Design Patterns in Java
Discover the modern implementation of design patterns in Java
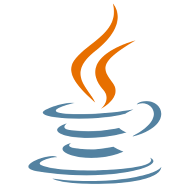
What you will learn?
- Recognize and apply design patterns
- Refactor existing designs to use design patterns
- Reason about applicability and usability of design patterns
Your trainer

Dmitri Nesteruk
Dmitri is a quant, developer, book author and course author. His interests lie in software development and integration practices in the areas of computation, quantitative finance and algorithmic trading. His technological interests include C# and C++ programming as well high-performance computing using technologies such as CUDA and FPGAs. He has been a C# MVP since 2009.
123 lessons
Easy to follow lectures and videos covering subject details.
10.5 hours
This course includes hours of video material. Watch on-demand, anytime, anywhere.
Certificate of Completion
You will earn a Certificate of Completion at the end of this course.
Course curriculum
- Introduction05:11
- Overview00:52
- Single Responsibility Principle (SRP)11:47
- Open-Closed Principle (OCP)18:00
- Liskov Substitution Principle (LSP)09:42
- Interface Segregation Principle (ISP)09:08
- Dependency Inversion Principle (DIP)15:16
- Summary02:26
- Gamma Categorization03:36
- Overview01:43
- Builders in Java06:18
- Builder09:39
- Fluent Builder02:21
- Fluent Builder Inheritance with Recursive Generics09:25
- Faceted Builder09:20
- Builder Coding Exercise1 question
- Summary00:57
- Overview03:08
- Factory Method07:51
- Factory04:27
- Abstract Factory13:02
- Factory Coding Exercise1 question
- Summary01:05
- Overview01:59
- Don't Use Cloneable11:13
- Copy Constructors02:49
- Copy Through Serialization03:56
- Prototype Coding Exercise1 question
- Summary01:08
- Overview02:43
- Basic Singleton03:09
- Serialization Problems07:23
- Static Block Singleton03:46
- Laziness and Thread Safety04:55
- Inner Static Singleton02:30
- Enum Based Singleton07:12
- Monostate03:54
- Multiton06:38
- Testability Issues09:53
- Singleton in Dependency Injection06:52
- Singleton Coding Exercise1 question
- Summary02:15
- Overview02:36
- Vector/Raster Demo08:42
- Adapter Caching07:53
- Adapter Coding Exercise1 question
- Summary01:10
- Overview01:46
- Bridge09:11
- Bridge Coding Exercise1 question
- Summary00:21
- Overview01:36
- Geometric Shapes07:54
- Neural Networks09:07
- Composite Coding Exercise1 question
- Summary01:10
- Overview01:32
- String Decorator04:45
- Dynamic Decorator Composition07:59
- Static Decorator Composition07:23
- Adapter-Decorator08:15
- Decorator Coding Exercise1 question
- Summary01:25
- Overview03:08
- Façade09:22
- Summary01:25
- Overview02:21
- Repeating User Names06:18
- Text Formatting10:19
- Flyweight Coding Exercise1 question
- Summary01:38
- Overview02:07
- Protection Proxy04:26
- Property Proxy06:35
- Dynamic Proxy for Logging09:37
- Proxy vs. Decorator01:18
- Proxy Coding Exercise1 question
- Summary00:46
- Overview03:33
- Method Chain11:06
- Command Query Separation01:28
- Broker Chain14:10
- Chain of Responsibility Coding Exercise1 question
- Summary00:58
- Overview02:11
- Command07:09
- Undo Operations05:30
- Command Coding Exercise1 question
- Summary01:09
- Overview03:15
- Handmade Interpreter: Lexing07:38
- Handmade Interpreter: Parsing09:12
- ANTLR02:44
- Interpreter Coding Exercise1 question
- Summary01:03
- Overview01:34
- Tree Traversal12:07
- Array-Backed Properties09:56
- Iterator Coding Exercise1 question
- Summary01:12
- Overview01:13
- Chat Room10:00
- Reactive Extensions Event Broker07:03
- Mediator Coding Exercise1 question
- Summary01:16
- Overview01:41
- Memento07:22
- Memento for Interop03:45
- Memento Coding Exercise1 question
- Summary01:08
- Overview02:05
- Null Object04:30
- Dynamic Null Object07:28
- Null Object Coding Exercise1 question
- Summary01:45
- Overview01:24
- Observer and Observable08:26
- An Event Class09:31
- The Problem with Dependent Properties02:30
- Observable Coding Exercise1 question
- Summary01:03
- Overview03:08
- Classic Implementation07:34
- Handmade State Machine09:37
- Spring Statemachine10:29
- State Coding Exercise1 question
- Summary01:01
- Overview01:50
- Dynamic Strategy10:04
- Static Strategy04:50
- Strategy Coding Exercise1 question
- Summary00:26
- Overview01:29
- Template Method06:28
- Template Method Coding Exercise1 question
- Summary00:45
- Overview02:47
- Intrusive Visitor05:04
- Reflective Visitor06:02
- Classic Visitor (Double Dispatch)09:33
- Acyclic Visitor09:38
- Visitor Coding Exercise1 question
- Summary01:17
- End of Course00:48
- Bonus Lecture: Other Courses at a Discount00:21
Online Courses
Learning Java doesn't have to be hard. Here is our curated list of recommended online courses that will guide you step-by-step in the learning process.
Learn moreBooks
Are you an avid book reader? Do you prefer paperback, or maybe Kindle version? Take a look at our curated list of Java related books and take your
YouTube videos
The number of high-quality and free Java video tutorials is growing fast. Check this curated list of recommended videos - there is no excuse to stop learning.
Learn more