Learn and Understand NodeJS
Dive deep under the hood of NodeJS. Learn V8, Express, the MEAN stack, core Javascript concepts, and more.
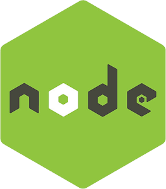
What you will learn?
- Grasp how NodeJS works under the hood
- Understand the Javascript and technical concepts behind NodeJS
- Structure a Node application in modules
- Understand and use the Event Emitter
- Understand Buffers, Streams, and Pipes
- Build a Web Server in Node and understand how it really works
- Use npm and manage node packages
- Build a web application and API more easily using Express
- Connect to a SQL or Mongo database in Node
- Understand how the MEAN stack works
- Be the coder that explains NodeJS to everyone else because you understand it better than anyone else
Your trainer

Anthony Alicea
Tony has been programming since he was 12 years old, and got into web sites and web application development at 16. After graduating with a Computer Science degree from Case Western Reserve University, Tony continued with that interest as a Microsoft certified software application developer and architect, database designer, and user interface designer.
His experience has ranged across technologies such as HTML5, CSS3, ASP .NET MVC, JavaScript, jQuery, KnockoutJS, AngularJS, NodeJS, LESS, Bootstrap, SQL, Entity Framework and more.
He believes strongly that deeply understanding any topic allows you to properly learn it and, even more importantly in a real-world environment, quickly overcome problems.
97 lessons
Easy to follow lectures and videos covering subject details.
13 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction and the Goal of this Course04:33
- Big Words and NodeJS01:13
- Watching this Course in High Definition00:50
- Conceptual Aside: The Command Line Interface09:49
- Command Line References00:18
- Conceptual Aside: Processors, Machine Language, and C++10:07
- Javascript Aside: Javascript Engines and The ECMAScript Specification04:01
- V8 Under the Hood05:57
- Adding Features to Javascript15:04
- Conceptual Aside: Servers and Clients06:45
- What Does Javascript Need to Manage a Server?02:18
- The C++ Core05:51
- The Javascript Core03:04
- Downloading Lecture Source Code00:33
- Let's Install and Run Some Javascript in Node16:47
- Conceptual Aside: Modules02:21
- Javascript Aside: First-Class Functions and Function Expressions12:03
- Let's Build a Module10:54
- Javascript Aside: Objects and Object Literals06:55
- Javascript Aside: Prototypal Inheritance and Function Constructors11:38
- Javascript Aside: By Reference and By Value05:44
- Javascript Aside: Immediately Invoked Function Expressions (IIFEs)07:42
- How Do Node Modules Really Work?: module.exports and require17:33
- Javascript Aside: JSON01:40
- More on require11:39
- Module Patterns19:12
- exports vs module.exports10:02
- Requiring Native (Core) Modules06:51
- Modules and ES602:36
- Web Server Checklist01:21
- Conceptual Aside: Events05:24
- Javascript Aside: Object Properties, First Class Functions, and Arrays05:08
- The Node Event Emitter - Part 113:58
- The Node Event Emitter - Part 211:58
- Javascript Aside: Object.create and Prototypes06:18
- Inheriting From the Event Emitter14:40
- Javascript Aside: Node, ES6, and Template Literals07:55
- Javascript Aside: .call and .apply03:40
- Inheriting From the Event Emitter - Part 209:43
- Javascript Aside: ES6 Classes08:43
- Inheriting From the Event Emitter - Part 306:00
- Javascript Aside: Javascript is Synchronous02:38
- Conceptual Aside: Callbacks01:29
- libuv, The Event Loop, and Non-Blocking Asynchronous Execution11:39
- Conceptual Aside: Streams and Buffers04:31
- Conceptual Aside: Binary Data, Character Sets, and Encodings11:09
- Buffers06:48
- ES6 Typed Arrays04:52
- Javascript Aside: Callbacks04:03
- Files and fs15:52
- Streams18:14
- Conceptual Aside: Pipes02:14
- Pipes15:51
- Web Server Checklist02:37
- Conceptual Aside: TCP/IP07:55
- Conceptual Aside: Addresses and Ports03:11
- Conceptual Aside: HTTP06:06
- http_parser06:56
- Let's Build a Web Server in Node17:26
- Outputting HTML and Templates11:43
- Streams and Performance05:11
- Conceptual Aside: APIs and Endpoints02:37
- Outputting JSON06:39
- Routing11:02
- Web Server Checklist02:24
- Conceptual Aside: Packages and Package Managers03:07
- Conceptual Aside: Semantic Versioning (semver)04:46
- npm and the npm registry: Other People's Code04:59
- init, nodemon, and package.json13:02
- npm Global Installation00:11
- init, nodemon, and package.json - Part 215:17
- Using Other People's Code01:43
- Installing Express and Making it Easier to Build a Web Server16:01
- Routes04:32
- Static Files and Middleware14:58
- Templates and Template Engines15:54
- Querystring and Post Parameters18:21
- RESTful APIs and JSON05:21
- Structuring an App13:45
- Conceptual Aside: Relational Databases and SQL03:48
- Node and MySQL09:28
- Conceptual Aside: NoSQL and Documents03:12
- MongoDB and Mongoose10:50
- Web Server Checklist01:07
- MongoDB, Express, AngularJS, and NodeJS06:58
- AngularJS: Managing the Client10:32
- AngularJS: Managing the Client (Part 2)08:09
- AngularJS: Managing the Client (Part 3)19:17
- Conceptual Aside: Angular 1, Angular 2, React, and more…03:06
- Working with The Full Stack (and being a Full Stack Developer) - Part 111:59
- NodeTodo: Software Requirements02:20
- Initial Setup03:38
- Setting up Mongo and Mongoose09:09
- Adding Seed Data09:31
- Creating our API13:33
- Testing our API16:17
- Adding a Front-End in Angular 2 (Part 1)23:22
Online Courses
Learning NodeJS doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of NodeJS related books and take your
YouTube videos
The number of high-quality and free NodeJS video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more