Master Microservices with Spring Boot and Spring Cloud
Java Spring Boot Microservices 5-in-1 - Spring Boot, Spring Cloud, Docker, Kubernetes and REST API (REST Web Services)
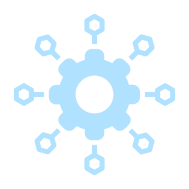
What you will learn?
- Develop and design REST API and REST WEB SERVICES with Spring Boot
- Develop MICROSERVICES with Spring Boot and Spring Cloud
- Orchestrate microservices with KUBERNETES
- Create containers for microservices with DOCKER
- IMPLEMENT Exception Handling, Validation, HATEOAS and filtering for RESTful Web Services.
- Implement client side load balancing (Ribbon), Dynamic scaling(Eureka Naming Server) and an API Gateway (Zuul)
- You will setup Centralized Microservices Configuration with Spring Cloud Config Server
- You will learn to implement Distributed tracing for microservices with Spring Cloud Sleuth and Zipkin
- You will implement Fault Tolerance for microservices with Hystrix
- You will understand how to version your RESTful Web Services
- You will understand how to monitor RESTful Services with Spring Boot Actuator
- You will understand how to document RESTful Web Services with Swagger
- You will understand the best practices in designing RESTful web services
- Using Spring Cloud Bus to exchange messages about Configuration updates
- Simplify communication with other Microservices using Feign REST Client
Your trainer

in28Minutes Official
Ranga is an AWS Certified Solutions Architect Associate.
We are teaching 30+ Courses to 450K Learners on DevOps, Cloud ( AWS, PCF, GCP, and Azure ), Full Stack ( React, Angular ), Java Programming, and Java Frameworks ( Spring, Spring Boot, Spring MVC, Hibernate ).
We use Problem-Solution based Step-By-Step Hands-on Approach With Practical, Real-World Application Examples.
We have a wide range of courses focused on Spring Boot - Creating APIs and Microservices, Deploying to Cloud ( AWS, Azure, Docker, Kubernetes, Azure ), and Integrating with Full Stack Front end frameworks ( React & Angular ).
260 lessons
Easy to follow lectures and videos covering subject details.
22 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Microservices and RESTful APIs with Spring Boot and Spring Cloud - Preview03:36
- DO NOT SKIP: Success Stories of Other Learners00:54
- Introduction to the Course & Course Guide02:33
- A surprise! New Course Updates00:51
- What is a Web Service?06:08
- Important How Questions related to Web Services06:41
- Web Services - Key Terminology04:13
- Introduction to SOAP Web Services04:40
- Introduction to RESTful Web Services07:33
- SOAP vs RESTful Web Services02:20
- DO NOT SKIP: New to Spring Boot?00:25
- Step 00 - Creating a REST API with Spring Boot - An Overview04:04
- CODE BACKUP FILES and STEP BY STEP CHANGES : For Reference00:10
- Step 01 - Initializing a REST API Project with Spring Boot07:21
- Step 02 - Creating a Hello World REST API with Spring Boot06:21
- Step 03 - Enhancing the Hello World REST API to return a Bean04:30
- Step 04 - What's happening in the background? Spring Boot Starters & Autoconfign09:06
- Step 05 - Enhancing the Hello World REST API with a Path Variable04:13
- Step 06 - Designing the REST API for Social Media Application07:28
- Step 07 - Creating User Bean and UserDaoService06:43
- Resources for Next Step00:06
- Step 08 - Implementing GET Methods for User Resource08:28
- Step 09 - Implementing POST Method to create User Resource08:36
- Step 10 - Enhancing POST Method to return correct HTTP Status Code and Location11:24
- Step 11 - Implementing Exception Handling - 404 Resource Not Found07:49
- Resources for Next Step00:30
- Step 12 - Implementing Generic Exception Handling for all Resources11:29
- Step 13 - Implementing DELETE Method to delete a User Resource03:25
- Step 14 - Implementing Validations for REST API11:47
- Step 15 - Overview of Advanced REST API Features01:12
- Step 16 - Understanding Open API Specification and Swagger04:57
- springdoc-openapi Dependency for next lecture00:08
- Step 17 - Configuring Auto Generation of Swagger Documentation09:53
- Step 18 - Exploring Content Negotiation - Implementing Support for XML05:56
- Step 19 - Exploring Internationalization for REST API09:39
- Resources for Next Step00:16
- Step 20 - Versioning REST API - URI Versioning09:01
- Step 21 - Versioning REST API - Request Param, Header and Content Negotiation11:12
- Step 22 - Implementing HATEOAS for REST API10:43
- Step 23 - Implementing Static Filtering for REST API09:54
- Step 24 - Implementing Dynamic Filtering for REST API09:26
- Step 25 - Monitoring APIs with Spring Boot Actuator06:54
- Step 26 - Exploring APIs with Spring Boot HAL Explorer04:54
- DO NOT SKIP - New to JPA and Hibernate?00:14
- Step 27 - Connecting REST API to H2 using JPA and Hibernate - An Overview01:17
- Step 28 - Creating User Entity and some test data05:26
- Step 29 - Enhancing REST API to connect to H2 using JPA and Hibernate10:35
- Step 30 - Creating Post Entity with Many to One Relationship with User Entity10:22
- Step 31 - Implementing a GET API to retrieve all Posts of a User03:13
- Step 32 - Implementing a POST API to create a Post for a User06:21
- Step 33 - Exploring JPA and Hibernate Queries for REST API06:36
- Step 34 - Connecting REST API to MySQL Database - An Overview01:06
- Step 34z - OPTIONAL - Installing Docker04:25
- Resources for Next Step00:19
- Step 35 - OPTIONAL - Connecting REST API to MySQL Database - Implementation15:19
- Step 36 - Implementing Basic Authentication with Spring Security05:18
- Step 37 - Enhancing Spring Security Configuration for Basic Authentication08:45
- Section Introduction - Microservices with Spring Cloud01:25
- Step 00 - 01 - Introduction to Microservices04:24
- Step 00 - 02 - Challenges with Microservices05:51
- Step 00 - 03 - Introduction to Spring Cloud07:48
- Step 00 - 04 - Advantages of Microservices Architectures02:46
- Step 00 - 05 - Microservice Components - Standardizing Ports and URL02:37
- Next Section uses Latest Version of Spring Boot01:29
- Step 01 - Part 1 - Intro to Limits Microservice and Spring Cloud Config Server04:51
- Step 01 - Part 2 - Setting up Limits Microservice05:34
- COURSE UPDATE : Limits service with >=2.4.0 of SPRING BOOT00:07
- Step 02 - Creating a hard coded limits service04:59
- Step 03 -Enhance limits service to get configuration from application properties05:14
- Step 04 - Setting up Spring Cloud Config Server03:55
- Step 05 - Installing Git01:45
- Step 06 - Creating Local Git Repository04:32
- Step 07 - Connect Spring Cloud Config Server to Local Git Repository05:11
- Step 08 - Configuration for Multiple Environments in Git Repository06:18
- Step 09 - Connect Limits Service to Spring Cloud Config Server04:58
- Debugging problems with Spring Cloud Config Server00:12
- Step 10 - Configuring Profiles for Limits Service05:44
- Step 11 - A review of Spring Cloud Config Server04:16
- Step 12 - Introduction to Currency Conversion and Currency Exchange Microservice03:14
- IMPORTANT : SPRING BOOT AND SPRING CLOUD VERSIONS00:07
- Step 13 - Setting up Currency Exchange Microservice03:35
- Step 14 - Create a simple hard coded currency exchange service06:29
- Step 15 - Setting up Dynamic Port in the the Response07:10
- Step 16 - Configure JPA and Initialized Data07:56
- Step 17 - Create a JPA Repository05:16
- Step 18 - Setting up Currency Conversion Microservice02:16
- Step 19 - Creating a service for currency conversion06:58
- Step 20 - Invoking Currency Exchange Microservice from Currency Conversion Micro08:15
- Step 21 - Using Feign REST Client for Service Invocation11:30
- Step 22 - Setting up client side load balancing with Ribbon05:11
- COURSE UPDATE : Ribbon DOES NOT work with Spring Boot 2.400:17
- Step 23 - Running client side load balancing with Ribbon04:39
- Debugging problems with Feign and Ribbon00:08
- Step 24 - Understand the need for a Naming Server03:21
- Step 25 - Setting up Eureka Naming Server05:25
- Step 26 - Connecting Currency Conversion Microservice to Eureka03:06
- Step 27 - Connecting Currency Exchange Microservice to Eureka03:02
- COURSE UPDATE : Exclude dependency on jackson-dataformat-xml00:14
- Step 28 - Distributing calls using Eureka and Ribbon06:35
- Debugging Problems with Naming Server ( Eureka ) and Ribbon00:08
- Step 29 - A review of implementing Eureka, Ribbon and Feign03:58
- Step 30 - Introduction to API Gateways02:50
- Step 31 - Setting up Zuul API Gateway04:36
- Step 32 - Implementing Zuul Logging Filter06:01
- Step 33 - Executing a request through Zuul API Gateway05:27
- Step 34 - Setting up Zuul API Gateway between microservice invocations07:16
- Debugging Problems with Zuul API Gateway00:08
- Step 35 - Introduction to Distributed Tracing03:26
- Step 36 - Implementing Spring Cloud Sleuth08:34
- Step 37 - Introduction to Distributed Tracing with Zipkin04:34
- Step 38 - Installing Rabbit MQ04:31
- Updates to Step 39 - Running Zipkin on Windows00:24
- Step 39 - Setting up Distributed Tracing with Zipkin07:06
- Step 40 - Connecting microservices to Zipkin03:35
- Updates to Step 40 : Use spring-cloud-starter-zipkin and spring-rabbit00:16
- Step 41 - Using Zipkin UI Dashboard to trace requests06:33
- Debugging Problems with Zipkin00:08
- Step 42 - Understanding the need for Spring Cloud Bus09:16
- Step 43 - Implementing Spring Cloud Bus05:01
- Step 44 - Fault Tolerance with Hystrix07:10
- What's NEW in V2?01:17
- Have you already completed V1?01:06
- Course Downloads00:09
- Step 01 - Setting up Limits Microservice - V205:59
- CODE BACKUP FILES and STEP BY STEP CHANGES : For Reference00:11
- Step 02 - Creating a hard coded limits service - V205:28
- Step 03 - Enhance limits service - Get configuration from application props - V204:24
- Step 04 - Setting up Spring Cloud Config Server - V204:45
- Step 05 - Installing Git and Creating Local Git Repository - V204:11
- Debugging problems with Spring Cloud Config Server - V200:08
- Step 06 - Connect Spring Cloud Config Server to Local Git Repository - V203:57
- Step 07 - Connect Limits Service to Spring Cloud Config Server - V204:07
- Step 08 - Configuring Profiles for Limits Service - V205:47
- Debugging Guide for Microservices V2 + Docker + Docker Compose02:53
- Step 09 - Introduction to Currency Conversion & Exchange Microservices - V202:26
- Step 10 - Setting up Currency Exchange Microservice - V204:09
- URL and Response Structure for Currency Exchange Service00:04
- Step 11 - Create a simple hard coded currency exchange service - V207:41
- Step 12 - Setting up Dynamic Port in the the Response - V205:39
- Step 13 - Configure JPA and Initialized Data - V210:56
- CODE BACKUP FILES and STEP BY STEP CHANGES : For Reference00:11
- Step 14 - Create a JPA Repository - V205:47
- Step 15 - Setting up Currency Conversion Microservice - V202:25
- URL and Response Structure for Currency Conversion Service00:06
- Step 16 - Creating a service for currency conversion - V207:19
- Step 17 - Invoking Currency Exchange from Currency Conversion Microservice - V207:10
- Step 18 - Using Feign REST Client for Service Invocation - V207:59
- Step 19 - Understand Naming Server and Setting up Eureka Naming Server - V207:37
- Debugging Problems with Eureka - V200:14
- Step 20 - Connect Currency Conversion & Currency Exchange Microservices - V204:54
- Step 21 - QuickStart by Importing Microservices05:18
- Step 22 - Load Balancing with Eureka, Feign & Spring Cloud LoadBalancer - V204:34
- Step 22 - Setting up Spring Cloud API Gateway04:45
- URLs for next Lecture00:08
- Step 23 - Enabling Discovery Locator with Eureka for Spring Cloud Gateway07:14
- Debugging Problems with Spring Cloud Gateway - V200:14
- Step 24 - Exploring Routes with Spring Cloud Gateway13:39
- Step 25 - Implementing Spring Cloud Gateway Logging Filter05:55
- Step 26 - Getting started with Circuit Breaker - Resilience4j07:26
- Step 27 - Playing with Resilience4j - Retry and Fallback Methods12:06
- Step 28 - Playing with Circuit Breaker Features of Resilience4j08:47
- Update to Step 29 - Change in Configuration00:02
- Step 29 - Exploring Rate Limiting and BulkHead Features of Resilience4j - V205:38
- Step 00 - Match made in Heaven - Docker and Microservices02:44
- Step 01 - Installing Docker - Docker - V204:25
- RECOMMENDATION : Use PowerShell in Windows!00:16
- Step 02 - Your First Docker Usecase - Deploy a Spring Boot Application02:58
- Step 03 - Docker Concepts - Registry, Repository, Tag, Image and Containers06:18
- Step 04 - Playing with Docker Images and Containers06:28
- Step 05 - Understanding Docker Architecture - Docker Client, Docker Engine04:11
- Step 06 - Why is Docker Popular03:02
- Step 07 - Playing with Docker Images08:26
- Step 08 - Playing with Docker Containers10:28
- Step 09 - Playing with Docker Commands - stats, system07:58
- Step 10 - Introduction to Distributed Tracing01:47
- Step 11 - Launching Zipkin Container using Docker03:05
- Step 12 - Connecting Currency Exchange Microservice with Zipkin08:57
- Step 13 - Connecting Currency Conversion Microservice & API Gateway with Zipkin05:55
- Link for the Next Lecture00:03
- Step 14 - Getting Setup with Microservices for Creating Container Images04:48
- Step 15 - Creating Container Image for Currency Exchange Microservice09:36
- Step 16 - Getting Started with Docker Compose - Currency Exchange Microservice06:28
- Step 17 - Running Eureka Naming Server with Docker Compose07:10
- Step 18 - Running Currency Conversion Microservice with Docker Compose04:15
- Step 19 - Running Spring Cloud API Gateway with Docker Compose03:49
- Debugging Problems with Docker Compose00:17
- Step 20 - Running Zipkin with Docker Compose04:51
- Step 21 - Running Zipkin and RabbitMQ with Docker Compose07:28
- Step 00 - Docker, Kubernetes and Microservices - Made for each other03:19
- Step 01 - Getting Started with Docker, Kubernetes and Google Kubernetes Engine10:57
- Step 02 - Creating Google Cloud Account03:54
- Step 03 - Creating Kubernetes Cluster with Google Kubernete Engine (GKE)07:20
- Step 04 - Review Kubernetes Cluster and Learn Few Fun Facts about Kubernetes04:11
- Step 05 - Deploy Your First Spring Boot Application to Kubernetes Cluster11:39
- Commands executed in this section01:39
- Step 06 - Quick Look at Kubernetes Concepts - Pods, Replica Sets and Deployment04:58
- Step 07 - Understanding Pods in Kubernetes06:34
- Step 08 - Understanding ReplicaSets in Kubernetes08:40
- Step 09 - Understanding Deployment in Kubernetes13:51
- Step 10 - Quick Review of Kubernetes Concepts - Pods, Replica Sets & Deployments03:22
- Step 11 - Understanding Services in Kubernetes07:56
- Step 12 - Quick Review of GKE on Google Cloud Console03:15
- Step 13 - Understanding Kubernetes Architecture - Master Node and Nodes08:13
- Installing Gcloud05:15
- Installing Kubectl02:44
- Link for the Next Lecture00:03
- Step 14 - Setup Currency Exchange & Conversion Microservices - Kubernetes08:59
- Step 15 - Container images for Exchange & Currency Conversion Microservices05:39
- Step 16 - Deploy Microservices to Kubernetes & Understand Service Discovery09:48
- Step 17 - Creating Declarative Configuration Kubernetes YAML for Microservices07:48
- Step 18 - Clean up Kubernetes YAML for Microservices06:51
- Step 19 - Enable Logging and Tracing APIs in Google Cloud Platform01:41
- Step 20 - Deploying Microservices using Kubernetes YAML Configuration04:17
- Step 21 - Playing with Kubernetes Declarative YAML Configuration03:46
- Step 22 - Creating Environment Variables to enable Microservice Communication10:03
- Step 23 - Understanding Centralized Configuration in Kubernetes - Config Maps06:31
- Step 24 - Exploring Centralized Logging and Monitoring in GKE05:41
- Step 25 - Exploring Microservices Deployments with Kubernetes07:49
- Step 26 - Configuring Liveness and Readiness Probes for Microservices with K8S08:47
- Step 27 - Autoscaling Microservices with Kubernetes06:30
- Step 28 - Delete Kubernetes Cluster and Thank You!01:02
- Do You Want To Help Us?00:16
- DO NOT SKIP - I Would Love To Congratulate You!00:35
- Thank You01:09
- Bonus Lecture00:18
- Step 01 - Getting Started with Spring Boot - Goals01:32
- Step 02 - Understanding the World Before Spring Boot - 10000 Feet Overview03:16
- Step 03 - Setting up New Spring Boot Project with Spring Initializr07:17
- Step 04 - Build a Hello World API with Spring Boot08:58
- Step 05 - Understanding the Goal of Spring Boot02:45
- Step 06 - Understanding Spring Boot Magic - Spring Boot Starter Projects04:54
- Step 07 - Understanding Spring Boot Magic - Auto Configuration07:41
- Step 08 - Build Faster with Spring Boot DevTools04:11
- Step 09 - Get Production Ready with Spring Boot - 1 - Profiles07:05
- Step 10 - Get Production Ready with Spring Boot - 2 - ConfigurationProperties07:31
- Step 11 - Get Production Ready with Spring Boot - 3 - Embedded Servers06:27
- Step 12 - Get Production Ready with Spring Boot - 4 - Actuator07:33
- Step 13 - Understanding Spring Boot vs Spring vs Spring MVC03:55
- Step 14 - Getting Started with Spring Boot - Review00:38
- Step 01 - Getting Started with JPA and Hibernate - Goals01:24
- Step 02 - Setting up New Spring Boot Project for JPA and Hibernate06:52
- Step 03 - Launching up H2 Console and Creating Course Table in H207:24
- Step 04 - Getting Started with Spring JDBC03:58
- Step 05 - Inserting Hardcoded Data using Spring JDBC07:49
- Step 06 - Inserting and Deleting Data using Spring JDBC09:01
- Step 07 - Querying Data using Spring JDBC05:36
- Step 08 - Getting Started with JPA and EntityManager12:23
- Step 09 - Exploring the Magic of JPA03:16
- Step 10 - Getting Started with Spring Data JPA05:41
- Step 11 - Exploring features of Spring Data JPA05:11
- Step 12 - Understanding difference between Hibernate and JPA05:00
- Step 00 - Introduction to Functional Programming - Overview01:21
- Step 01 - Getting Started with Functional Programming with Java06:23
- Step 02 - Writing Your First Java Functional Program05:39
- Step 03 - Improving Java Functional Program with filter08:07
- Step 04 - Using Lambda Expression to enhance your Functional Program05:17
- Step 05 - Do Functional Programming Exercises with Streams, Filters and Lambdas05:30
- Step 06 - Using map in Functional Programs - with Exercises05:54
- Step 07 - Understanding Optional class in Java07:14
- Step 08 - Quick Review of Functional Programming Basics03:14
Online Courses
Learning Microservices doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Microservices related books and take your
YouTube videos
The number of high-quality and free Microservices video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more