Java for Absolute Beginners
A course on Java for complete beginners to computer programming, for those who want concepts explained in plain English.
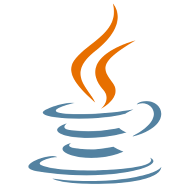
What you will learn?
- The fundamentals of Java
- How to understand and write simple Java programs
- Practice exercises to strengthen your coding knowledge
Your trainer

Nick H
A self-taught programmer with many years of experience using Java and C#. My aim is to teach subjects in a concise and approachable way. I put a lot of effort into conveying topics so that all necessary details are included, to ensure that you can learn and exercise your knowledge with as little frustration as possible.
121 lessons
Easy to follow lectures and videos covering subject details.
8.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Downloading and installing IntelliJ IDEA01:12
- Creating a new Java project03:35
- A Java program is like a recipe05:02
- A recipe's method and Java methods06:16
- The structure of a basic program05:29
- Displaying a message on the screen04:04
- Formatting text using escape sequences04:22
- A glimpse into methods and System.out.println()04:32
- Using packages to organise our classes05:53
- Coding Challenge 1 - HelloWorld00:25
- Introduction to variables05:55
- The int and double data types04:26
- Variable manipulation and integer division04:55
- The char, boolean, and String data types05:58
- Variable naming rules and best practices04:11
- Type casting04:32
- Reading input using the Scanner06:02
- Writing a question and answer program05:56
- The System.out.format() method07:15
- Applying System.out.format()03:27
- Tokens and the nextLine() method04:34
- Coding Challenge 2 - User Input01:16
- Introduction to if-statements05:55
- If, else-if, and else blocks06:24
- Complex conditions with logical operators06:33
- Checking String equality04:30
- Nesting if-statements05:10
- Lexical scope04:23
- The switch statement03:53
- Coding Challenge 3 - If-then Statements01:24
- The while loop05:38
- The for loop05:30
- Break and continue02:20
- The do-while loop06:12
- The modulo operator02:46
- Coding Challenge 4 - Loops01:28
- Introduction to arrays05:16
- Adaptive iteration04:04
- Simple processing using arrays06:48
- Sizes, types, and fortune tellers06:48
- Inputting data and generating a statistic10:01
- Foreach loop02:34
- Coding Challenge 5 - Arrays01:30
- Introduction to methods06:44
- Passing data to methods05:13
- Returning data from methods04:22
- Passing and returning data07:17
- References (part 1)05:20
- References (part 2)04:30
- Class/Static members06:49
- Program walkthrough: Tic-Tac-Toe21:06
- Program walkthrough: Prime Numbers08:02
- Coding Challenge 6 - End of Section Challenges03:27
- Creating the menu and displaying the guest list05:03
- Adding guests05:05
- Deleting guests (part 1)04:44
- Deleting guests (part 2)03:41
- The full code00:26
- Exercise 100:05
- Solution: Exercise 103:49
- Exercise 200:19
- Solution: Exercise 201:40
- Exercise 300:08
- Solution: Exercise 301:20
- Exercise 400:05
- Solution: Exercise 401:50
- Exercise 500:03
- Solution: Exercise 500:49
- Exercise 600:08
- Solution: Exercise 601:56
- Exercise 700:06
- Solution: Exercise 701:24
- Exercise 800:32
- Solution: Exercise 807:29
- Exercise 900:10
- Solution: Exercise 900:42
- Exercise 1000:14
- Solution: Exercise 1002:44
- Exercise 1100:16
- Solution: Exercise 1101:27
- Exercise 1200:04
- Solution: Exercise 1201:14
- Exercise 1300:22
- Solution: Exercise 1302:17
- Exercise 1400:41
- Solution: Exercise 1402:53
- Exercise 1500:07
- Solution: Exercise 1503:15
- Exercise 1600:21
- Solution: Exercise 1605:50
- The final program01:09
- Introduction to OOP06:38
- Data structures06:28
- State and behaviour06:00
- State and behaviour (part 2)06:08
- Encapsulation07:55
- Encapsulation (part 2)08:15
- Constructors07:04
- ArrayList07:57
- Composition (part 1)08:00
- Composition (part 2)08:26
- Enums06:46
- Introduction05:15
- Object Communication Overview02:48
- Initialising Objects03:04
- Searching by ISBN03:31
- Program Walkthrough04:08
- Searching by Keyword in Title05:26
- Checking Books In and Out04:22
- Check-Out Discrepancy03:03
- Inheritance and Access Level Modifiers09:03
- The Bank Account Program05:32
- Constructors and Inheritance07:51
- Method Overriding (Part 1)10:59
- Method Overriding (Part 2)09:25
- Polymorphism09:37
- Abstract Classes04:51
- Interfaces09:04
- Example: Shape Interface04:48
- Type Conversion and the 'instanceof' Operator05:25
- Further Resources00:26
Online Courses
Learning Java doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Java related books and take your
YouTube videos
The number of high-quality and free Java video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more