
- Wrap Up01:25
- Useful Resources & Links00:01
- Module Introduction00:41
- What are Modules?02:26
- Analyzing the AppModule06:24
- Getting Started with Feature Modules08:59
- Splitting Modules Correctly04:09
- Adding Routes to Feature Modules04:35
- Component Declarations01:58
- The ShoppingList Feature Module04:27
- Understanding Shared Modules08:10
- Understanding the Core Module05:48
- Adding an Auth Feature Module04:22
- Understanding Lazy Loading02:52
- Implementing Lazy Loading12:20
- More Lazy Loading03:02
- Preloading Lazy-Loaded Code03:39
- Modules & Services05:46
- Loading Services Differently08:24
- Useful Resources & Links00:01
- Module Introduction00:52
- Deployment Preparation & Steps03:18
- Using Environment Variables04:44
- Deploying Angular Applications00:14
- Deployment Example: Firebase Hosting09:01
- Server Routing vs Browser Routing00:06
- Module Introduction01:27
- Starting Setup & Why We Want Standalone Components04:48
- Building a First Standalone Component09:49
- Standalone Directives & Connecting Building Blocks02:19
- Migrating Another Component01:49
- A Standalone Root Component04:20
- Services & Standalone Components03:59
- Routing with Standalone Components06:36
- Lazy Loading08:04
- Summary01:56
- Module Introduction00:46
- What is Application State?09:07
- What is NgRx?06:55
- Getting Started with Reducers06:44
- Adding Logic to the Reducer05:47
- Understanding & Adding Actions06:16
- Setting Up the NgRx Store04:48
- Selecting State08:51
- Dispatching Actions07:09
- Multiple Actions09:37
- Preparing Update & Delete Actions03:08
- Updating & Deleting Ingredients10:59
- Expanding the State09:17
- Managing More State via NgRx09:52
- Removing Redundant Component State Management03:32
- First Summary & Clean Up05:25
- One Root State11:39
- Setting Up Auth Reducer & Actions08:25
- Dispatching Auth Actions05:53
- Auth Finished (For Now...)06:43
- And Important Note on Actions06:24
- Exploring NgRx Effects03:38
- Defining the First Effect05:19
- Effects & Error Handling08:57
- Login via NgRx Effects09:08
- Managing UI State in NgRx07:30
- Finishing the Login Effect07:11
- Preparing Other Auth Actions04:09
- Adding Signup06:17
- Further Auth Effects06:13
- Adding Auto-Login with NgRx08:16
- Adding Auto-Logout06:31
- Finishing the Auth Effects02:29
- Using the Store Devtools06:20
- The Router Store02:11
- Getting Started with NgRx for Recipes11:03
- Fetching Recipe Detail Data09:14
- Fetching Recipes & Using the Resolver12:00
- Fixing the Auth Redirect02:10
- Update, Delete and Add Recipes15:11
- Storing Recipes via Effects05:52
- Cleanup Work01:58
- Wrap Up02:12
- Alternative NgRx Syntax00:42
- Useful Resources & Links00:01
- Module Introduction03:04
- Angular Universal & ModuleMapLoader00:07
- Adding Angular Universal10:01
- Adding Angular Universal with NestJS06:18
- Deploying Universal Apps00:29
- Important: Remaining Lectures00:19
- Module Introduction01:40
- Getting Started with Angular Universal01:31
- Working on the App Module04:02
- Adding a Server-Side Build Workflow04:55
- Adding a NodeJS Server08:26
- Pre-Rendering the App on the Server04:11
- Next Steps01:38
- Angular Universal Gotchas00:31
- Making Animations Work with Angular 4+00:25
- Introduction01:03
- Setting up the Starting Project00:52
- Animations Triggers and State07:11
- Switching between States02:04
- Transitions01:54
- Advanced Transitions05:22
- Transition Phases03:57
- The "void" State06:11
- Using Keyframes for Animations05:32
- Grouping Transitions02:11
- Using Animation Callbacks02:38
- Module Introduction02:09
- Adding Service Workers12:41
- Caching Assets for Offline Use05:28
- Caching Dynamic Assets & URLs07:02
- Further Links & Resources00:02
- About this Section00:11
- Introduction01:18
- Why Unit Tests?02:12
- Analyzing the Testing Setup (as created by the CLI)08:01
- Running Tests (with the CLI)01:59
- Adding a Component and some fitting Tests06:14
- Testing Dependencies: Components and Services06:55
- Simulating Async Tasks09:41
- Using "fakeAsync" and "tick"02:14
- Isolated vs Non-Isolated Tests06:10
- Further Resources & Where to Go Next00:19
- Module Introduction01:44
- A Closer Look at "ng new"05:18
- IDE & Project Setup02:31
- Understanding the Config Files09:01
- Important CLI Commands05:25
- The "angular.json" File - A Closer Look11:18
- Angular Schematics - An Introduction01:41
- The "ng add" Command04:31
- Using Custom "ng generate" Schematics03:05
- Smooth Updating of Projects with "ng update"02:14
- Simplified Deployment with "ng deploy"05:46
- Understanding "Differential Loading"05:10
- Managing Multiple Projects in One Folder04:35
- Angular Libraries - An Introduction02:51
- Wrap Up01:27
- A First Look At Angular Elements15:34
- Thanks for being part of the course!00:55
- Bonus: More Content!00:20
- Module Introduction01:15
- What & Why?06:32
- Installing & Using TypeScript06:22
- Base Types & Primitives03:55
- Array & Object Types05:33
- Type Inference02:47
- Working with Union Types02:48
- Assigning Type Aliases02:42
- Diving into Functions & Function Types05:12
- Understanding Generics07:20
- Classes & TypeScript07:58
- Working with Interfaces05:36
- Configuring the TypeScript Compiler02:29
- Module Resources00:07
Online Courses
Learning Angular doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Angular related books and take your
YouTube videos
The number of high-quality and free Angular video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more