Advanced React and Redux
Walkthroughs on advanced React v16.6.3 and Redux v4.0.0 - Authentication, Testing, Middlewares, HOC's, and Deployment
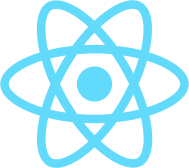
What you will learn?
- Build a scaleable API with authentication using Express, Mongo, and Passport
- Learn the differences between cookie-based and token-based authentication
- Figure out what a Higher Order Component and how to use it to write dramatically less code
- Write Redux middleware from scratch to uncover what is happening behind the scenes with Redux
- Set up your own testing environment with Jest and Enzyme
- Realize the power of building composable components
Your trainer

Stephen Grider
Stephen Grider has been building complex Javascript front ends for top corporations in the San Francisco Bay Area. With an innate ability to simplify complex topics, Stephen has been mentoring engineers beginning their careers in software development for years, and has now expanded that experience onto Udemy, authoring the highest rated React course. He teaches on Udemy to share the knowledge he has gained with other software engineers. Invest in yourself by learning from Stephen's published courses.
240 lessons
Easy to follow lectures and videos covering subject details.
21 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction01:46
- Course Resources00:38
- Join Our Community!00:07
- npx Create React App Generation00:21
- Project Generation02:05
- Our First Test03:51
- Introduction to Jest03:43
- App Overview02:01
- Installing Dependencies00:44
- React and Redux Design02:44
- What Do We Test?04:24
- Starting from Scratch02:48
- Rendering the App04:07
- Showing Components in the App03:16
- Valid Test File Names03:31
- Test Structure03:56
- Tricking React with JSDOM07:52
- Verifying Component Existence04:11
- Test Expectations04:11
- Limiting Test Knowledge06:13
- Enzyme Setup07:25
- Enzyme Renderers06:19
- Expectations for Component Instances07:06
- Exercise Time!01:18
- Expecting the Comment List01:56
- Update for Handling Absolute Imports00:22
- Absolute Path Imports05:00
- Code Reuse with BeforeEach04:25
- Comment Box Component03:16
- TextArea Implementation05:08
- CommentBox Test File03:22
- Getting an Empty ReactWrapper in your console.log?00:10
- Asserting Element Existence06:48
- AfterEach Statements04:02
- Simulating Change Events05:15
- Providing Fake Events06:35
- Forcing Component Updates03:55
- Retrieving Prop Values04:19
- Form Submit Exercise02:08
- Exercise Solution06:41
- Describe Statements06:18
- Redux Setup04:30
- The Provider Tag05:00
- The SaveComment Action Creator04:11
- Bonding React with Redux03:57
- Redux Test Errors06:56
- Adding a Root Component07:36
- Testing Reducers05:21
- Handling Unknown Types02:46
- Testing Action Creators05:31
- Comment List Wireup04:34
- Getting Data Into Redux04:55
- Redux Initial State07:51
- Cheerio Queries08:29
- One More Feature03:51
- Fetching a Remote Resource04:37
- Parsing Comment List05:08
- Integration Tests03:31
- Integration Tests in Action03:03
- Fixing a Broken Test02:30
- Simulating Button Clicks03:04
- Why the Failure?04:32
- Faking Requests with Moxios05:58
- The Reason for Failure02:47
- Introducing a Pause06:55
- Moxios's Wait Function02:43
- App Wrapup02:01
- An Introduction to Higher Order Components01:42
- Connect - A Higher Order Component01:58
- App Overview04:30
- Adding React Router01:45
- Adding Routes03:34
- Auth Reducer05:04
- Rendering a Header04:05
- Wiring Up State04:18
- Changing Auth State03:47
- Steps for Building a HOC06:29
- Forced Navigation with React Router04:27
- Creating the HOC10:06
- Placing Reusable Logic03:26
- Passing Through Props09:10
- Introduction to Middlewares02:30
- The Purpose of Redux Promise09:04
- How Async Middlewares Work08:33
- Crazy Middleware Syntax08:42
- Forwarding Actions04:06
- Waiting for Promise Resolution05:57
- Observing the Middleware07:02
- State Validation Middleware04:34
- JSON Schema05:13
- Generating JSON Schema04:13
- Middleware Creation06:08
- Emitting Warnings05:52
- Introduction to Authentication04:32
- Cookies vs Tokens04:57
- Scalable Architecture04:49
- Server Setup03:45
- More Server Setup05:41
- Express Middleware06:29
- Express Route Handler06:09
- Mongoose Models10:22
- MongoDB Setup06:29
- Inspecting the Database06:19
- Authentication Controller04:57
- Searching for Users07:57
- Creating User Records06:31
- Encrypting Passwords with Bcrypt08:12
- Salting a Password06:16
- JWT Overview04:58
- Creating a JWT07:13
- Installing Passport05:50
- Passport Strategies08:55
- Using Strategies with Passport04:02
- Making an Authenticated Request05:55
- Signing in with Local Strategy05:37
- Purpose of Local Strategy04:17
- Bcrypt Full Circle05:08
- Protecting Signin Route04:12
- Signing Users In04:04
- Server Review02:39
- Client Overview03:25
- Lib Installs01:49
- The App Component03:51
- Creating the Header06:02
- Wiring Up React Router05:23
- Scaffolding the Signup Form04:37
- Including Redux07:45
- ReduxForm for Signup06:27
- Handling Form Submission05:05
- Wiring Up Middleware02:16
- Basics of Redux Thunk09:20
- Calling the API05:01
- Code Cleanup with Compose05:50
- CORS in a Nutshell11:21
- Solution to CORS Errors05:26
- Dispatching Actions04:04
- Displaying Auth Errors05:16
- Redirect on Signup02:37
- Feature Component02:28
- The Require Auth HOC04:02
- Reference - HOC Code00:12
- Persisting Login State04:54
- Signing Out a User04:20
- Automatic Sign Out02:26
- The Signin Component02:14
- The Signin Action Creator02:35
- Updating the Header03:20
- Header Styling03:09
- Auth Wrapup02:55
- Note00:08
- Project Setup03:29
- A First Spec12:42
- Core Testing - Describe, It, Expect09:39
- Purpose of Describe, It, and Expect3 questions
- Test Reporting04:05
- Test Structure2 questions
- Feature Mockups06:09
- Test Structure Setup06:55
- Comment Box Tests08:19
- Testing Class Names07:50
- Using beforeEach to Condense Tests06:22
- Expecting Child Elements05:13
- Simulating Events07:24
- Testing Controlled Components07:23
- Form Submit Event07:13
- Stub Comment List06:00
- Expectations on Content07:43
- Assertions with Lists05:42
- Testing Action Creators09:34
- Action Creator Shortcuts04:47
- TDD Comments Reducer12:37
- Spec Failures After Code Change03:36
- App Review03:07
- Purpose of Chai and Mocha05:19
- Test Helper From Scratch02:43
- JSDom Setup07:14
- More JSDom Setup05:28
- TestUtils Library07:53
- Defining RenderComponent07:52
- Finishing RenderComponent Helper05:12
- Simulate Helper07:09
- Test Helper Review03:08
- What is a Higher Order Component?03:06
- Connect and Provider05:49
- Authentication HOC Overview04:19
- Header Setup06:54
- React Router Setup07:36
- Authentication Reducer07:05
- Action Creator Hookup08:04
- Authentication Higher Order Component04:57
- HOC Scaffold Code09:09
- Nesting Higher Order Components04:48
- Accessing React Router on Context05:30
- Class Level Properties02:12
- Handling HOC Edge Cases05:03
- Higher Order Components Review03:05
- Middleware Overview03:26
- App Building Plan03:50
- Users Reducer04:14
- Static Users Action Creator02:15
- Rendering a List of Users07:32
- CSS Cleanup04:20
- Pains Without Middleware07:15
- Middleware Stack04:43
- Middleware Internals07:55
- Handling Unrelated Actions05:17
- Handling Promises08:05
- Middleware Review06:50
- Client Setup02:28
- App Architecture05:48
- Component and State Design07:30
- Header Component04:17
- Scaffolding the Signin Form11:03
- Adding Signin Form05:58
- Action Creator with Many Responsibilities08:22
- Introducing Redux Thunk07:10
- Signin Action Creator10:38
- CORS In a Nutshell09:13
- Serverside Solution for CORS06:16
- Programmatic Navigation07:11
- Updating Auth State06:29
- Breather and Review05:39
- LocalStorage and JWT06:39
- Auth Error Messaging04:36
- Displaying Errors04:40
- Header Logic10:29
- Signout Component05:32
- Signout Action Creator04:45
- Signup Component04:12
- Signup Form Scaffolding08:02
- Redux Form Validation04:30
- Implementing Validation Logic09:28
- More On Validation05:40
- Signup Action Creator07:11
- Finish Up Signup08:55
- Securing Individual Routes10:21
- Root IndexRoute02:34
- Automatically Authenticating Users07:11
- Making Authenticated API Requests08:01
- Handling Data from Authenticated Requests05:13
- Authentication Wrapup03:29
- Bonus!00:29
Online Courses
Learning React doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of React related books and take your
YouTube videos
The number of high-quality and free React video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more