The Complete Angular Course: Beginner to Advanced
The most comprehensive Angular 4 (Angular 2+) course. Build a real e-commerce app with Angular, Firebase and Bootstrap 4
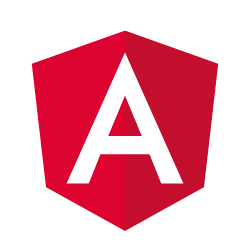
What you will learn?
- Establish yourself as a skilled professional developer
- Build real-world Angular applications on your own
- Troubleshoot common Angular errors
- Master the best practices
- Write clean and elegant code like a professional developer
Your trainer

Mosh Hamedani
Hi! My name is Mosh (Moshfegh) Hamedani! I'm a passionate software engineer with two decades of experience and I've taught over 3 million people who to code or how to become professional software engineers through my YouTube channel and online courses.
My goal is to make software engineering fun and accessible to everyone. That's why my courses are simple, pragmatic and free of BS.
376 lessons
Easy to follow lectures and videos covering subject details.
29.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction02:51
- What is Angular02:00
- Architecture of Angular Apps03:48
- Setting Up the Development Environment02:40
- Your First Angular App02:25
- Structure of Angular Projects06:54
- Webpack03:15
- Angular Version History03:34
- Angular Basics5 questions
- Course Structure03:46
- Making a Promise00:48
- Asking Questions00:33
- Introduction00:41
- What is TypeScript?02:24
- Your First TypeScript Program03:00
- Declaring Variables04:49
- Types05:43
- Type Assertions02:47
- Arrow Functions01:44
- Interfaces03:54
- Classes04:31
- Objects04:09
- Constructors02:52
- Access Modifiers02:56
- Access Modifiers in Constructor Parameters01:41
- Properties05:18
- Modules04:31
- Exercise00:58
- Solution05:09
- A Problem with the Current Implementation03:39
- Introduction00:19
- Building Blocks of Angular Apps03:41
- Components09:38
- Generating Components Using Angular CLI04:41
- Templates02:28
- Directives03:27
- Services04:29
- Dependency Injection07:20
- Generating Services Using Angular CLI02:11
- List of Authors1 question
- Introduction00:24
- Property Binding03:16
- Attribute Binding03:35
- Adding Bootstrap04:53
- Class Binding01:47
- Style Binding01:19
- Event Binding04:30
- Event Filtering01:50
- Template Variables01:53
- Two-way Binding08:05
- Pipes06:38
- Custom Pipes06:16
- Favorite Component1 question
- Title Casing1 question
- Introduction00:26
- Component API04:22
- Input Properties04:44
- Aliasing Input Properties04:22
- Output Properties03:22
- Passing Event Data05:51
- Aliasing Output Properties02:05
- Templates02:41
- Styles05:10
- View Encapsulation09:11
- ngContent04:56
- ngContainer02:34
- Like Component1 question
- Introduction00:31
- ngIf06:11
- Hidden Property03:25
- ngSwitchCase06:36
- ngFor04:18
- ngFor and Change Detection03:28
- ngFor and Trackby05:47
- The Leading Asterisk01:47
- ngClass01:51
- ngStyle02:31
- Safe Traversal Operator02:45
- Creating Custom Directives09:52
- Exercise: ZippyComponent1 question
- Introduction00:26
- Building a Bootstrap Form04:05
- Types of Forms03:21
- ngModel05:30
- Adding Validation03:17
- Specific Validation Errors04:20
- Styling Invalid Input Fields01:26
- Cleaner Templates01:52
- ngForm05:05
- ngModelGroup02:38
- Control Classes and Directives01:47
- Disabling the Submit Button01:08
- Working with Check Boxes02:18
- Working with Drop-down Lists06:02
- Working with Radio Buttons02:55
- Course Form1 question
- Introduction01:15
- Building a Bootstrap Form00:50
- Creating Controls Programmatically06:14
- Adding Validation07:07
- Specific Validation Errors02:33
- Implementing Custom Validation07:01
- Asynchronous Operations05:13
- Asynchronous Validators08:04
- Showing a Loader Image01:37
- Validating the Form Input Upon Submit04:58
- Nested FormGroups02:40
- FormArray08:43
- FormBuilder03:59
- Quick Recap01:16
- Change Password Form1 question
- Introduction01:03
- JSONPlaceHolder01:52
- Getting Data08:17
- Creating Data07:59
- Updating Data05:05
- Deleting Data01:59
- OnInit Interface03:54
- Separation of Concerns03:41
- Extracting a Service07:09
- Handling Errors03:21
- Handling Unexpected Errors02:27
- Handling Expected Errors04:28
- Throwing Application-specific Errors08:32
- Handling Bad Request Errors02:59
- Importing Observable Operators and Factory Methods03:08
- Global Error Handling07:03
- Extracting a Reusable Error Handling Method03:35
- Extracting a Reusable Data Service07:39
- The Map Operator04:27
- Optimistic vs Pessimistic Updates06:25
- Observables vs Promises06:44
- GitHub Followers Page1 question
- Introduction00:30
- Routing in a Nutshell01:04
- Configuring Routes06:47
- RouterOutlet02:29
- RouterLink05:41
- RouterLinkActive01:52
- Getting the Route Parameters05:23
- Why Route Parameters Are Observables08:57
- Routes with Multiple Parameters01:48
- Query Parameters04:28
- Subscribing to Multiple Observables04:45
- The SwitchMap Operator07:59
- Programmatic Navigation02:16
- Blog Archives1 question
- Introduction00:46
- Application Overview02:29
- Architecture03:02
- JSON Web Tokens05:47
- Starter Code08:02
- Implementing Login06:08
- Implementing Logout01:47
- Showing or Hiding Elements06:40
- Showing or Hiding Elements based on the User's Role04:15
- Getting the Current User00:49
- CanActivate Interface05:35
- Redirecting Users After Logging In04:34
- Protecting Routes Based on the User's Role05:45
- Accessing Protected API Resources07:27
- Quick Recap01:54
- Introduction00:36
- Preparing for Deployment05:36
- JIT vs AOT Compilation05:29
- Angular Compiler in Action03:34
- Building Applications with Angular CLI06:40
- Environments04:27
- Adding Custom Environments03:10
- Linting with Angular CLI04:45
- Linting in VSCode01:59
- Other Deployment Options03:59
- Deploying to GitHub Pages07:29
- Deploying to Firebase07:03
- Heroku01:44
- Deploying to Heroku08:04
- Engines01:28
- Exercise00:20
- Introduction01:16
- What is Firebase?02:20
- Your First Firebase Project00:41
- Working with Firebase Databases04:58
- Installing Firebase05:08
- Reading Lists07:17
- A Real-time Database01:32
- Observables and Memory Leaks02:53
- Unsubscribing from Subscriptions04:08
- Async Pipe02:40
- Reading an Object03:26
- As Keyword02:21
- Adding an Object08:00
- Updating an Object05:06
- Removing an Object02:12
- Additional Resources02:49
- Introduction00:44
- Examples of Animations02:23
- Different Ways to Create Animations04:32
- Angular Animations04:08
- Importing the Animations Module and Polyfills02:44
- Implementing a Fade-in Animation07:11
- Implementing a Fade-out Animation01:58
- States03:12
- Transitions02:10
- Animatable Properties00:06
- Creating Reusable Triggers02:12
- Build a re-usable slide animation1 question
- Easings05:34
- Keyframes05:27
- Creating Reusable Animations with animation()07:53
- Parameterizing Reusable Animations08:12
- Animation Callbacks02:45
- Querying Child Elements with query()05:32
- Animating Child Elements with animateChild()03:02
- Running Parallel Animations with group()03:01
- Staggering Animations with stagger()07:24
- Working with Custom States10:01
- Multi-step Animations02:16
- Separation of Concerns03:31
- Introduction00:42
- What is Angular Material04:31
- Installing Angular Material07:30
- Check Boxes04:28
- Radio Buttons05:43
- Selects05:07
- Inputs07:31
- Text Areas01:49
- Date Pickers09:37
- Icons03:40
- Buttons04:50
- Chips05:45
- Progress Spinners07:31
- Tooltips02:18
- Tabs02:24
- Dialogs08:39
- Passing Data to Dialogs10:47
- Other Components00:29
- Creating a Reusable Module05:51
- Themes04:20
- SASS08:46
- Creating a Custom Theme09:10
- Using Angular Material's Typography03:01
- Customizing Typography06:31
- An Important Note00:39
- Introduction00:34
- What is Redux06:33
- Building Blocks of Redux03:29
- Pure Functions04:13
- Installing Redux06:26
- Working with Actions07:56
- The Select Decorator06:25
- Avoiding State Mutation05:03
- Using Immutable.js05:41
- Exercise01:53
- Redux DevTools08:45
- Calling Backend APIs09:06
- Refactoring Fat Case Statements02:33
- Dealing with Complex Domains09:00
- Introduction01:12
- What is Automated Testing?07:27
- Types of Tests06:04
- Unit Testing Fundamentals07:44
- Working with Strings and Arrays03:14
- Set Up and Tear Down06:05
- Working with Forms03:30
- Working with Event Emitters02:51
- Working with Spies06:56
- Interaction Testing06:26
- Working with Confirmation Boxes03:09
- Limitations of Unit Tests01:31
- Code Coverage03:30
- Exercises00:34
- Introduction00:44
- The Setup04:41
- Generating the Setup Code04:53
- Testing Property Bindings07:55
- Testing Event Bindings04:59
- Providing Dependencies02:37
- Getting the Dependencies05:48
- Providing Stubs07:11
- Testing the Navigation04:02
- Dealing with Route Params06:33
- Testing RouterOutlet Components07:05
- Shallow Component Tests04:54
- Testing Attribute Directives04:30
- Dealing with Asynchronous Operations05:41
- Introduction04:57
- Challenge02:41
- Accessing the Source Code00:38
- Creating a New Project05:16
- Installing Bootstrap 404:54
- Extracting NavBar Component01:59
- Defining the Routes07:51
- Adding a Drop-down Menu06:44
- Cleaning Up the NavBar02:26
- Fixing a Few Minor Issues03:02
- Deployment02:51
- Introduction02:06
- Implementing Google Login08:27
- Implementing the Logout03:12
- Displaying the Current User02:20
- Using the Async Pipe04:42
- Extracting a Service07:57
- Protecting the Routes06:25
- Redirecting after Logging In08:27
- Storing Users in Firebase05:51
- Defining Admins05:47
- Protecting the Admin Routes11:13
- Showing or Hiding the Admin Links08:05
- Fixing a Bug01:32
- Introduction02:00
- Building a Bootstrap Form07:35
- Populating the Categories Drop-down List08:57
- Saving the Product in Firebase07:24
- Implementing Validation05:32
- Adding Custom Validation04:42
- Adding a Bootstrap Card07:10
- Displaying the List of Products04:05
- Editing a Product10:30
- Updating a Product04:09
- Deleting a Product03:59
- Searching for Products09:26
- Extracting an Interface01:20
- Adding a Data Table Component05:07
- Configuring the Data Table04:32
- Feeding the Data Table08:04
- Filtering with the Data Table03:18
- Fixing a Bug with Redirecting Users02:52
- Cleaning Up the Product Form01:36
- Introduction01:20
- Displaying All the Products07:54
- Displaying the Categories03:37
- Filtering Products by Category08:09
- Dealing with Multiple Asynchronous Operations04:54
- Refactoring: Extracting ProductFilterComponent07:54
- Refactoring: Extracting ProductCardComponent08:32
- Improving the Product Card02:31
- Making Categories Sticky02:24
- Wrap Up00:36
- Introduction02:59
- Creating a Shopping Cart08:59
- Refactoring: Moving the Responsibility to the Service07:38
- Adding a Product to the Shopping Cart07:28
- Refactoring the addToCart Method06:10
- Displaying the Quantity09:41
- Improving the Card Footer04:36
- Implementing the Change Quantity Buttons06:59
- Displaying the Number of Shopping Cart Items in NavBar09:10
- Refactoring: Creating a Rich Model09:03
- Building the Shopping Cart Page06:11
- Fixing a Design Issue04:56
- Displaying the Total Price05:43
- Refactoring: Extracting ProductQuantityComponents08:33
- Discovering a Design Issue06:18
- Flattening Shopping Cart Item Objects08:40
- Better Object Initialization04:47
- Clearing the Shopping Cart03:21
- Fixing a Bug with Updating the Quantity01:36
- Improving the Layout02:41
- Adding a Thumbnail03:20
- More Refactoring08:12
- Introduction02:00
- Adding the Check Out Button02:43
- Building a Shipping Form01:14
- Saving the Order in Firebase08:39
- Associating the Order with the Current User02:10
- Refactoring: Extract an Order Model04:29
- Redirecting the User to the Order Success Page02:54
- Clearing the Shopping Cart02:52
- Transactions00:27
- Adding an Order Summary Widget08:47
- Refactoring: Extract ShippingFormComponent09:15
- Displaying the Orders03:07
- Fixing a Bug01:12
- What about Processing Payments?01:18
- Introduction01:02
- Modules05:54
- Essential TypeScript Plugins04:12
- Moving Files and Folders05:35
- Creating the Shared Module05:55
- Creating the Admin Module09:27
- Creating the Shopping Module04:52
- Creating the Core Module05:26
- Importing and Exporting Modules06:22
- Adding Icons02:29
- Aligning the NavBar Items03:34
- Defining a Theme05:38
- Coupon to My Other Courses00:20
Online Courses
Learning Angular doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Angular related books and take your
YouTube videos
The number of high-quality and free Angular video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more